In C++, classes can be passed to functions either by value or by reference, allowing for efficient management of objects and control over their modifications.
Here’s a code snippet demonstrating passing a class by reference:
#include <iostream>
class MyClass {
public:
void display() {
std::cout << "Hello from MyClass!" << std::endl;
}
};
void passByReference(MyClass& obj) {
obj.display();
}
int main() {
MyClass myObject;
passByReference(myObject); // Passing MyClass object by reference
return 0;
}
Understanding Class Passing in C++
What is Class Passing?
In C++, "class pass" refers to the method used to pass objects of user-defined classes to functions. This concept is crucial because it affects how an object is managed in memory, how its data can be accessed or modified, and the overall performance of the program. Understanding how classes are passed can greatly influence code efficiency and maintainability.
Why Consider the Way You Pass Classes?
When dealing with class passing, several factors come into play:
-
Performance: Depending on how you pass a class, it can either be efficient or introduce considerable overhead. This can significantly impact applications with high-performance requirements.
-
Safety: Safeguarding data integrity is essential. Const correctness ensures that functions do not modify objects that should remain unchanged.
-
Readability: Clear and maintainable code is vital for collaboration and future adjustments. The way you pass classes should enhance the clarity of your intent.
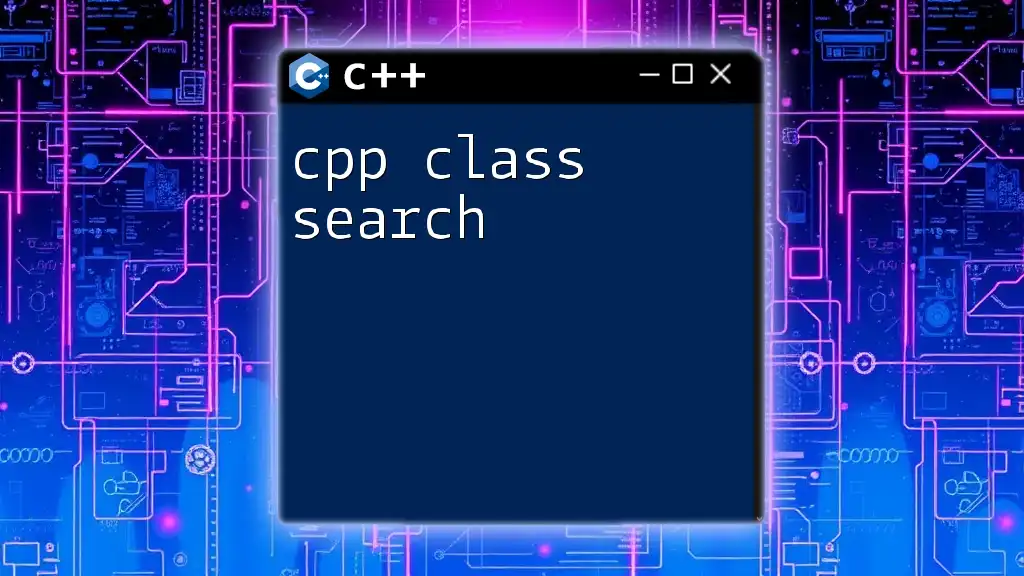
Types of Class Passing in C++
Passing by Value
Passing by value means creating a copy of the object. When you pass an object by value, the function receives a new instance of that object, distinct from the original.
Code Example: Here’s a simple demonstration of passing a class instance by value.
class Example {
public:
int data;
Example(int d) : data(d) {}
};
void displayValue(Example e) {
std::cout << e.data << std::endl;
}
int main() {
Example ex(5);
displayValue(ex);
return 0;
}
In this example, `displayValue` receives a copy of `ex`. Any changes made to `e` do not affect `ex`. While this approach is straightforward, it can be inefficient for large objects due to the overhead of copying.
Passing by Reference
Passing by reference avoids the overhead of copying by allowing the function to operate directly on the original object. When you pass a class by reference, any modifications will apply to the original object.
Code Example: Observe how modifying a passed reference affects the original object.
void modify(Example& e) {
e.data += 10;
}
int main() {
Example ex(5);
modify(ex);
std::cout << ex.data << std::endl; // Outputs 15
return 0;
}
In this case, `modify` takes a reference to `ex`. Changing `e.data` directly modifies the original object, which is beneficial for performance when dealing with larger objects.
Passing by Pointer
Passing by pointer gives you similar control as passing by reference but involves the use of pointers. This method can handle dynamic memory and polymorphic behavior, but it also requires careful memory management.
Code Example: Passing a pointer allows you to manipulate an object via its address.
void manipulate(Example* e) {
e->data *= 2;
}
int main() {
Example ex(10);
manipulate(&ex); // Pass the address of ex
std::cout << ex.data << std::endl; // Outputs 20
return 0;
}
In this example, `manipulate` modifies `ex` through dereferencing the pointer. However, developers must watch out for potential issues, such as using null pointers or encountering memory leaks if dynamic allocation is involved.
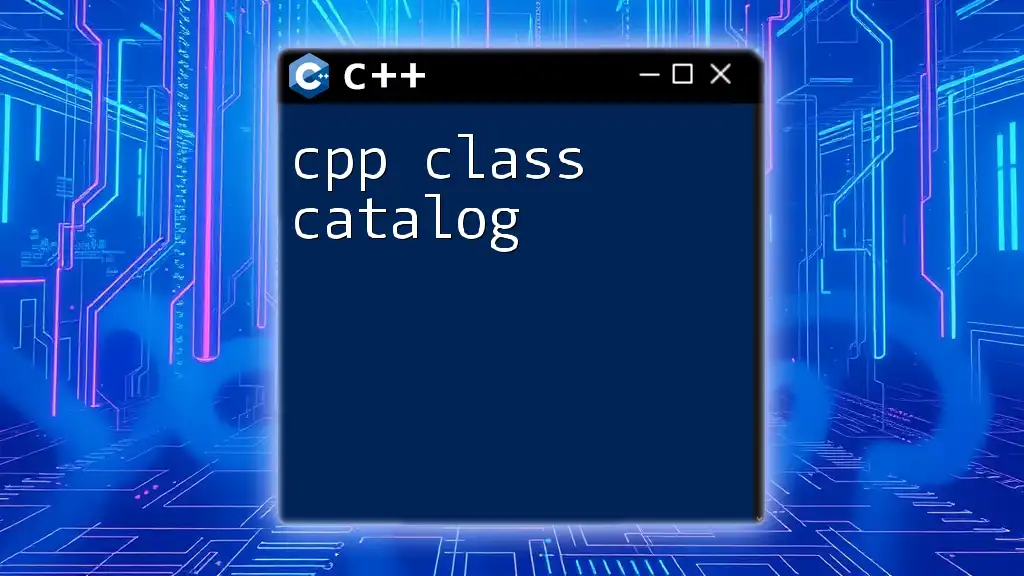
When to Use Each Method
Selecting the proper passing technique requires consideration of several factors:
-
Performance: For small, simple classes, passing by value is acceptable. For large or complex objects, passing by reference is often ideal to avoid unnecessary copies.
-
Readability: Clear code helps reduce errors. For public interfaces, passing by const reference can indicate that the object won’t be altered, enhancing clarity.
-
Safety: When ownership is complex or when working with dynamically allocated memory, pointer passing may be the way to go, but with clear documentation to prevent misuse.
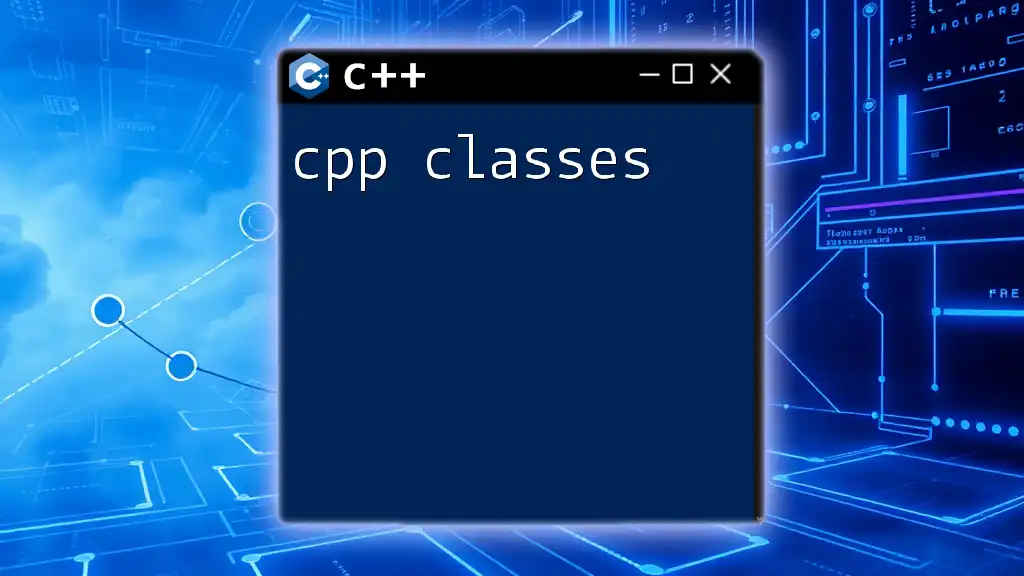
Advanced Concepts in Class Passing
Const References
Using `const` references can prevent unintended modifications while still taking advantage of reference semantics. This is particularly useful for large objects that you don’t want to copy.
Code Example: Here’s a typical use of const references.
void printData(const Example& e) {
std::cout << e.data << std::endl; // Can read but not modify
}
int main() {
Example ex(25);
printData(ex);
return 0;
}
This technique offers both safety and efficiency while allowing read-only access to the object’s state. It's a powerful tool in writing clear and intent-driven APIs.
Move Semantics and Perfect Forwarding
Introduced in C++11, move semantics enhance your ability to efficiently transfer resources. Instead of copying, move semantics allow you to transfer ownership, minimizing resource overhead further.
Code Example: A demonstration of move semantics for efficiency:
#include <utility>
void process(Example&& e) {
std::cout << std::move(e.data) << std::endl;
}
int main() {
process(Example(20)); // Moves temporary object instead of copying
return 0;
}
Here, the function `process` takes a temporary object without copying it, providing a boost in performance. This is especially beneficial when working with large datasets.
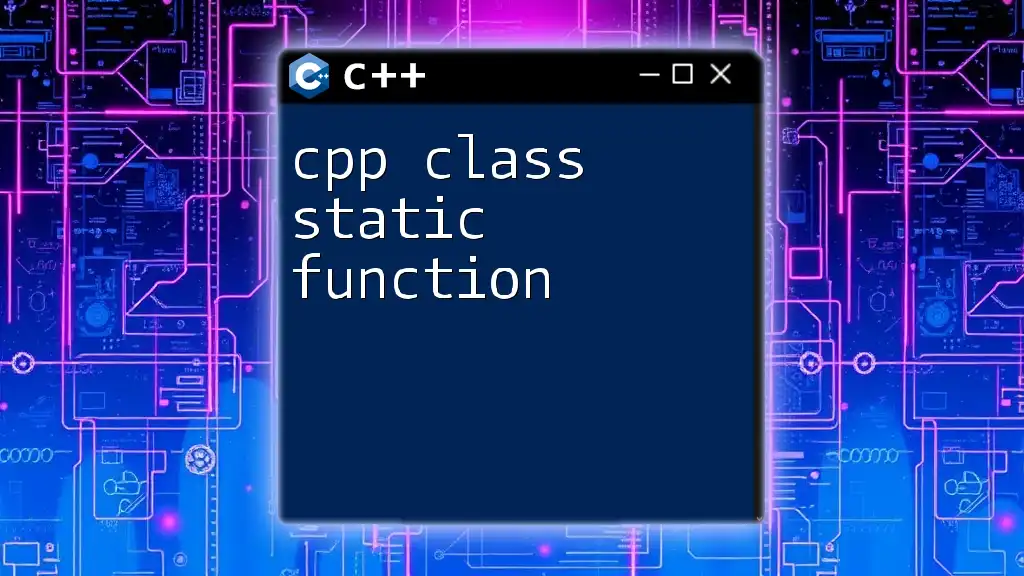
Common Pitfalls in Class Passing
Misunderstanding Ownership
Handling ownership can lead to issues, particularly with pointers. Developers must be vigilant about memory management to avoid dangling pointers or leaks. When using raw pointers, establish clear rules about which part of the code is responsible for managing the object's lifetime.
Passing Temporary Objects
Passing temporary objects can lead to lifetimes where the object no longer exists after the function call. Care must be taken to ensure that the references or pointers do not refer to these temporaries.
Example:
displayValue(Example(10)); // Valid but memory management is key
Here, `Example(10)` is a temporary object passed by value. Once `displayValue` exits, the temporary object is destructed. Always keep object lifetimes in mind to prevent accessing invalid memory.
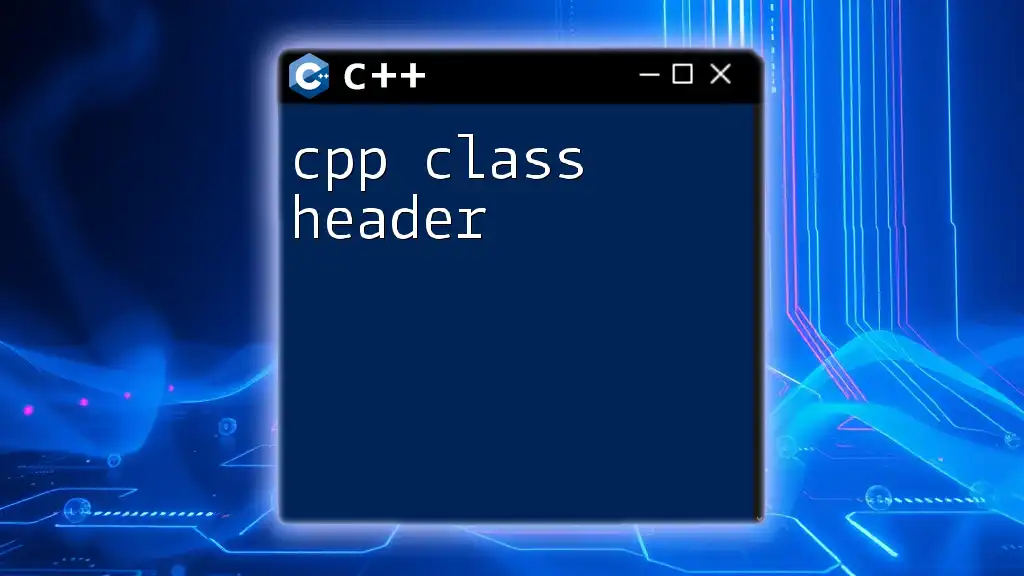
Conclusion
In this guide, we explored the different ways to handle cpp class pass, detailing how to optimize your code for efficiency and clarity through the various techniques of passing classes. Understanding the nuances of these methods can significantly impact performance, safety, and code readability. By applying these principles, you can write more efficient, safe, and maintainable C++ code.
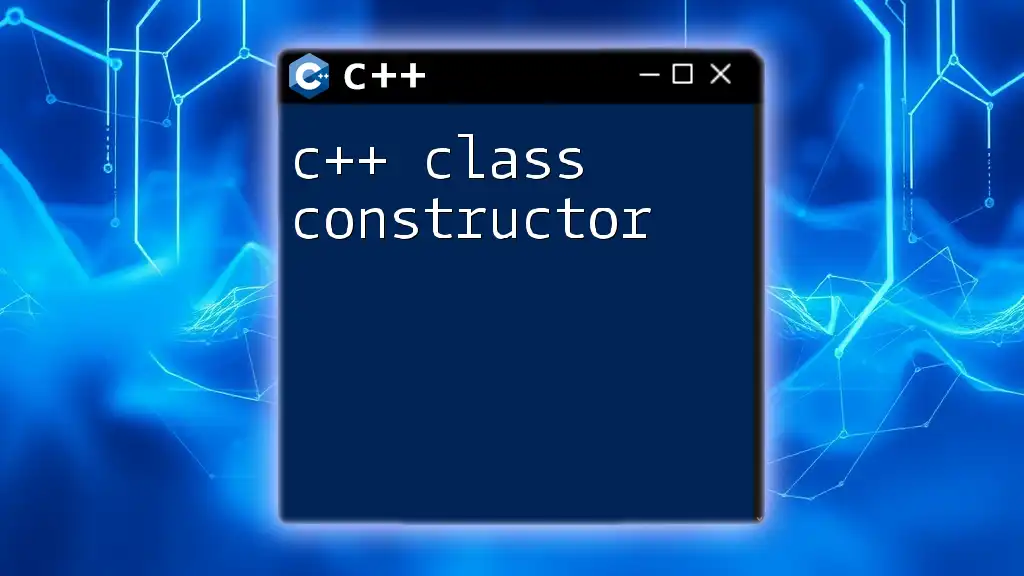
Additional Resources
To further enhance your understanding of this topic, consider these resources:
- Recommended books on C++ design patterns and best practices
- Online tutorials focusing on C++ memory management
- Forums for discussions with other developers about complex class passing scenarios
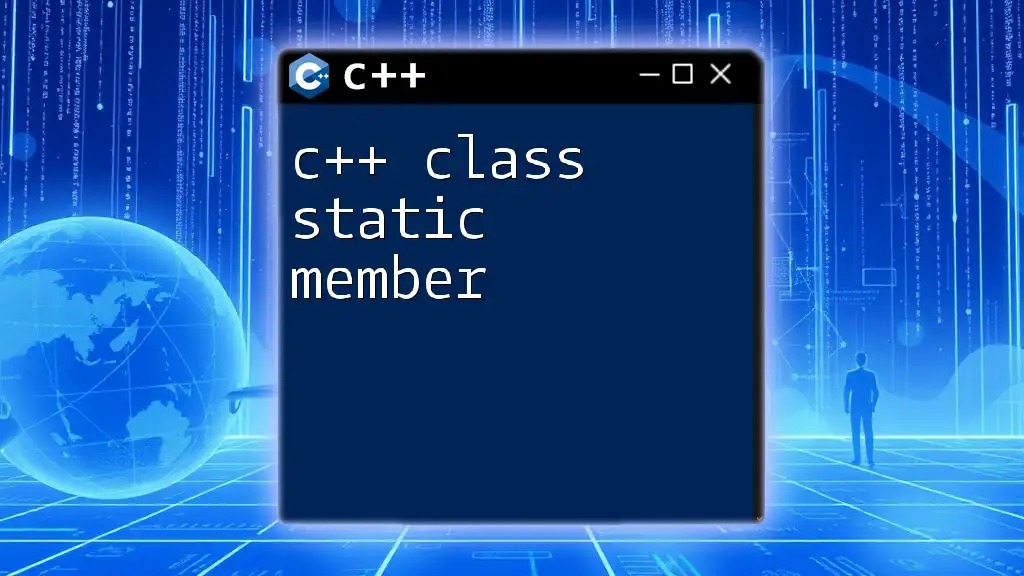
FAQs
What is the difference between passing by reference and passing by pointer?
While both methods can manipulate the original object without copying, passing by reference is generally easier to use and understand. Pointers can introduce complexities such as dereferencing and memory management.
Can I pass a const object by value?
Yes, you can pass a const object by value. This will create a copy of the object that cannot be modified during the function call. However, doing so does not utilize the benefits of const correctness in terms of preventing alterations to the original instance.