In C++, you can determine the size of an array by dividing the total size in bytes of the array by the size of a single element, as shown in the following code snippet:
#include <iostream>
int main() {
int arr[] = {1, 2, 3, 4, 5};
size_t size = sizeof(arr) / sizeof(arr[0]);
std::cout << "Array size: " << size << std::endl;
return 0;
}
Understanding C++ Arrays
What are Arrays in C++?
Arrays are fundamental data structures that allow programmers to store multiple items of the same type in a single variable. In C++, an array can be likened to a collection of boxes, each capable of holding data. This storage method is crucial for efficiently organizing and accessing large amounts of related data.
Arrays simplify many programming tasks, particularly those involving sequences of information where the number of elements is known in advance, such as lists of numbers, character strings, and more.
How to Declare an Array
In C++, declaring an array is straightforward. The syntax involves specifying the type of the array elements followed by the array name and the size of the array in square brackets. Here’s how an array is declared:
int myArray[10];
In this example, `myArray` can hold up to ten integers. It's important to remember that C++ arrays are zero-indexed, meaning the valid indices for `myArray` are from 0 to 9.
Initializing Arrays in C++
Initialization can be done at the time of declaration or later on. C++ offers various ways to initialize an array:
- Default Initialization: The elements are automatically initialized to zero.
- Custom Initialization: You can specify the values to initialize the array with.
Example of custom initialization:
int myArray[5] = {1, 2, 3, 4, 5};
Here, the first five elements of `myArray` are initialized with the values 1 through 5.
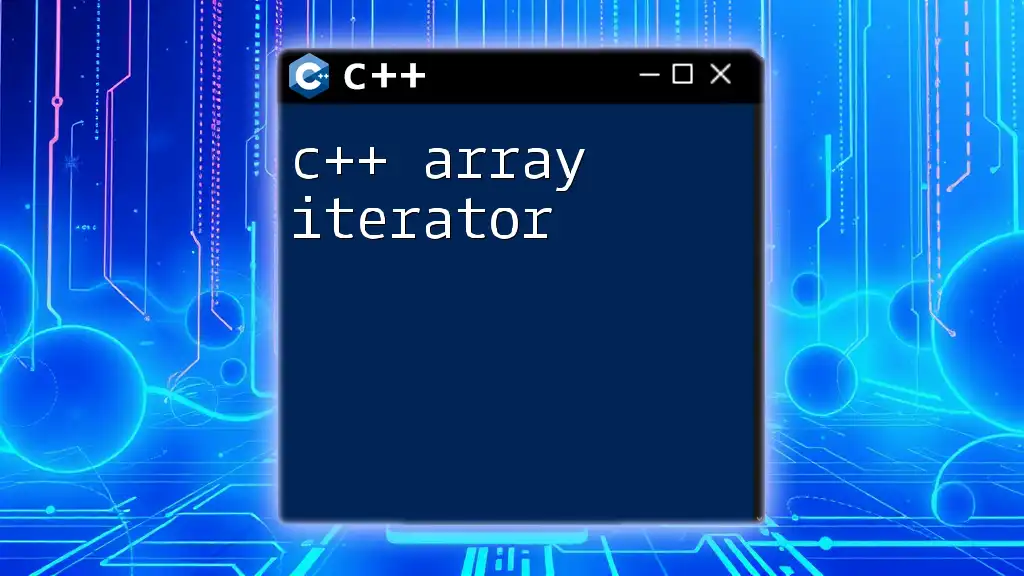
The Concept of Array Size
What Does Array Size Refer To?
The term "array size" refers to the number of elements in the array that can be stored. Knowing the array size is essential for effective programming, as it helps prevent errors like out-of-bounds access that can lead to unpredictable behavior or crashes.
Determining Array Size in C++
In C++, the `sizeof` operator is frequently used to determine the size of an array, which is particularly useful when working with local arrays. The syntax requires employing the `sizeof` operator in conjunction with the element size.
Code example:
int myArray[10];
int size = sizeof(myArray) / sizeof(myArray[0]);
In this example, `sizeof(myArray)` returns the total size in bytes allocated for `myArray`, while `sizeof(myArray[0])` provides the size of one element. Dividing the former by the latter yields the total number of elements stored in the array, which in this case is 10.
Limitations of Using `sizeof`
While the `sizeof` operator is highly efficient, it comes with certain limitations. Notably, `sizeof` does not work as expected with pointers. If you pass an array to a function, it decays into a pointer, and using `sizeof` on it will yield the size of the pointer, not the array.
Example demonstrating the limitation:
void function(int arr[]) {
std::cout << sizeof(arr); // Outputs the size of the pointer, not the array
}
In this case, `sizeof(arr)` will not return the total size of the array passed to the function but rather the size of the pointer itself.
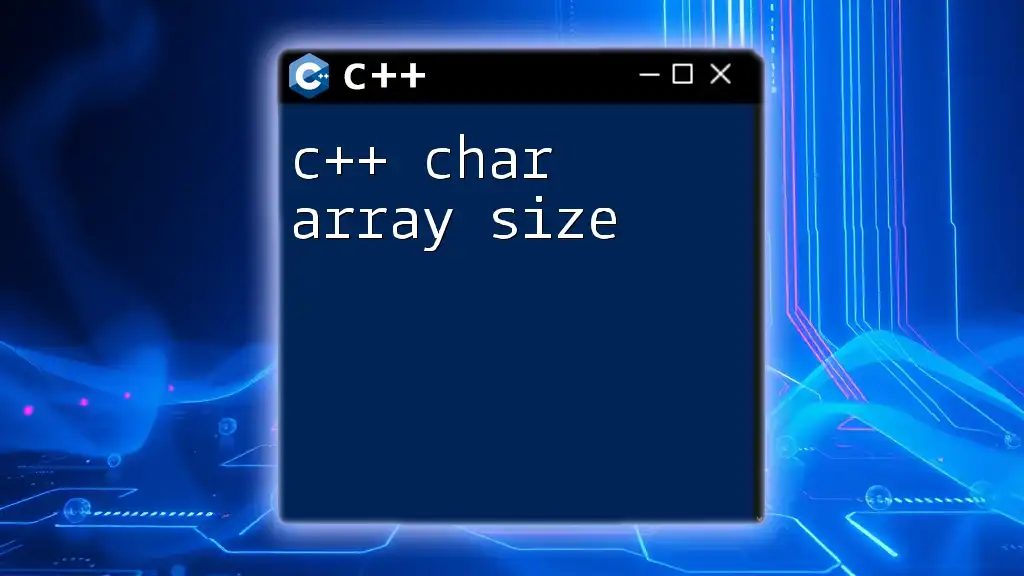
Best Practices for Managing Array Size
Using Constants for Array Sizes
To promote code maintainability and avoid hardcoding values, it is often beneficial to use constants for array sizes. This practice not only improves readability but also minimizes the risk of errors if the array size needs to change.
Example of defining constants:
const int ARRAY_SIZE = 10;
int myArray[ARRAY_SIZE];
This makes it easier to modify the array size in one location without having to hunt down and update multiple instances throughout the code.
Dynamic Arrays and Their Size
When the required size of an array is uncertain at compile time, you can opt for dynamic arrays. C++ enables dynamic memory allocation via the `new` and `delete` keywords.
Example of dynamic array creation:
int size;
std::cout << "Enter the size of the array: ";
std::cin >> size;
int* myArray = new int[size];
// Perform operations on the dynamic array
// Don't forget to free the allocated memory
delete[] myArray;
In this scenario, the user supplies the size of `myArray`, allowing for flexibility not available with static arrays.
STL Containers as Alternatives to Arrays
While arrays serve valuable purposes, the Standard Template Library (STL) offers more robust alternatives, such as `std::vector`. Vectors provide the advantages of dynamic sizing, automatic memory management, and a rich set of built-in functions.
Code example demonstrating the use of `std::vector`:
#include <vector>
std::vector<int> myVector = {1, 2, 3, 4, 5};
Vectors automatically adjust their size as elements are added or removed, alleviating the need for manual memory management issues tied to traditional arrays.
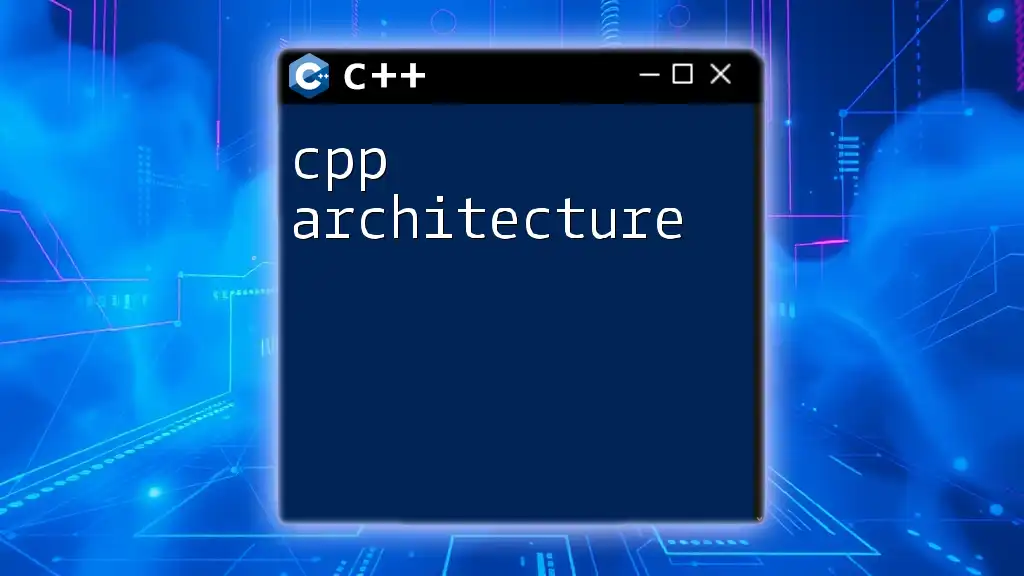
Common Mistakes Related to Array Size
Out of Bounds Access
One of the most common mistakes encountered with arrays is out-of-bounds access. This occurs when you attempt to read or write an index outside the allocated size of the array. Such operations can lead to unpredictable behavior, including crashes or memory corruption.
Example showing the effect of out-of-bounds access:
int myArray[5] = {1, 2, 3, 4, 5};
int outOfBoundsValue = myArray[5]; // This is incorrect! Accessing an invalid index
In this case, accessing `myArray[5]` is out of bounds and could lead to undefined behavior.
Mismatched Array Size and Loop Iterations
Another frequent issue arises from mismatched array sizes and the corresponding loop iterations. When looping through an array, using incorrect boundaries can easily lead to out-of-bounds access.
Example demonstrating bad loop practices:
for (int i = 0; i <= 5; i++) { // Incorrect loop condition
std::cout << myArray[i];
}
As the condition `i <= 5` results in trying to access `myArray[5]`, this could result in an error. Always ensure that the loop condition stays within the array's valid index range.
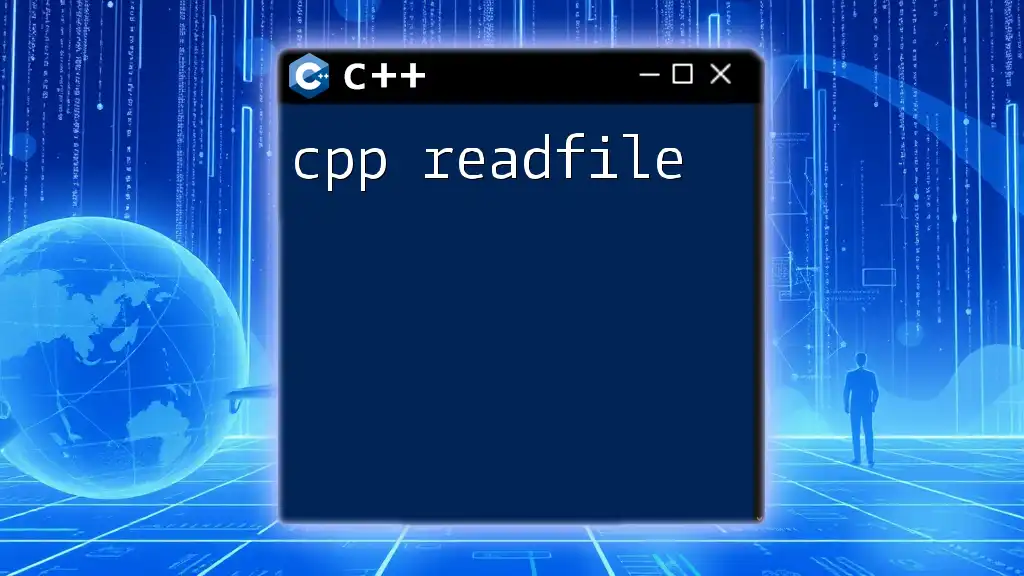
Summary and Best Takeaways
The journey through understanding cpp array size brings forth several critical takeaways. Remember that array size is reserved at compile time, understanding its limitations is crucial when working with functions and pointers, and that utilizing constants helps in maintaining cleaner code.
Moreover, keep in mind that while classic arrays serve a valuable purpose, modern C++ offers powerful alternatives such as vectors which can handle dynamic memory management more gracefully.
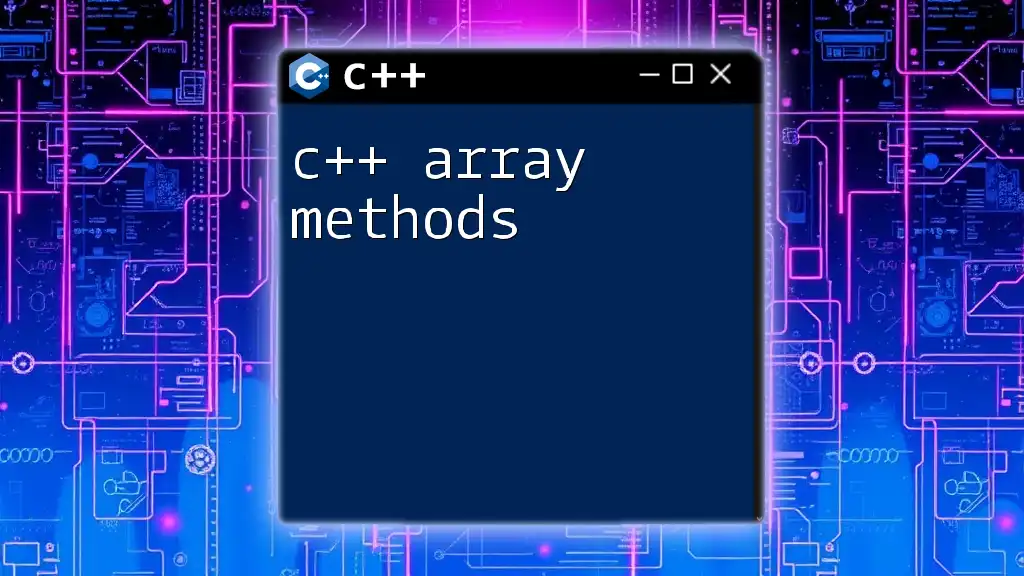
Additional Resources
Recommended Readings
For those interested in deepening their understanding, we recommend exploring comprehensive resources on C++ arrays and data structures that delve into more advanced topics and best practices.
Online Courses or Tutorials
There are many online platforms offering courses on C++ programming that cover arrays and data structures, providing hands-on projects and in-depth study materials to solidify your knowledge.