Creating a C++ website involves using C++ for server-side operations, often leveraging frameworks like Wt to serve web content dynamically.
Here's a simple example of a C++ server using the Wt framework:
#include <Wt/WApplication.h>
#include <Wt/WText.h>
class MyApp : public Wt::WApplication {
public:
MyApp(const Wt::WEnvironment& env) : Wt::WApplication(env) {
setTitle("My C++ Web Application");
Wt::WText *text = new Wt::WText("Hello, C++ Web World!", root());
}
};
Wt::WApplication *createApplication(const Wt::WEnvironment& env) {
return new MyApp(env);
}
Understanding C++ in Web Development
What is C++?
C++ is a high-level programming language renowned for its ability to deliver performance and efficiency. It encompasses both low-level and high-level programming capabilities, making it suitable for a range of applications, including systems programming, game development, and web technologies. Key characteristics of C++ include:
- Object-Oriented Approach: Facilitates code reusability and organization through encapsulation, inheritance, and polymorphism.
- Rich Standard Library: Offers a wealth of built-in functions and libraries, allowing developers to carry out complex tasks with minimal effort.
- Performance: C++ is compiled to machine-level code, enhancing execution speed, making it an appealing choice for performance-sensitive web applications.
Role of C++ in Web Technologies
While many associate web development with languages like JavaScript or Python, C++ holds its ground, especially in high-performance areas.
-
Comparison of C++ with Other Languages: C++ delivers advantages such as speed and memory control. Unlike interpreted languages, C++ compiles directly to machine code, resulting in faster execution time. This makes C++ an appropriate choice for back-end systems that require intensive data processing.
-
Common Use Cases of C++ in Web Development: C++ is predominantly used in server-side applications, where speed is paramount. Additionally, it lends itself well to game development, real-time systems, and applications that demand resource efficiency.
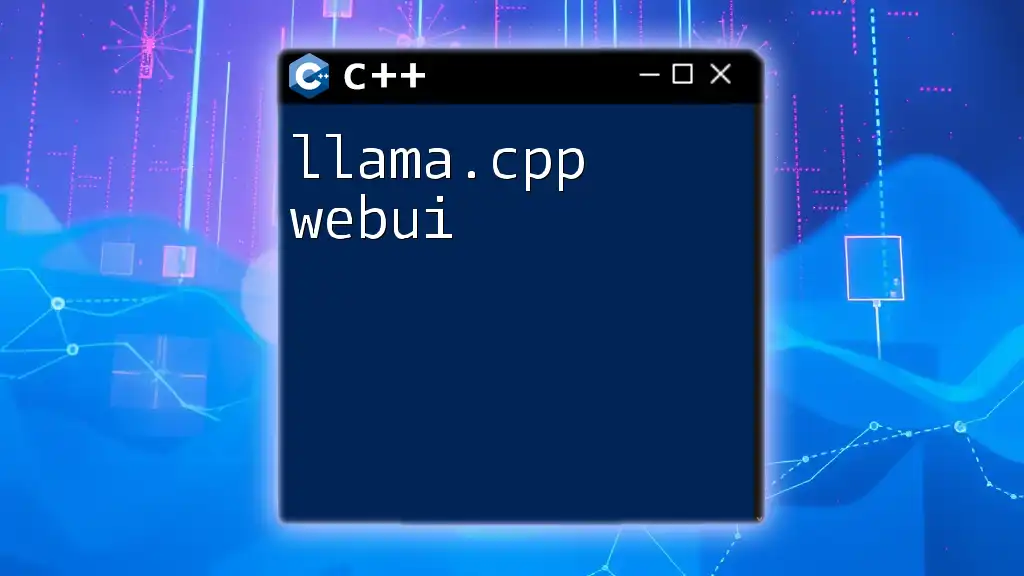
Setting Up a C++ Web Development Environment
Required Tools and Software
To begin your journey with C++ web development, a solid setup is essential. You'll need a combination of a compiler, a web server, and potentially a framework.
-
Compilers: Critical for transforming your C++ code into an executable format. Popular choices include:
- GCC: The GNU Compiler Collection, widely revered for its robustness.
- Clang: A compiler front end for the C family of programming languages, known for its fast compilation speeds.
- Visual Studio: An IDE that includes an excellent C++ compiler for Windows development.
-
Integrated Development Environments (IDEs): Using an IDE can significantly streamline your workflow. Some notable ones are:
- Code::Blocks: Lightweight and straightforward.
- Eclipse CDT: Extensible and suitable for larger projects.
Installing a Web Server for C++ Applications
To host your C++ web applications, you will require a web server that can handle C++ code, such as Apache or NGINX.
- Apache with C++ Modules: Apache is an open-source HTTP server capable of serving C++ applications using specific extensions.
Example to install Apache on Ubuntu:
# Install Apache on Ubuntu
sudo apt-get install apache2
# Enable C++ modules
sudo a2enmod c++_your_module
- NGINX with C++ Back-ends: NGINX can be set up to serve applications built with C++, as it is known for its concurrency handling.
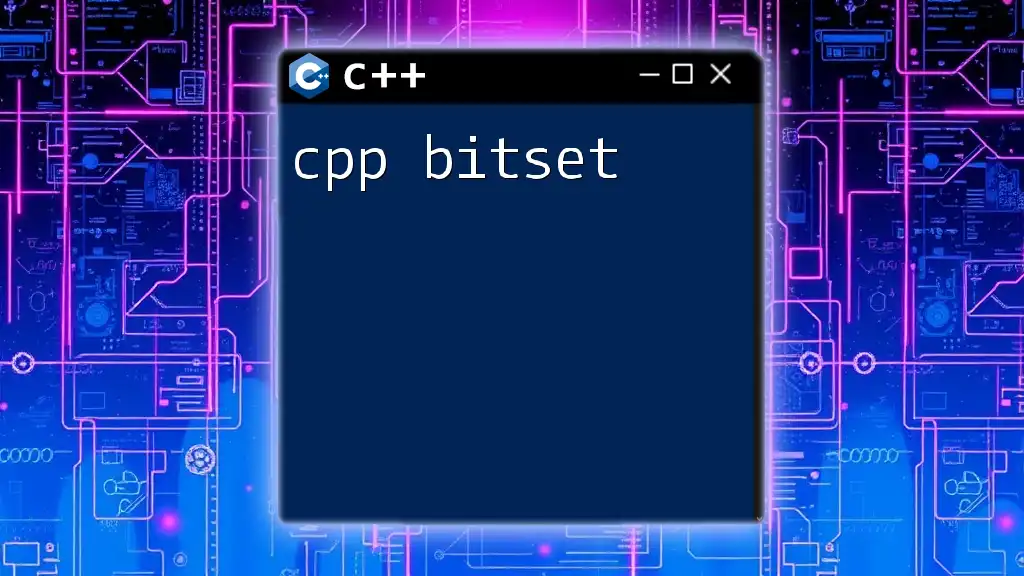
Key C++ Libraries and Frameworks for Web Development
Web Frameworks
Several frameworks enable you to build web applications with C++ seamlessly.
-
CPPCMS: This high-performance web application framework allows you to develop complex web applications efficiently. It focuses on delivering fast responses without sacrificing features.
-
Wt: A C++ library for developing web applications, Wt offers a widget-centric approach, similar to desktop GUI frameworks. It abstracts many low-level details, allowing for easier application development.
-
Crow: A lightweight web framework that enables you to create responsive web applications effortlessly. Its syntax is highly intuitive for C++ developers.
Example usage of Crow to create a basic application:
#include <crow.h>
int main()
{
crow::SimpleApp app;
CROW_ROUTE(app, "/")([](){
return "Hello, C++ Web!";
});
app.port(18080).multithreaded().run();
}
Networking Libraries
You can also utilize popular networking libraries to boost your C++ web applications' functionality.
- Using Boost.Asio: A powerful library, Boost.Asio enables you to build networked applications and handle asynchronous operations effectively.
Example of a simple C++ server using Boost.Asio:
#include <boost/asio.hpp>
using boost::asio::ip::tcp;
int main() {
boost::asio::io_context io_context;
tcp::acceptor acceptor(io_context, tcp::endpoint(tcp::v4(), 12345));
// Accept connections and handle requests here
}
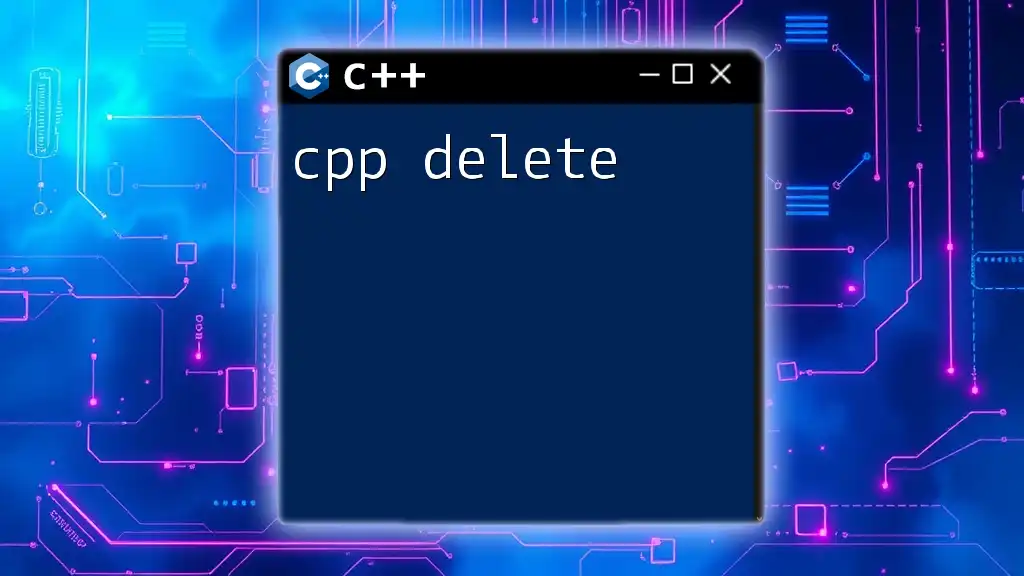
Building a Simple C++ Web Application
Project Structure
To maintain readability and manageability, organize your C++ web application into a logical folder structure. A suggested layout is:
- `src/`: Contains your source code files.
- `include/`: Holds header files for your C++ classes.
- `lib/`: Libraries and dependencies.
- `test/`: Where your test files reside.
- `build/`: The folder where your compiled binaries will be placed.
Coding Your First C++ Web App
Let’s create a basic C++ web application using the Crow framework. This application will serve a simple "Hello, World!" message to users.
Step-by-step Guide:
- Set up your project folder with the structure mentioned above.
- Include Crow as a dependency in your `src` folder.
- Write the following code in your `main.cpp`:
#include <crow.h>
int main()
{
crow::SimpleApp app;
CROW_ROUTE(app, "/")([](){
return "Hello, C++ Web!";
});
app.port(18080).multithreaded().run();
}
This minimal code snippet initiates a web server on port 18080 and serves a greeting at the root URL.
Compiling and Running Your Application
To compile and run your application effortlessly, you can use `make` or CMake, both of which are efficient tools for managing C++ projects.
Example `make` command:
# Basic make command
make your_application
# Running your application
./your_application
This will compile the code and generate an executable that you can run to see your "Hello, World!" message in the web browser.
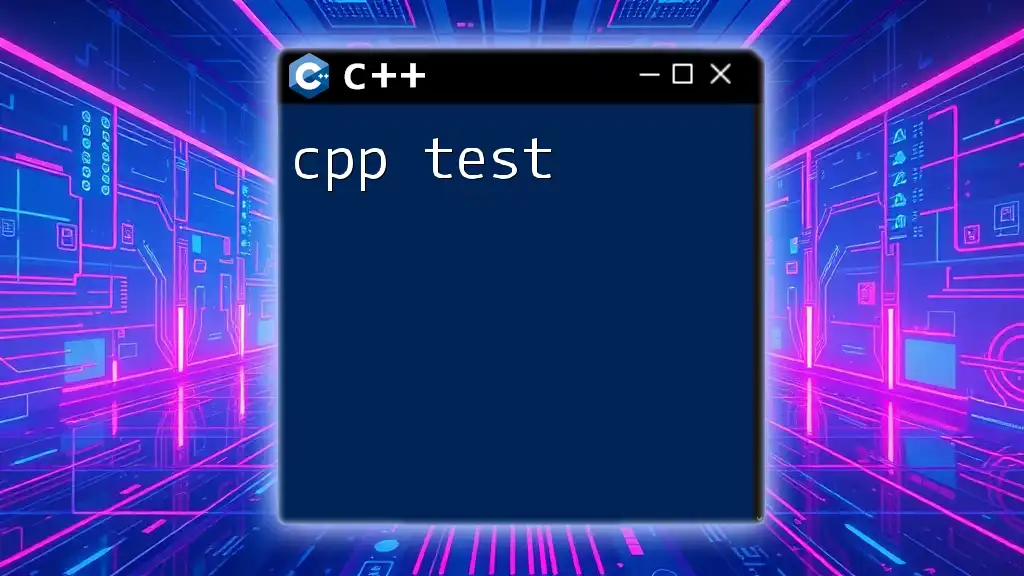
Exploring C++ Command Basics for Web Development
Important C++ Commands and Syntax
Mastering key C++ commands is crucial. Focus on:
- Data Types: Understanding `int`, `float`, `double`, `char`, and `std::string`.
- Control Structures: Utilizing `if`, `else`, `for`, and `while` loops effectively.
Applying Commands to Web Context
Consider practical scenarios where C++ commands come into play:
- Arithmetic Operations: Use C++ operators to handle client request parameters.
- Loops and Conditionals: Navigate web routing based on user input, optimizing user experience.
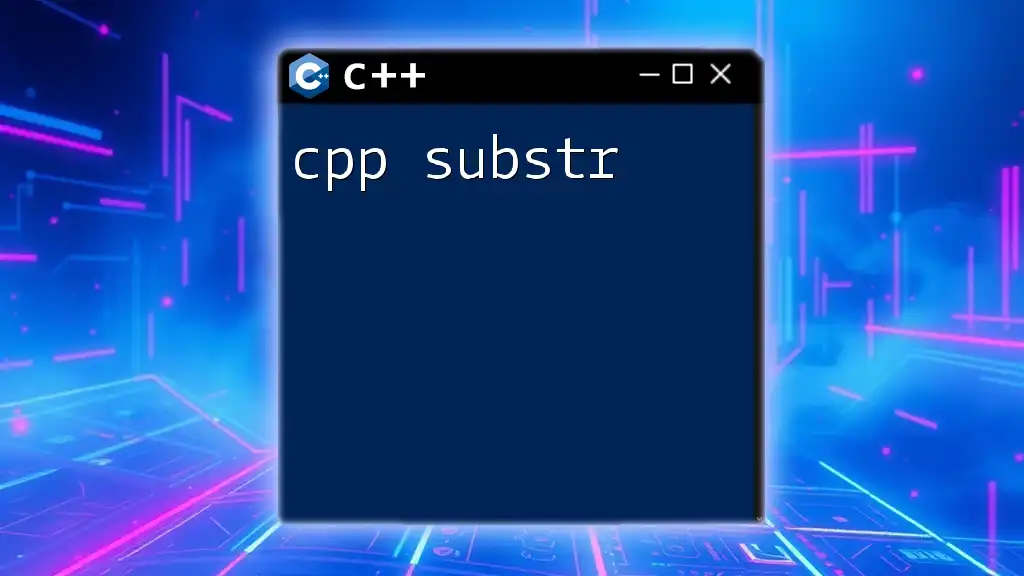
Testing and Debugging C++ Web Applications
Importance of Testing in C++ Web Development
Testing is essential to ensure the reliability and performance of your C++ web applications. Common methodologies include unit testing and integration testing.
- Unit Testing with Google Test: A robust framework that allows you to write test cases for your C++ functions ensures they behave as expected under various conditions.
Debugging Techniques
Debugging can be simplified with the right tools. Familiarize yourself with:
-
gdb (GNU Debugger): Offers a powerful way to step through your code, inspect variables, and catch errors.
-
Valgrind: A tool for memory debugging that helps you identify memory leaks and profiling your application's performance.
Example of debugging a simple web app:
# Start gdb with your application
gdb ./your_application
# Inside gdb, use breakpoints and run commands to inspect the code execution
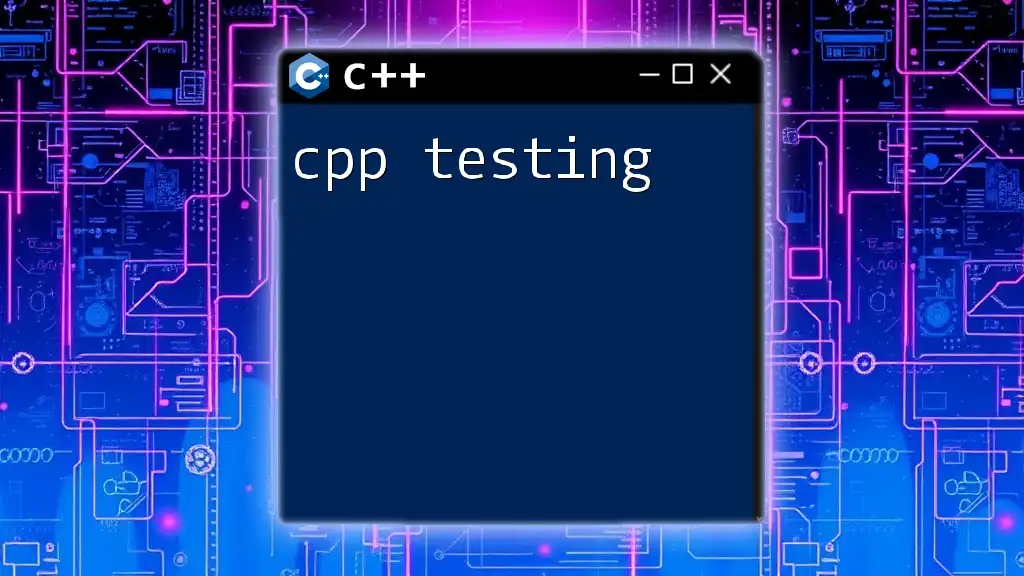
Conclusion
Mastering C++ for web development opens doors to high-performance and resource-efficient applications. From understanding the nuances of C++ commands to setting up a server and utilizing frameworks, this guide serves as a thorough introduction.
Next Steps for Learners
Continue your journey in C++ web development by exploring more complex frameworks, participating in coding challenges, and engaging with the community. Embrace the learning process, and start building your C++ projects today.
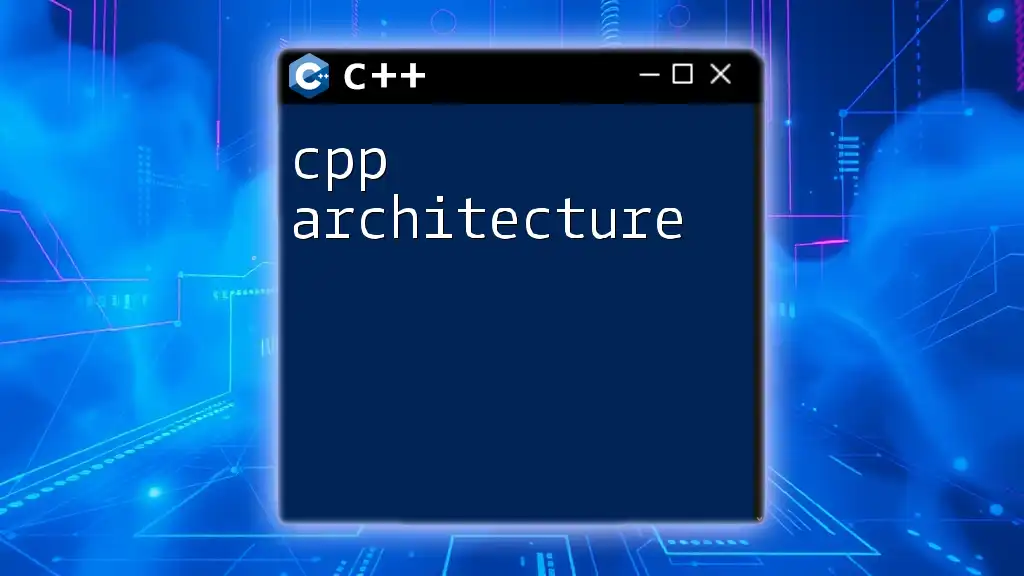
Call to Action
Join our C++ web development community to access resources, participate in workshops, and share your learning experience with fellow developers. Dive into the exciting world of C++ web applications and elevate your programming skills!