The `cpp chrono` library provides a set of utilities for measuring time intervals and manipulating durations, making it easier to work with time-related functionalities in C++.
Here’s a simple code snippet showing how to use `std::chrono` to measure the execution time of a code block:
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::high_resolution_clock::now();
// Code block to measure
for (int i = 0; i < 1000000; ++i); // Just an example loop
auto end = std::chrono::high_resolution_clock::now();
std::chrono::duration<double> duration = end - start;
std::cout << "Execution time: " << duration.count() << " seconds" << std::endl;
return 0;
}
What is C++ Chrono?
C++ Chrono is a powerful library that comes from the C++11 standard, designed for manipulating time durations, points on a timeline, and clocks. Its primary purpose is to offer robust abstractions for time and high-resolution clocks, making it essential for applications that require precision timing, such as performance measurement or timing events.
Why Use Chrono in C++?
Chrono provides several advantages over traditional time-handling methods:
- Precision: It offers high-resolution clocks that can measure short durations accurately.
- Type Safety: With specific types for durations, time points, and clocks, you minimize errors that often occur with raw time values.
- Ease of Use: The API is designed to be intuitive, reducing the complexity of working with time-related functionality.
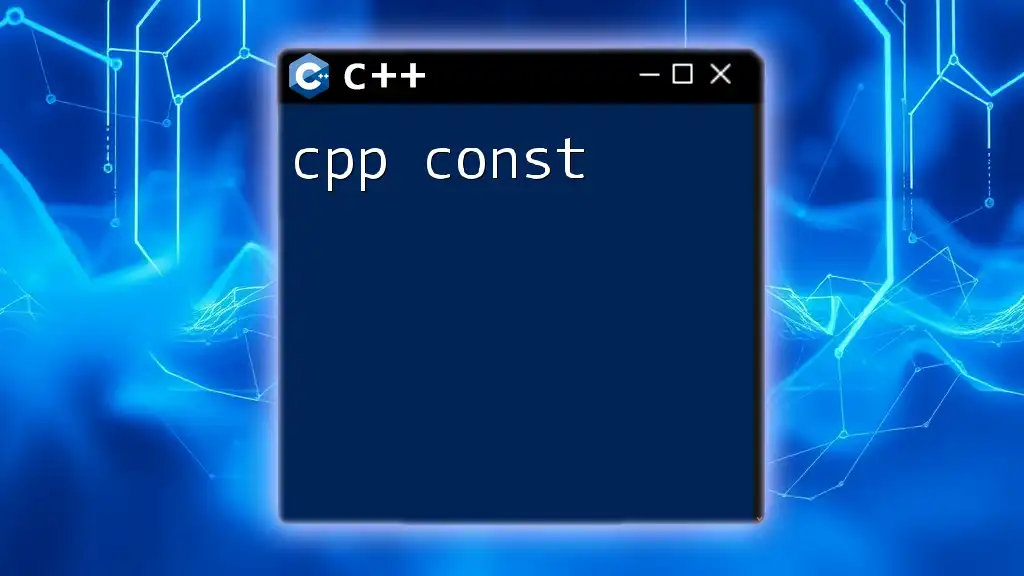
Understanding the Basics of C++ std::chrono
What is std::chrono?
The std::chrono namespace is part of the standard library in C++. It consists of several components essential for time manipulation. The main building blocks of std::chrono include:
- Durations: Represent intervals of time, such as seconds, milliseconds, and hours.
- Time Points: Specific points in time based on a certain clock.
- Clocks: Mechanisms to represent time. Examples include `steady_clock`, `system_clock`, and `high_resolution_clock`.
Key Features of std::chrono
Understanding the key concepts such as durations, time points, and clocks is vital for effective time manipulation.
Durations
Durations define the elapsed time in specific units. You can create durations like this:
#include <chrono>
std::chrono::seconds seconds(10); // 10 seconds
std::chrono::milliseconds milliseconds(100); // 100 milliseconds
std::chrono::microseconds microseconds(1000); // 1000 microseconds (1 millisecond)
Time Points
A time point represents a point on a timeline. You can create a time point using `system_clock`, for example:
auto now = std::chrono::system_clock::now();
Clocks
C++ provides three primary clocks:
- steady_clock: A clock that is guaranteed to be monotonically increasing.
- system_clock: Represents the system-wide real-time clock.
- high_resolution_clock: Provides the highest available precision in terms of time measurement.
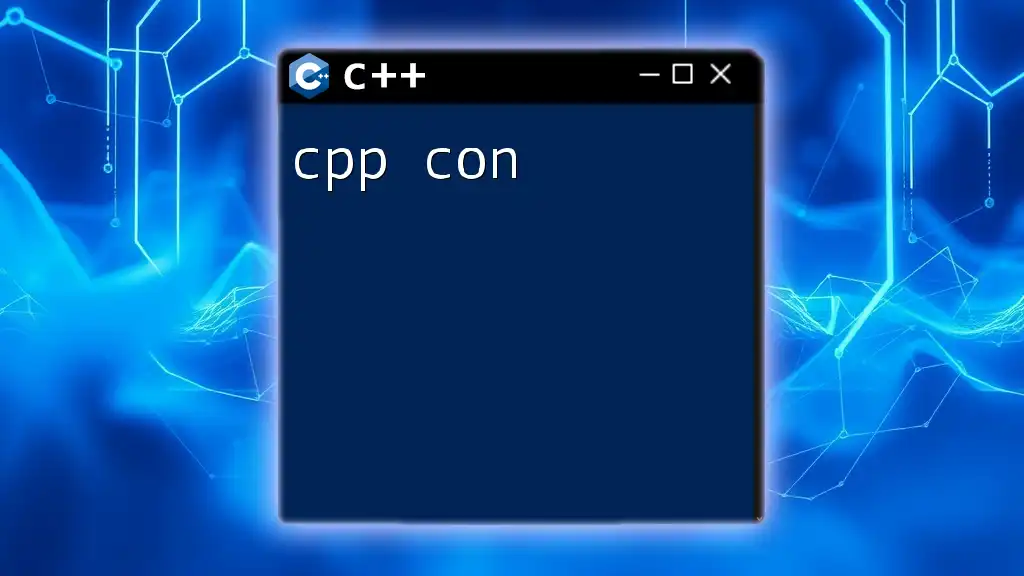
Working with Duration in C++
What is a Duration?
A duration in C++ represents a span of time. It allows you to express time intervals using different units like seconds, milliseconds, etc. Utilizing durations enables you to perform clock-related arithmetic and comparisons effectively.
Creating and Using Durations
You can create and manipulate durations in various units. For instance:
auto duration1 = std::chrono::seconds(60); // 60 seconds
auto duration2 = std::chrono::milliseconds(500); // 500 milliseconds
Arithmetic Operations with Durations
Performing arithmetic with durations is straightforward. You can add or subtract durations just as you would with integers:
auto total_duration = duration1 + duration2; // Adds durations
This ability to manipulate durations is especially beneficial for calculating elapsed time for operations or tasks.
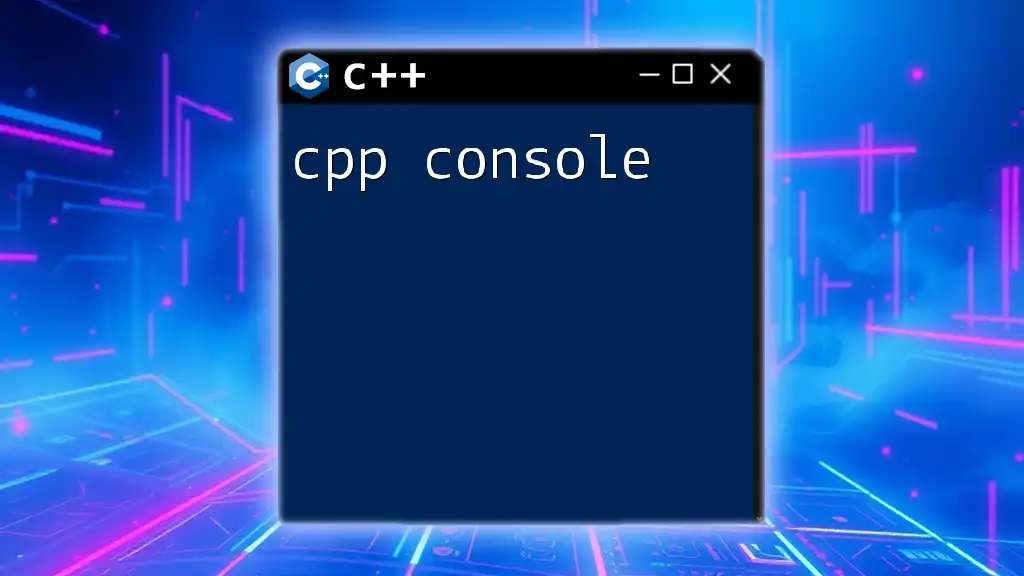
Time Points in C++ Chrono
Understanding Time Points
Time points denote a specific moment in time, based on a clock. They are essential when you need to timestamp events, like measuring the start and end of a process.
Creating Time Points with std::chrono
Creating a time point is as simple as grabbing the current time:
auto start_time = std::chrono::steady_clock::now(); // Getting the current time point
Time Point Calculations
To find the difference between two time points, you can use the following example:
auto end_time = std::chrono::steady_clock::now();
auto elapsed_time = end_time - start_time; // Duration object
This measure of elapsed time can provide insights into event durations, helping in profiling code execution.
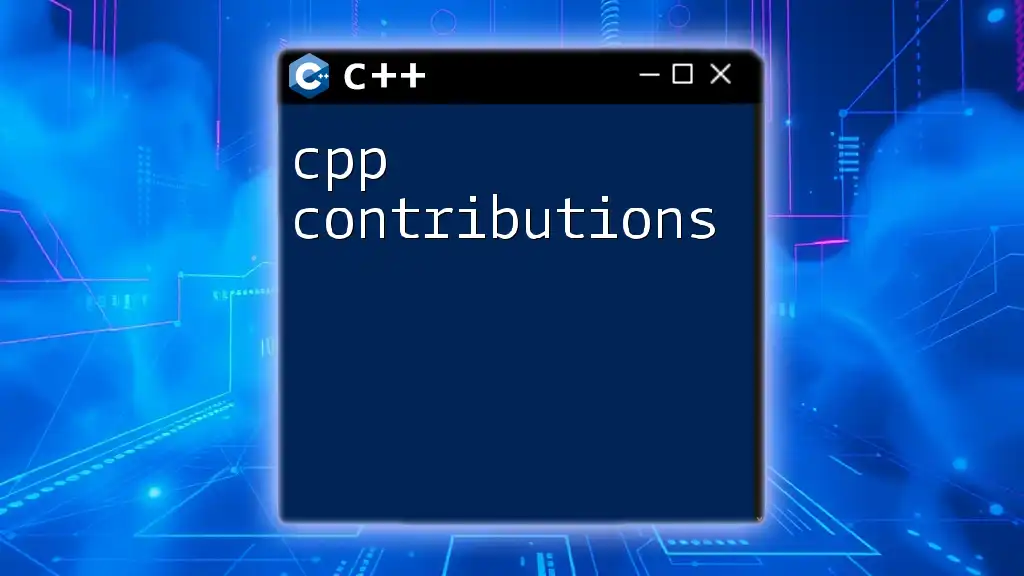
C++ Clocks: Getting Current Time
Introduction to C++ Clocks
C++ clocks provide different mechanisms to access and represent time. Selecting the right clock is vital depending on your requirements.
Using steady_clock
The `steady_clock` is perfect for measuring the execution duration of code:
auto start = std::chrono::steady_clock::now();
// ... your code ...
auto end = std::chrono::steady_clock::now();
auto elapsed = end - start; // Measure duration
Using system_clock
The `system_clock` can be used to fetch wall-clock time:
auto current_time = std::chrono::system_clock::now();
// Convert to time_t to output
std::time_t now_c = std::chrono::system_clock::to_time_t(current_time);
Understanding the differences between these clocks ensures that you select the appropriate clock for your specific timing needs.
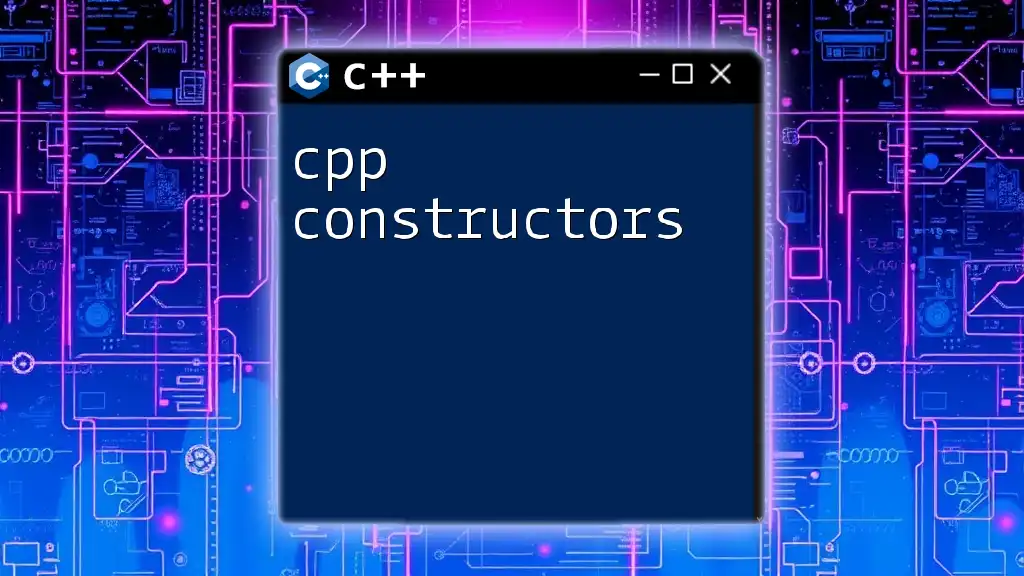
Practical Applications of C++ Chrono
Benchmarking Functions
Benchmarking is vital in software development, and the chrono library greatly aids this. By measuring execution time accurately, developers can identify bottlenecks in code.
#include <chrono>
void function_to_benchmark() {
// Your code logic
}
auto start = std::chrono::steady_clock::now();
function_to_benchmark();
auto end = std::chrono::steady_clock::now();
std::chrono::duration<double> elapsed_seconds = end - start;
std::cout << "Function executed in: " << elapsed_seconds.count() << " seconds\n";
Timeouts and Delays
For applications that need to wait or delay certain actions, chrono makes it easy. For example, you can implement a delay using:
#include <thread>
std::this_thread::sleep_for(std::chrono::seconds(1)); // Delays for 1 second
Scheduling Tasks
You can also schedule tasks using durations to ensure actions occur at specific intervals. For example:
while(true) {
// Code to execute periodic tasks
std::this_thread::sleep_for(std::chrono::milliseconds(1000)); // 1-second intervals
}
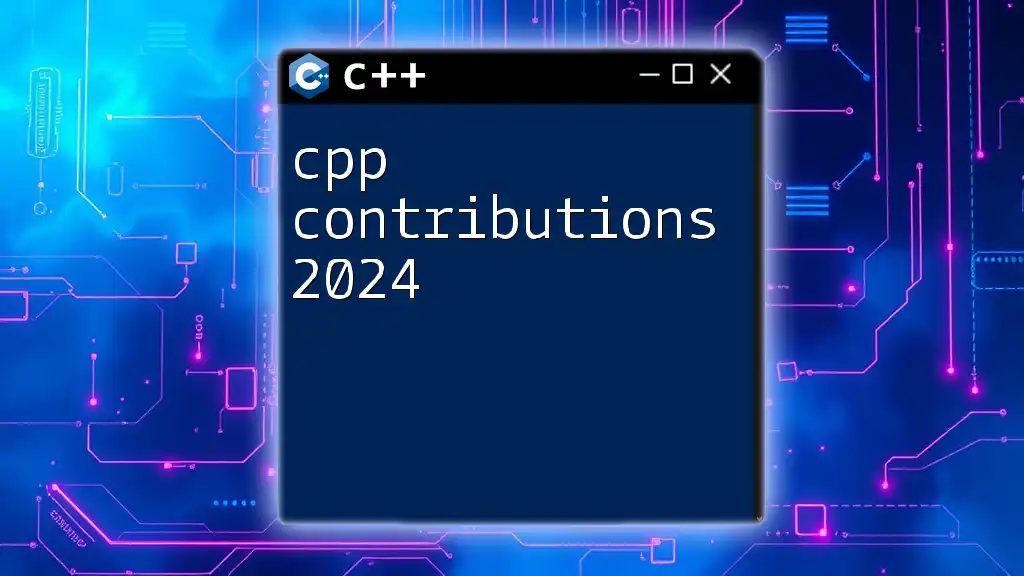
Best Practices When Using C++ Chrono
Choosing the Right Clock
When working with clocks, it’s essential to pick the right one:
- Use steady_clock for measuring durations, as it never goes backwards.
- Opt for system_clock when you need real-world time, such as logging timestamps.
Precision and Performance Considerations
Understanding precision limits helps developers avoid potential pitfalls. For instance, microsecond precision may not be necessary, and using `steady_clock` will incur some overhead. Always balance the need for precision with performance requirements.
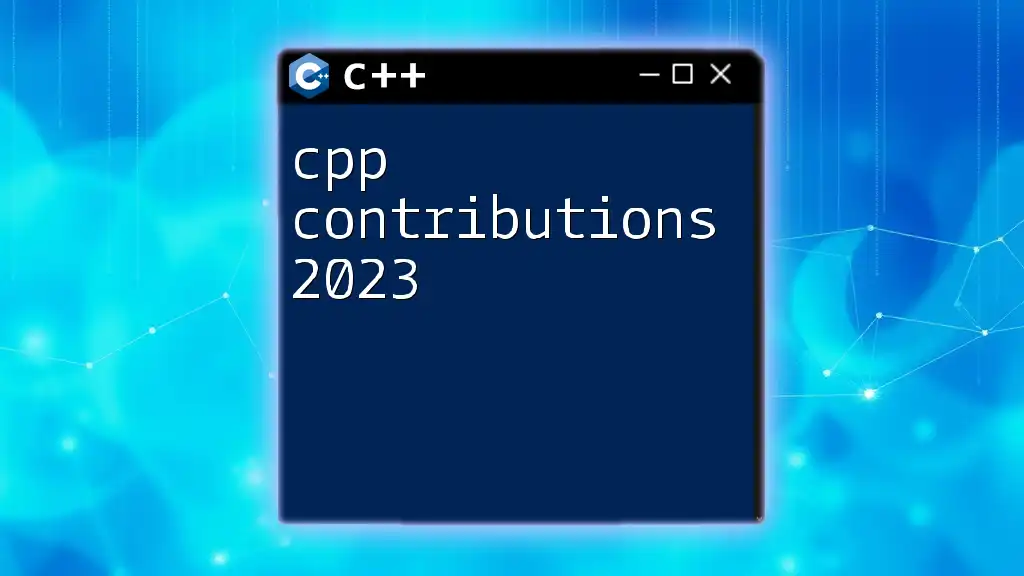
Common Pitfalls with C++ Chrono
Understanding Time Zones and Limits
A common mistake is misunderstanding the difference between system and steady clocks. The `system_clock` can change, while the `steady_clock` remains constant. Ensuring you use the correct clock can save you from subtle bugs in time-related computations.
Overhead of Chrono Functions
Although chrono functions provide better precision, utilizing them excessively for very short durations can introduce overhead. It’s vital to weigh whether the precision enhances your application or simply adds unnecessary computation overhead.
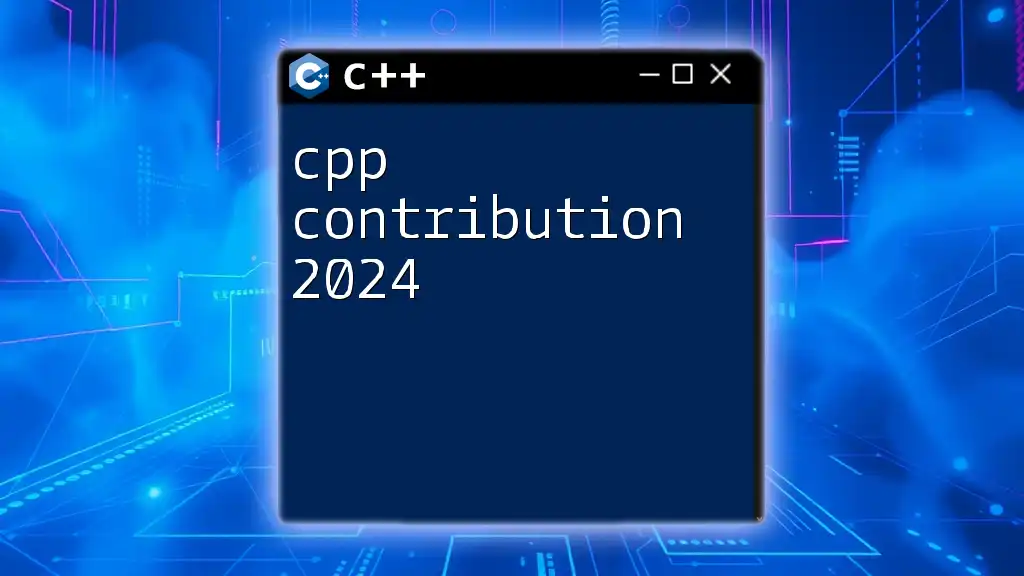
Conclusion
In summary, C++ Chrono provides a flexible and powerful way to work with time in your applications. With its ease of use, type safety, and high precision, it’s an invaluable tool for both casual programming and critical performance measurements. To deepen your understanding, practice with real-world examples and experiment with the different components of the chrono library.
Further Reading and Resources
For additional guidance, consider visiting the official C++ documentation and exploring tutorials that delve deeper into the functionalities provided by C++ chrono.
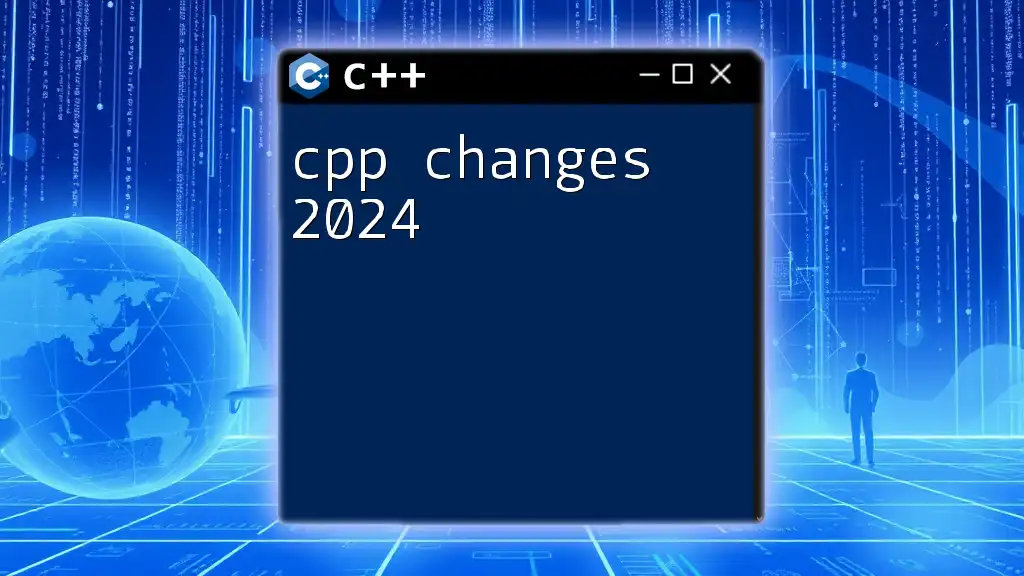
Call to Action
Join us to enhance your C++ programming skills further! Subscribe to our platform for more informative articles, tips, and tricks designed to help you master C++ and other programming languages.