A `struct` in C++ is a user-defined data type that groups related variables under a single name, allowing for the creation of complex data structures.
struct Person {
std::string name;
int age;
};
Understanding Structs in C++
What is a Struct?
A struct in C++ is a user-defined data type that allows you to group related variables into a single type. It serves as a blueprint for creating objects, each representing a collection of different data types. Structs are especially useful for organizing complex data in a logical way, which improves both readability and maintenance of the code.
Structs differ from classes but share similarities. The primary difference is that by default, all members of a struct are public, whereas, in classes, members are private unless specified otherwise. Thus, structs are often seen as simpler data holders with a focus on public access.
Why Use Structs?
The use of structs offers several advantages:
- Simplification of Code: They allow you to define a single variable to represent complex data, reducing the number of variables you need to manage individually.
- Enhanced Readability: Structs make it clear which pieces of data are related, enhancing the overall structure of your code. This makes it easier for others (and yourself) to understand once you return to it after some time.
- Data Grouping: Related information can be kept together, facilitating better data handling and manipulation.

Defining a Struct
Basic Syntax
Defining a struct in C++ is straightforward. Below is the basic syntax for declaring a struct:
struct StructName {
DataType member1;
DataType member2;
};
This format variables of any datatype as members of the struct.
Example: Defining a Simple Struct
Let’s define a simple struct called `Person` that holds a name and an age:
struct Person {
std::string name;
int age;
};
In this example, `name` is a member of type `std::string`, and `age` is of type `int`. This struct now allows us to create `Person` variables to hold both name and age together.

Creating Struct Instances
Instantiation of Structs
Creating an instance of a struct allows you to utilize the data defined within the struct. Using the previous example, you can create an instance of the `Person` struct as shown here:
Person person1;
person1.name = "Alice";
person1.age = 30;
In this case, we created an instance `person1` and assigned values to its members.
Initializing Struct Instances
Struct instances can also be initialized in various convenient ways. One common method is direct initialization:
Person person2 = {"Bob", 25};
Moreover, C++ allows you to use constructor-like initialization:
Person person3{"Charlie", 28};
Both of these methods provide a clean way to create instances filled with initial values.

Accessing Struct Members
Dot Operator Method
To access the members of a struct, you use the dot operator. For example, to display the values of `person1`, you can use:
std::cout << person1.name << " is " << person1.age << " years old.";
This prints out the name and age of `person1` with a clear message.
Pointer to Struct Members
You can also access members through pointers. Here's how you can do it:
Person* ptr = &person1;
std::cout << ptr->name; // Using the arrow operator
The arrow operator (`->`) is used here for dereferencing the pointer and accessing a member directly.

Structs with Functions
Passing Structs to Functions
Structs can be passed to functions, providing a convenient way to manipulate grouped data. Below is a function that accepts a `Person` struct:
void displayPerson(const Person& p) {
std::cout << p.name << " is " << p.age << " years old.";
}
In this function, `const Person& p` ensures that the struct is passed by reference without copying, improving performance.
Returning Structs from Functions
You can return structs from a function as well. Here is an example of a function that creates and returns a `Person` struct:
Person createPerson(std::string name, int age) {
return Person{name, age};
}
This allows for a straightforward way to generate and obtain struct instances.

Advanced Struct Features
Nested Structs
You can define structs within other structs, allowing for a more organized data structure. Consider the following example with an `Address` struct nested inside an `Employee` struct:
struct Address {
std::string city;
std::string country;
};
struct Employee {
Person details;
Address address;
};
This approach is useful for grouping related data together, such as storing employee details alongside their addresses.
Structs with Arrays
Structs can also contain arrays, making them versatile tools for managing collections of related data. Here is how you could define a struct with an array of team members:
struct Team {
std::string members[5];
};
This struct allows you to manage a fixed group of team members in a single entity.
Using Structs with Standard Library Containers
C++’s STL containers, like vectors, allow storing structs in a dynamic array format. Here’s how you can declare a vector of `Person` structs:
#include <vector>
std::vector<Person> team;
team.push_back({"David", 35});
Using vectors for dynamic data management adds flexibility to how you handle collections of structs.
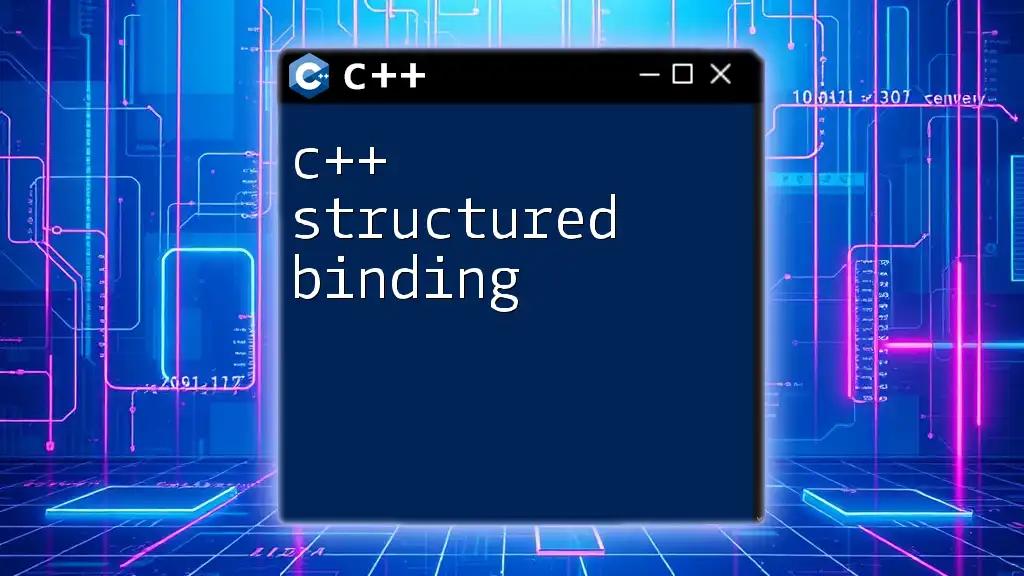
Conclusion
In summary, cpp struct provides a powerful way to manage and organize data in your C++ programs. With the ability to define your own data types, group related variables, and encapsulate complex data structures, structs significantly improve code readability and maintainability.
I encourage you to practice using structs in your C++ projects. They are essential for writing clean, efficient, and understandable code. Stay tuned for more tips and guides on mastering C++ commands!