C++ (often referred to as cpp) is a powerful programming language that enables developers to write efficient and flexible code, allowing for lower-level manipulation and high-performance applications.
Here's a concise example demonstrating how to use a simple C++ command to print "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Programming Language
What is C++?
C++ is a powerful general-purpose programming language that was developed by Bjarne Stroustrup at Bell Labs in the early 1980s. It is an extension of the C language with added features that support object-oriented programming (OOP) and generic programming, making it a versatile choice for a variety of programming tasks. The evolution of C++ includes major versions, leading to its widespread adoption in software development.
Key Features of C++
C++ stands out due to several key features that make it suitable for high-performance applications.
-
Object-Oriented Programming (OOP): C++ supports the fundamental concepts of OOP including:
- Abstraction, which allows focusing on the essential features while hiding the unnecessary details.
- Encapsulation, which is the bundling of data with the functions that operate on that data.
- Inheritance, enabling new classes to inherit features from existing classes, promoting code reuse.
- Polymorphism, which allows functions to use objects of different types at runtime.
-
Standard Template Library (STL): The STL provides a collection of algorithms and data structures, including:
- Containers like vectors, lists, and maps that store collections of data.
- Algorithms that operate on these containers, allowing for actions such as sorting and searching.
- Iterators that provide a way to navigate through the data structured in the containers.
-
Performance and Memory Management: C++ offers greater control over system resources:
- It allows low-level resource manipulation, making it highly efficient for performance-critical applications.
- C++ enables manual memory management through pointers, which can increase performance but requires careful management to avoid memory leaks.
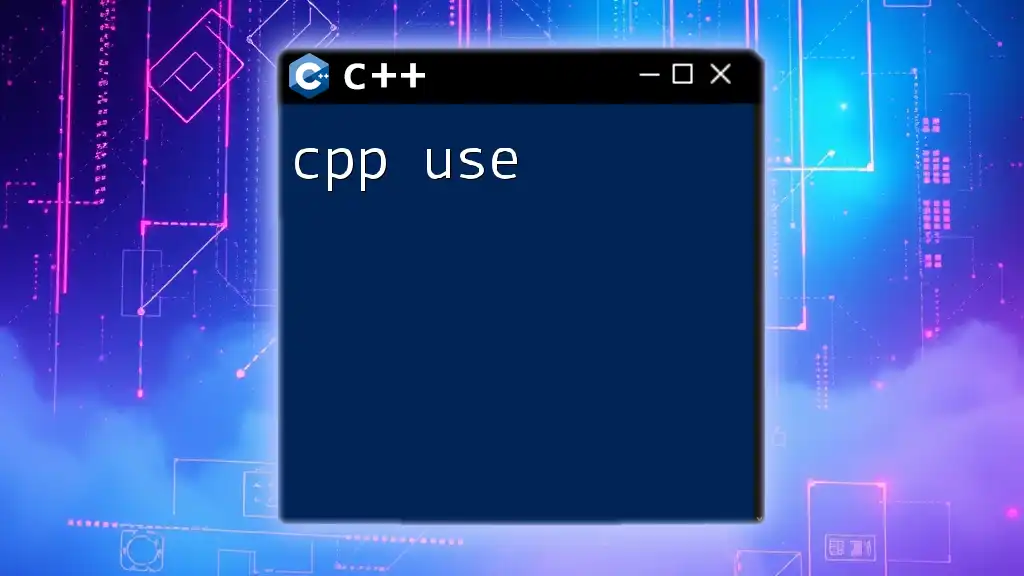
Common Uses of C++ Programming Language
Software Development
C++ is widely used in software development across different platforms. High-profile applications such as Microsoft Office and Adobe's Creative Suite benefit from C++’s performance and versatility. A simple C++ application to demonstrate its syntax is as follows:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, C++ World!" << endl;
return 0;
}
Game Development
C++ is a favorite in the gaming industry due to its capability to deliver high performance and speed, essential for rendering complex graphics in real time. Game engines like Unreal Engine and even parts of Unity are written in C++. These engines rely on C++ for crafting interactive experiences, allowing developers to harness powerful hardware features efficiently.
Embedded Systems
Another significant usage of C++ is in embedded systems, where developers write firmware for devices like automotive control systems and IoT devices. The low-level operations and efficiency of C++ make it perfect for the constraints of embedded systems. An example of a basic class responsible for managing a light in an embedded system may look like this:
class Light {
public:
void turnOn() { /* code to turn on */ }
void turnOff() { /* code to turn off */ }
};
Mobile and Web Applications
C++ is increasingly popular in the development of mobile applications, especially cross-platform solutions. Frameworks such as Qt enable developers to create applications that run on multiple operating systems seamlessly. Web applications, particularly performance-critical ones like game servers and high-performance trading platforms, also utilize C++ for its efficiency.
High-Performance Applications
C++ is often used in financial systems where performance is crucial; applications for stock trading rely on rapid data processing. A simple example of a performance-intensive algorithm using C++ is demonstrated below:
#include <vector>
#include <algorithm>
int maxPerformance(std::vector<int> data) {
return *std::max_element(data.begin(), data.end());
}
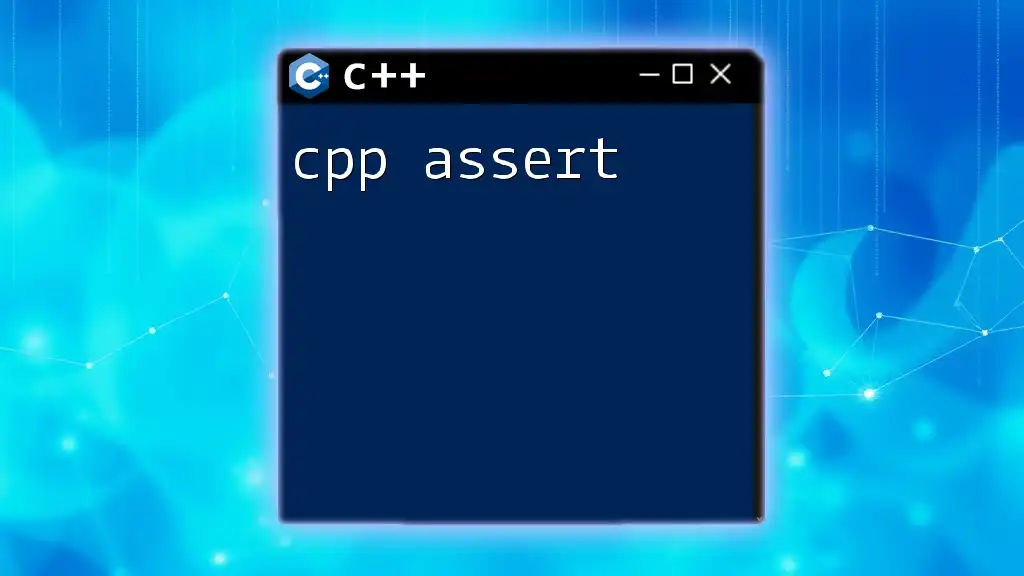
Key C++ Concepts for Effective Usage
Variables and Data Types
Understanding variables and data types is fundamental in C++. C++ supports a variety of data types such as `int`, `float`, `char`, and `string`, each serving different purposes. Selecting the right data type can significantly impact memory usage and performance. Here’s how you can define variables in C++:
int number = 10;
float decimalValue = 10.5f;
char character = 'A';
string text = "Hello, C++!";
Control Structures
C++ offers a range of control structures that dictate the flow of the program. Decision constructs like `if` and `switch` allow branching, while looping mechanisms like `for`, `while`, and `do-while` enable repeated execution of code blocks. A simple code snippet for a loop is illustrated here:
for (int i = 0; i < 5; ++i) {
cout << "Iteration " << i << endl;
}
Functions and Scope
Functions in C++ promote code reuse and enhance program organization. By using function overloading and templates, developers can create more versatile and robust applications. An example comparing pass-by-value and pass-by-reference reveals important nuances in function parameters:
void modifyValue(int value) {
value = 100; // This won't affect the original variable
}
void modifyReference(int& value) {
value = 100; // This will modify the original variable
}
Classes and Objects
Classes form the backbone of C++ OOP, encapsulating data and functions. They lead to the creation of objects, which are instances of classes. Constructors and destructors manage resources efficiently. Here’s a basic implementation showcasing constructors and destructors:
class SampleClass {
public:
SampleClass() { cout << "Constructor called!" << endl; }
~SampleClass() { cout << "Destructor called!" << endl; }
};
Exception Handling
Exception handling in C++ allows developers to manage errors gracefully. Using `try-catch` blocks ensures that the program can handle runtime errors robustly. Here’s an example demonstrating the exception handling mechanism:
try {
throw std::runtime_error("An error occurred!");
} catch (const std::exception& e) {
cout << e.what() << endl;
}
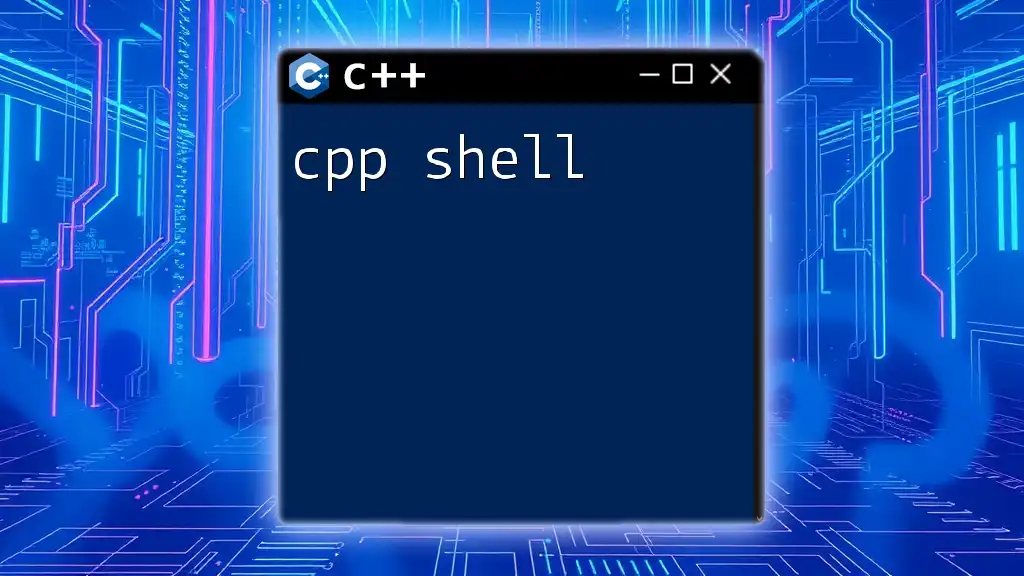
Resources for Learning C++ Fast and Effectively
Online Courses and Tutorials
Numerous platforms offer C++ programming courses to facilitate rapid learning. Websites like Coursera, Udacity, and Udemy provide structured learning experiences with hands-on coding. Additionally, platforms such as Codecademy and LeetCode are excellent for practice and real-time coding challenges.
Books and Reference Materials
For a deeper understanding, several books are regarded as essential reading for C++ developers. Notable titles include "Effective C++" by Scott Meyers and "C++ Primer" by Lippman. Online resources such as cppreference.com and Cplusplus.com serve as invaluable documentation references for syntax and function usage.
Community and Forum Engagement
Engaging with the programming community can greatly enhance the learning experience. Platforms like Stack Overflow and Reddit provide spaces for discussion, question-answering, and knowledge sharing. Joining dedicated C++ groups can foster learning through collaborative problem-solving and project development.
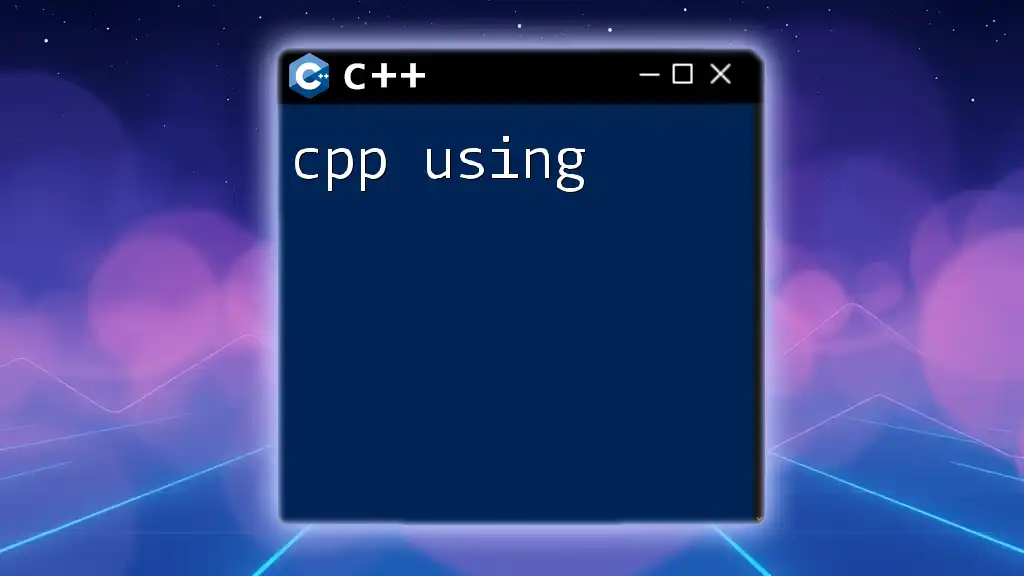
Conclusion
To recap, C++ usage spans various domains including software development, game programming, embedded systems, and high-performance applications. Learning C++ can empower developers to create efficient and powerful applications across a multitude of settings. We encourage all readers to take the next step by applying these concepts practically, exploring resources, and sharing experiences in the journey of mastering C++. Dive into the exciting world of C++ programming, and start building your real-world projects today!