The "C++ CS roadmap" outlines a structured approach for mastering C++ programming through key concepts, libraries, and effective coding practices.
Here’s a simple code snippet in C++ that demonstrates the syntax for defining a function and using it:
#include <iostream>
void greet() {
std::cout << "Hello, C++ World!" << std::endl;
}
int main() {
greet();
return 0;
}
Understanding C++ Basics
What is C++?
C++ is a versatile programming language that evolved from the C language, adding object-oriented features and rich library support. One of its key strengths lies in its ability to provide low-level memory manipulation while still allowing high-level programming constructs. As a member of the C family, C++ is widely used in software development, game development, systems programming, and applications requiring high performance.
Setting Up Your Environment
To begin your journey in C++, you first need an appropriate development environment. Some of the most popular Integrated Development Environments (IDEs) include:
- Visual Studio: Ideal for Windows users, it provides a comprehensive suite of development tools.
- Code::Blocks: A lightweight and cross-platform IDE that is easy to configure.
- CLion: A powerful IDE from JetBrains with excellent support for CMake projects.
Once you have chosen an IDE, you will also need to set up a C++ compiler. Common options are:
- GCC (GNU Compiler Collection): An open-source compiler available on most platforms.
- MSVC (Microsoft Visual C++): A compiler that is part of Visual Studio, ideal for Windows development.
- Clang: Known for its speed and is particularly useful for cross-platform development.
Here's an example of how to compile a simple C++ program using GCC in the command line:
g++ -o hello hello.cpp
This command compiles `hello.cpp` and produces an executable named `hello`.
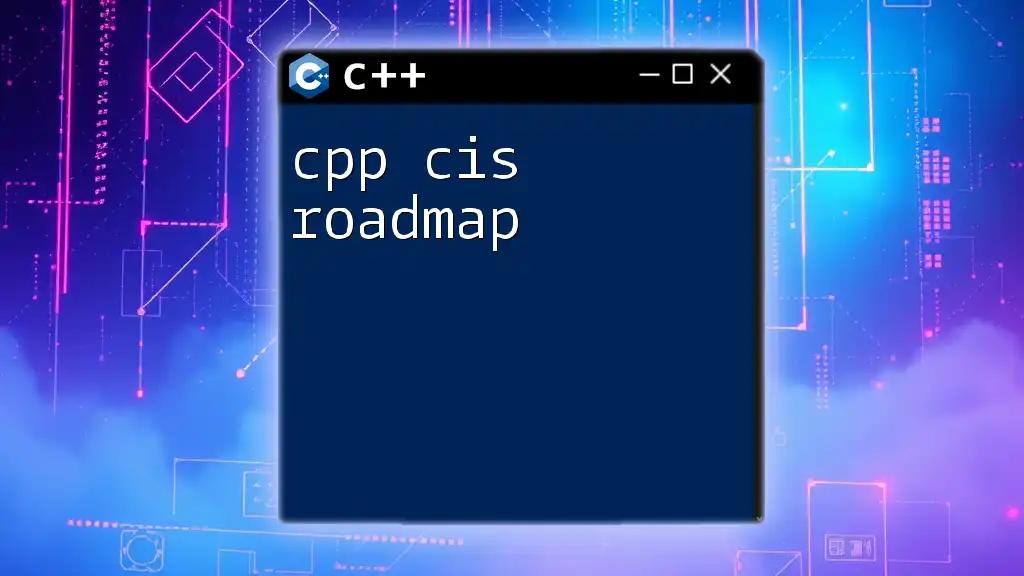
Fundamental C++ Commands
Basic Syntax
Understanding the basic syntax of C++ is crucial for writing effective code. C++ follows a structured approach, where every program starts with a `main` function. Here’s a simple "Hello, World!" program that illustrates this:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet, `#include <iostream>` allows us to use input/output features, while `std::cout` prints "Hello, World!" to the console.
Data Types and Variables
C++ supports various data types, including:
- int: Integer numbers
- float: Floating-point numbers
- char: Single characters
- string: Sequences of characters
To declare and initialize variables, use the following syntax:
int age = 25;
float salary = 55000.95;
Here, we have initialized an integer variable `age` and a floating-point variable `salary`.
Control Structures
Conditional Statements
Conditional statements allow for decision-making in code execution. The `if`, `else`, and `switch` statements serve this purpose. For example:
if (age < 18) {
std::cout << "Minor" << std::endl;
} else {
std::cout << "Adult" << std::endl;
}
This snippet checks if the `age` variable is less than 18 and outputs "Minor" or "Adult" based on the condition.
Loops
Loops are fundamental for repetitive tasks. In C++, `for`, `while`, and `do-while` loops are the primary types used. An example of a `for` loop iterating over an array would be:
for (int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
This loop prints the iteration count from 0 to 4.
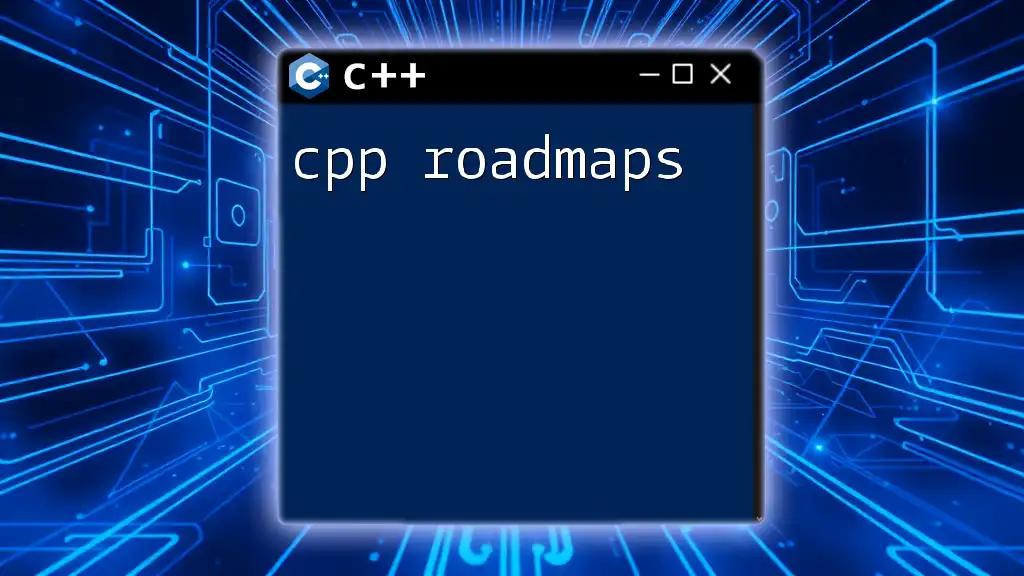
Advanced C++ Commands
Functions
Defining and Calling Functions
Functions help modularize code and improve readability. A function can be defined with parameters and a return type. Here’s how you can define and call a function that adds two integers:
int add(int a, int b) {
return a + b;
}
std::cout << add(5, 10) << std::endl;
The function `add` takes two integers as arguments and returns their sum.
Object-Oriented Programming
Classes and Objects
C++ supports object-oriented programming (OOP), which emphasizes data encapsulation through classes. Here is a simple example of a class:
class Car {
public:
void drive() {
std::cout << "Driving..." << std::endl;
}
};
Car myCar;
myCar.drive();
In this case, we define a class `Car`, and the method `drive` prints a message. We then create an object `myCar` of the class and call the `drive` method.
Inheritance and Polymorphism
Inheritance allows a class to inherit attributes and methods from another class, promoting code reuse. Additionally, polymorphism enables objects to be treated as instances of their parent class. Consider the following code:
class Animal {
public:
virtual void sound() {
std::cout << "Animal sound" << std::endl;
}
};
class Dog : public Animal {
public:
void sound() override {
std::cout << "Bark" << std::endl;
}
};
The `Animal` class has a virtual method `sound` that can be overridden in the `Dog` class. This approach allows different implementations of the same method.
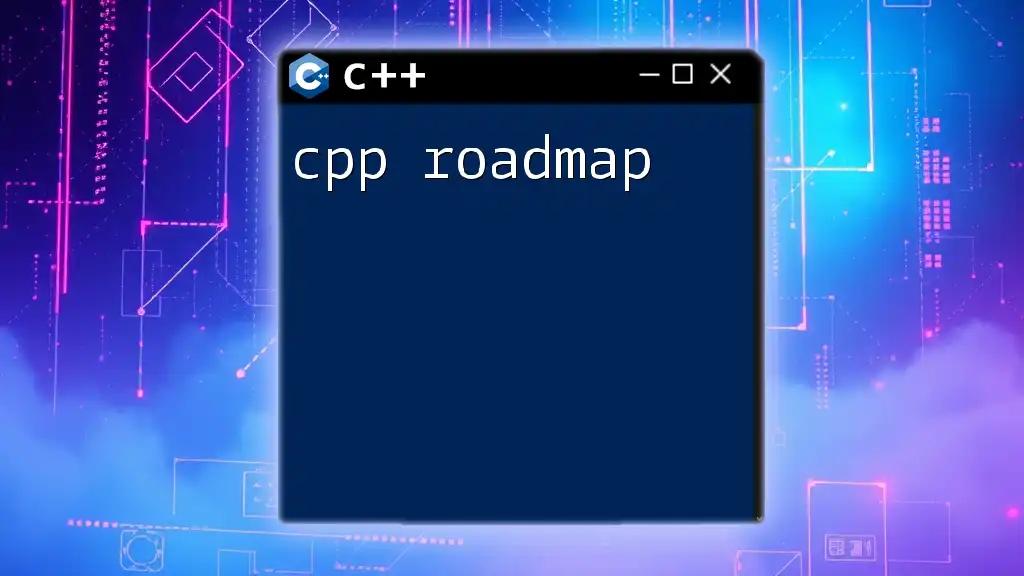
Working with Libraries and Frameworks
Standard Template Library (STL)
The Standard Template Library (STL) is an essential component of C++ that provides generic classes and functions, such as containers and algorithms. Commonly used containers include:
- vector: A dynamic array that can grow in size.
- map: A collection of key-value pairs.
Here’s a simple example of using a vector:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
In this snippet, we create a vector of integers initialized with five elements.
Boost Library
Boost is a powerful, peer-reviewed library that extends C++ capabilities. It provides numerous portable components, ranging from mathematical functions to multithreading support. Here’s an example using Boost to convert a string to uppercase:
#include <boost/algorithm/string.hpp>
std::string str = "Boost Libraries";
boost::to_upper(str);
This example utilizes the Boost's string algorithms to transform `str` into uppercase.
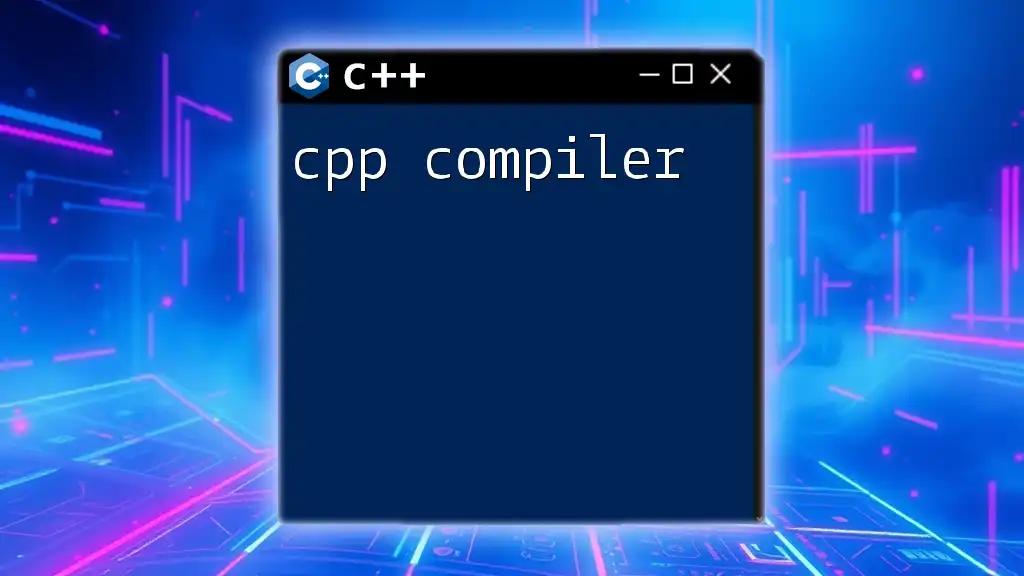
Best Practices and Optimization Techniques
Code Clarity and Readability
Writing clean and maintainable code is crucial for collaborative projects and long-term maintenance. Here are some tips:
- Use meaningful variable names.
- Comment your code judiciously.
- Structure your code logically, separating functionalities into distinct functions and classes.
Performance Optimization
Optimizing C++ code leads to improved performance, especially in resource-intensive applications. Here are some techniques to consider:
- Memory Management: Always initialize pointers and consider using smart pointers to avoid memory leaks.
- Algorithm Efficiency: Choose the appropriate algorithms and data structures to improve execution time and resource usage.
- Inline Functions: Use inline functions for small functions to reduce the overhead of function calls.
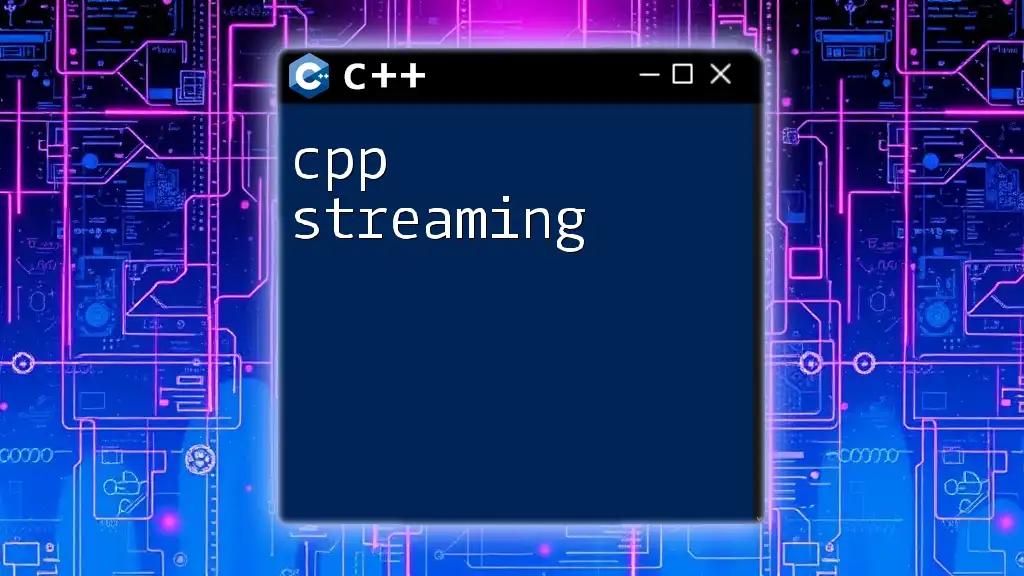
Conclusion
This roadmap has taken you through the fundamental and advanced C++ commands, providing you with a solid foundation for your coding journey. Mastering these commands will increase your programming efficiency and open doors to more complex projects.
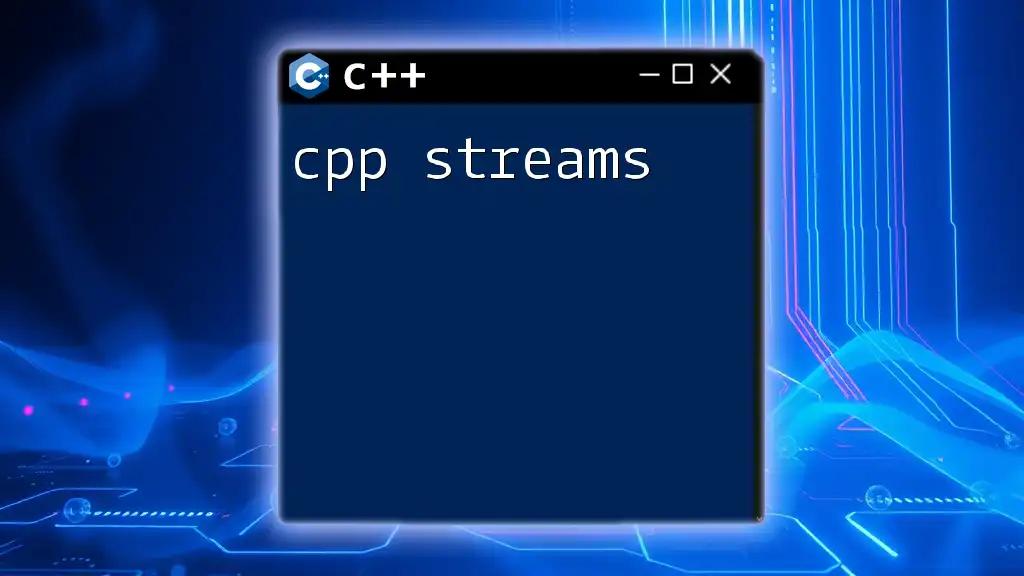
Call to Action
We encourage you to share your thoughts and experiences as you delve into the world of C++. Your insights can help others along their journey. Also, feel free to sign up for our upcoming tutorials where we will delve deeper into using C++ commands effectively!