In C++, `malloc` is a function used to allocate a specified amount of memory on the heap, returning a pointer to the beginning of the allocated memory block.
#include <cstdlib> // Include header for malloc
#include <iostream>
int main() {
int* arr = (int*)malloc(5 * sizeof(int)); // Allocating memory for an array of 5 integers
if (arr == nullptr) {
std::cerr << "Memory allocation failed!" << std::endl;
return 1; // Exit if malloc fails
}
// Use the allocated array (example)
for (int i = 0; i < 5; i++) {
arr[i] = i + 1; // Initialize the array
}
// Free the allocated memory
free(arr);
return 0;
}
Understanding Memory Management in C++
When programming in C++, managing memory effectively is crucial for ensuring your applications run smoothly and efficiently. Memory management revolves around two key types of memory allocation: static and dynamic.
Static memory allocation occurs at compile time and is managed automatically by the program. In contrast, dynamic memory allocation, which involves allocating memory during runtime using functions like `c++ malloc`, gives developers more flexibility.
Dynamic memory primarily operates on the heap, a region of memory dedicated to dynamic allocation, while the stack is used for static memory management. Understanding the distinctions between these memory types is essential because it determines how memory is allocated, accessed, and freed.
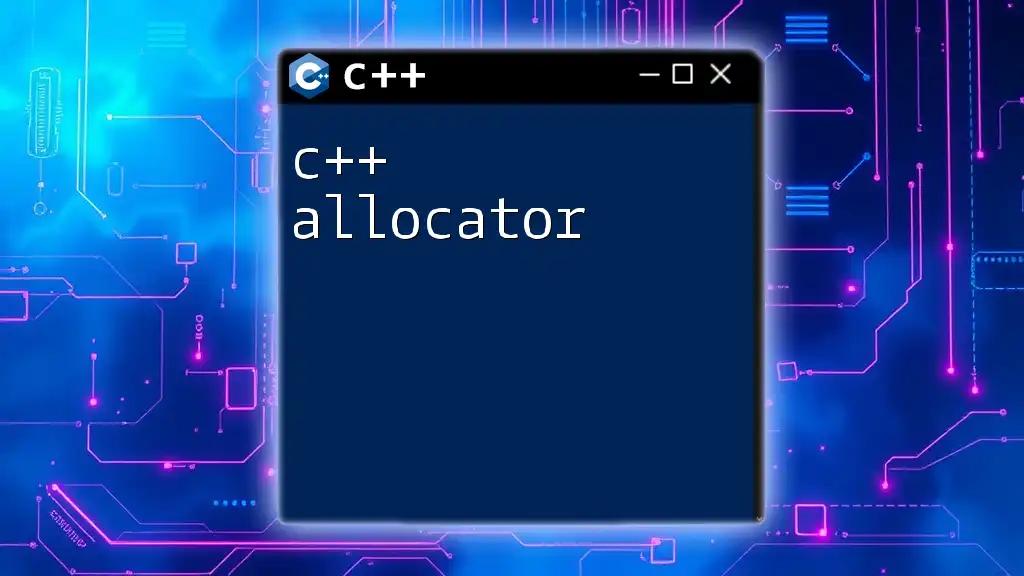
What is Malloc?
`malloc`, short for memory allocation, is a function available in C and C++ (though more commonly associated with C) that is used to allocate a specified amount of memory during runtime. The signature of the `malloc` function is:
void* malloc(size_t size);
This function takes one parameter: the size of the memory block required in bytes. Upon successful allocation, it returns a pointer to the beginning of the allocated memory; if the allocation fails (e.g., insufficient memory), it returns `NULL`.
Understanding the purpose and usage of `malloc` is vital because it allows your program to handle varying data sizes dynamically, a critical feature in many applications.
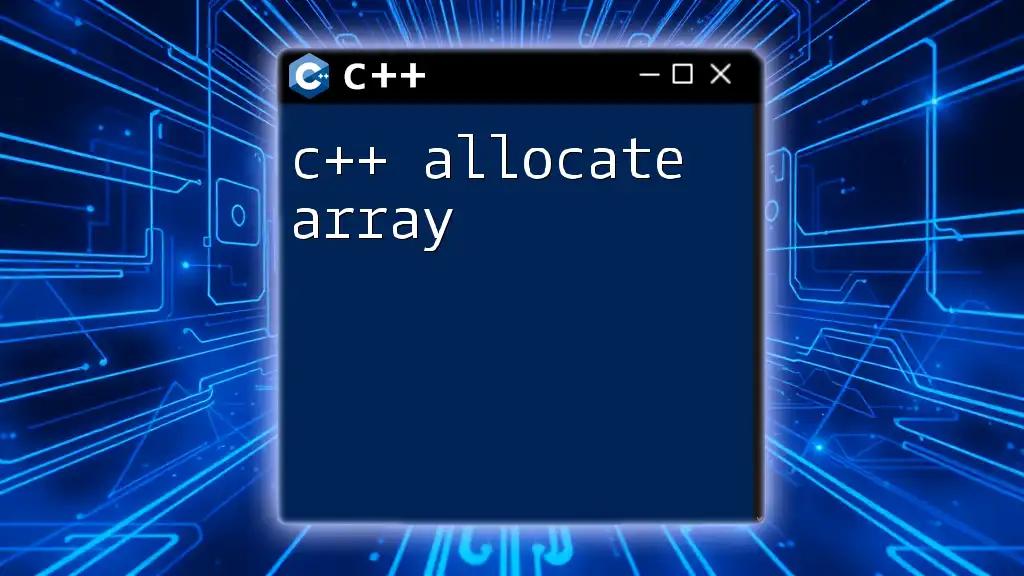
How to Use Malloc in C++
Basic Syntax of Malloc
The syntax of `malloc` can seem straightforward:
void* ptr = malloc(size);
In this code snippet, `ptr` is the pointer that will hold the address of the allocated memory block. The size of memory can be defined explicitly or, more commonly, with the `sizeof` operator to ensure type safety.
Example of Malloc in C++
Let’s take a closer look at a basic example of allocating memory for a single integer:
int* ptr = (int*)malloc(sizeof(int));
Here, we are allocating enough memory to hold a single integer. It is essential to check if the memory allocation was successful, which is done by verifying whether `ptr` is not equal to `NULL`:
if (ptr == NULL) {
// Handle memory allocation failure
std::cerr << "Memory allocation failed!" << std::endl;
} else {
// Use the allocated memory
*ptr = 42; // Assigning a value
std::cout << "Value: " << *ptr << std::endl; // Printing the value
}
This process ensures that your program will not crash due to memory allocation errors.
Malloc for Arrays
Allocating memory for an array is one of the most common uses of `malloc`. For example:
int* arr = (int*)malloc(5 * sizeof(int));
This line allocates memory for an array that can hold five integers. To initialize and print the values, we can use a loop:
if (arr == NULL) {
std::cerr << "Memory allocation failed!" << std::endl;
} else {
for (int i = 0; i < 5; i++) {
arr[i] = i + 1; // Initializing the array
}
for (int i = 0; i < 5; i++) {
std::cout << "Value at index " << i << ": " << arr[i] << std::endl;
}
}
Remember to free the allocated memory after its use:
free(arr);
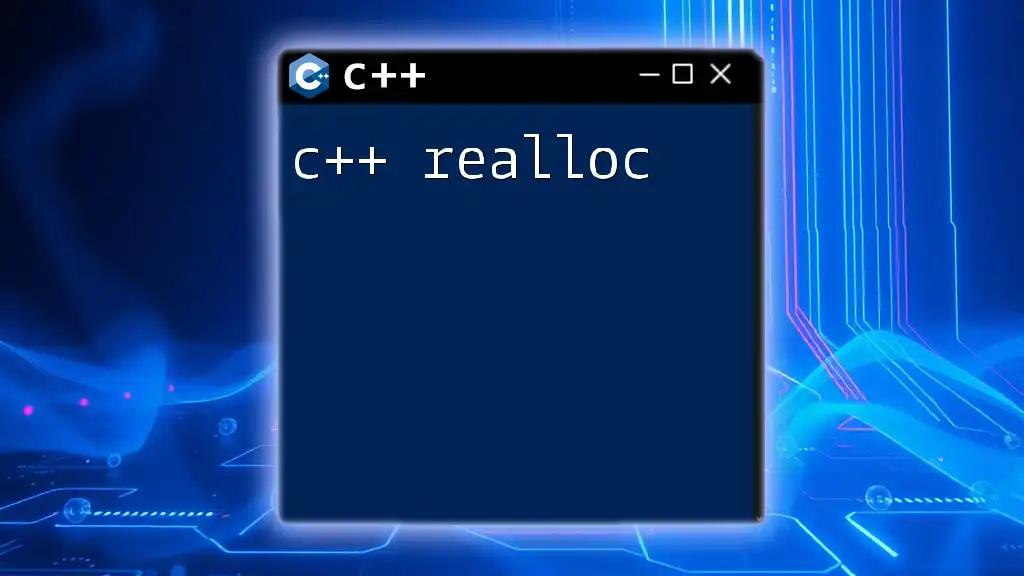
Malloc vs. Other Allocation Methods
Malloc vs. Calloc
While `malloc` is commonly used for memory allocation, an alternative function, `calloc`, also exists but is often misunderstood. `calloc` allocates memory for an array of elements and initializes all bytes to zero.
Here’s a comparison:
int* arr1 = (int*)malloc(5 * sizeof(int)); // Uninitialized
int* arr2 = (int*)calloc(5, sizeof(int)); // Initialized to 0
In scenarios where initialized values are required, `calloc` is preferable. Using `malloc` will require manual initialization, which can lead to errors if not handled properly.
Malloc vs. New Operator
In C++, another way to allocate memory is using the `new` operator:
int* ptr = new int; // Allocates memory for an integer
The `new` operator has several benefits over `malloc`, including automatically calling constructors and providing type safety. Hence, the typecasting necessary with `malloc` becomes unnecessary. You also need to use `delete` to free memory allocated with `new`:
delete ptr;
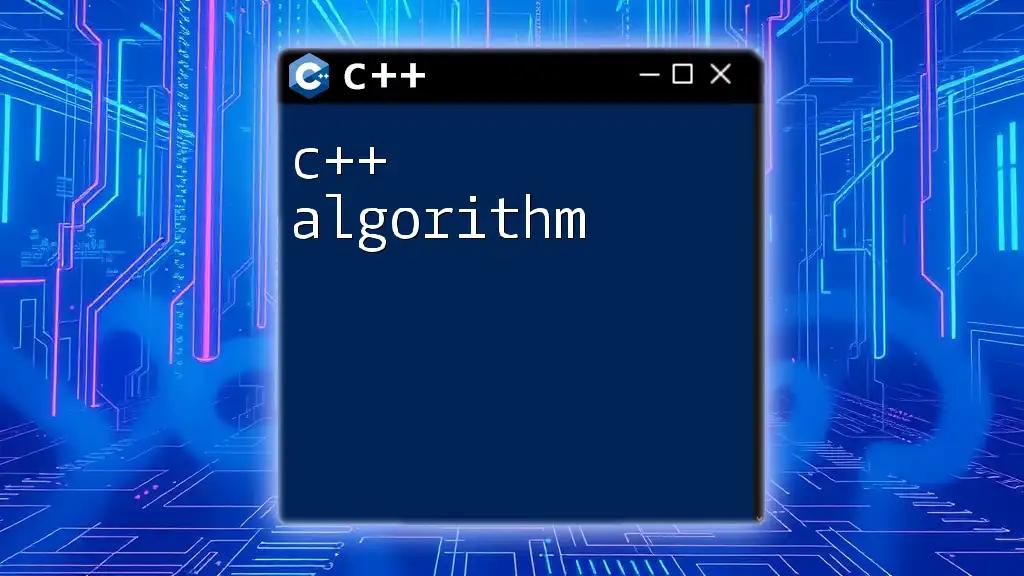
Managing Memory with Malloc
Importance of Freeing Memory
When using `malloc`, it is crucial to free any allocated memory when it is no longer needed. Failing to do so may lead to memory leaks, where memory remains allocated even after it has been used, ultimately consuming system resources and potentially causing performance issues.
Always ensure you include `free()` calls when memory is no longer required:
free(ptr);
Proper Use of Free
The `free` function releases memory back to the operating system, allowing that space to be reused. Incorrect usage, such as attempting to free memory that was not allocated by `malloc`, will lead to undefined behavior. Always ensure the pointer has been allocated properly with `malloc` or `calloc` before passing it to `free`.
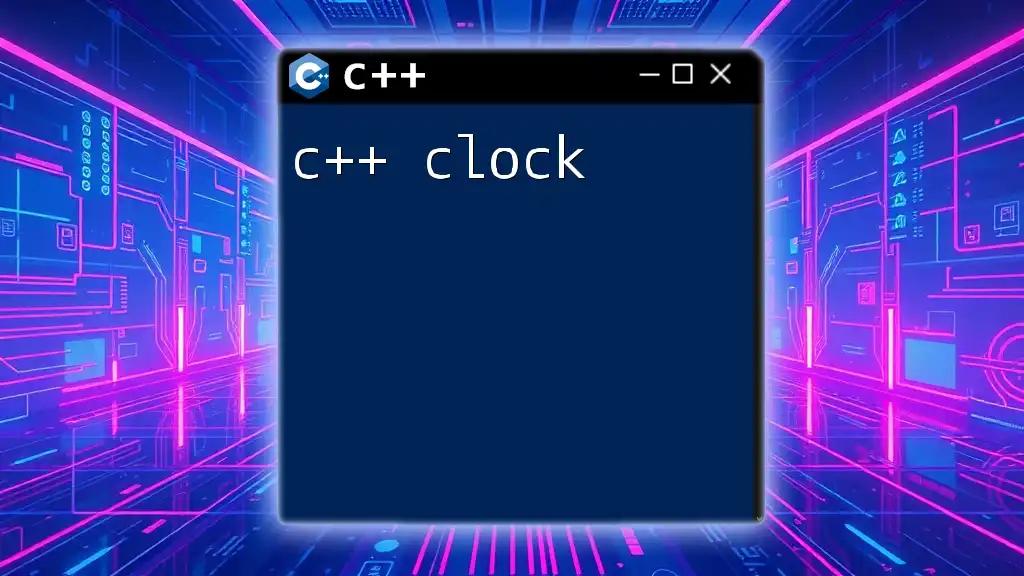
Common Mistakes When Using Malloc
Forgetting to Free Memory
One of the most common mistakes with `malloc` is neglecting to free allocated memory. This oversight can lead to memory leaks, which should be avoided at all costs. Implementing rigorous checks and a disciplined approach to memory management can prevent this issue.
Incorrect Pointer Typecasting
Typecasting can lead to errors if not done correctly. When using `malloc`, pointers must be cast properly. For instance:
double* num = (double*)malloc(sizeof(double));
Improper typecasting can lead to memory corruption, crashes, or unpredictable behavior in your application. Always ensure that the pointer type matches the allocated memory type.
Buffer Overflows and Underflows
Buffer overflows occur when writing more data to a block of allocated memory than it can hold, which can overwrite adjacent memory and lead to unpredictable results. Calculate your sizes correctly and always check boundaries. Likewise, buffer underflows can occur when trying to read memory before the allocated memory block, which can severely impact program integrity.

Advanced Concepts of Malloc in C++
Reallocating Memory with Realloc
Sometimes, memory needs change during the program execution. In such cases, you may need to resize the allocated memory using the `realloc` function:
ptr = (int*)realloc(ptr, 10 * sizeof(int));
`realloc` reallocates the memory block to a new size, and if it fails, the original memory remains allocated. It’s a good practice to store the result back to the same pointer variable and check for `NULL` to handle errors properly.
Performance Considerations
Dynamic memory allocation comes with some inherent overhead. Using `malloc` can be costly in terms of performance, particularly in scenarios that involve frequent allocations and deallocations. It is important to optimize memory usage by reusing allocated memory wherever possible and minimizing the size of dynamically allocated blocks.

Conclusion
In this comprehensive guide on `c++ malloc`, we explored the foundational aspects of memory management in C++. We outlined how to utilize `malloc` effectively, recognized common pitfalls, and highlighted alternatives for memory allocation. For anyone looking to master C++ memory management, understanding `malloc` is an essential step towards developing robust and efficient applications. Continue exploring best practices, as well as advanced memory management techniques, to increase your proficiency in programming with C++.