Discover our cpp courses designed to teach you efficient C++ commands quickly and concisely, empowering you to master the language with practical examples.
Here's a simple code snippet demonstrating how to output text in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Commands
What are C++ Commands?
C++ commands are the essential statements that compose C++ programs. They govern program behavior by defining functions, control structures, input/output operations, and much more. Mastering these commands is crucial for anyone aspiring to write effective C++ code.
Types of C++ Commands
Control Structures Control structures dictate the flow of execution within a program. This includes conditional statements like `if` and `switch`, as well as looping constructs such as `for`, `while`, and `do while`. Effective use of these structures can significantly enhance the logic of your program.
Example:
int main() {
int number = 10;
if (number > 5) {
cout << "Number is greater than 5" << endl;
}
return 0;
}
Input/Output Commands Input and output operations in C++ are primarily handled using `cin` and `cout`. Understanding how to manipulate these commands is vital for user interaction in console applications.
Example:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "You are " << age << " years old." << endl;
return 0;
}
Functions and Operations Functions are blocks of reusable code that perform specific tasks. They can take parameters and return values, providing a way to organize code logically and efficiently.
Example:
int add(int a, int b) {
return a + b;
}
int main() {
cout << "Sum: " << add(5, 3) << endl;
return 0;
}
Object-Oriented Commands C++ is famous for its object-oriented features like classes and inheritance. Learning to define and utilize these concepts can help you structure your programs effectively.
Example:
class Animal {
public:
void speak() {
cout << "Animal speaks" << endl;
}
};
int main() {
Animal myAnimal;
myAnimal.speak();
return 0;
}
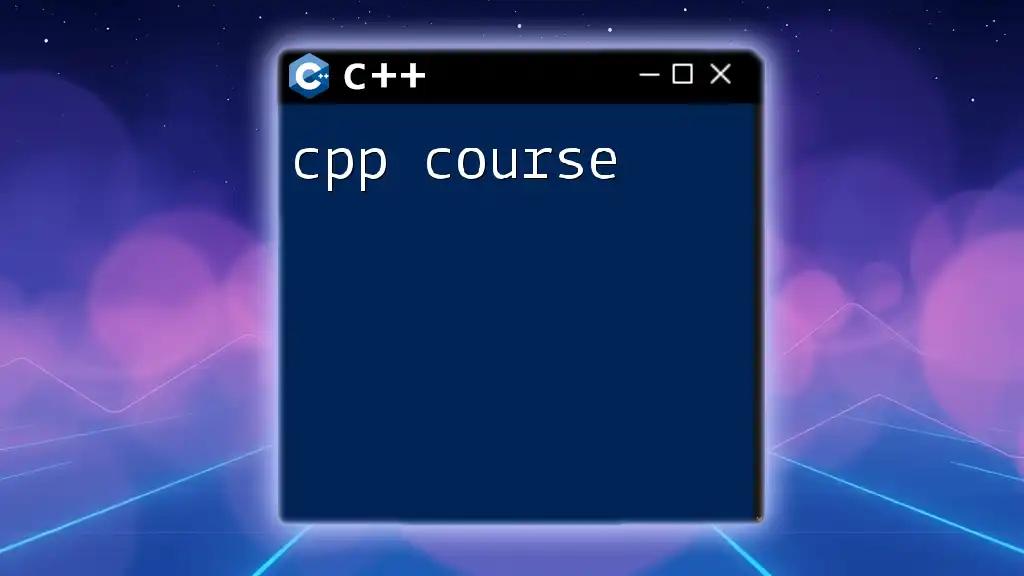
The Importance of C++ Courses
Why Learn C++?
C++ is a versatile programming language used in various fields, from software development to game design and embedded systems. The demand for C++ skills is consistently high, making it a lucrative language to learn.
Benefits of Structured Learning
Structured courses provide a systematic approach to mastering C++. They teach you best practices, help avoid common pitfalls, and offer a clear curriculum that guides you from fundamental concepts to advanced topics.

Types of C++ Courses Available
Online Courses
With numerous platforms offering C++ courses, such as Coursera, Udemy, and edX, learners have access to a wealth of resources. Each platform has its unique features, such as video content, quizzes, and community discussion forums. Research and compare courses based on duration, pricing, and user feedback to find the best fit for your learning style.
In-Person Workshops
In-person workshops can provide the benefits of direct interaction, immediate feedback, and hands-on experience. These settings often foster collaboration, making them ideal for team learning. Many attendees appreciate the opportunity to network with instructors and peers alike.
Bootcamps
C++ bootcamps offer intense, immersive experiences geared toward rapid skill acquisition. These are especially beneficial for those looking to transition into tech careers quickly. Bootcamps often culminate in hands-on projects where participants can apply their new skills in practical settings.
Choosing the Right Course
Assessing Your Learning Style
Before selecting a course, consider whether you prefer a self-paced learning model or a more traditional, instructor-led format. Your engagement level with the material and interactivity provided in the course can significantly affect your learning experience.
Skill Level Considerations
Identify where you stand on the C++ proficiency spectrum. Are you an absolute beginner needing foundational lessons, or an intermediate learner seeking to expand your skill set? Tailoring your course selection based on your existing knowledge ensures you won’t feel overwhelmed or under-challenged.
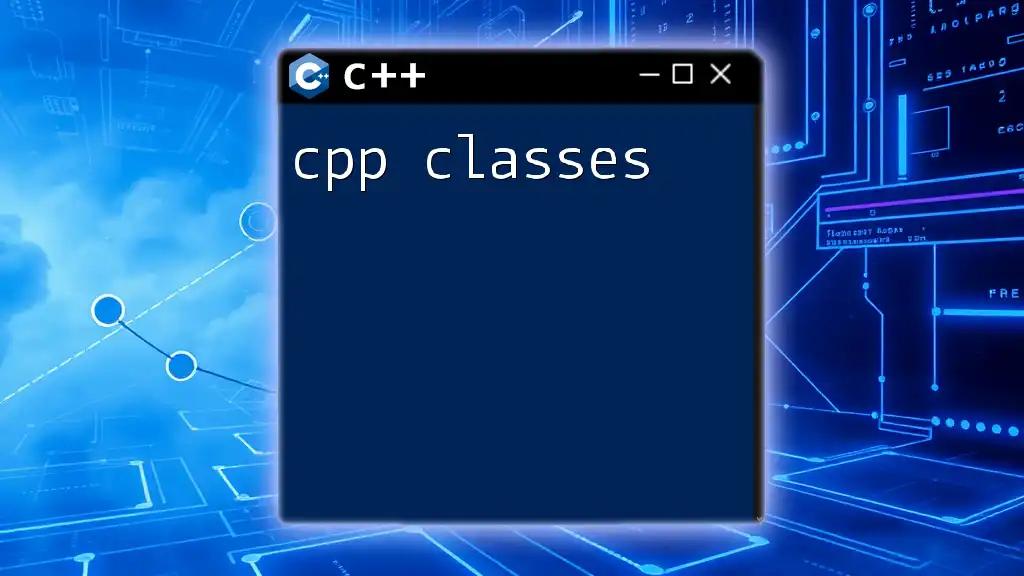
Course Content Breakdown
Fundamental Concepts Covered in C++ Courses
Though course content may vary, most C++ courses cover foundational concepts such as variables, data types, and operators.
Variables, Data Types, and Operators
Understanding how to declare variables and utilize different data types (like `int`, `char`, and `float`) is crucial in writing C++ programs. Operators play a significant role in performing computations and comparisons.
Example:
int main() {
int a = 5, b = 3;
cout << "Sum: " << (a + b) << endl;
return 0;
}
Control Flow Statements
Control flow statements allow you to implement conditions and loops, aiding in decision-making within your program.
Function Basics
Courses typically teach you how to define and call functions. This fundamental knowledge fosters better code organization and reuse.
Object-Oriented Programming Fundamentals
Understanding concepts like classes, objects, encapsulation, inheritance, and polymorphism is paramount in C++.
Advanced Topics
Templates and STL
C++ templates allow functions and classes to operate with generic types. The Standard Template Library (STL) simplifies common algorithms and data structure management, enhancing programming efficiency.
Example:
#include <iostream>
#include <vector>
using namespace std;
template <typename T>
void printVector(const vector<T>& v) {
for (const auto& item : v) {
cout << item << " ";
}
cout << endl;
}
int main() {
vector<int> intVec = {1, 2, 3, 4};
printVector(intVec);
return 0;
}
Memory Management
Proficiency in memory management is crucial in C++, where you manually allocate and deallocate memory. This knowledge helps prevent memory leaks and optimizes performance.
Example:
#include <iostream>
using namespace std;
int main() {
int* p = new int(10); // dynamic allocation
cout << *p << endl; // access value
delete p; // deallocate
return 0;
}
File Handling in C++
C++ includes functionalities to read from and write to files, enabling data persistence beyond program execution.
Example:
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ofstream outfile("example.txt");
outfile << "Hello, file!" << endl;
outfile.close();
string line;
ifstream infile("example.txt");
while (getline(infile, line)) {
cout << line << endl;
}
infile.close();
return 0;
}
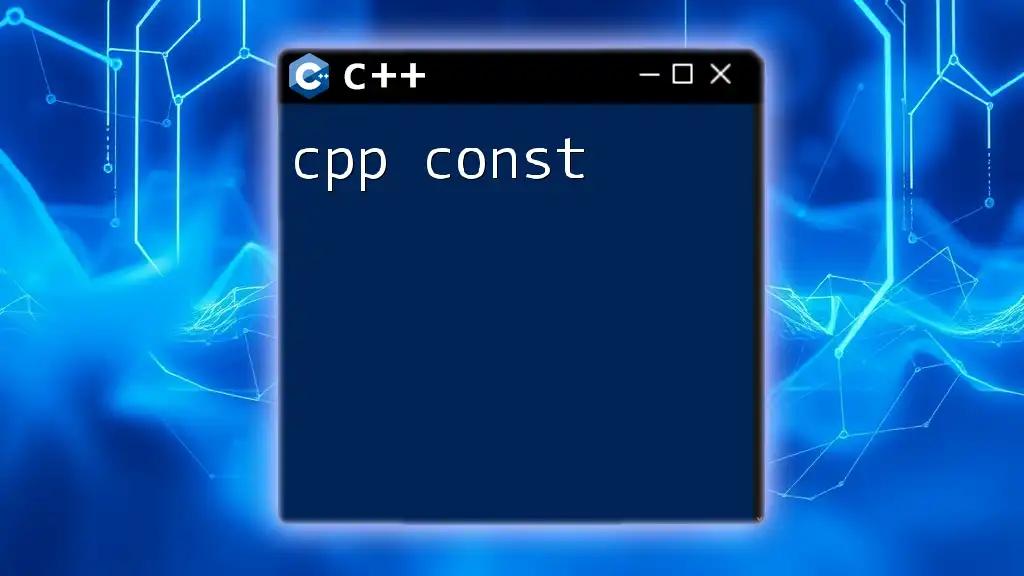
Learning Resources
Books and Online References
Numerous books cater to distinct skill levels, from "C++ Primer" for beginners to more advanced texts like "Effective Modern C++". Consider complementing your course with these recommended readings.
Community and Forums
Engage with communities such as Stack Overflow or specialized Reddit communities. Being part of a community allows you to ask questions, share insights, and learn from others’ experiences.
Video Tutorials and Free Resources
Leverage quality video lessons available on platforms like YouTube or freeCodeCamp. These resources provide a more engaging visual context to your learning.
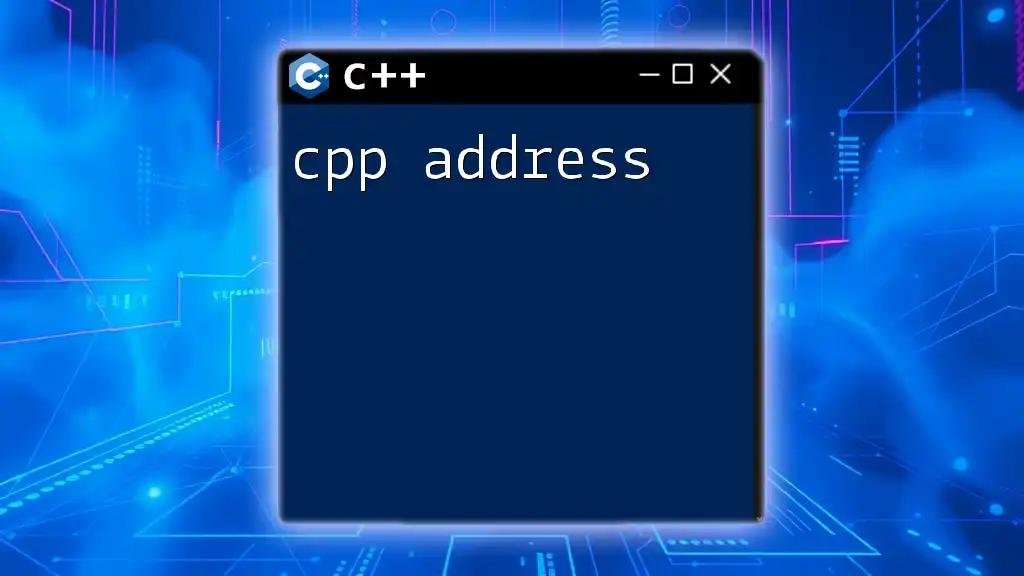
Tips for Success in C++ Learning
Practice Regularly
Consistent practice is essential for mastering C++. Aim to code every day, even if it’s just a simple exercise or a small project challenge. Applying what you've learned will reinforce your knowledge.
Engage with the Community
Build a network of peers and mentors. Participation in coding forums and local meetups can enhance your learning experience while providing opportunities for collaboration.
Leverage Online Tools
Familiarize yourself with Integrated Development Environments (IDEs) and online code playgrounds, like Code::Blocks or Replit. These tools can streamline your coding process and improve your efficiency.
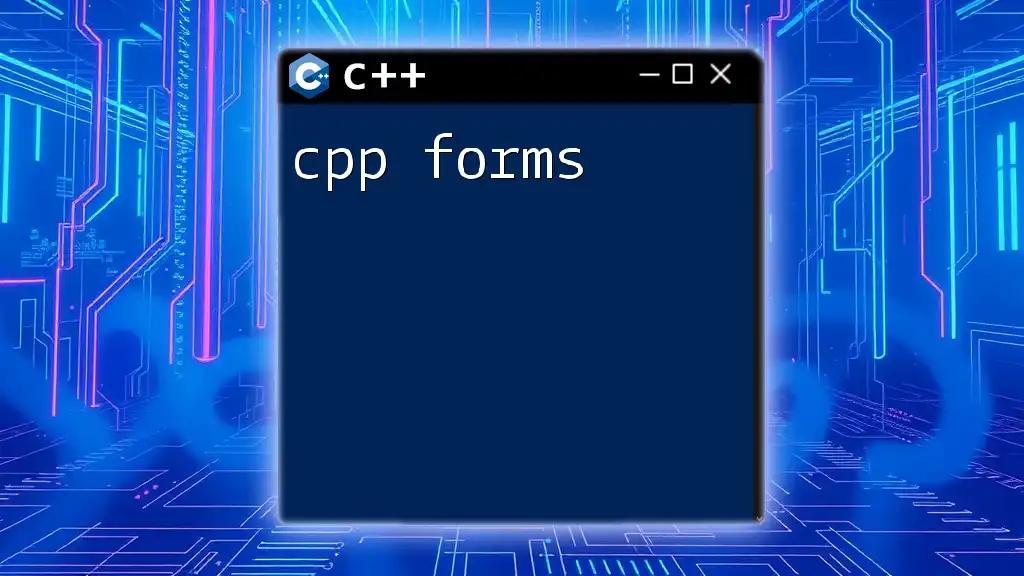
Conclusion
Embarking on the journey to learn C++ through structured cpp courses offers you a solid foundation for navigating the programming world. With its diverse applications and robust community support, mastering C++ opens numerous professional doors and opportunities for growth.
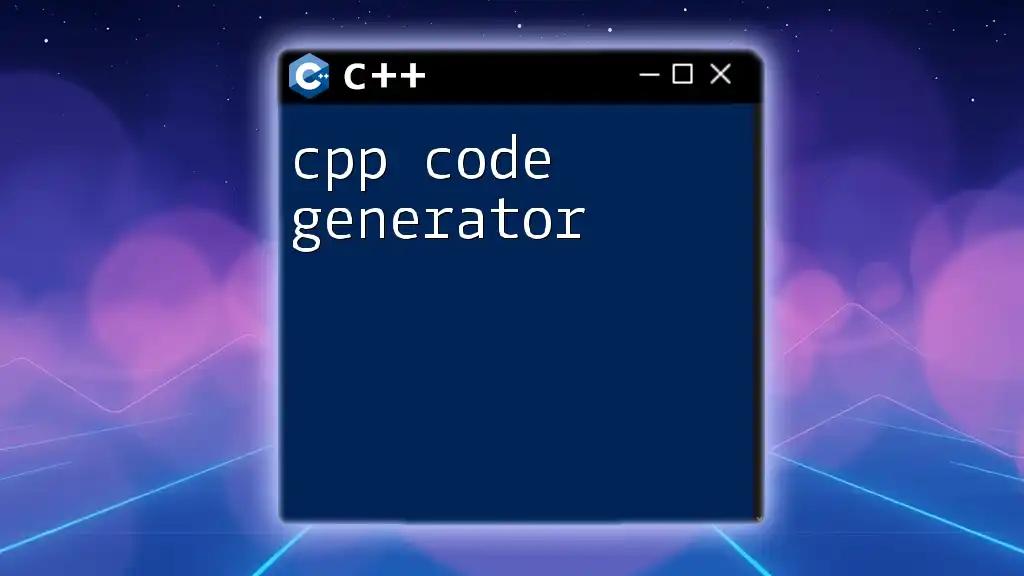
Call to Action
Are you ready to dive into the world of C++? Explore our curated cpp courses tailored to your learning style and advance your programming journey today. Sign up for our newsletters to stay updated on new resources and learning opportunities!
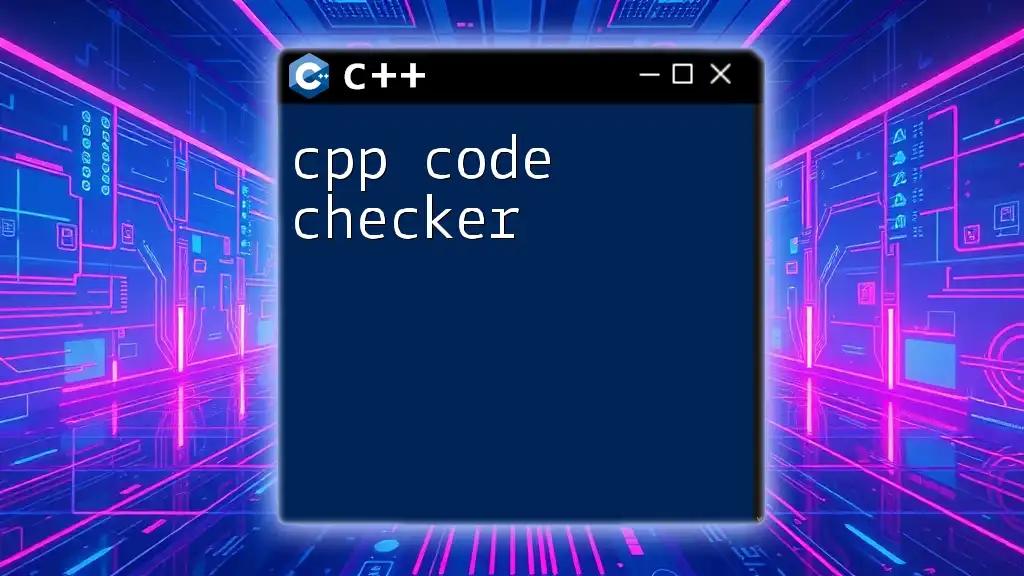
Appendix
Glossary of C++ Terms
- Class: A user-defined data type that represents real-world entities with properties and methods.
- Object: An instance of a class.
- Template: A feature that allows functions and classes to work with any data type.
Links to Recommended Courses and Resources
- [Udemy C++ Courses](https://www.udemy.com/topic/c-plus-plus/)
- [Coursera C++ Courses](https://www.coursera.org/courses?query=c%2B%2B)
Further Reading and Learning Paths
- C++ Primer by Lippman et al.
- Effective Modern C++ by Scott Meyers
- Online coding platforms like LeetCode and HackerRank.