`std::array` is a container in C++ that encapsulates fixed-size arrays, providing benefits like safety and ease of use with its STL features.
#include <array>
#include <iostream>
int main() {
std::array<int, 5> arr = {1, 2, 3, 4, 5};
for (const auto& elem : arr) {
std::cout << elem << " ";
}
return 0;
}
Benefits of Using C++ std::array
Using `cpp std array` comes with numerous benefits that enhance the safety and performance of your C++ applications. Here are some of the main advantages:
-
Type Safety: Unlike raw arrays, which can lead to various runtime errors, `std::array` enforces type safety. This means that when you define an array of a specific type, you cannot accidentally assign a value of a different type.
-
Size Fixed at Compile Time: The size of `std::array` is determined at compile time, which can lead to better performance. This eliminates the overhead associated with dynamic memory allocation and allows the compiler to optimize your code more effectively.
-
Integration with C++ Standard Library: `std::array` seamlessly fits into the C++ Standard Library, meaning you can use it with other STL algorithms and utilities, enhancing your coding efficiency and performance.
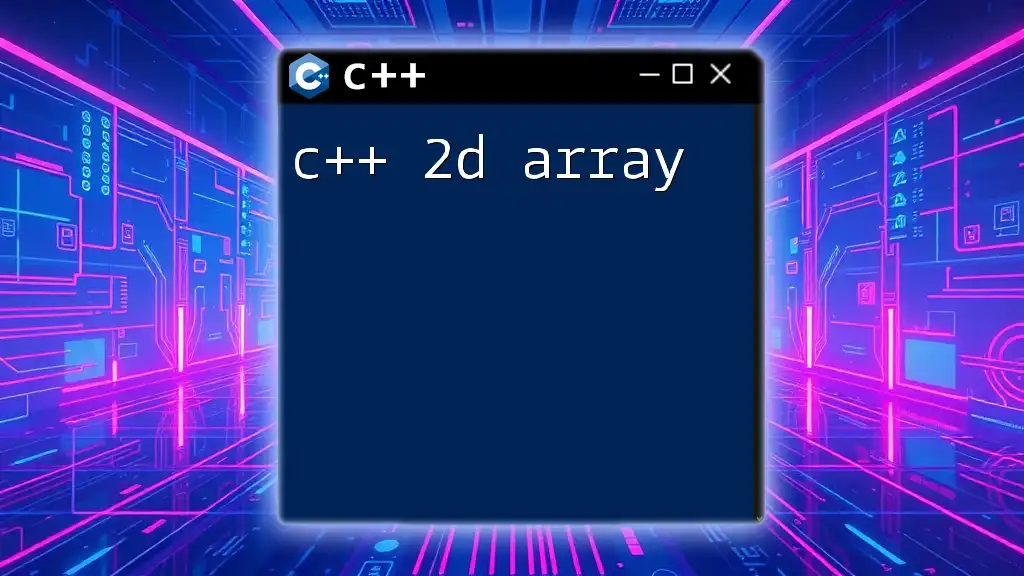
Creating an Instance of std::array
Syntax of std::array
To declare an instance of `std::array`, you can use the following syntax:
std::array<Type, Size> arrayName;
For example, to create an array of integers with five elements, you can write:
std::array<int, 5> myArray;
Initializing std::array
There are several ways to initialize a `std::array`, making it versatile for different scenarios.
-
Default Initialization: You can create an empty array like this:
std::array<int, 5> myArray{};
-
List Initialization: You can initialize your array with specific values:
std::array<int, 5> myArray = {1, 2, 3, 4, 5};
-
Using std::array::fill() Method: The `fill()` method allows you to set all elements to a specific value:
std::array<int, 5> myArray; myArray.fill(10); // all elements set to 10
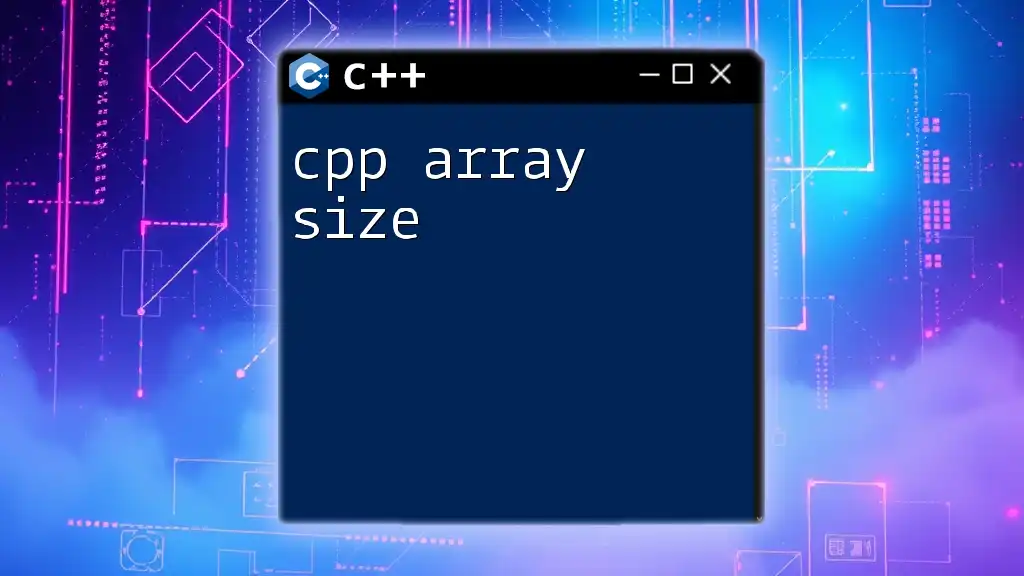
Accessing Elements in a std::array
Using the `[]` Operator
Accessing elements in a `std::array` is straightforward using the `[]` operator:
int firstElement = myArray[0];
This method is efficient, but keep in mind that it does not perform bounds checking.
Using the `at()` Method
The `at()` method offers a safer way to access elements as it checks for out-of-bounds access:
int secondElement = myArray.at(1); // Throws exception if out of bounds
Using `at()` helps prevent runtime errors, contributing to safer code.
Iterating Over std::array
You can iterate over a `std::array` using various methods:
Using Range-Based For Loop
Range-based for loops simplify the syntax to traverse arrays:
for(const auto& element : myArray) {
std::cout << element << " ";
}
Using Standard Iterators
Another way to iterate is to use iterators for more manual control:
for(auto it = myArray.begin(); it != myArray.end(); ++it) {
std::cout << *it << " ";
}
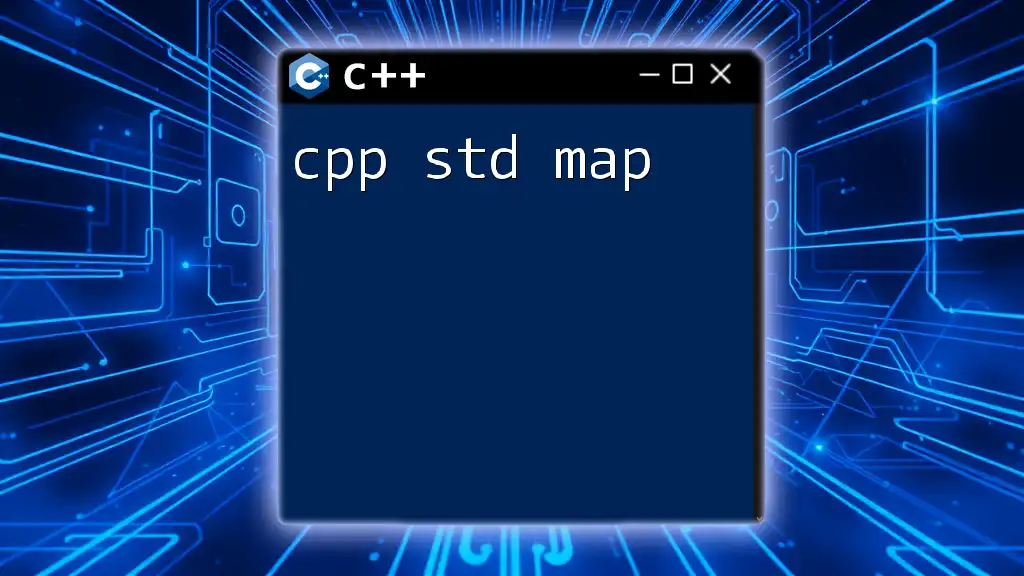
Modifying Elements in std::array
Using the `[]` Operator
You can easily modify elements using the `[]` operator:
myArray[0] = 10;
This method directly modifies the specified element.
Using the `at()` Method
If you prefer to add a layer of safety, you can also use the `at()` method to modify elements:
myArray.at(1) = 20;
This ensures that you're not interacting with out-of-bounds indices, making your code more robust.
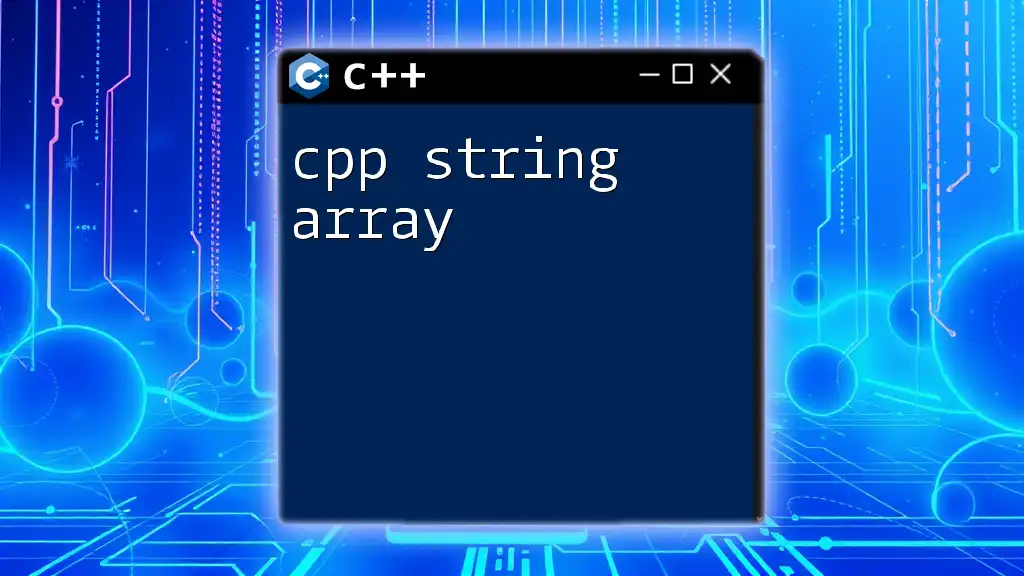
Important Member Functions of std::array
size() and max_size()
The `size()` method returns the number of elements in the array:
std::cout << "Size: " << myArray.size();
The `max_size()` method tells you the maximum size the array can hold, which is generally the same as the size for `std::array` since it is fixed.
empty() Method
You can check if the array is empty with the `empty()` method:
if(myArray.empty()) {
std::cout << "Array is empty";
}
For `std::array`, this will always return `false`, because sizes are fixed at compile-time.
data() Method
The `data()` method provides access to the underlying array storage:
int* ptr = myArray.data();
This is useful if you need to interface with C libraries requiring raw pointers.
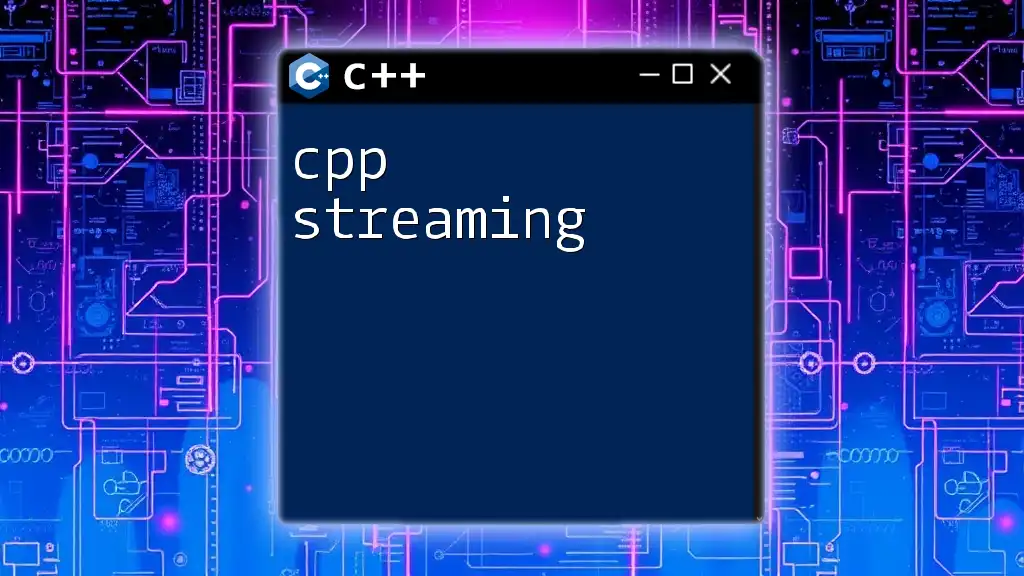
Comparison of std::array with Other Containers
std::vector vs std::array
While `std::array` provides a fixed-size array with performance advantages, `std::vector` offers dynamic sizing but with performance trade-offs. Use `std::array` when you know the size will remain constant, allowing for more efficient memory usage.
std::array vs C-style Arrays
One of the most significant advantages of `std::array` over C-style arrays is safety. With `std::array`, you get bounds checking with the `at()` method, eliminating common pitfalls of C-style arrays that can lead to undefined behavior.
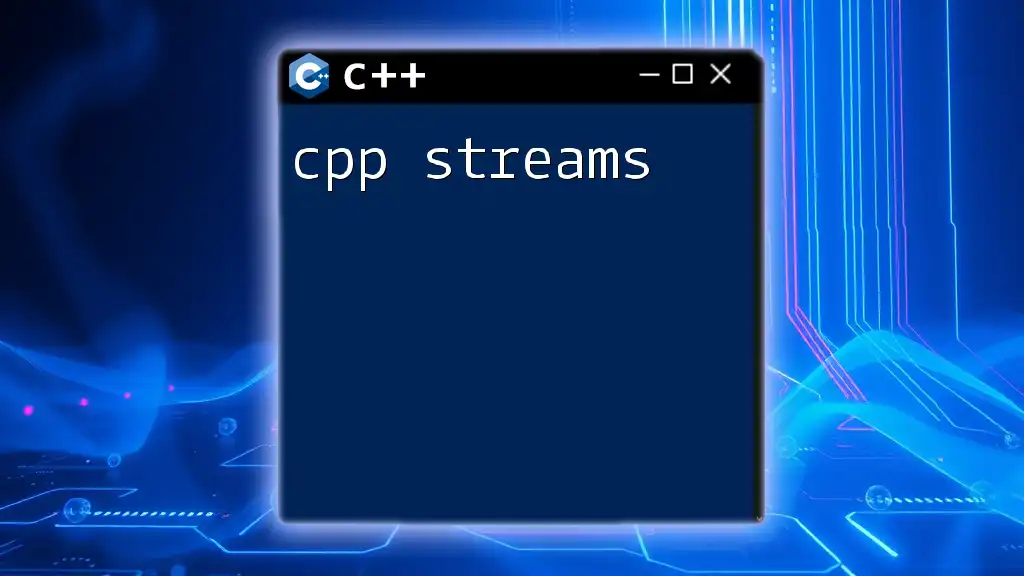
Common Use Cases for std::array
Using std::array in Function Parameters
`std::array` can be passed as function parameters, benefiting from its size information, which can lead to cleaner, more efficient code.
void printArray(const std::array<int, 5>& arr) {
for(const auto& val : arr) {
std::cout << val << " ";
}
}
std::array in Complex Data Structures
You can use `std::array` as a member of your own classes or structures. Its fixed-size nature can ensure that every object maintains a consistent state.
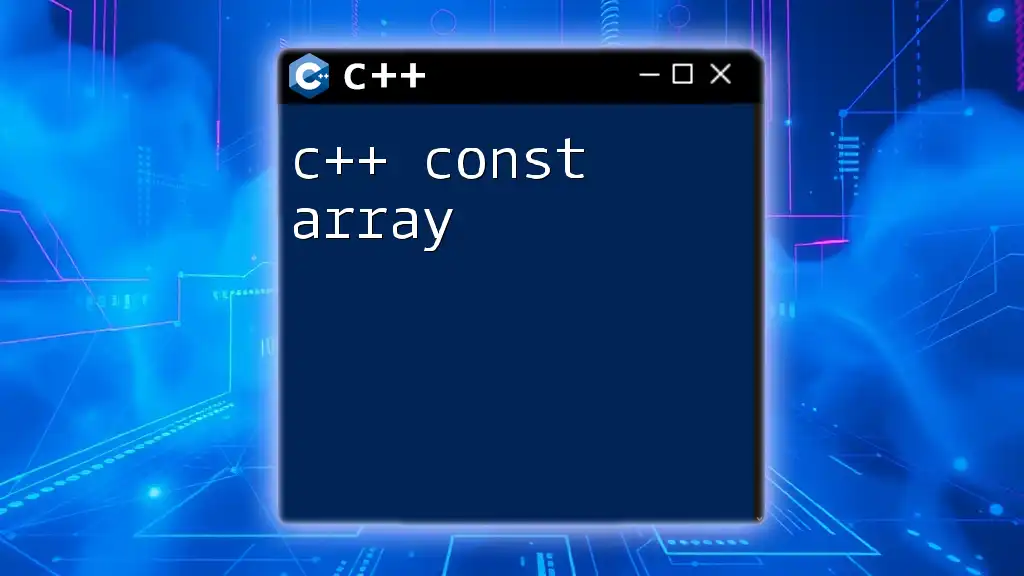
Conclusion
In conclusion, `cpp std array` is a powerful feature of C++ that offers developers significant advantages over traditional C-style arrays. With features like type safety, compile-time sizing, and seamless integration with the C++ Standard Library, it becomes a crucial tool for efficient and reliable programming. Embracing `std::array` in your projects can significantly improve both your coding experience and the performance of your applications.
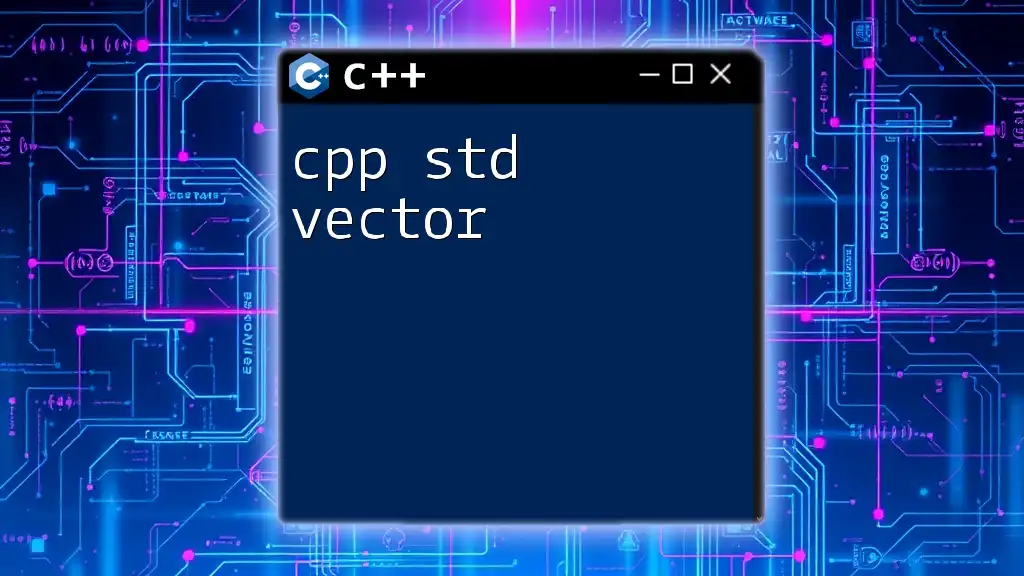
Additional Resources
For further learning, consider checking out comprehensive books on C++ programming, engaging with community tutorials, or exploring the official C++ documentation for in-depth coverage of the `std::array` and related concepts.