The "CPP Computer Science Roadmap" is a structured guide designed to help learners efficiently master C++ programming concepts and commands, enabling them to become proficient developers.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl; // Simple C++ command to print a message
return 0;
}
Understanding Core Concepts of C++
Basic Syntax and Structure
C++ syntax is the foundation upon which all C++ programs are built. Understanding this syntax is pivotal to writing any C++ code. A typical C++ program starts with a preprocessor directive, followed by the `main` function, which is the entry point of the program. For instance, here's a simple program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This snippet demonstrates an essential aspect of C++: the interaction with the standard output stream using `std::cout`.
Data Types and Variables
C++ offers a rich set of primitive data types, which include:
- `int` for integers
- `char` for single characters
- `float` and `double` for floating-point numbers
Declaring and initializing variables is straightforward. For example:
int age = 25;
char grade = 'A';
Here, `age` holds an integer value, and `grade` is a character variable. Understanding data types is crucial for efficient memory management and performance.
Operators in C++
Operators allow you to perform operations on variables and values in C++. Here are some significant types of operators:
- Arithmetic Operators: Used for basic calculations.
- Relational Operators: Manage comparisons between values.
- Logical Operators: Combine multiple conditions.
Consider the following example that uses arithmetic operators:
int result = (5 + 10) * 2;
The output will yield `30`, demonstrating the use of addition and multiplication in a single expression.
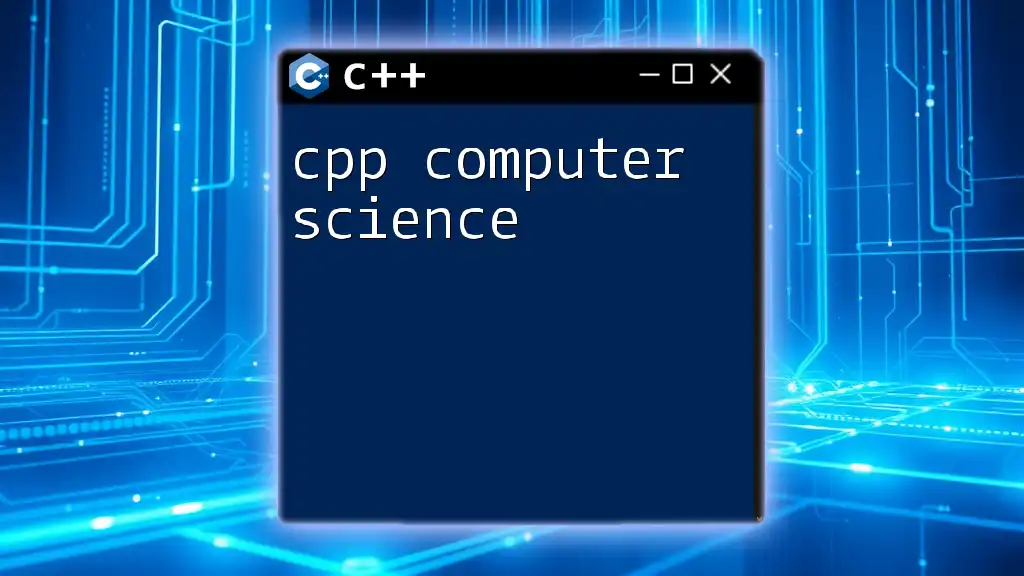
Fundamentals of C++ Programming
Control Structures
Control structures are used to dictate the flow of your programs based on conditions. C++ provides several types, including conditional statements like `if`, `else`, and `switch`.
Here’s how an `if-else` statement looks in practice:
if (age >= 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
In this code, if the variable `age` is 18 or above, the console will display "Adult"; otherwise, it will display "Minor".
Loops and Iteration
Loops enable you to execute a block of code multiple times. C++ has different types of loops, including `for`, `while`, and `do-while` loops. The `for` loop is frequently used for counting or iterating through an array.
Here is an example using a `for` loop to print numbers from 0 to 4:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
This loop iterates five times, demonstrating how to control loop variables effectively.
Functions and Scope
Functions are blocks of reusable code designed to perform a specific task. They take input parameters and return a value, providing a means to modularize your code effectively. For instance, consider this simple addition function:
int add(int a, int b) {
return a + b;
}
This function will take two integers as parameters and return their sum. Understanding how to create and call functions is critical for code maintainability.
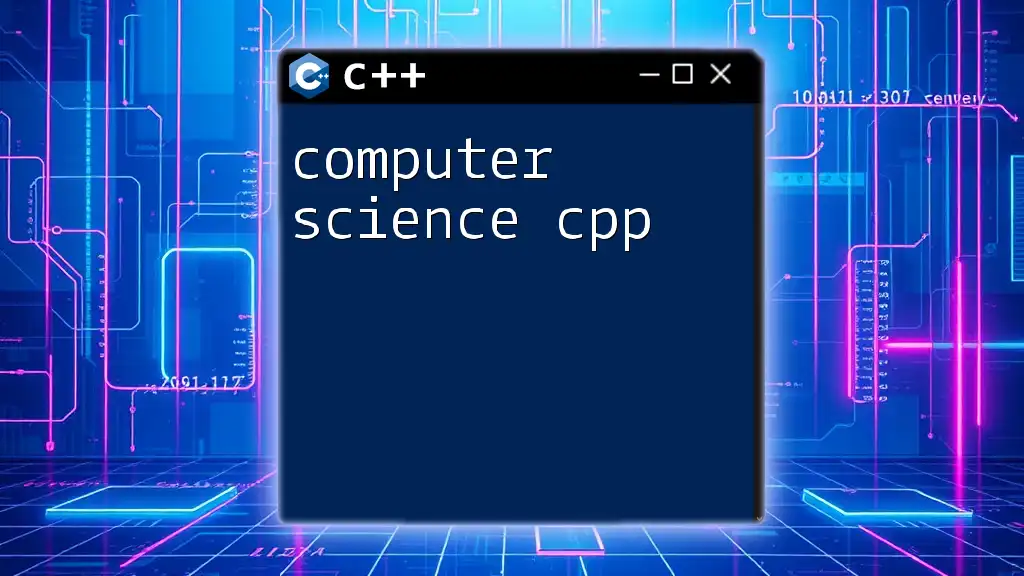
Advanced C++ Concepts
Object-Oriented Programming (OOP)
C++ supports object-oriented programming, which is a paradigm that enhances code organization and reusability. Key principles of OOP include:
- Encapsulation: Bundling data and methods within a class.
- Inheritance: Deriving new classes from existing ones.
- Polymorphism: Allowing methods to do different things based on the object it is acting upon.
Here’s a demonstration by creating a simple class:
class Animal {
public:
void speak() {
std::cout << "Animal speaks" << std::endl;
}
};
Animal myAnimal;
myAnimal.speak();
In this example, we define a class `Animal` and create an object `myAnimal` that invokes the `speak` function.
Templates in C++
Templates allow you to create generic functions or classes that work with any data type. This promotes code reusability and reduces redundancy. For example:
template <typename T>
T add(T a, T b) {
return a + b;
}
This template function allows addition of two numbers of any type—be it integers, floats, etc. This flexibility is one of the key strengths of C++.
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful set of C++ template classes to provide general-purpose classes and functions with templates. It includes a plethora of containers, algorithms, and iterators. Familiarizing yourself with STL can significantly improve your coding efficiency.
For instance, using a vector from STL could look like this:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
This snippet declares a vector of integers, illustrating how to manage dynamic arrays seamlessly.
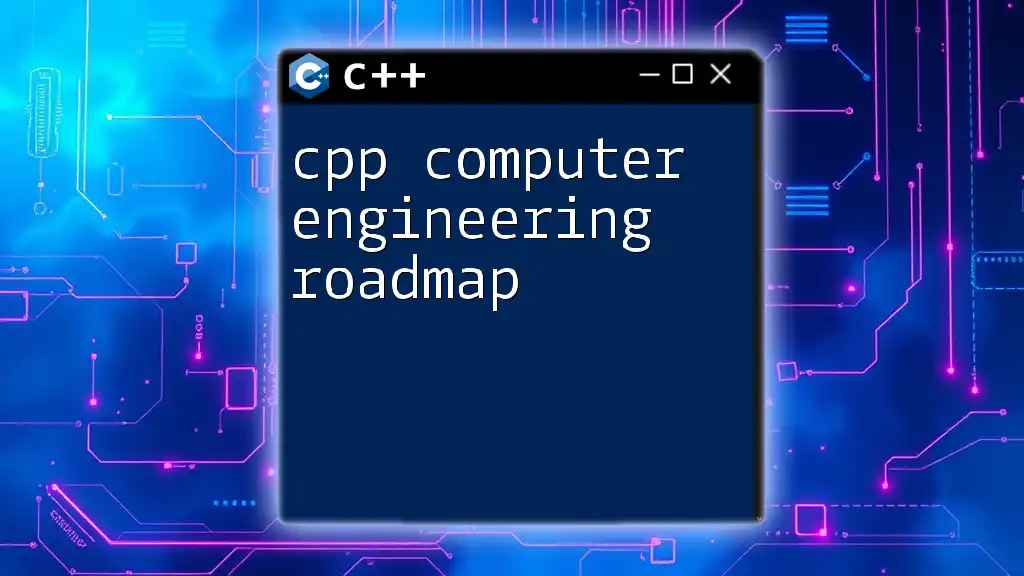
Practical Applications of C++
Game Development
C++ is extensively used in the gaming industry. Popular game engines like Unreal Engine are built on C++ due to its performance and control over system resources, enabling developers to optimize interactive content.
Systems Programming
C++ provides the tools necessary for low-level programming. It's often used in operating systems, embedded systems, and performance-critical applications where direct hardware manipulation or resource management is crucial.
Web Development
While C++ isn't the first language that comes to mind for web development, it can still play a pivotal role in backend services through applications like CGI (Common Gateway Interface) programming, where it can handle dynamic content generation.
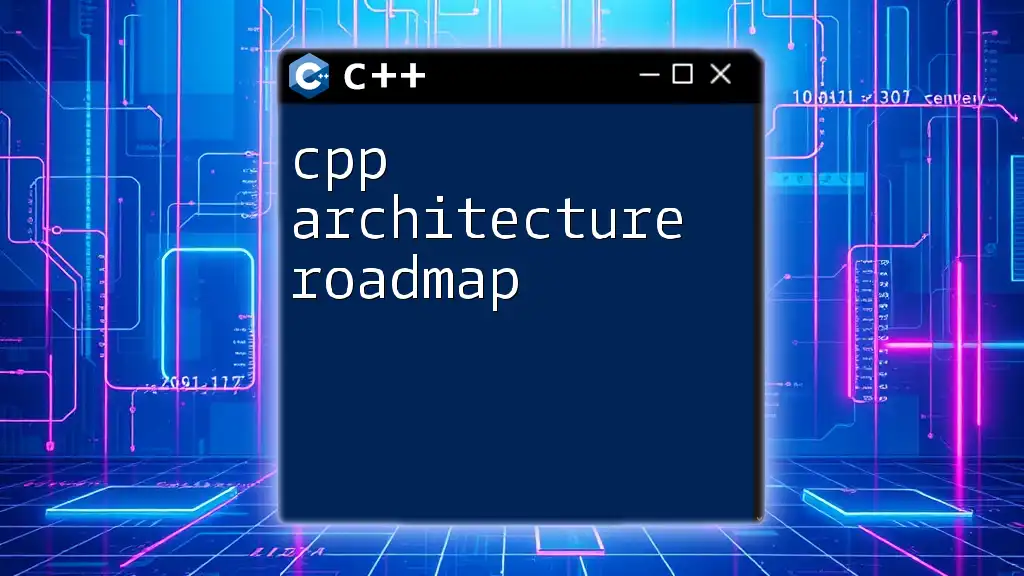
Resources for Further Learning
Books and Online Courses
To deepen your C++ knowledge, consider exploring comprehensive books such as "C++ Primer" or "Effective C++." Additionally, online platforms like Coursera or edX offer courses tailored to various skill levels.
Communities and Forums
Engaging with online communities can greatly enhance your learning experience. Platforms such as Stack Overflow and Reddit have vibrant discussion threads regarding C++ programming practices.
Practice Platforms
To hone your coding skills, websites like LeetCode and HackerRank provide a variety of coding challenges that focus on data structures and algorithms in C++. Regular practice can help bridge the gap between theoretical knowledge and practical application.
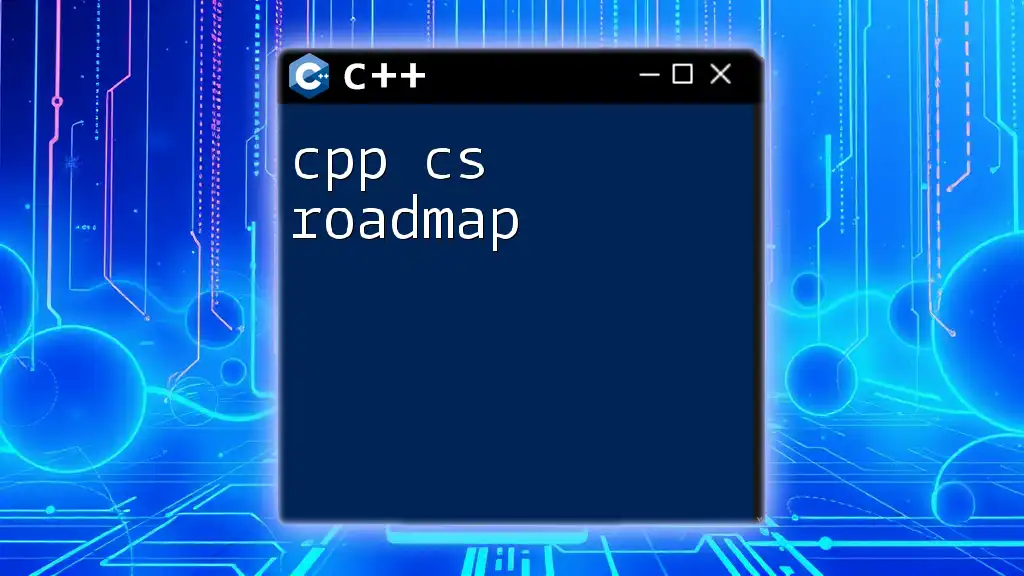
Conclusion
Mastering the C++ computer science roadmap requires dedication and continuous learning. As you build upon the foundation laid by basic concepts to advanced ones, remember that practice is key to developing your skills. Don’t hesitate to explore diverse applications of C++ in real-world scenarios.
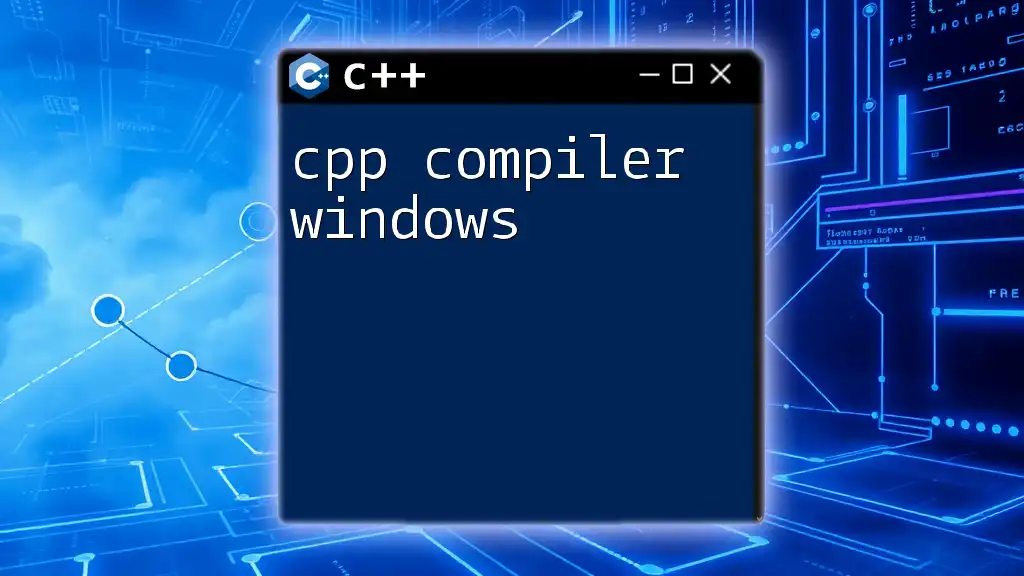
Call to Action
Share your C++ learning journey—what challenges you face or what successes you achieve. We encourage you to subscribe to our platform for the latest updates on tutorials and resources to support your growth in C++. Your path to mastery in C++ starts here!