C++ apps are software applications developed using the C++ programming language, known for its efficiency and performance in system-level programming and application development.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What Are C++ Apps?
C++ applications, or cpp apps, are software programs developed using the C++ programming language, known for its efficiency, performance, and versatility. C++ is commonly utilized across various domains, leading to the development of software ranging from simple utilities to complex system applications.
Types of C++ Applications
Desktop Applications
Desktop applications are programs that run on personal computers or laptops. These applications often have graphical user interfaces (GUIs) and can perform a variety of tasks, enabling users to work efficiently. Examples include integrated development environments (IDEs) like Visual Studio, media players, and office productivity tools.
System Software
C++ plays a crucial role in developing system software, which includes operating systems, device drivers, and utility software. C++ delivers the performance and control necessary for low-level programming, making it a top choice for system-level applications.
Game Development
C++ is a cornerstone in the gaming industry, utilized in the creation of both games and game engines. The language offers direct access to hardware and high-performance capabilities, essential for producing graphically-rich and responsive gaming experiences. Popular game engines, like Unreal Engine, rely heavily on C++ for game logic and performance optimization.
Embedded Systems
Embedded systems are specialized computing systems designed for specific tasks within larger systems. C++ is often used in programming microcontrollers and IoT devices, where performance and efficient resource management are paramount. Its object-oriented capabilities help in creating scalable and maintainable code for such complex systems.
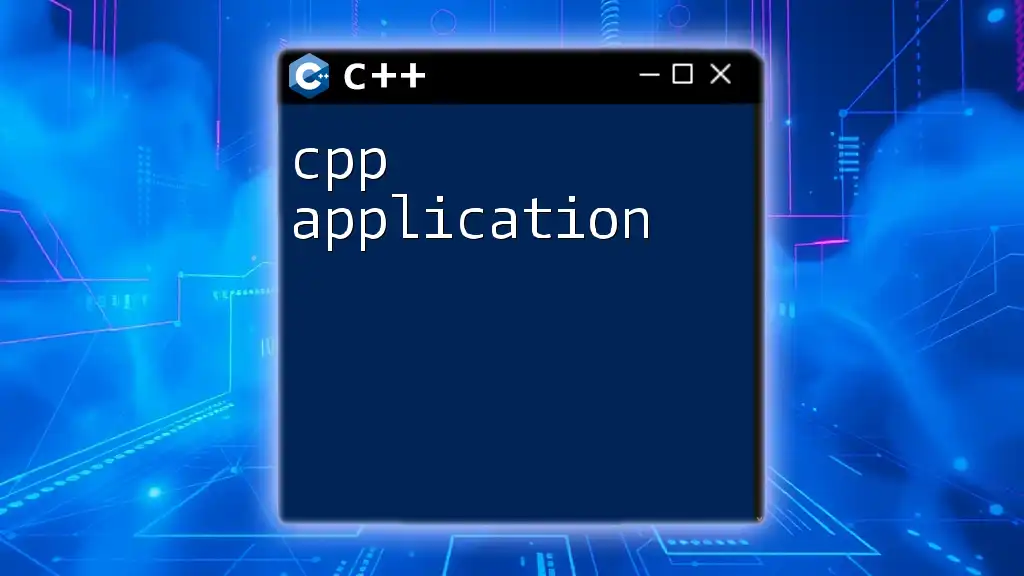
Setting Up Your C++ Development Environment
Choosing the Right IDE
Selecting the Integrated Development Environment (IDE) is critical for easing C++ application development. Popular options include:
- Visual Studio: Offers extensive debugging and profiling tools.
- Code::Blocks: A user-friendly, open-source IDE suitable for beginners.
- CLion: A powerful IDE focused on modern C++ standards.
Compiler Options
The choice of a compiler can significantly impact your C++ application. Notable compilers include:
- GNU Compiler Collection (GCC): Open-source, widely used in Linux environments.
- Clang: Provides excellent diagnostic capabilities and is often used for macOS development.
- Microsoft Visual C++ (MSVC): The premier compiler for Windows development, integrated within Visual Studio.
Essential Tools and Libraries
Utilizing libraries can tremendously simplify development. Two notable libraries are:
- Standard Template Library (STL): Offers a wealth of pre-defined data structures and algorithms.
- Boost: A comprehensive set of libraries that enhance C++ functionality and facilitate robust application development.
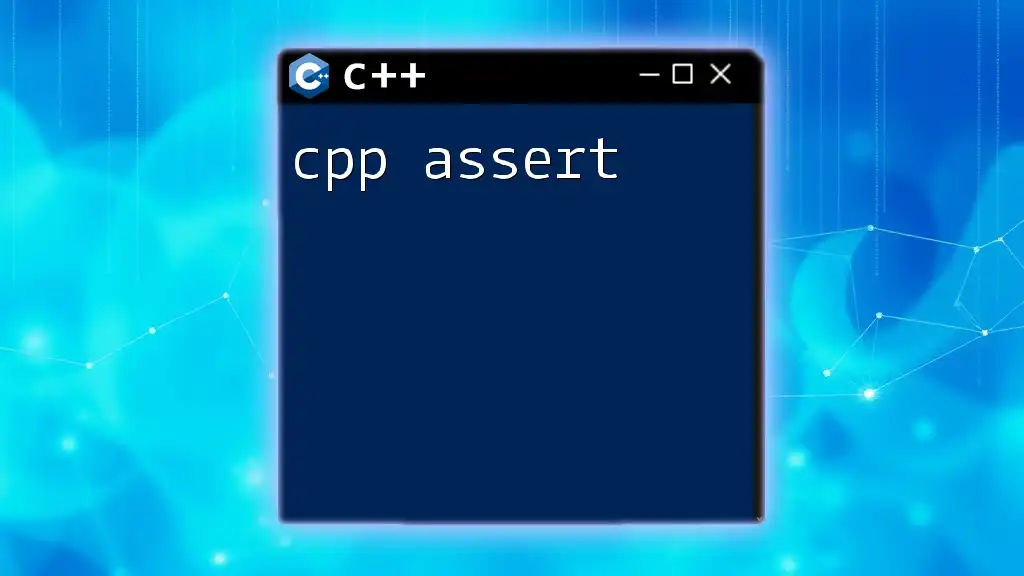
Key Features of C++ for Application Development
Object-Oriented Programming
C++ is synonymous with object-oriented programming (OOP), which allows developers to create modular and reusable code. Key principles include:
- Encapsulation: Bundling data and methods into classes allows for greater control over access.
- Inheritance: Facilitates code reusability by allowing a class to inherit attributes and methods from another class.
- Polymorphism: Enables functions to operate in different ways based on object type at runtime.
Here is a simple example demonstrating polymorphism:
class Base {
public:
virtual void show() { std::cout << "Base class show function called."; }
};
class Derived : public Base {
public:
void show() override { std::cout << "Derived class show function called."; }
};
Memory Management
C++ gives developers explicit control over memory management, a critical feature for performance-intensive applications. Dynamic memory allocation through pointers allows for efficient usage of resources, but it requires careful management to prevent leaks.
Here’s a fundamental example of dynamic memory allocation:
int *ptr = new int; // dynamically allocate memory
*ptr = 10; // assign value
delete ptr; // free allocated memory
Performance and Efficiency
C++ enables developers to write high-performance applications due to its ability to interact closely with system hardware. The language's efficiency makes it a preferred choice for resource-constrained environments like embedded systems.
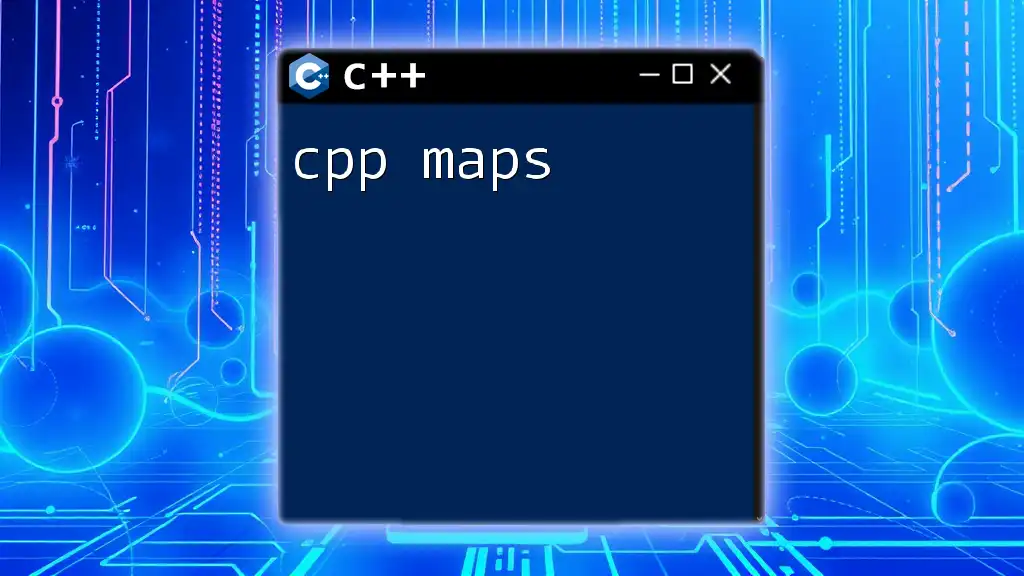
Writing Your First C++ Application
Basic Structure of a C++ Program
To illustrate how simple it is to develop cpp apps, here’s a basic "Hello World" program. This straightforward application demonstrates the structure of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Compiling and Running the Application
To compile and run the application, follow these steps using your preferred environment:
- Open the terminal or command prompt.
- Navigate to the directory where your C++ file is saved.
- Compile the program (assuming you named it `hello.cpp`):
g++ hello.cpp -o hello
- Run the compiled application:
./hello
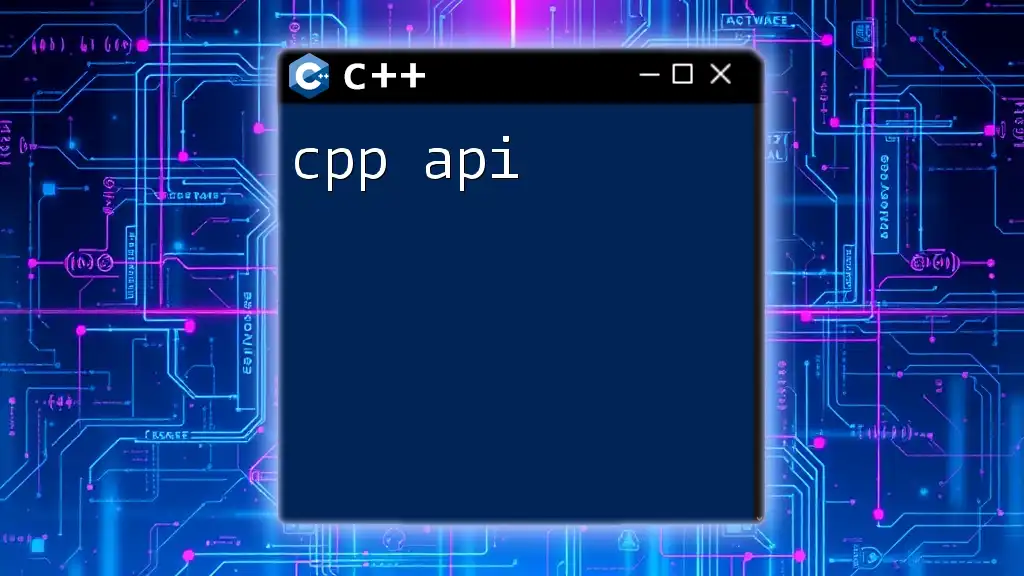
Exploring Advanced C++ Concepts for Apps
Templates and Generic Programming
C++ templates allow developers to create functions and classes that work with any data type. This leads to code that is both reusable and type-safe. Here’s a simple template function for addition:
template <typename T>
T add(T a, T b) {
return a + b;
}
Exception Handling
Robust applications must gracefully handle errors. C++ supports exception handling to deal with potential runtime errors. Here’s an example:
try {
// Code that may throw an exception
throw std::runtime_error("An error occurred.");
} catch (const std::exception &e) {
std::cerr << e.what() << '\n';
}
Concurrency and Multithreading
C++11 introduced threads and concurrency support, allowing developers to write applications that can perform multiple tasks simultaneously. This is vital for modern applications which require responsiveness and performance.
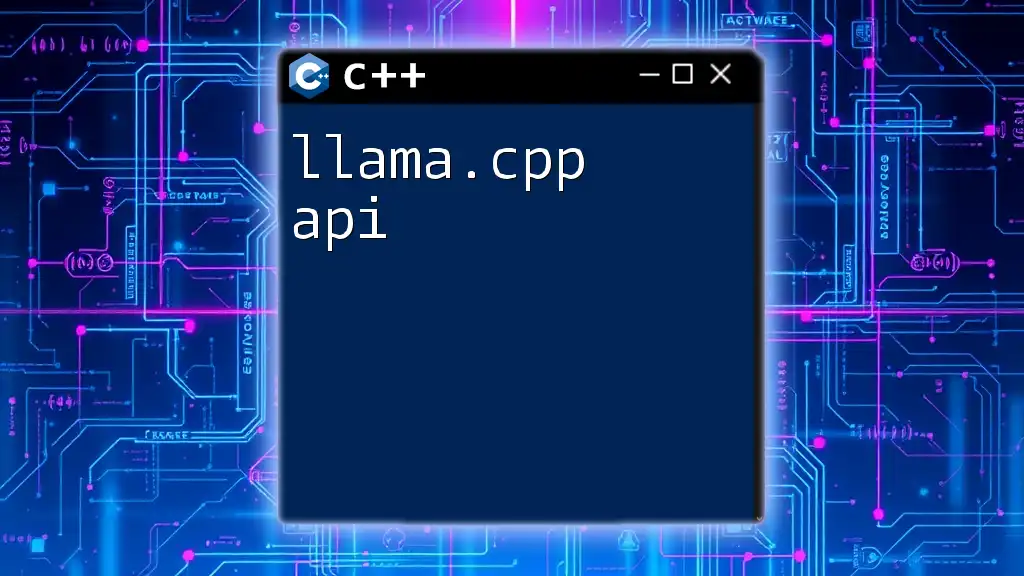
Popular C++ Libraries for Application Development
Introduction to C++ Libraries
Using robust libraries can accelerate the development process, reducing boilerplate code and improving maintainability.
Boost Library
Boost is a collection of peer-reviewed C++ libraries that extend C++’s capabilities, offering functionalities for linear algebra, multi-threading, and regular expressions, among others.
Qt Framework
Qt is a powerful framework ideal for creating GUI applications. It simplifies the development process through its signal-slot mechanism for event-driven programming, thereby enhancing user interaction.
SFML
The Simple and Fast Multimedia Library (SFML) is suited for game development and multimedia applications, providing a simple interface for handling graphics, audio, and input.
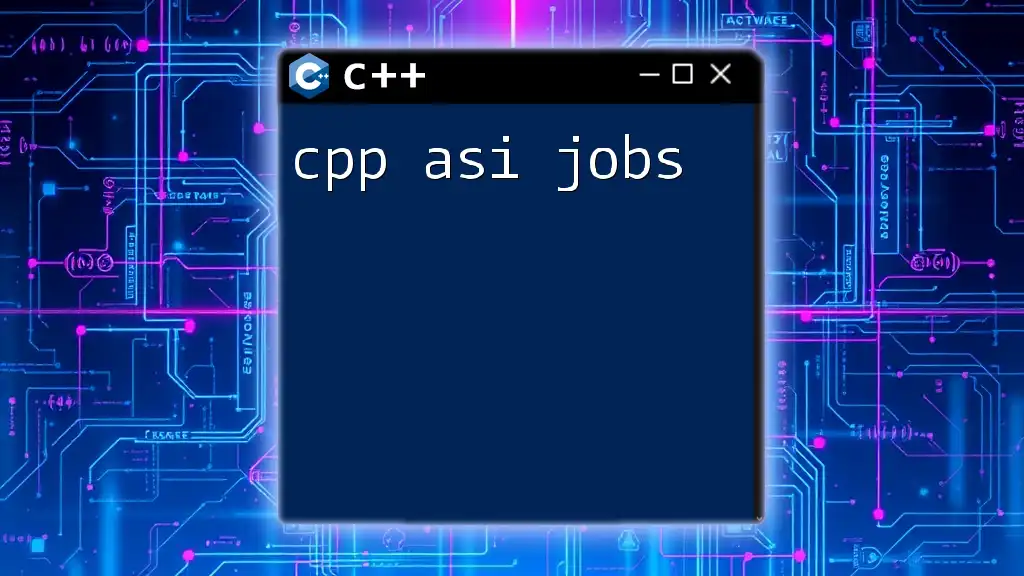
Real-World Applications of C++
Case Studies
Many renowned applications have been developed using C++. For instance, Adobe Photoshop utilizes C++ for its performance capabilities, while Microsoft Office is built on C++ foundations for its text processing and spreadsheet functionalities.
Emerging Trends
C++ continues to play a vital role in emerging technologies, particularly in artificial intelligence and machine learning, where speed and efficiency are critical. Moreover, C++ is making inroads into virtual and augmented reality applications, leveraging its performance advantages to create immersive experiences.
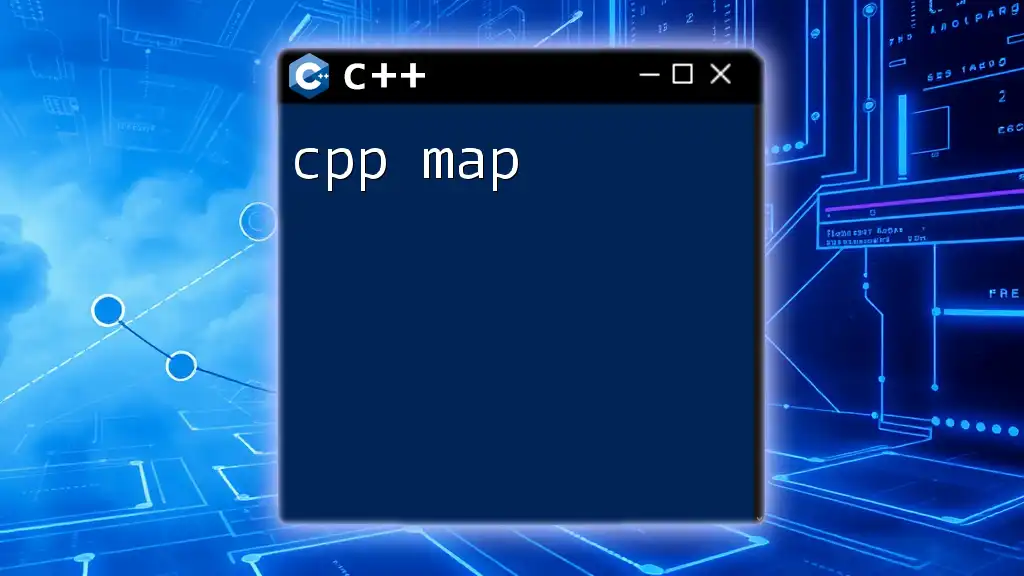
Best Practices for C++ Application Development
Code Optimization Techniques
Optimizing your application is crucial for performance. Employ profiling tools to identify bottlenecks and refactor your code accordingly, focusing on algorithm efficiency and memory management.
Following C++ Coding Standards
Adhering to established coding standards promotes code quality. Consider using standards such as C++ Core Guidelines to ensure your code is readable and maintainable.
Version Control and Collaboration
Using version control systems like Git is essential in collaborative development. It allows teams to work concurrently, manage changes effectively, and roll back if necessary.
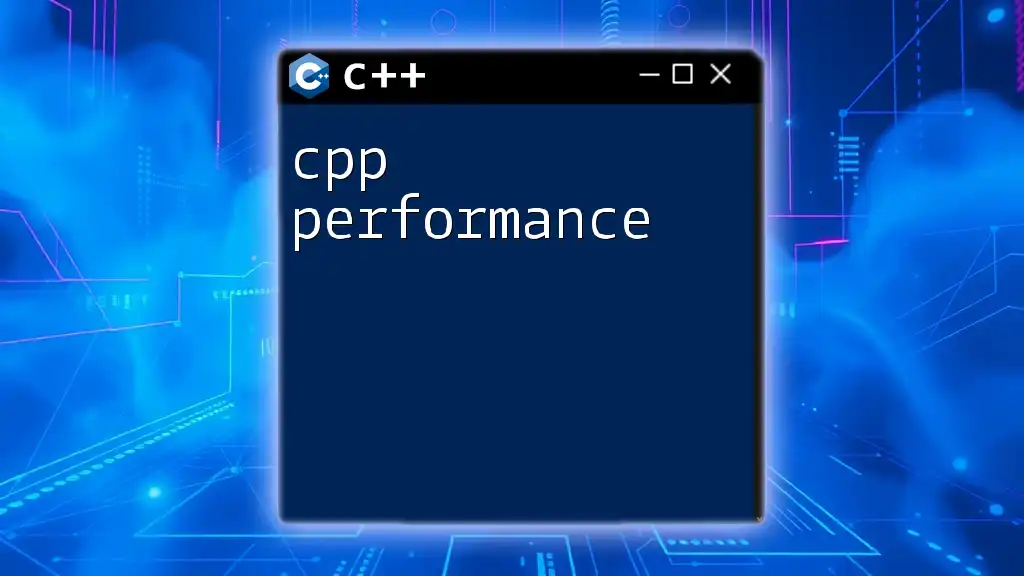
Conclusion
Developing cpp apps offers vast opportunities across industries, from embedded systems to high-performance applications. C++ combines power and flexibility, making it an indispensable tool for a developer’s toolkit. With its rich features and a vibrant community, diving deeper into C++ application development can lead to exciting innovations and career advancements.