The `sqrt` function in C++ computes the square root of a given number, which is part of the `<cmath>` library.
#include <iostream>
#include <cmath>
int main() {
double number = 16.0;
double result = sqrt(number);
std::cout << "The square root of " << number << " is " << result << std::endl;
return 0;
}
Understanding the sqrt Function in C++
What is the sqrt Function in C++?
The cpp sqrt function is a built-in mathematical function that calculates the square root of a given number. It serves a critical role in various programming scenarios, particularly in mathematics, physics, and engineering applications.
Including the Required Header File
To use the cpp sqrt function, including the proper header file is essential. The function is defined in the `<cmath>` library, which must be included at the beginning of your C++ program.
Here’s how you should do it:
#include <cmath> // Required for sqrt function
This inclusion allows your code to access all the mathematical functions provided by the library, including sqrt.

Syntax of the C++ sqrt Function
Understanding the Syntax
The syntax for using the cpp sqrt function is straightforward. It takes a single parameter and returns the square root of that number as follows:
double sqrt(double x);
Parameters and Return Type
- Parameter: `double x` – The number for which you want to find the square root. It's important to note that `x` should be a non-negative number.
- Return Type: The function returns a value of type `double`, representing the square root of the number provided.
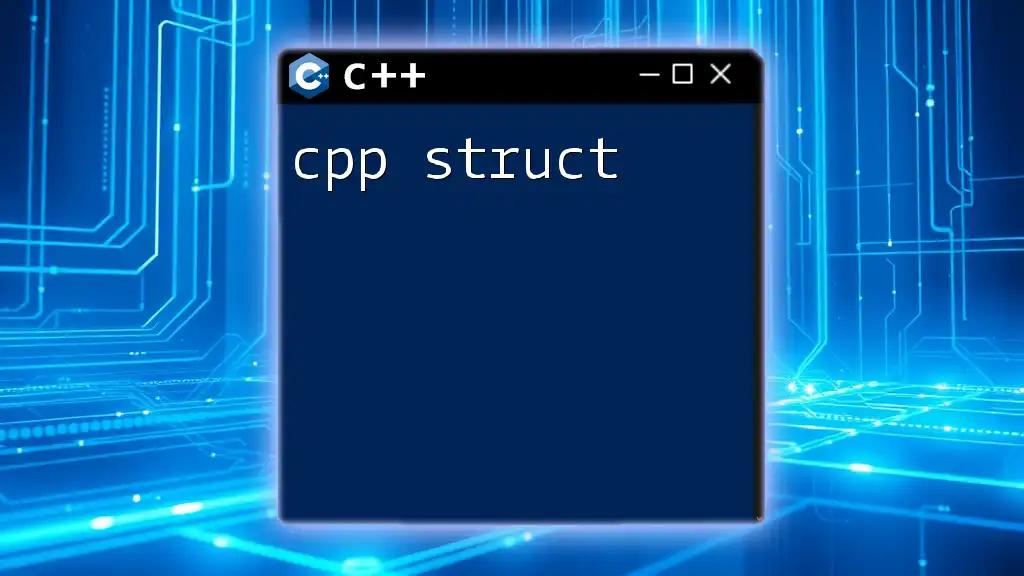
How to Use sqrt in C++
Basic Usage of sqrt in C++
The cpp sqrt function can be used for basic calculations involving square roots. Here’s a simple example that demonstrates this:
#include <iostream>
#include <cmath>
int main() {
double number = 25.0;
double result = sqrt(number);
std::cout << "The square root of " << number << " is " << result << std::endl;
return 0;
}
In this code, the program calculates the square root of 25. The output will be:
The square root of 25 is 5
This illustrates an essential application of the cpp sqrt function in C++ programming.
Handling Negative Inputs
The cpp sqrt function isn't designed to handle negative numbers, as the square root of a negative number is not defined in the realm of real numbers. If you call sqrt with a negative argument, it may result in an undefined behavior or a domain error.
You can prevent this scenario by validating the input. Here’s an example:
double negative_num = -25.0;
if (negative_num < 0) {
std::cout << "Error: Cannot calculate the square root of a negative number." << std::endl;
} else {
double result = sqrt(negative_num);
std::cout << "The square root of " << negative_num << " is " << result << std::endl;
}
This code checks if the input is negative and informs the user instead of attempting to compute the square root.
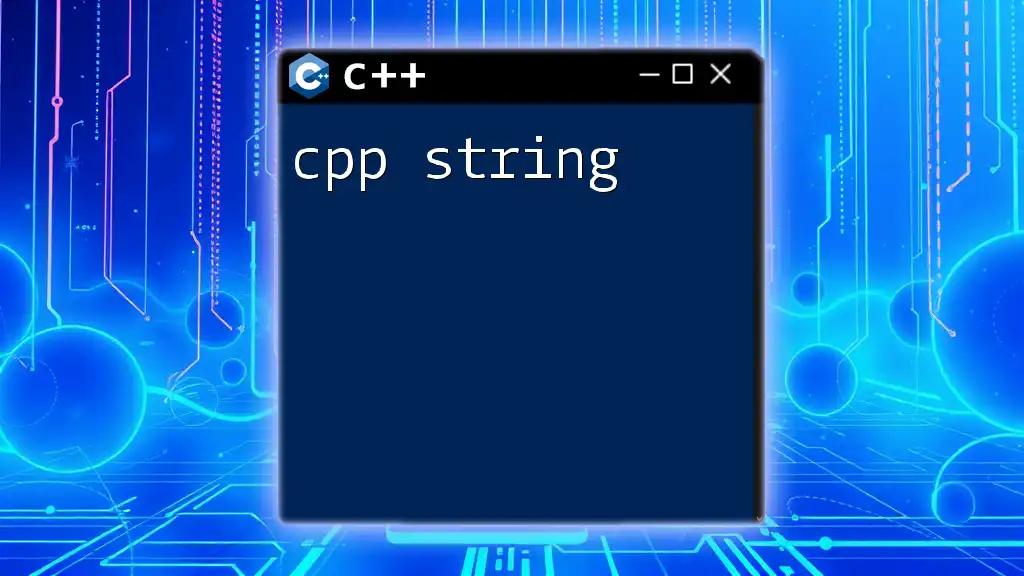
Advanced Usage of the C++ sqrt Function
Using sqrt with Different Data Types
The cpp sqrt function can also handle different data types, such as `float` and `int`. The function will internally convert the argument to a `double`, which provides consistency in calculations. Here’s an example that showcases this flexibility:
int intNum = 16;
float floatNum = 9.0f;
std::cout << "Square root of " << intNum << " is " << sqrt(intNum) << std::endl;
std::cout << "Square root of " << floatNum << " is " << sqrt(floatNum) << std::endl;
Performance Considerations
In high-performance applications, the speed of computing the square root may become a concern. The standard cpp sqrt function is optimized for general use, but if you find that performance is an issue, there are alternatives, such as using fast approximation algorithms. These could involve techniques like Newton's method for square root calculation.

Common Mistakes When Using sqrt in C++
Floating Point Precision Issues
A common pitfall when using the cpp sqrt function is dealing with floating-point precision. Due to how floating points are represented in memory, small inaccuracies can occur, especially in large computations.
Forgetting to Include the cmath Library
An often-overlooked mistake is failing to include the `<cmath>` library, leading to compilation errors. Ensure you always begin your C++ files that use mathematical functions with the correct header:
#include <cmath>
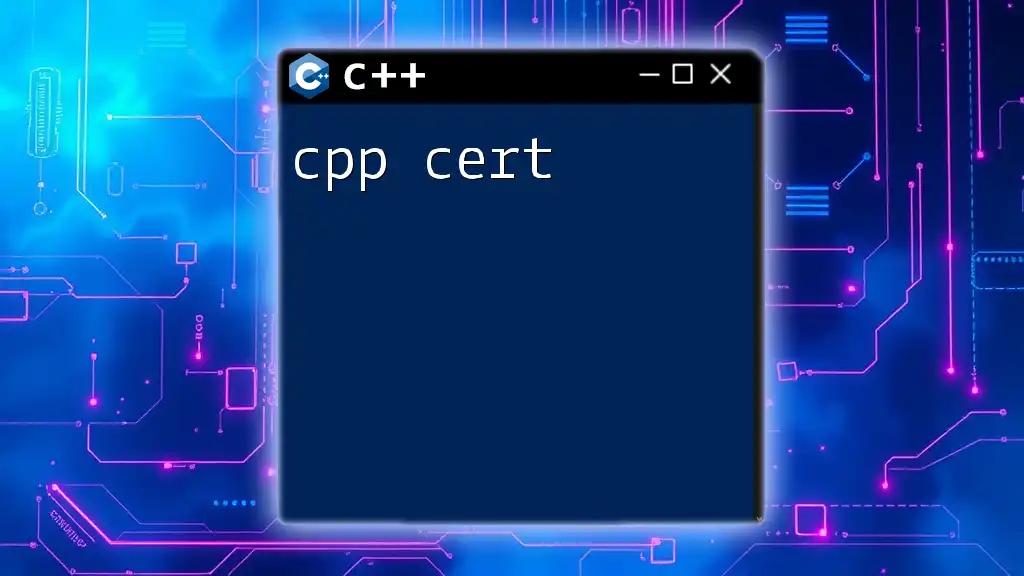
Practical Applications of sqrt in C++
Real-World Examples
The cpp sqrt function finds its application across various fields:
- Physics: Calculating distances or forces that involve square root computations.
- Finance: Deriving statistical measures, such as standard deviation, is crucial for financial analysis.
Example Project Ideas
You can incorporate the cpp sqrt function in various projects, such as:
- Creating a calculator application that performs various mathematical operations, including square roots.
- Developing a simulation model in physics that requires distance calculations.
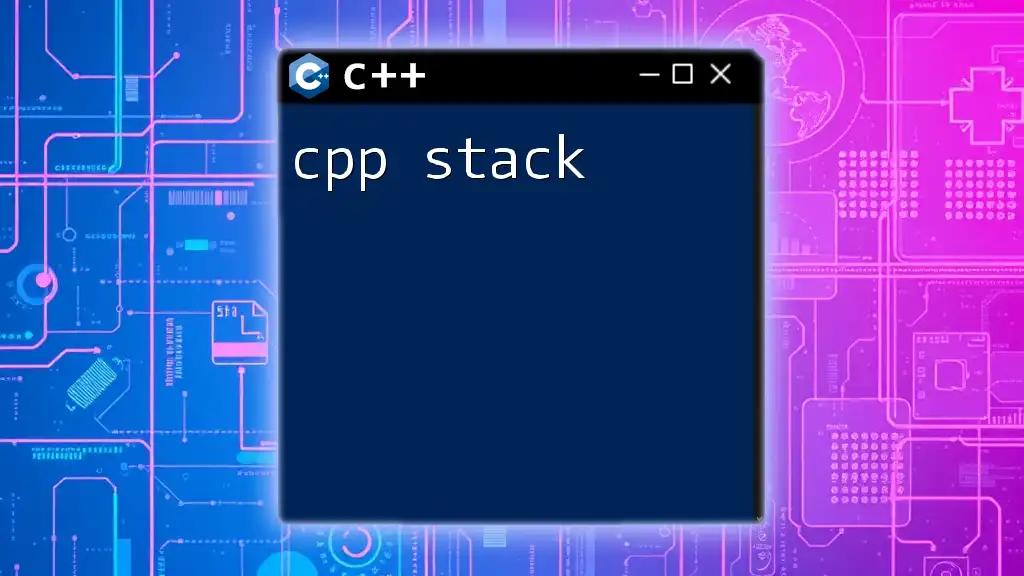
Conclusion
In summary, the cpp sqrt function is a vital tool in the C++ programming language, designed for easy and efficient square root computation. Understanding its usage, potential pitfalls, and applications can significantly enhance your programming capabilities. Practice using the cpp sqrt function through various projects to strengthen your skills and confidence.

Frequently Asked Questions (FAQs)
What is the return type of the sqrt function? The cpp sqrt function returns a `double` type, which is the calculated square root of the input parameter.
Does sqrt handle complex numbers? No, the cpp sqrt function does not handle complex numbers. If you need to compute square roots of complex numbers, consider using the `<complex>` library instead.
Is there a safe way to handle invalid inputs for sqrt? Yes, always validate your input to ensure it is non-negative before calling the cpp sqrt function to avoid errors.