C++ (cpp) is a powerful programming language commonly used for system/software development that emphasizes performance and efficiency through commands and constructs that enable low-level memory manipulation.
Here’s a simple example demonstrating how to output "Hello, World!" using `cpp`:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Commands
What are C++ Commands?
C++ commands are the building blocks of C++ programming, guiding the execution of the program. They encompass various programming constructs, such as control structures, functions, and data handling techniques. Understanding how to effectively use these commands is crucial for a successful programming experience.
Setting Up Your C++ Environment
Choosing a Development Environment
Before diving into C++, it is vital to set up a suitable development environment. Popular choices include:
- Visual Studio: Rich feature set especially for Windows users.
- Code::Blocks: A lightweight, customizable IDE.
- Eclipse: Versatile and well-suited for larger projects.
- CLion: A powerful IDE from JetBrains focused on C/C++.
Installation Steps
- Download a Compiler: Choose from GCC, Clang, or any pre-packaged IDE that includes a compiler.
- Install the IDE: Follow installation instructions specific to the chosen IDE.
- Set Up Environment Variables (if necessary): Ensure your compiler is accessible via command line.
Running Your First C++ Command
Now, let's run a simple "Hello, World!" program, which is a traditional first step in learning any programming language. Create a new C++ file and insert the following code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
When compiled and executed, this code outputs "Hello, World!" to the console, demonstrating how C++ commands work together.
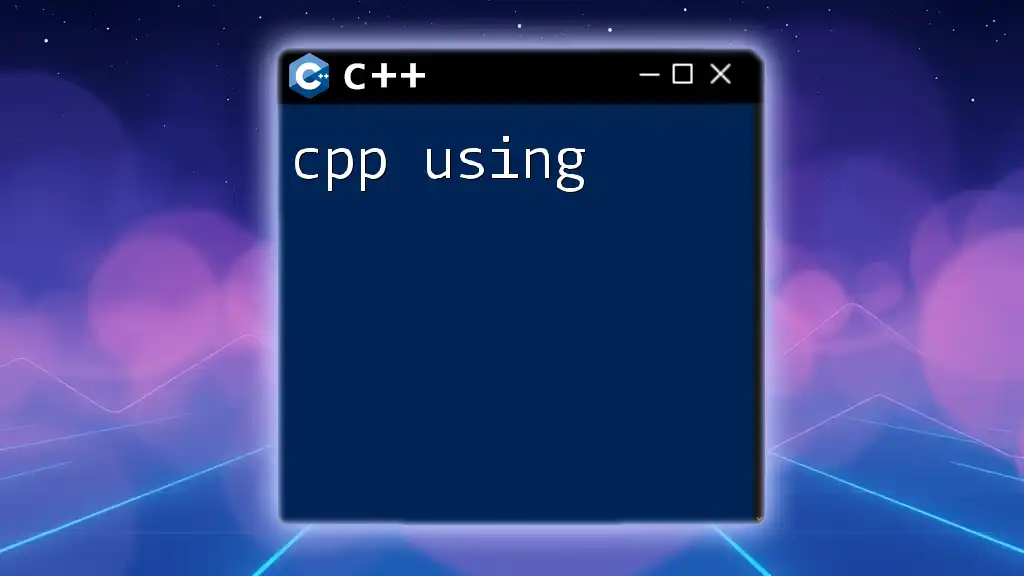
Fundamental C++ Commands
Data Types and Variables
Understanding data types and how to declare variables is fundamental in C++. Common data types include:
- int: Integer values.
- float: Floating-point numbers.
- char: Character values.
- string: A sequence of characters.
For example, you can declare and initialize variables as follows:
int age = 30;
float temperature = 36.5;
char grade = 'A';
Such declarations allow you to store and manipulate data efficiently.
Control Structures
Control structures direct the flow of execution in a C++ program.
Conditional Statements
C++ uses `if`, `else if`, and `else` statements to perform conditional logic. Here is a basic example:
if (age >= 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
This snippet checks the value of the variable `age` and prints whether the individual is an adult or minor.
Loops
Loops, such as `for`, `while`, and `do-while`, enable repeated execution of code:
for (int i = 0; i < 5; i++) {
std::cout << "Count: " << i << std::endl;
}
In this for loop, the code will output integers from 0 to 4.
Functions
Functions are reusable blocks of code that perform specific tasks. They significantly enhance the organization of your programs. Here’s how to define a simple function and call it:
void greet() {
std::cout << "Welcome to C++!" << std::endl;
}
int main() {
greet();
return 0;
}
The `greet` function encapsulates the greeting logic, enabling easy reuse and readability.
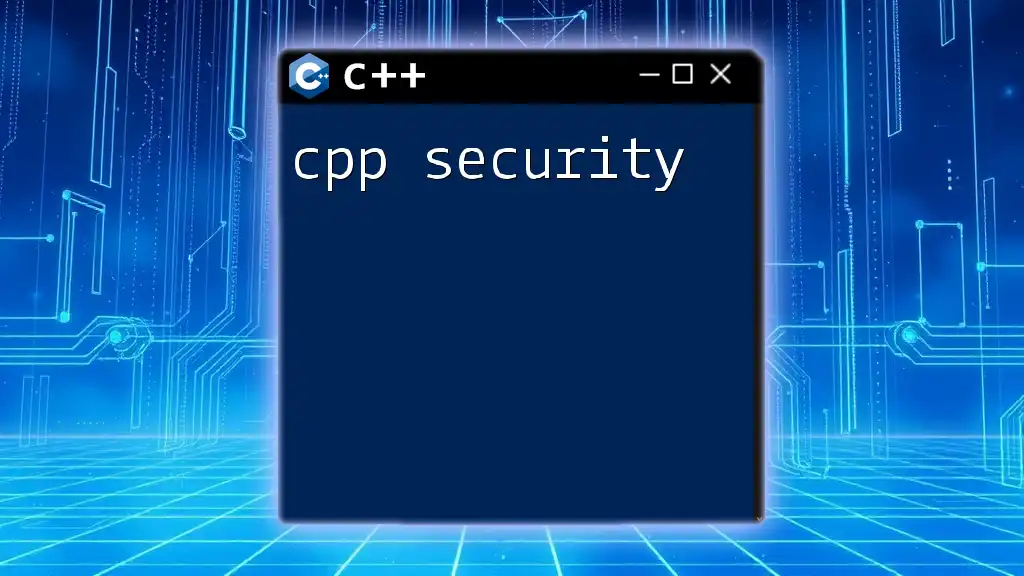
Advanced C++ Commands
Pointers and References
Pointers are variables that store memory addresses, facilitating dynamic memory usage.
For example:
int var = 5;
int* ptr = &var;
std::cout << *ptr; // Prints 5
In this snippet, `ptr` points to the address of `var`, and dereferencing `ptr` retrieves the value stored at that address.
Classes and Objects
C++ supports Object-Oriented Programming (OOP), allowing for the creation of classes and objects. This approach enhances modularity and code reuse. Here’s a typical class example:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
In this case, the `Dog` class has a method `bark`. An object of this class (`myDog`) can invoke the method, showcasing the principles of encapsulation and abstraction.
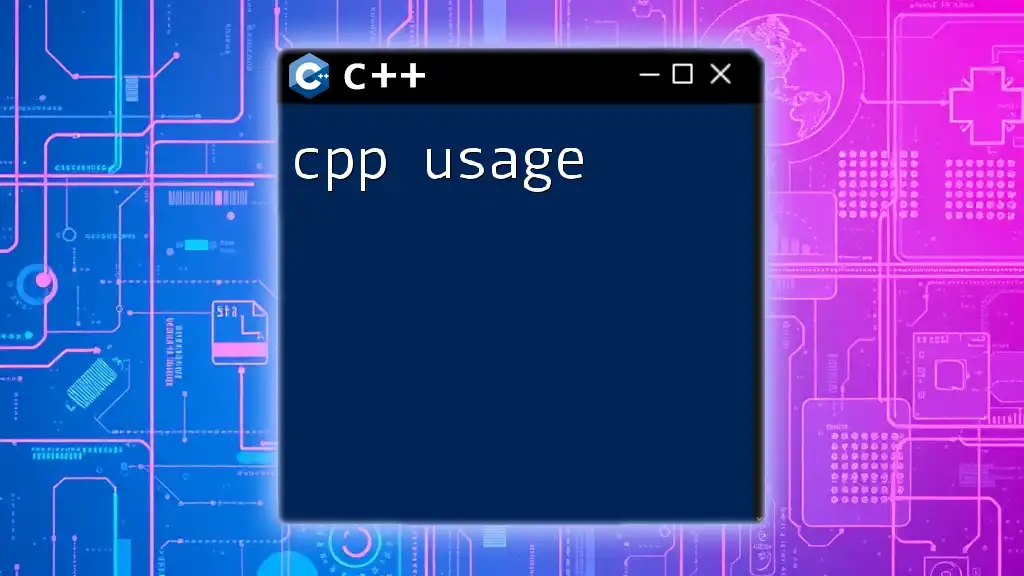
Best Practices for Using C++ Commands
Code Readability
Writing clean and readable code is paramount. Use meaningful variable names that convey purpose. For example, instead of using `x`, utilize `totalSum` for clarity. Properly indent your code and adhere to consistent formatting.
Commenting Your Code
Comments help explain complex sections and make code easier to maintain. Follow this structure to comment your C++ code effectively:
// This function adds two numbers
int add(int a, int b) {
return a + b;
}
Use comments to provide context, especially for intricate logic or unique implementations.
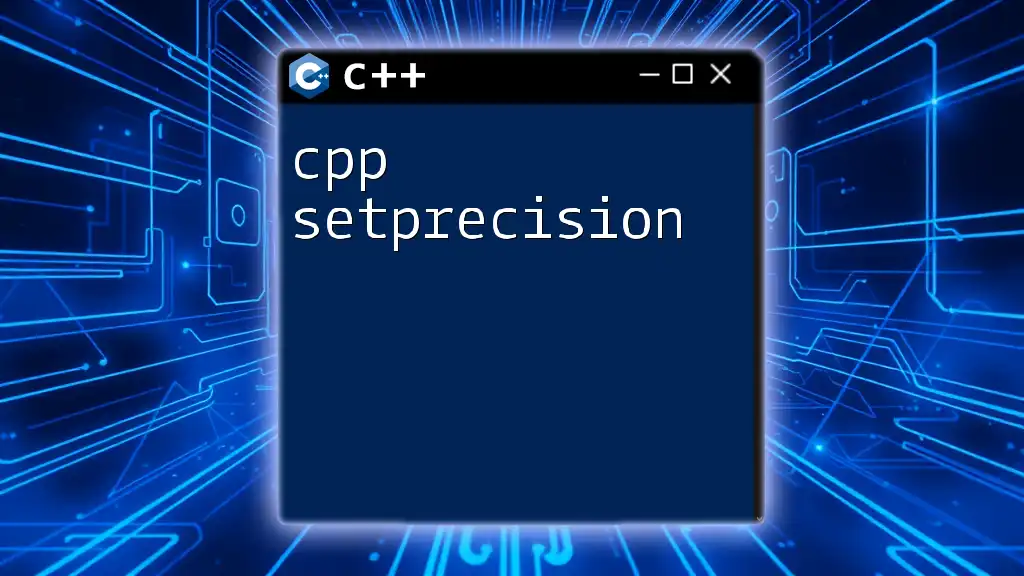
Common Pitfalls and How to Avoid Them
Memory Leaks
Be conscious of memory management when using pointers. Failure to deallocate memory leads to memory leaks that can slow down or crash applications. Always ensure that you free up any dynamically allocated memory.
Undefined Behavior
Undefined behavior can occur when using uninitialized variables or accessing out-of-bounds array indices. Always initialize variables and check array boundaries before accessing them.
Debugging Techniques
Debugging is an essential part of programming. Use available tools such as GDB (GNU Debugger) or integrated debugging features in your IDE. Familiarize yourself with breakpoints, watch variables, and step-through execution to identify and fix errors efficiently.
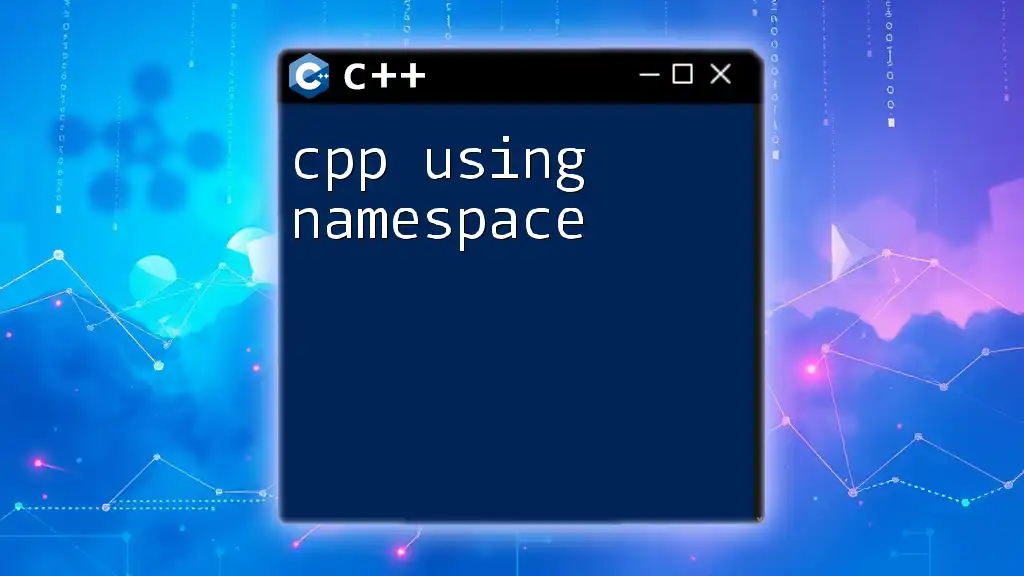
Conclusion
In this comprehensive guide, we've explored the essential aspects of C++ use, covering fundamental commands, advanced concepts, best practices, and common pitfalls. Mastery of these principles requires practice and experimentation. As you continue to learn and apply your knowledge, you will find that the ability to use C++ effectively opens up numerous opportunities in software development. Don't hesitate to try out different commands and see how they can be employed in your coding projects!
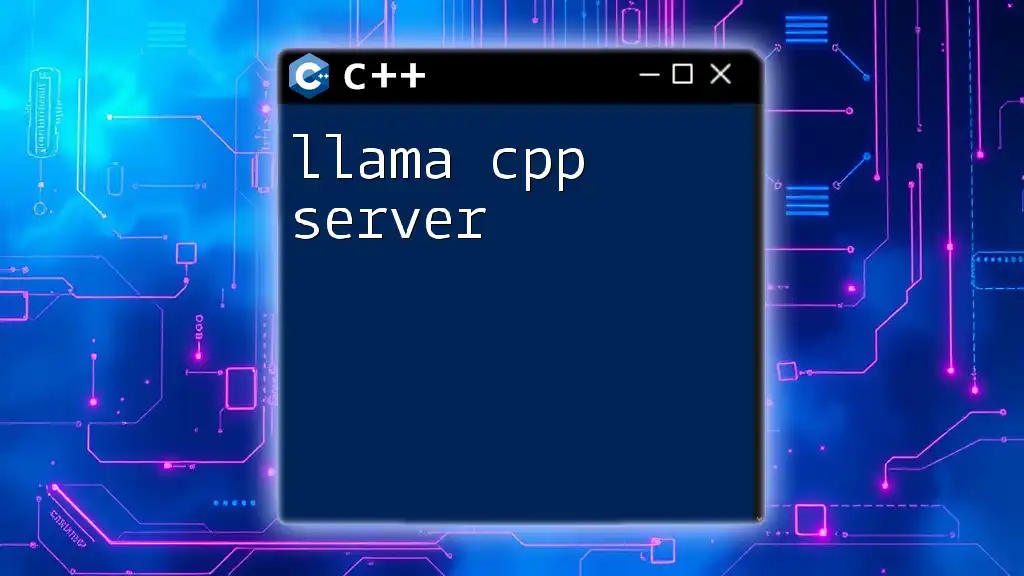
Additional Resources
To further your understanding and skill set, explore recommended books and online courses tailored for different learning stages. Engaging with online communities and forums can also provide valuable support and insights from fellow programmers, enhancing your C++ journey.