A C++ application is a software program written in the C++ programming language that can perform tasks, process data, and interact with users or other systems.
Here's a simple code snippet that demonstrates basic input and output in a C++ application:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++
C++ is a powerful programming language that has been a cornerstone in the software development landscape since its creation in the early 1980s. It combines both low-level and high-level features, making it suitable for a broad range of applications, from system-level programming to game development. The language supports object-oriented programming, which promotes more manageable and reusable code.
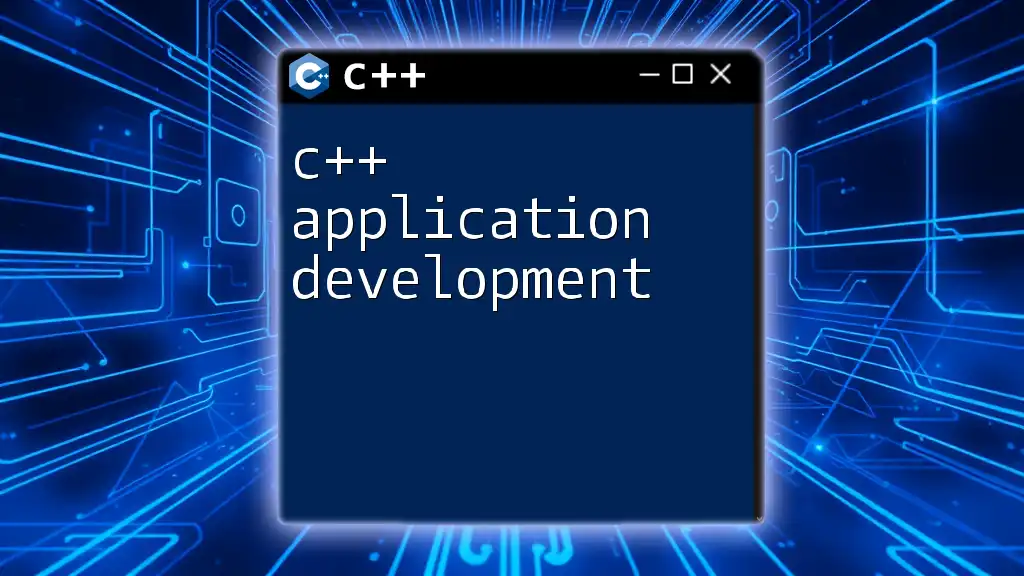
What is a CPP Application?
A documentation or software tool developed using C++ is referred to as a CPP application. These applications can take various forms, including desktop applications, server-side solutions, and embedded systems. An essential characteristic of a CPP application is its performance; C++ allows developers to write efficient code that can optimize for speed and resource usage.
Real-world Applications
- Games: Neural networks and game engines, like Unreal Engine, leverage C++ for its speed and flexibility.
- Operating Systems: Parts of operating systems (like Windows and Linux) are developed in C++ due to its low-level capabilities.
- Embedded Systems: Applications in embedded systems (microwave ovens, cars) extensively use C++ for their efficiency.
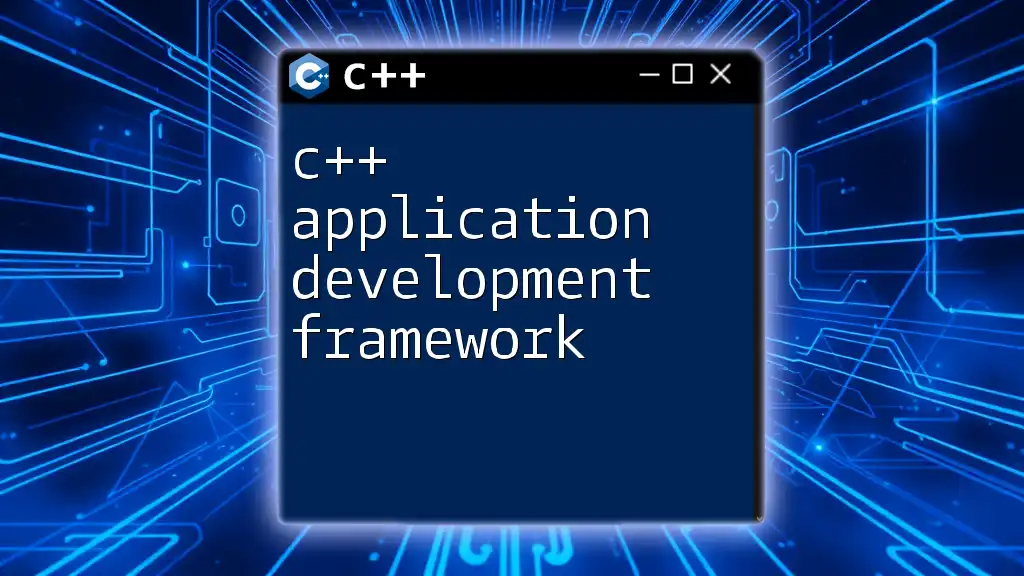
Key Components of CPP Applications
Syntax and Structure
Before diving into writing a CPP application, understanding its syntax is crucial. C++ has a specific set of rules that dictate how code is written.
Basic Syntax Overview
C++ employs various keywords, data types, and control structures, which are essential to building functional applications.
- Variables: Used to store data.
- Control Structures: Such as loops (`for`, `while`) and conditionals (`if`, `switch`), control the flow of the code.
Writing a Simple CPP Application
Setting Up the Development Environment
Before writing a CPP application, setting up an appropriate development environment is imperative. You can choose from several Integrated Development Environments (IDEs) like Visual Studio, Code::Blocks, or CLion. Additionally, make sure to install a compiler, such as GCC or Clang, to convert your C++ code into executable applications.
Hello World Example
The quintessential starting point for any programmer is the classic "Hello, World!" application. Below is an example in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this simple application, we include the `iostream` library, which provides functionalities for input-output operations. The `main()` function is where the program execution starts. The `std::cout` statement prints "Hello, World!" to the console, followed by a newline established by `std::endl`. Understanding this basic structure is vital, as it lays the foundation for more complex applications.
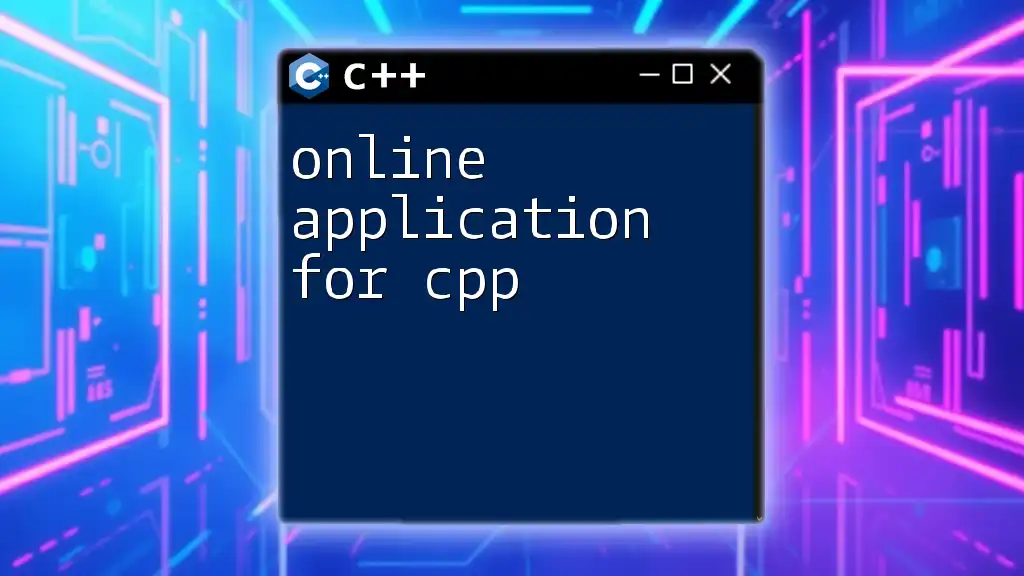
Developing More Complex CPP Applications
Object-Oriented Programming (OOP) in C++
C++ stands out for its support of object-oriented programming (OOP), a paradigm that organizes software design around data, or objects rather than functions and logic.
Understanding Classes and Objects
In C++, a class serves as a blueprint for creating objects. Key OOP concepts include:
- Encapsulation: Bundling data with methods that operate on the data.
- Inheritance: Creating new classes from existing ones to promote code reusability.
- Polymorphism: Allowing methods to do different things based on the object it is acting upon.
Creating a Simple Class
Here’s an example that showcases these principles in action:
class Animal {
public:
void speak() {
std::cout << "Animal speaks" << std::endl;
}
};
int main() {
Animal myAnimal;
myAnimal.speak();
return 0;
}
In this snippet, we define a class `Animal` with a method `speak()`. The `main()` function creates an `Animal` object and calls the `speak()` method.
File Handling in C++
File handling is a crucial part of most applications, allowing them to read from and write to files.
Reading from Files
Reading data from files allows your CPP application to process external data. Here’s how you can read from a text file:
#include <fstream>
#include <iostream>
int main() {
std::ifstream inputFile("example.txt");
std::string line;
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
inputFile.close();
return 0;
}
In this code, we utilize the `ifstream` class to open and read from a file named `example.txt`. The `std::getline` function reads the file line by line, outputting each line to the console.
Writing to Files
Equally important is the ability to write to files. Here’s how to do this:
#include <fstream>
#include <iostream>
int main() {
std::ofstream outputFile("output.txt");
outputFile << "Hello, World!" << std::endl;
outputFile.close();
return 0;
}
In this example, `ofstream` is used to create and write to a file named `output.txt`. This simple operation highlights how your CPP application can output data to external files effectively.
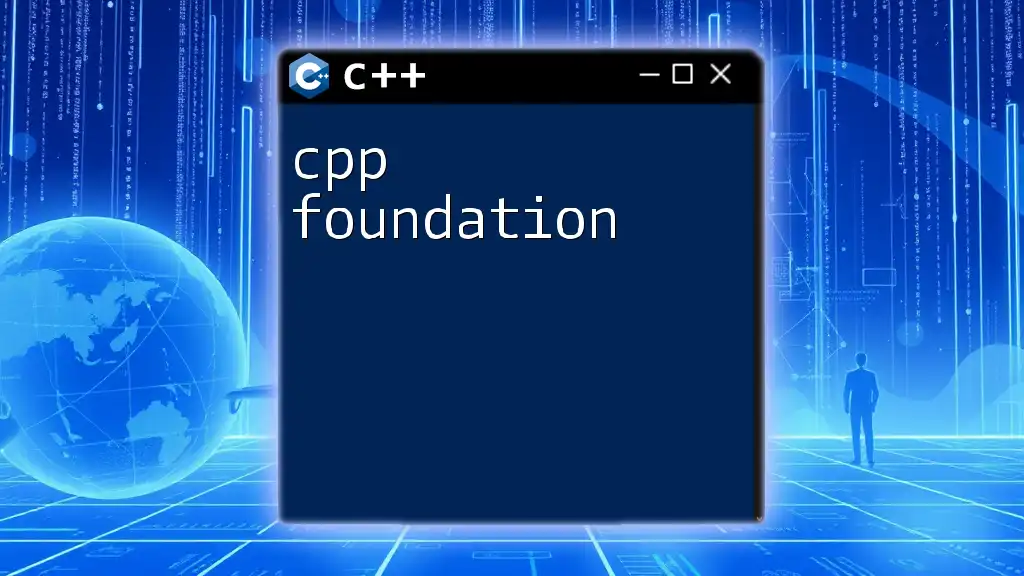
Libraries and Frameworks in CPP
Standard Template Library (STL)
The Standard Template Library (STL) is an essential part of C++, containing a set of C++ template classes to provide general-purpose classes and functions. It includes several useful data structures and algorithms.
- Common Data Structures: Such as vectors, lists, and maps that help with organizing and managing data efficiently.
- Example of Using STL Containers: Here’s how to use a `vector` from STL:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << std::endl;
}
return 0;
}
Using External Libraries
C++ has an extensive ecosystem of libraries that can expand functionality beyond the Standard Library. An example is the Boost library, which offers numerous libraries for various functions such as multi-threading and file handling.
- Installing Boost: You typically can find installation instructions on their official website, which generally involves downloading the library or using a package manager like `vcpkg`.
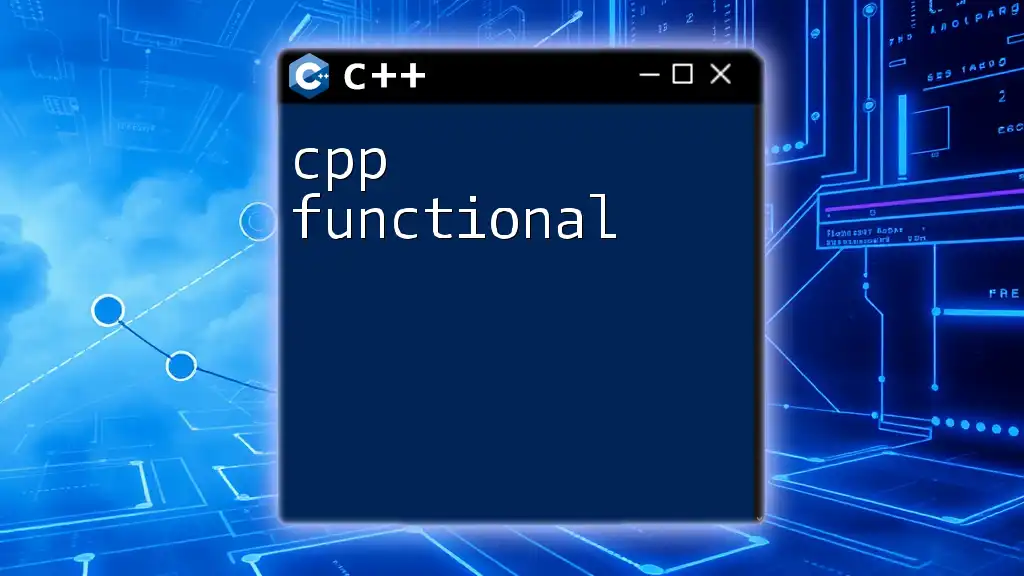
Debugging and Testing CPP Applications
Best Practices for Debugging
Debugging is an indispensable skill for any developer. Common errors include syntax errors, logical errors, and runtime errors:
- Syntax Errors: Often caught during compilation, these occur when the code does not conform to the C++ language rules.
- Logical Errors: More subtle, these arise when the code runs without crashing but produces the wrong output.
Using Debugging Tools
Employing debugging tools can help identify issues quickly. gdb (GNU Debugger) is a popular command-line tool for debugging C++ applications. IDEs often come with built-in debugging capabilities, making it easier to set breakpoints and inspect variable states.
Writing Unit Tests
Testing is an essential aspect of software development. Writing unit tests can help ensure that each part of your application works as intended.
What are Unit Tests?
These tests validate individual components of the software, confirming that each one behaves as expected.
Creating a Simple Unit Test Example
Here's a basic unit test example that checks arithmetic correctness:
#include <cassert>
void testFunction() {
assert(1 + 1 == 2);
}
int main() {
testFunction();
return 0;
}
In this code, we use `assert()` to verify that the expression evaluates to true. This is a simple demonstration of how unit tests can be integrated into your CPP application.
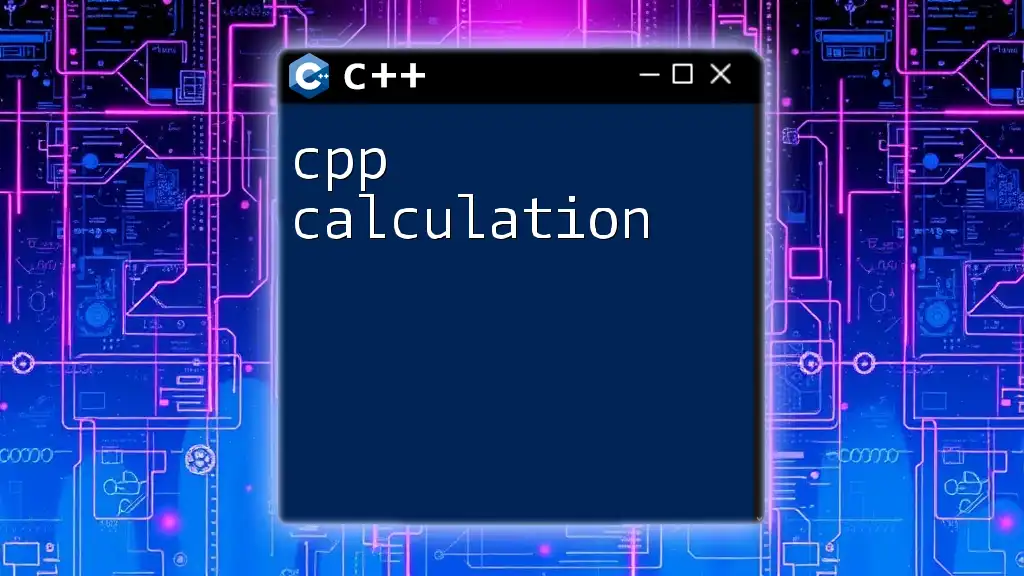
Performance Optimization in CPP Applications
Analyzing Performance
Performance is key for CPP applications, especially in a world where efficiency matters. Profiling tools can help analyze your application's performance, letting you identify bottlenecks.
Techniques to Optimize Code
Here are some common techniques to enhance the performance of C++ applications:
Memory Management Best Practices
Using smart pointers (`std::unique_ptr`, `std::shared_ptr`) instead of raw pointers can help manage memory automatically and prevent leaks, enhancing application stability.
Efficient Algorithms
Understanding and applying efficient algorithms is crucial for high-performance applications. Basic searching and sorting algorithms can profoundly affect the application responsiveness when managing large datasets.
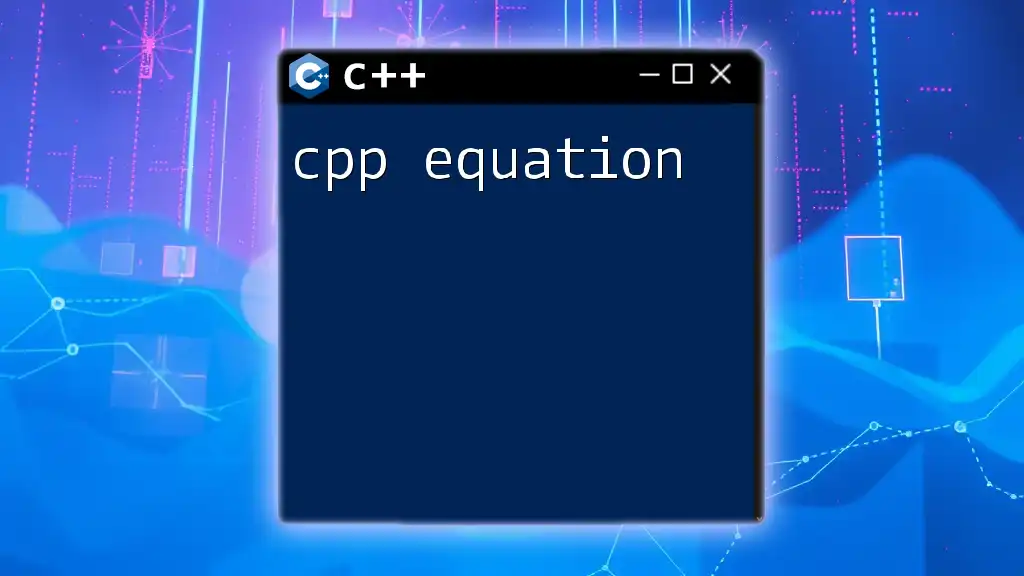
Conclusion
In wrapping up, we've delved into the realm of CPP applications, covering essential syntax, complex concepts like OOP, file handling methods, the use of libraries, debugging, unit testing, and performance optimization. Understanding these aspects empowers developers to create efficient, robust applications that leverage the full potential of C++.
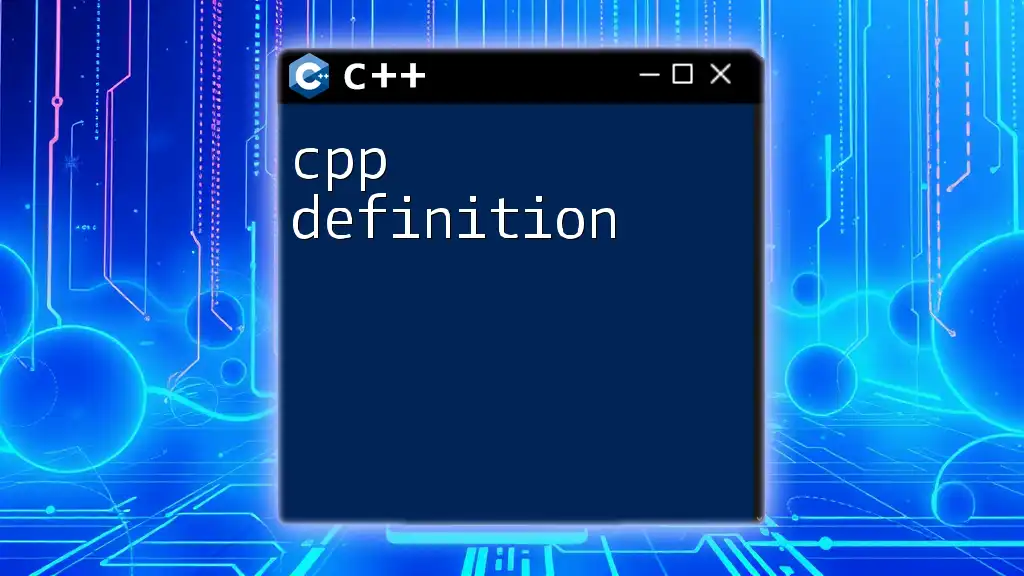
Call to Action
If you're interested in diving deeper into C++ programming, join our community of learners! Stay updated with our resources, tutorials, and insights about CPP applications, and let's grow together in this exciting journey of coding! Subscribe for tips and more information to enhance your learning experience in C++.