The C++ architecture roadmap outlines the essential components and principles of C++ programming, guiding learners from foundational syntax to advanced features for effective software development.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Basic output command in C++
return 0;
}
Understanding C++ Architecture
What is C++ Architecture?
C++ architecture refers to the foundational design principles and structural components that define how C++ programs are constructed and how they function. It encompasses everything from how the code is compiled and linked to how it runs in memory. Understanding C++ architecture is essential for developers looking to optimize their applications for performance and maintainability.
Key Components of C++ Architecture
Compilers and Linkers
The compiler plays a critical role in transforming human-readable C++ code into machine code that the computer can execute. When you write a program, the compiler parses the code, performs syntax checks, optimizations, and then generates the binary output.
For example, consider the following simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
When compiled, this code is converted into a format that a computer's CPU can understand and execute. The linker then takes this binary output, along with any libraries your program requires, and combines them into a final executable file. It's crucial to understand these processes because they affect how effectively your code runs and how you can manage dependencies.
Standard Library
The C++ Standard Library is an essential part of C++ architecture, providing numerous built-in functionalities that simplify programming tasks. It includes data structures, algorithms, input/output functionalities, and more, allowing developers to focus on crafting their applications without reinventing the wheel.
Runtime Environment
The runtime environment is responsible for executing the compiled code. It handles crucial tasks, such as memory management, ensuring that the program runs efficiently and safely. When your program is executed, the runtime environment allocates memory for variables, manages the stack and heap, and facilitates interactions with the operating system.
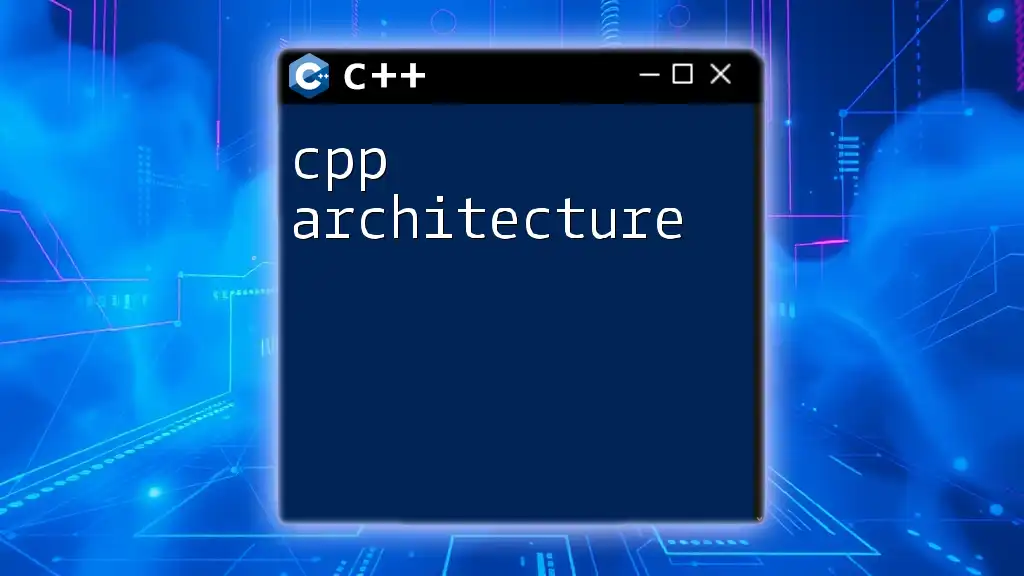
C++ Architectural Styles
Procedural Programming
Procedural programming in C++ focuses on writing procedures or functions that operate on data. This style emphasizes a step-by-step approach to solving problems, making it easier to read and maintain code. A simple function might look like this:
void process() {
// Implementation of the procedure
}
While functional, procedural code can become cumbersome as projects grow in complexity, leading to the need for more sophisticated architectural approaches.
Object-Oriented Programming (OOP)
Object-Oriented Programming revolutionizes C++ architecture by introducing the concepts of encapsulation, inheritance, and polymorphism. These principles enable developers to create modular, reusable, and maintainable code.
-
Encapsulation: This principle restricts direct access to some of an object's components, promoting reduced complexity. Here's a simple example:
class Box { private: int width; public: void setWidth(int w) { width = w; } };
-
Inheritance: This allows new classes to derive from existing classes, enhancing code reuse. For example:
class Shape { // Base class implementation }; class Circle : public Shape { // Derived class implementation };
-
Polymorphism: It allows methods to have different implementations based on the objects invoking them. Here's an illustration:
class Animal { public: virtual void sound() { } }; class Dog : public Animal { public: void sound() override { std::cout << "Bark"; } };
This high level of abstraction provided by OOP allows developers to think in terms of real-world objects, making complex systems easier to manage.
Generic Programming
Generic programming is a cornerstone of modern C++, facilitating code reusability. Utilizing templates allows you to write functions and classes that can operate with any data type. This is particularly powerful for creating collections or algorithms. Here's a simple example of a function template:
template <typename T>
T add(T a, T b) {
return a + b;
}
With this template, you can call `add` with different data types (like `int` or `double`) without needing to rewrite the function. This approach enhances both flexibility and efficiency in coding.
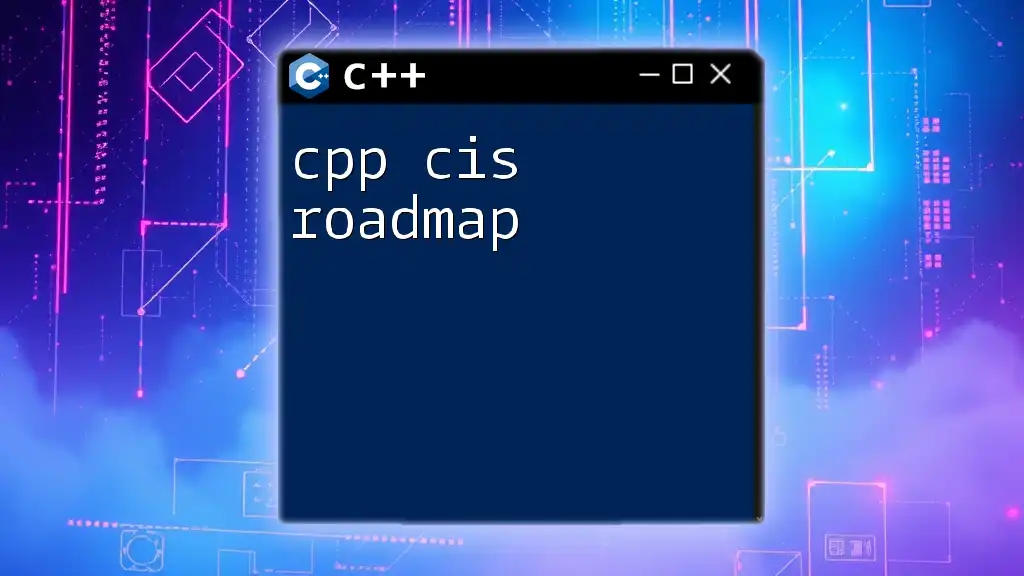
The Evolution of C++
Historical Overview
C++ has evolved significantly since its inception in the early 1980s. Starting as an enhancement to C, the language has grown to incorporate numerous paradigms. Major revisions have introduced new features and improvements, with standards like C++11, C++14, C++17, and C++20 adding powerful capabilities such as:
- Auto type deduction
- Range-based for loops
- Lambda expressions
- Smart pointers
Modern C++ Features
Understanding modern C++ features is essential to optimizing architecture design. Features like smart pointers help manage memory automatically, reducing the chances of memory leaks. For example, here's how you can use `std::unique_ptr`:
#include <iostream>
#include <memory>
void useSmartPointer() {
std::unique_ptr<int> ptr = std::make_unique<int>(5);
std::cout << *ptr << std::endl; // Outputs: 5
}
This reduces complexity in memory management and enhances safety in your applications.
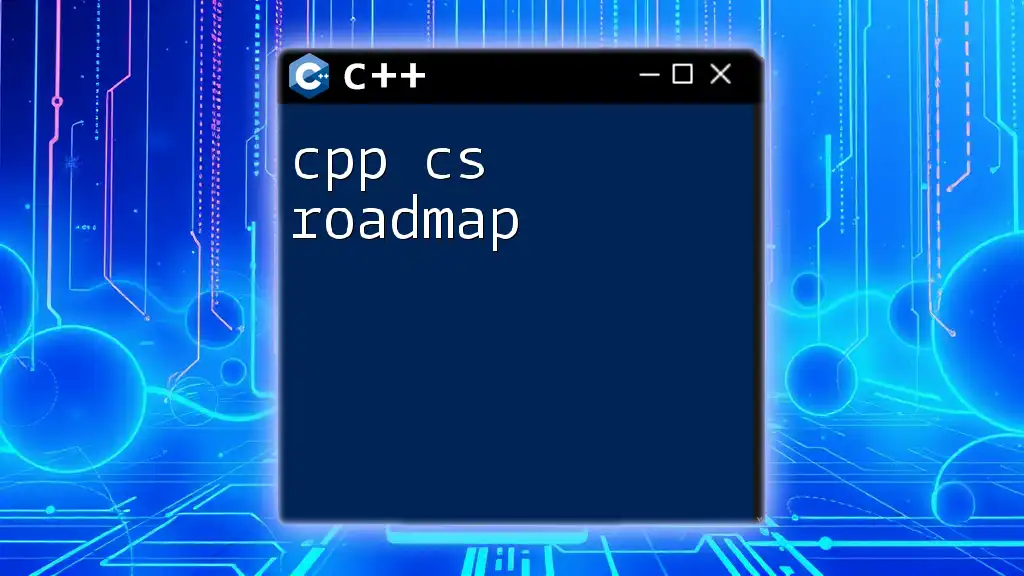
Building a C++ Architecture Roadmap
Learning Path
To master C++ architecture, consider developing the following skills:
- Understanding object-oriented design is critical for creating reusable code.
- Effective use of templates will allow you to maximize flexibility.
- Familiarity with memory management strategies to optimize performance.
- Leveraging the Standard Template Library (STL) for efficient data manipulation and algorithm implementation.
Resources
Books
Investing time in classic literature is crucial. Books like "Effective C++" by Scott Meyers provide insights into practical C++ programming and architectural principles.
Online Courses and Tutorials
There are numerous online resources available for learning C++ architecture. Look for reputable platforms that provide in-depth tutorials focused on both foundational concepts and modern practices.
Practical Applications
Understanding C++ architecture is not just of theoretical importance; it applies directly to various fields in software development. Applications can range from:
- Game Development: Utilizing efficient data structures and algorithms to manage complex systems.
- Systems Programming: Writing operating systems, drivers, and other system-level applications.
- Embedded Systems: Applying the principles of low-level programming in resource-constrained environments.
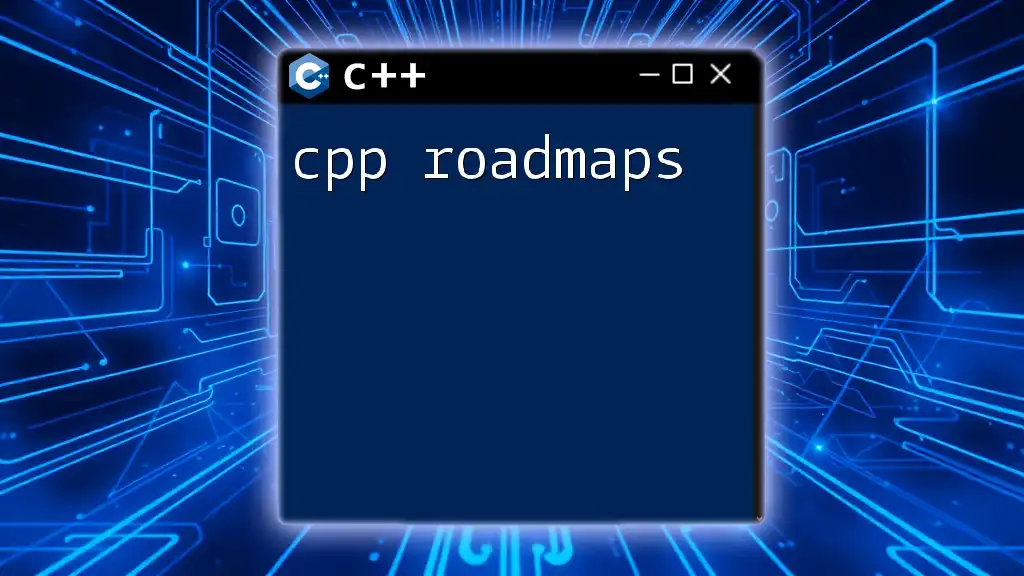
Conclusion
Grasping C++ architecture is fundamental for any developer looking to create efficient, maintainable, and scalable software. The cpp architecture roadmap outlined here serves as a guide to help you navigate the complexities of C++. Continuous learning and adaptation to advancements in C++ programming will undoubtedly cultivate expertise and proficiency. Engage with the community, share your knowledge, and grow in your C++ journey.