"cpp using" refers to the `using` declaration in C++, which allows you to introduce a name from a namespace or a type into the current scope, thereby simplifying code references.
Here's a code snippet demonstrating its usage:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl; // 'cout' can be used directly without 'std::'
return 0;
}
What is the "using" Keyword?
The `using` keyword in C++ is a powerful tool that helps manage scope and namespaces, which are essential in writing clean and efficient code. This keyword allows programmers to simplify the use of names within specific namespaces and even create type aliases, enhancing code readability.
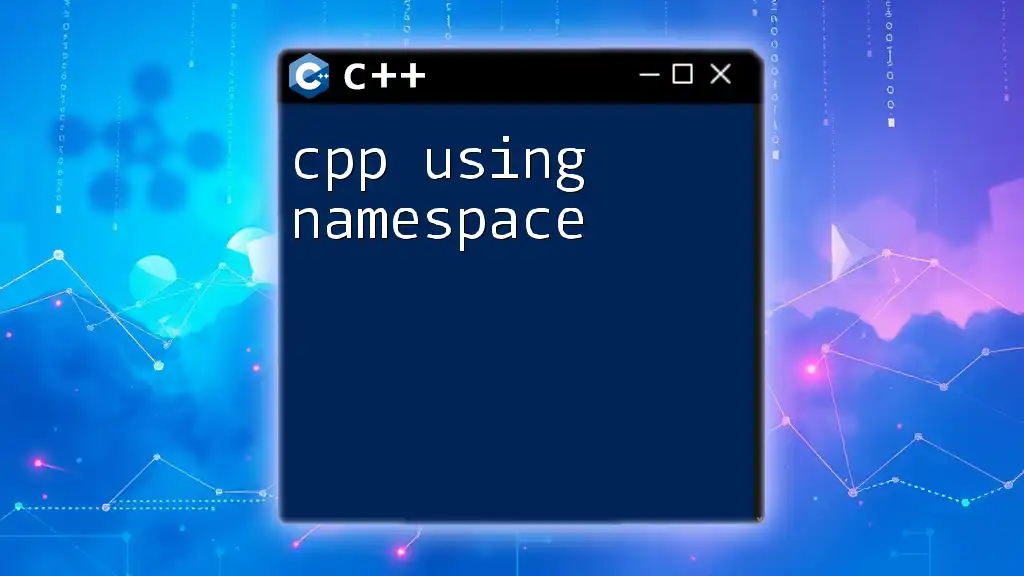
Using Namespaces in C++
What is a Namespace?
A namespace is a declarative region that provides a scope to the identifiers (the names of types, functions, variables, etc.) inside it. This helps in grouping entities of similar functionality and avoids name conflicts. C++ offers a flexible way to handle these namespaces, particularly useful in larger projects where naming collisions might occur.
How to Use Namespaces
The `using` directive allows you to access a namespace without prefixing its members with the namespace's name.
The basic syntax of the `using` directive looks like this:
using namespace std;
In this example, `std` is a namespace that contains functionalities provided by the C++ Standard Library. By including this directive, you can use standard library features (like `cout` or `cin`) without the need to prefix them with `std::`.
Pros and Cons of Using the "using" Directive
Using the `using` directive has distinct pros and cons:
Pros:
- Simplifies Code Readability: By eliminating the need for a namespace prefix, code becomes cleaner and easier to read.
- Reduces Qualified Names: Helps developers avoid verbosity when frequently accessing functions or types from a specific namespace.
Cons:
- Potential for Name Clashes: If two namespaces contain entities with the same name, it can lead to ambiguities.
- Impact on Code Maintainability: Overusing `using` can make it difficult to track where each name originates, reducing code clarity over time.
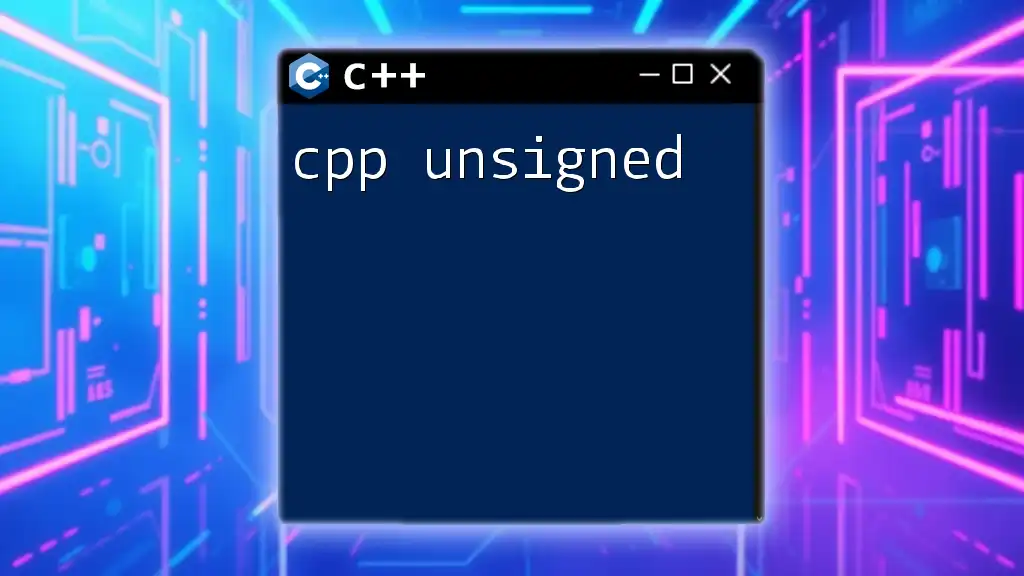
Declaring Specific Entities with "using"
Using a Specific Name from a Namespace
Instead of bringing an entire namespace into scope, you can declare only specific entities. This is more precise and lowers the risk of naming conflicts.
For example:
using std::cout;
using std::cin;
Here, only `cout` and `cin` from the `std` namespace are brought into the current scope. This means you can now use `cout` and `cin` directly without the `std::` prefix, while keeping the rest of the namespace excluded.
Combining Using Declarations
You can also voice multiple entities from a single namespace, which helps in maintaining clarity while accessing frequently used elements:
using std::cout;
using std::cin;
using std::endl;
This technique makes it easier and neater to access items while keeping the code concise. It’s recommended when you frequently interact with the same types or functions from a namespace.
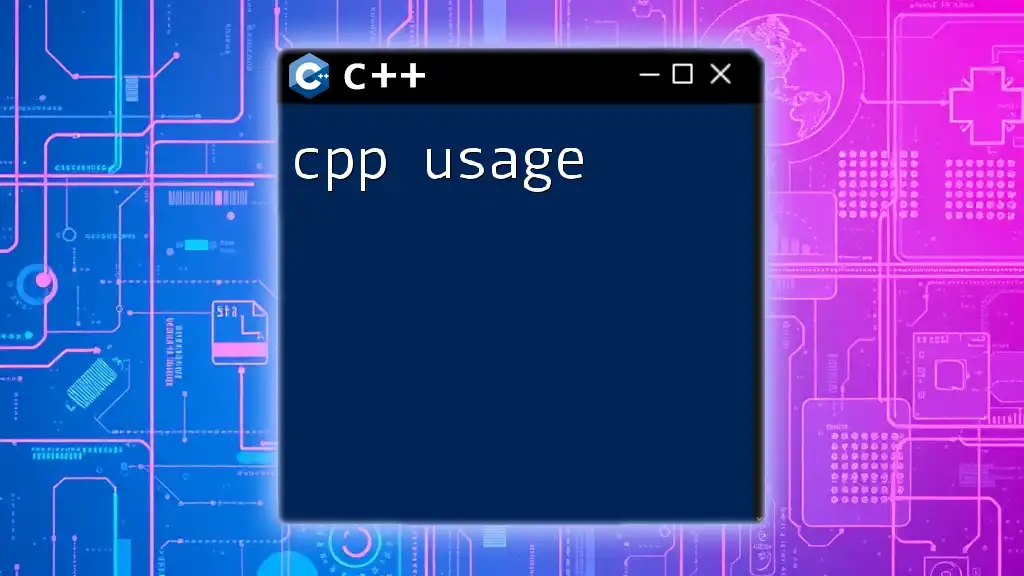
Advanced Usage of "using" Keyword
Type Aliases with "using"
C++ allows you to create type aliases with the `using` keyword. This is particularly beneficial for enhancing code clarity or simplifying complex type definitions.
For instance:
using IntPtr = int*;
Here, `IntPtr` becomes an alias for `int*`, making it clearer when you declare pointer variables. This can lead to greater maintainability, as the meaning behind your types becomes more explicit.
Using in Template Programming
The `using` keyword can be exceptionally useful in template programming, where type definitions can become cumbersome.
Consider:
template<typename T>
using Vec = std::vector<T>;
In this snippet, `Vec` is now a shorthand for `std::vector<T>`. This enhances code readability, and if you ever need to change the container type, you only need to replace it in one place.
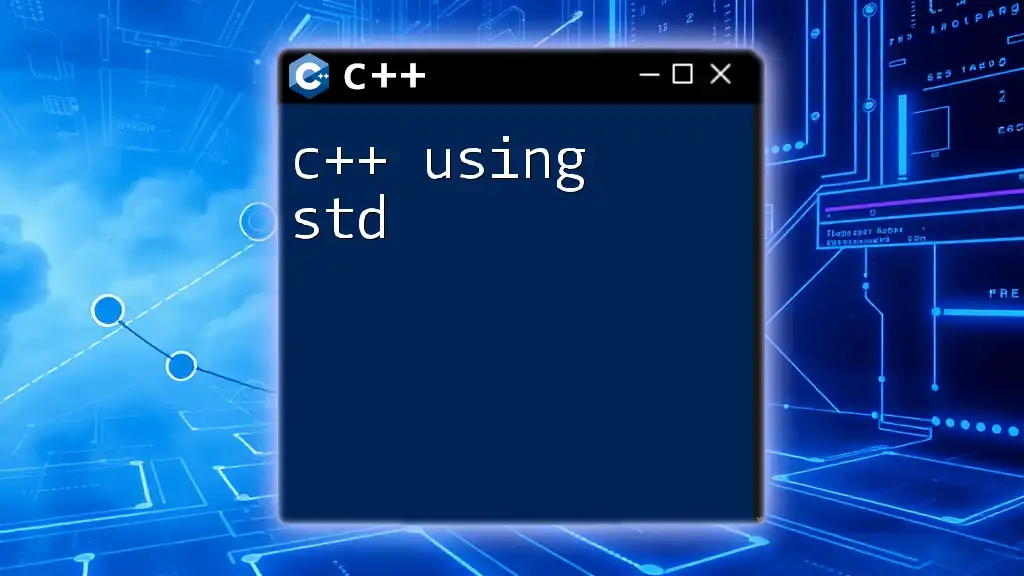
Best Practices for Using "using" in C++
To maximize the effectiveness of the `using` directive, consider the following best practices:
- Limit Scope: Use `using` only in file or function scopes to prevent cluttering the global namespace.
- Prefer Specific Aliases: Opt for specific entity declarations over broad namespace inclusions to mitigate the risk of clashes.
- Be Descriptive: When creating type aliases, choose clear and descriptive names that convey the intent of the type, such as `IndexType` instead of a generic `T`.
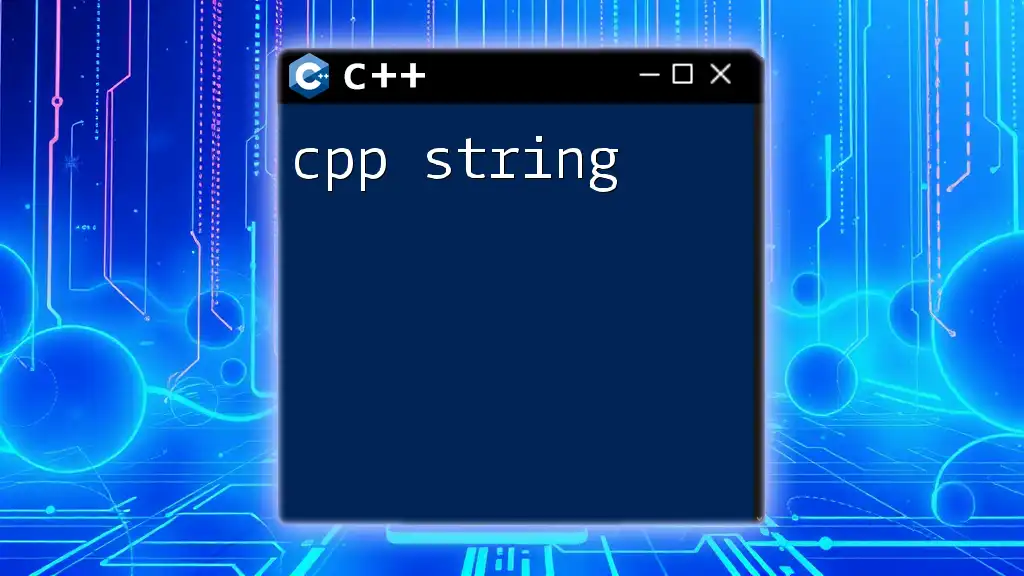
Common Mistakes to Avoid
Overusing the "using" Directive
One common pitfall is overusing the `using` directive, especially at global scope. Doing so can lead to ambiguous names and hinder code tracking. It's generally best to limit its use to local or specific contexts where conflicts are minimized.
Not Understanding Namespace Scope
Mismanagement of namespace scope can result in unexpected behaviors or name conflicts. Always be aware of which namespaces are in scope and strive to avoid bringing in entire namespaces that are unnecessary for your implementation.
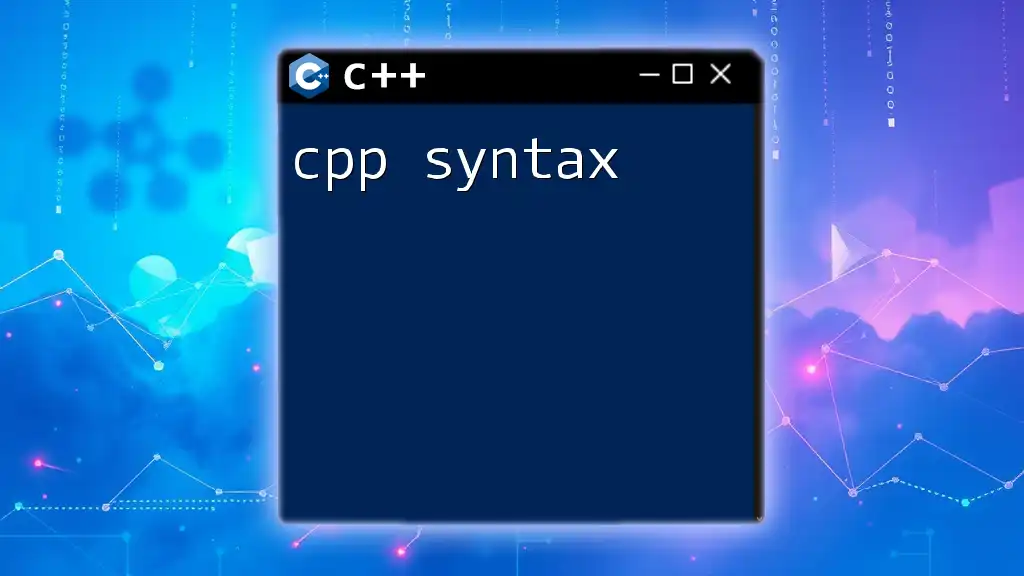
Conclusion
By mastering the `using` keyword in C++, you can create more organized, readable, and maintainable code. Whether you are dealing with namespaces or type aliases, understanding how to effectively use `using` will enhance your coding proficiency. Embrace its capabilities in your coding practices, and continuously seek opportunities to improve your C++ skill set.
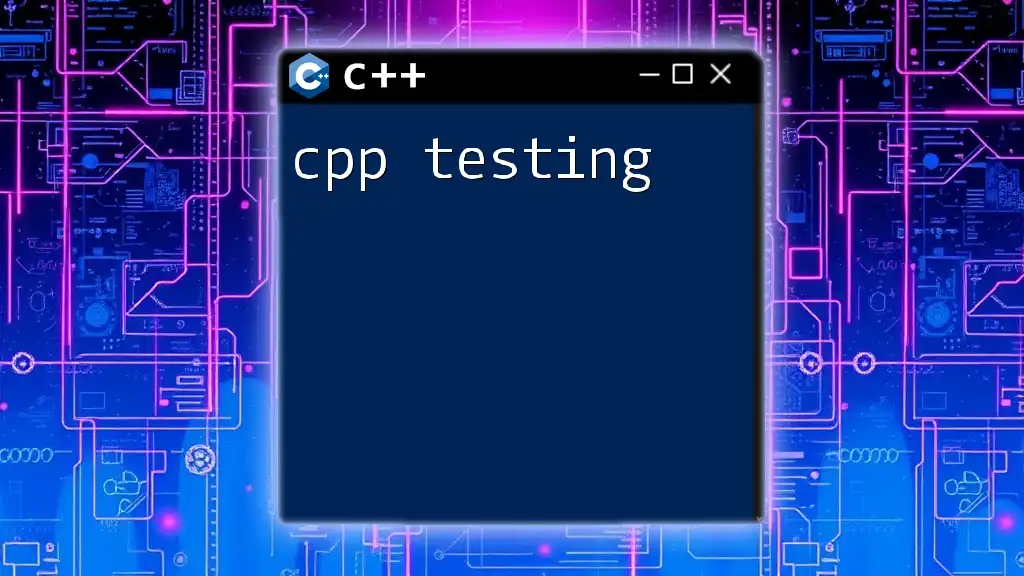
Additional Resources
To further your understanding of C++ and the effective use of the `using` keyword, consider exploring textbooks, online courses, and the rich documentation provided by the C++ community. Engage in forums and coding groups to collaborate with others who are keen on improving their C++ skills.