A C++ allocator is a class responsible for abstracting memory allocation and deallocation for containers, allowing customized memory management strategies.
Here’s a simple example using the standard allocator:
#include <iostream>
#include <memory>
#include <vector>
int main() {
std::allocator<int> alloc; // Create an allocator for int
int* p = alloc.allocate(5); // Allocate memory for 5 integers
for (int i = 0; i < 5; ++i) {
alloc.construct(&p[i], i * 10); // Construct integers in allocated memory
}
for (int i = 0; i < 5; ++i) {
std::cout << p[i] << " "; // Output the integers
}
for (int i = 0; i < 5; ++i) {
alloc.destroy(&p[i]); // Destroy the objects
}
alloc.deallocate(p, 5); // Deallocate the memory
return 0;
}
Introduction to Allocators
What is an Allocator?
An allocator in C++ is a class responsible for abstracting memory allocation and deallocation for various objects. In essence, it provides a mechanism for the allocation and construction of objects, as well as the deallocation and destruction of those objects. Allocators are integral to the Standard Template Library (STL), allowing for the seamless management of memory for containers and other data structures.
Why Use Allocators?
Using an allocator provides several benefits:
- Custom Memory Management: By creating custom allocators, developers can tailor memory allocation strategies that are better suited for specific applications, potentially improving performance.
- Performance Enhancements: Different types of allocators can reduce overhead, limit fragmentation, and enhance the speed of memory operations, which is often crucial in performance-intensive applications.
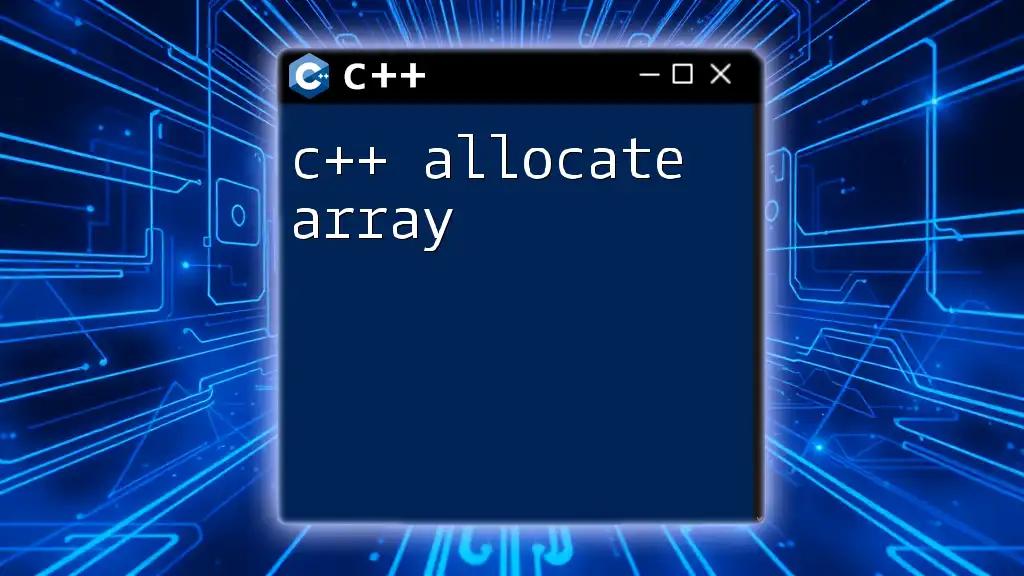
Understanding Memory Management in C++
Basics of Memory Allocation
Memory in C++ can be divided mainly into two categories: stack and heap memory.
- Stack Memory: Automatically managed by the compiler, stack memory is used for local variables and cannot be resized once allocated.
- Heap Memory: Dynamically allocated and managed by the programmer, heap memory allows for more flexible memory management but requires careful allocation and deallocation to prevent memory leaks.
C++ offers several standard approaches to memory allocation, such as using `new` and `delete` operators. Understanding how these work is essential for grasping the role of allocators.
Standard Allocator in C++
The STL provides a default memory management mechanism through `std::allocator`. This template class enables the allocation of memory for any data type and serves as the default allocator for STL containers.
When using `std::allocator`, you can allocate, construct, destroy, and deallocate memory for objects with minimal hassle.
Code Snippet: Basic Usage of `std::allocator`
#include <iostream>
#include <memory>
int main() {
std::allocator<int> alloc; // Create an allocator for int
int* p = alloc.allocate(5); // Allocate space for 5 integers
for (size_t i = 0; i < 5; ++i) {
alloc.construct(p + i, i + 1); // Construct the integers
}
for (size_t i = 0; i < 5; ++i) {
std::cout << p[i] << " "; // Output the integers
}
for (size_t i = 0; i < 5; ++i) {
alloc.destroy(p + i); // Destroy the integers
}
alloc.deallocate(p, 5); // Deallocate the memory
return 0;
}
In this example, we first create an `std::allocator` for integers. Then, we allocate and deallocate space for five integers while constructing and destroying them in the process. This leads to efficient memory management, ensuring that there are no leaks.
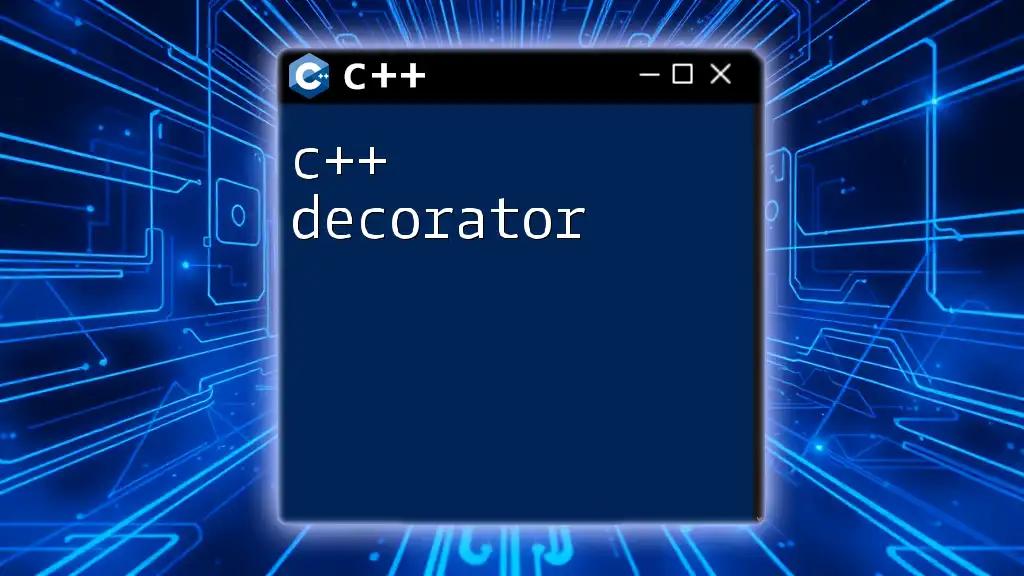
Custom Allocators
When to Create a Custom Allocator?
Creating a custom allocator can be advantageous in situations where the default allocation strategies do not suffice. For instance, if:
- Your application frequently allocates and deallocates small amounts of memory.
- You need to manage memory allocation across multiple threads.
- You want to implement a specific allocation pattern (like pooled memory).
Defining a Custom Allocator
A custom allocator must implement several key methods that define how memory is allocated, deallocated, constructed, and destroyed. Here, we describe the essential components.
Example: Implementing a Simple Custom Allocator
template <typename T>
class SimpleAllocator {
public:
T* allocate(std::size_t n) {
return static_cast<T*>(::operator new(n * sizeof(T)));
}
void deallocate(T* p, std::size_t n) {
::operator delete(p);
}
template <typename U, typename... Args>
void construct(U* p, Args&&... args) {
new (p) U(std::forward<Args>(args)...);
}
template <typename U>
void destroy(U* p) {
p->~U();
}
};
In the above implementation, our `SimpleAllocator` provides four critical functionalities:
- allocate(): Allocates raw storage for `n` objects of type `T`.
- deallocate(): Deallocates memory when it's no longer needed.
- construct(): Constructs an object in allocated storage through placement new.
- destroy(): Calls the destructor for the object, cleaning up before deallocation.
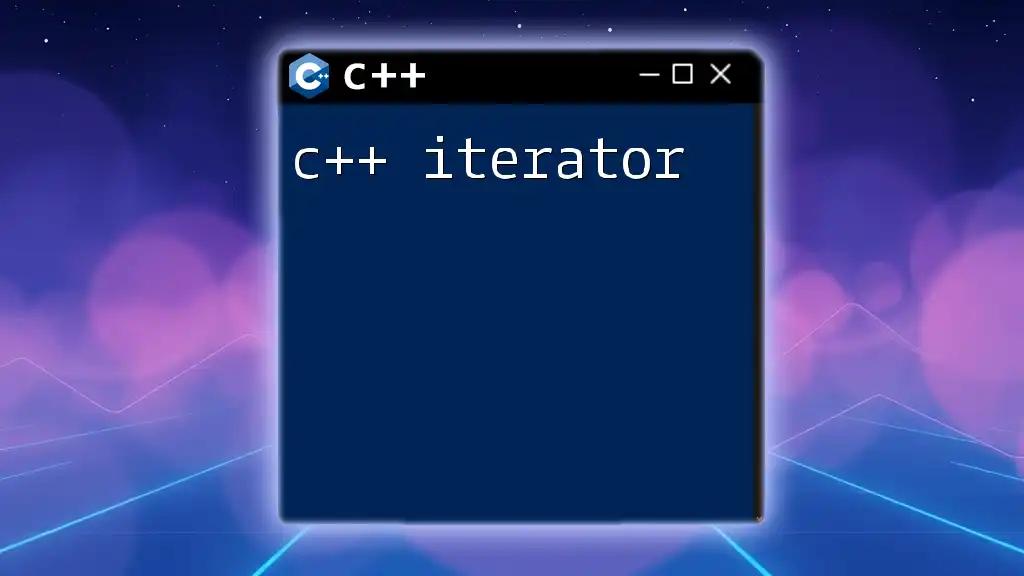
Using Custom Allocators with STL Containers
Custom allocators can easily be utilized with STL containers. This integration allows for specialized memory management strategies tailored for specific needs.
Code Snippet: Using `SimpleAllocator` with `std::vector`
#include <vector>
int main() {
std::vector<int, SimpleAllocator<int>> vec; // Use custom allocator.
vec.push_back(1);
vec.push_back(2);
vec.push_back(3);
for (const auto& val : vec) {
std::cout << val << " ";
}
return 0;
}
In this example, we utilize `SimpleAllocator` within a `std::vector`. The vector automatically uses our custom allocation strategy, allowing us to take advantage of the custom logic we defined.
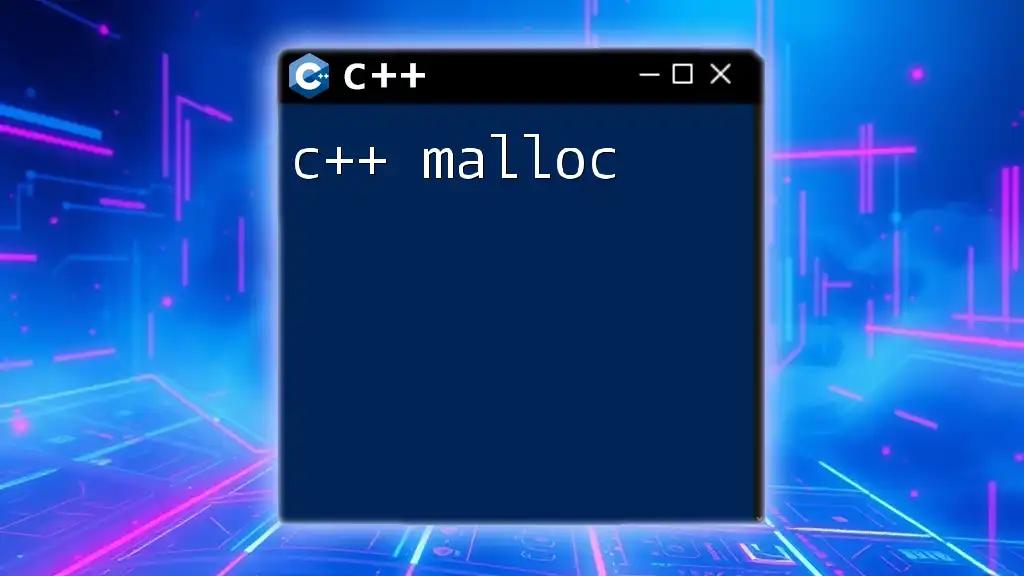
Best Practices for Allocators
Performance Considerations
When designing an allocator, performance is paramount. Some critical practices include:
- Minimize Overhead: Ensure that the allocator's functionality doesn’t significantly impact performance.
- Manage Fragmentation: Allocate sizes appropriately to minimize fragmentation, thereby optimizing memory usage.
- Thread Safety: If the allocator will be used in a multi-threaded environment, ensure that it properly manages potential race conditions.
Debugging and Testing Allocators
Debugging a custom allocator requires careful attention to memory operations:
- Use tools like Valgrind or AddressSanitizer to monitor memory usage.
- Implement assertions in your allocator code to validate memory states and catch errors early.
- Consider writing unit tests to verify that your allocator behaves as expected across various scenarios.
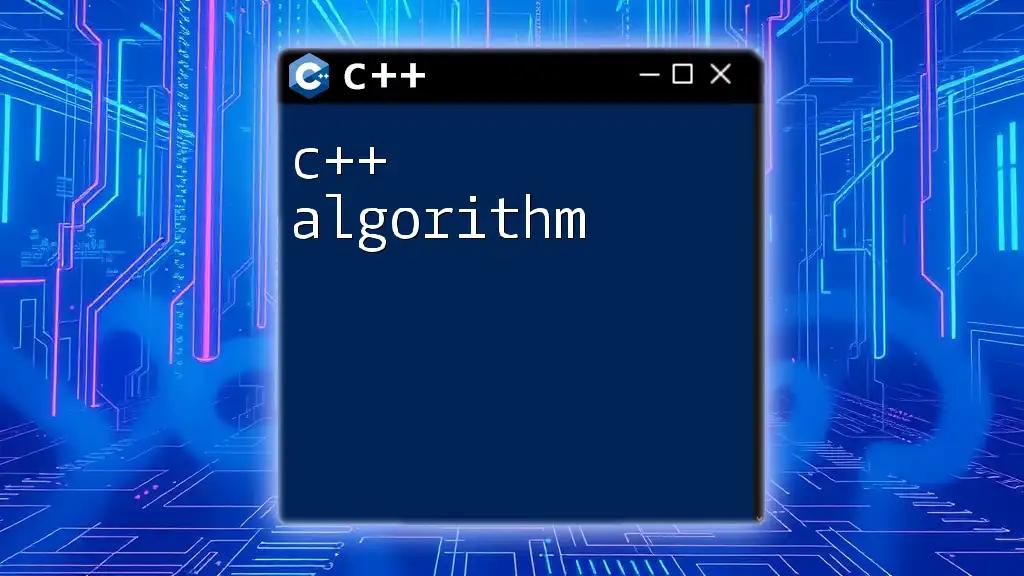
Advanced Allocator Concepts
Pool Allocators
Pool allocators are specialized allocators that manage memory in fixed-size chunks, minimizing the overhead typically associated with frequent allocation and deallocation. These are particularly useful for applications where the size and number of allocated objects are well defined.
Implementing a pool allocator requires an understanding of how to optimize memory requests rather than dealing directly with raw allocation/deallocation for each object.
Memory Resource Management
C++17 introduced a more sophisticated way to handle allocators with the introduction of `std::pmr` (Polymorphic Memory Resources).
In `std::pmr`, memory resources can be swapped or shared easily. This creates greater flexibility when managing memory without manually defining every memory operation.

Conclusion
A strong grasp of allocators not only enhances your understanding of C++ memory management but also equips you with the tools to improve application performance significantly. The ability to create custom allocators enables developers to tailor memory management to the specific needs of their applications. Experimenting with allocators fosters deeper insights into memory management patterns, encouraging best practices that can lead to more efficient and robust code.
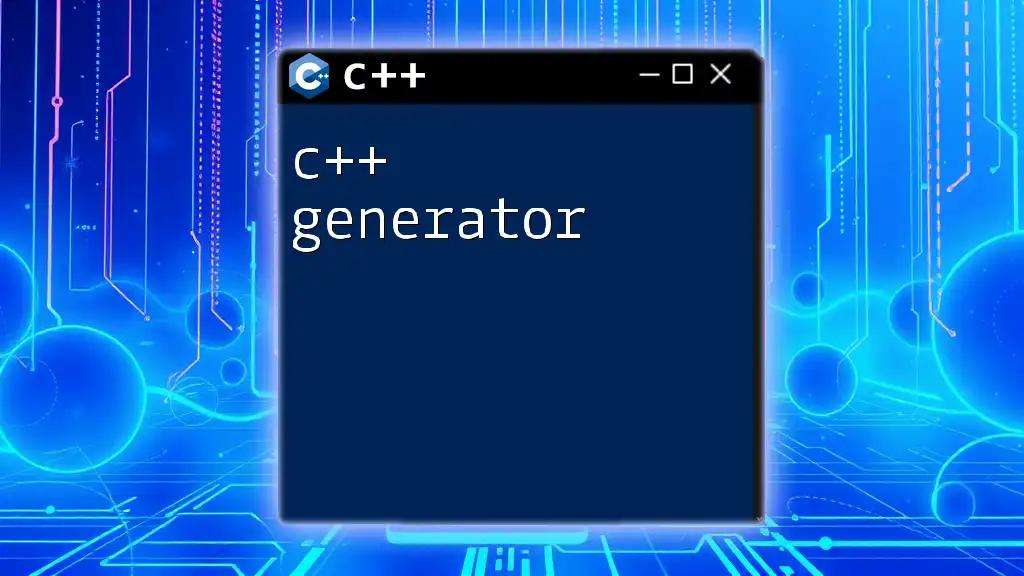
Additional Resources
To further enhance your understanding and application of C++ allocators, consider exploring additional literature and community resources. Engaging with fellow developers through forums can provide insights into innovative techniques and best practices in allocator design and usage.