A `std::map` in C++ is an associative container that stores elements in key-value pairs, allowing efficient retrieval and manipulation of data based on unique keys.
Here's a code snippet demonstrating how to create and use a `std::map`:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
// Accessing values
std::cout << "Alice's age: " << ageMap["Alice"] << std::endl;
std::cout << "Bob's age: " << ageMap["Bob"] << std::endl;
return 0;
}
Understanding the Basics
What is a Map?
In C++, a `map` is an associative container that stores elements in key-value pairs. Each unique key corresponds to exactly one value, which allows efficient retrieval, insertion, and deletion of elements based on that key.
While there are several types of data structures in C++, such as arrays and vectors, `std::map` offers significant advantages for specific use cases due to its key-value association. For example, if you want to maintain a dictionary where words (keys) map to their definitions (values), `std::map` is an excellent choice.
Key Characteristics of `std::map`
-
Ordered vs. Unordered: `std::map` maintains the order of keys based on their sorting criterion. This means that when you iterate over a `std::map`, elements are returned in ascending order by their keys. In comparison, an `unordered_map` will not maintain any particular order, which results in faster average lookups, but without guaranteed order.
-
Associative Container: You can access elements in a `std::map` through their keys, rather than by an index, which makes data retrieval much more intuitive when dealing with non-linear data structures.
-
Dynamic Size: `std::map` automatically adjusts its size when you add or remove elements. This dynamic resizing is an advantage for applications with varying data capacities, as it simplifies memory management.
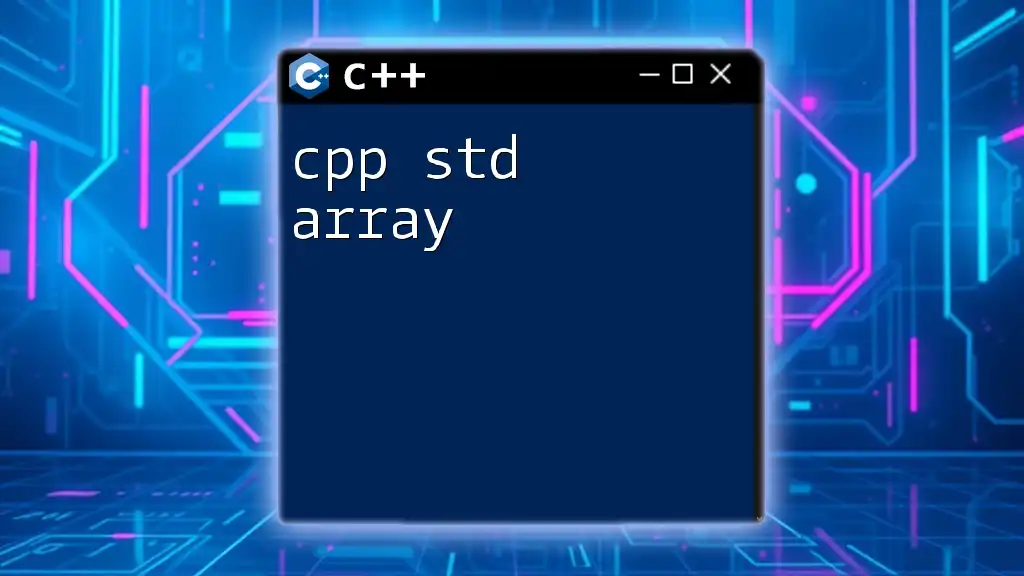
Basic Operations in `std::map`
Creating a `std::map`
To use a `std::map`, you first need to declare it. This involves specifying the types for the keys and the values it will hold. Here’s how to create a simple `std::map` that stores names as keys and ages as values:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ages;
}
Inserting Elements
Inserting elements into a `std::map` can be done using the `insert` method or the subscript operator (`[]`).
Here’s an example using both methods:
ages.insert({"Alice", 30}); // Inserts Alice with age 30
ages["Bob"] = 25; // Another way to insert Bob with age 25
Accessing Elements
Retrieving values in a `std::map` can be done using the `at()` method or the subscript operator. While both methods serve the same purpose, there is an important distinction: the `at()` method throws an exception when the key does not exist, while the subscript operator will create a new element with a default value.
Example:
std::cout << "Alice's age: " << ages.at("Alice") << std::endl; // Outputs 30
Modifying Elements
Updating values in a `std::map` is straightforward. You simply use the key associated with the value you want to change.
ages["Bob"] = 26; // Updates Bob's age to 26
Deleting Elements
To remove elements from a `std::map`, you can use the `erase` method.
For example:
ages.erase("Alice"); // Removes Alice from the map
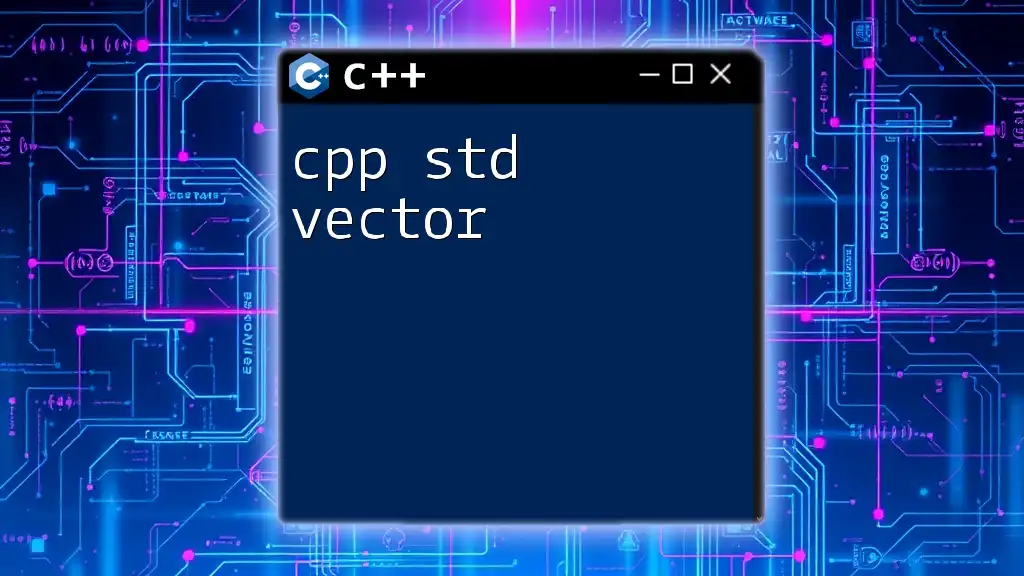
Iterating Through a `std::map`
Using Iterators
C++ provides iterators for traversing through a `std::map`. Here’s how you can use iterators to loop through your map:
for (auto it = ages.begin(); it != ages.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
Range-Based For Loop
An easier and more modern way to iterate over a `std::map` is to use a range-based for loop, which simplifies the syntax and improves readability.
for (const auto& pair : ages) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
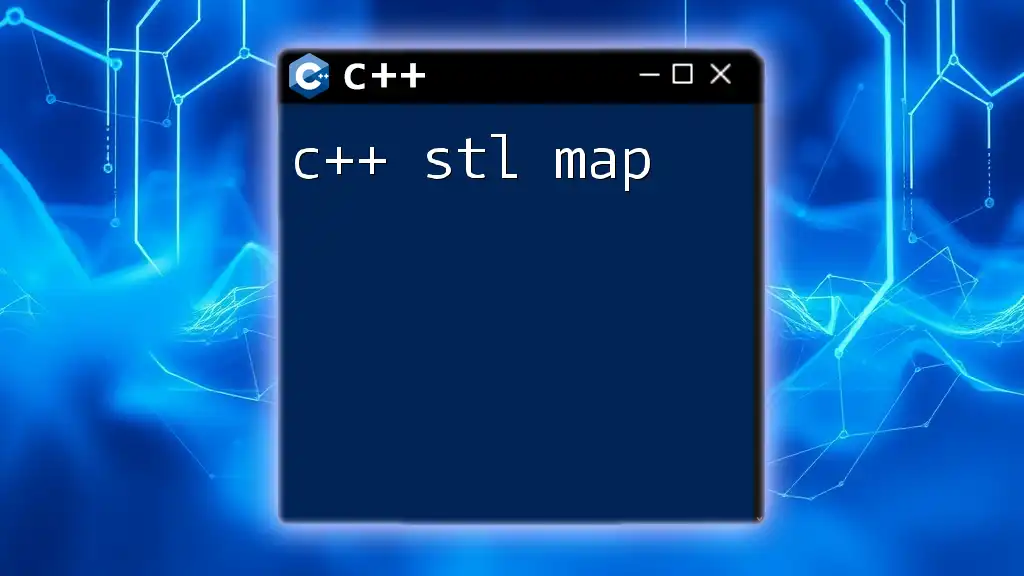
Advanced Features of `std::map`
Custom Comparison Functions
One of the powerful features of `std::map` is that it allows you to define your own comparison rules. This means you can create a `std::map` that sorts elements based on your own criteria.
Consider the following example where we sort strings by their length:
struct CustomCompare {
bool operator()(const std::string& a, const std::string& b) const {
return a.length() < b.length(); // Sort by string length
}
};
std::map<std::string, int, CustomCompare> customMap;
Maps of Maps
Sometimes, you might need a map of maps, which allows for more complex data structures. This is useful in scenarios like representing a graph or a nested configuration structure.
Here's an example:
std::map<std::string, std::map<std::string, int>> nestedMap;
nestedMap["ClassA"]["Student1"] = 90; // Student1 in ClassA
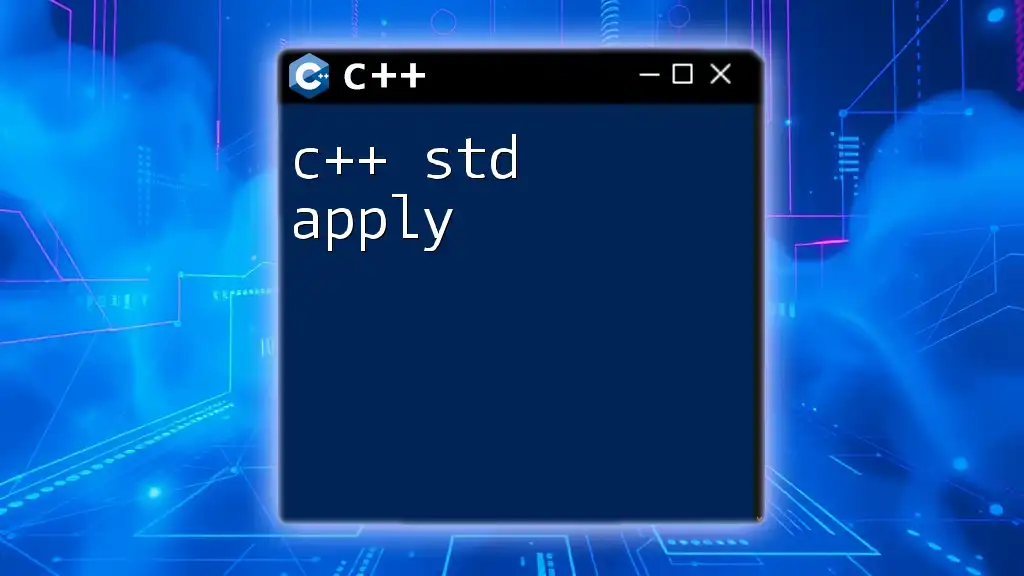
Performance Considerations
Time Complexity Analysis
Understanding time complexity is crucial when using `std::map`. The average time complexity for key operations (insertion, deletion, access) is O(log n) due to its underlying structure, which uses a balanced binary search tree, such as a Red-Black tree. Although this is efficient, operations on `std::unordered_map` offer average constant time complexity due to hash table implementation.
Memory Overhead
While `std::map` is efficient in terms of performance, it does come with some memory overhead because it needs extra space for maintaining its tree structure and managing pointers to create node relationships. Therefore, if memory usage is a concern, using `std::unordered_map` might be more suitable in situations where order is not important.
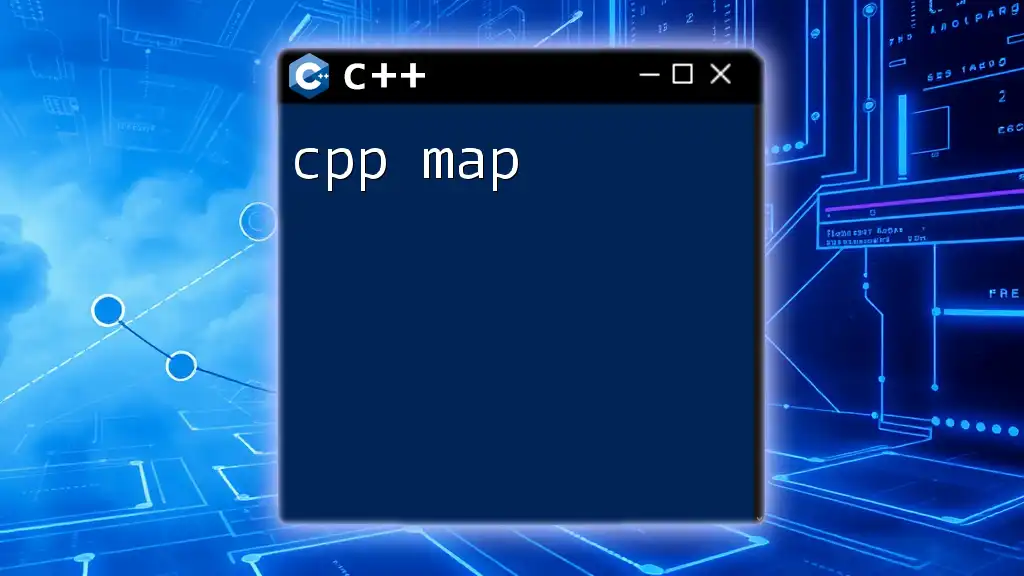
Common Pitfalls
Using non-unique keys in `std::map` is a common pitfall. Since `std::map` only allows unique keys, attempting to insert a duplicate key will silently overwrite the existing entry rather than raising an error. It's essential to verify whether a key exists before insertion if preserving original values is necessary.
Iterator invalidation is another key aspect to consider. Removing elements from a `std::map` can invalidate iterators, so it's advisable to be cautious or use the advanced techniques available in C++ to avoid errors in your code.
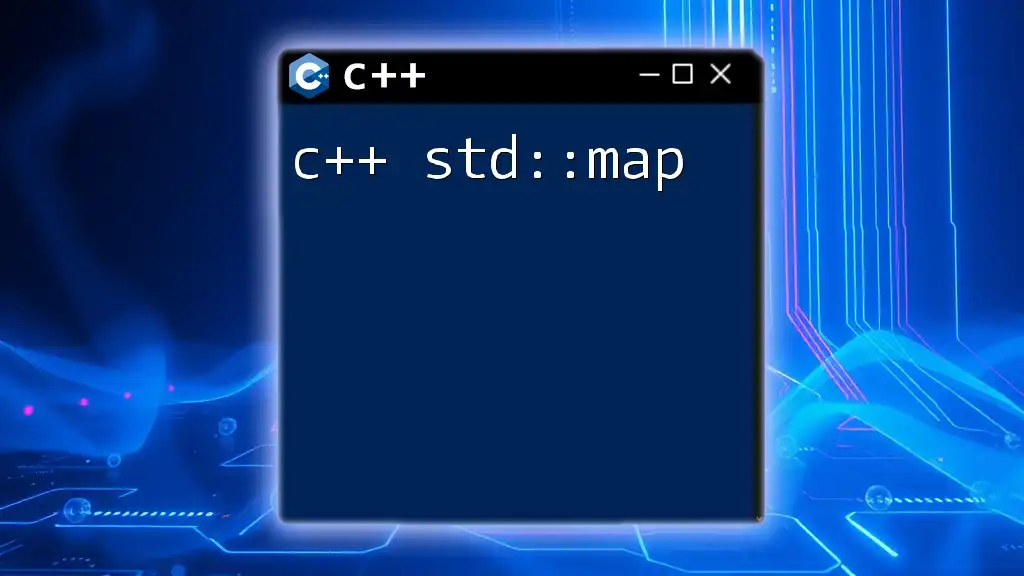
Real-world Applications
`std::map` finds its utility in numerous real-world applications. For instance, it can be used to create dictionaries, store configurations, or represent relationships between entities in databases. It allows programmers to keep their data organized and accessible while maintaining a balance between performance and ease of use.
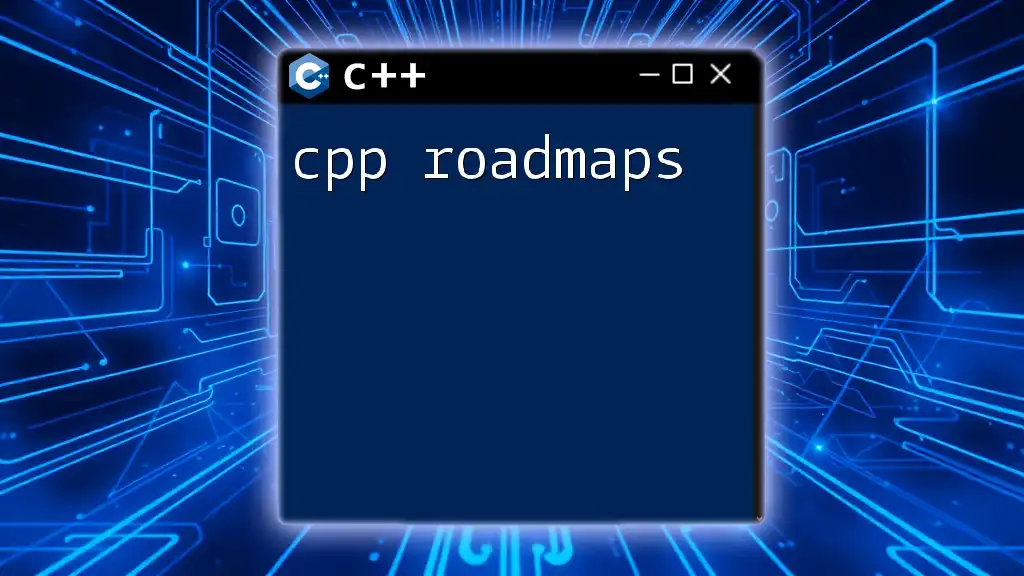
Conclusion
`cpp std map` is an invaluable tool for any C++ programmer, offering a rich feature set that allows for efficient data management through key-value pairs. By leveraging its capabilities, you can create robust and flexible applications that make the most of C++'s strengths. Experimenting with its different functionalities will not only improve your coding skills but also enhance your understanding of data structure principles in programming.
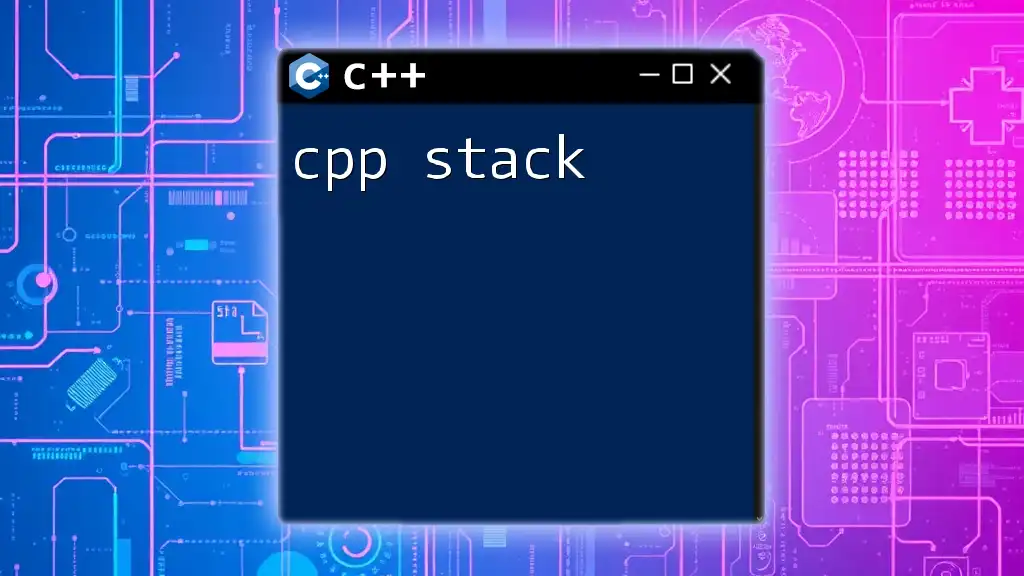
Additional Resources
For further learning, the official C++ documentation contains extensive insights into `std::map`. Online tutorials, coding challenges, and community forums can also provide practical experience and interactions that will deepen your mastery of C++ and its powerful standard library features.