In C++, `std::nullopt` is used to indicate that an `std::optional` object does not contain a value, allowing for better handling of optional values without using sentinel values.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <optional>
int main() {
std::optional<int> optValue = std::nullopt; // No value assigned
if (!optValue) {
std::cout << "The optional value is empty!" << std::endl;
}
return 0;
}
Understanding `nullopt`
What is `nullopt`?
`nullopt` is a constant provided by the C++ standard library, specifically within the `<optional>` header. It is used to indicate that an `std::optional` object should contain no value. This is a powerful feature that allows developers to express "no value" in a clear and type-safe manner without resorting to pointers or undefined values.
The Role of `std::optional`
`std::optional` is a utility class that encapsulates optional values—meaning a variable can either hold a valid value or signify the absence of a value. It enhances code quality by making nullability explicit at the type level, helping to prevent errors associated with uninitialized values.
For example, imagine a function that returns a user's age. Instead of using a return type that could lead to confusion—like returning -1 to signify "unknown"—using `std::optional<int>` clarifies the intent:
std::optional<int> getUserAge() {
return std::nullopt; // explicitly signifies unknown age
}
When to Use `nullopt`
Utilizing `nullopt` is especially useful when dealing with conditional return values. It provides clarity in scenarios where a value may or may not be present. This leads to better code documentation and reduces the potential for logic errors.
For instance, consider a function that searches for a record in a database. If the record doesn’t exist, returning `nullopt` is more informative than returning a special value that could be misinterpreted.
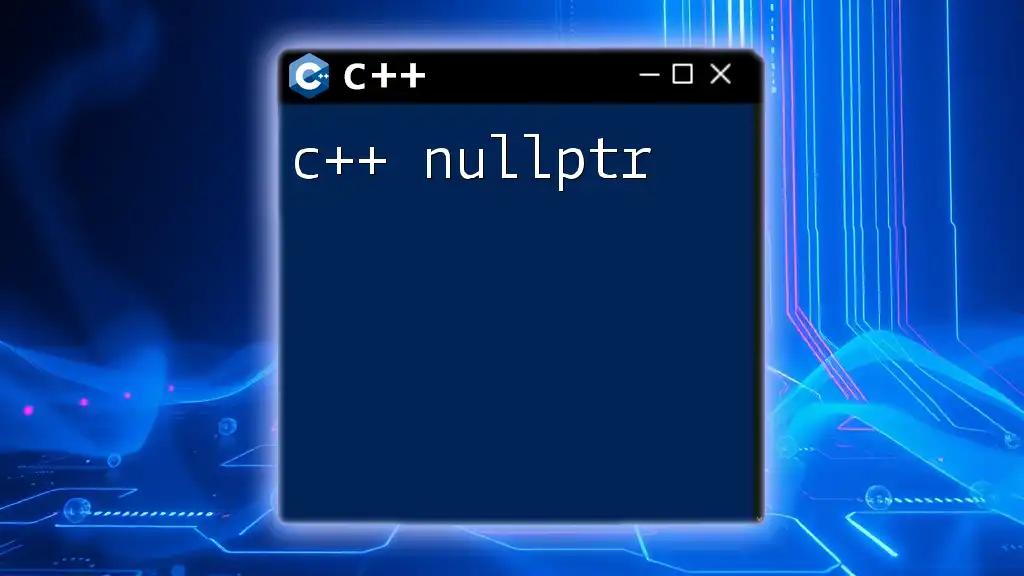
Working with `nullopt`
Including Necessary Headers
To make use of `nullopt`, ensure you include the following header in your code:
#include <optional>
Creating `std::optional` Instances
You can create `std::optional` instances in various ways. By default, an optional variable is initialized to `std::nullopt` if left uninitialized:
std::optional<int> optInt; // Default-initialized to nullopt
std::optional<int> setOptInt = 10; // Initialized with a value
Assigning `nullopt` to `std::optional`
Assigning `nullopt` to an `std::optional` effectively clears its value, setting it to an empty state:
std::optional<int> optInt = 10;
optInt = std::nullopt; // optInt is now empty and does not hold any value
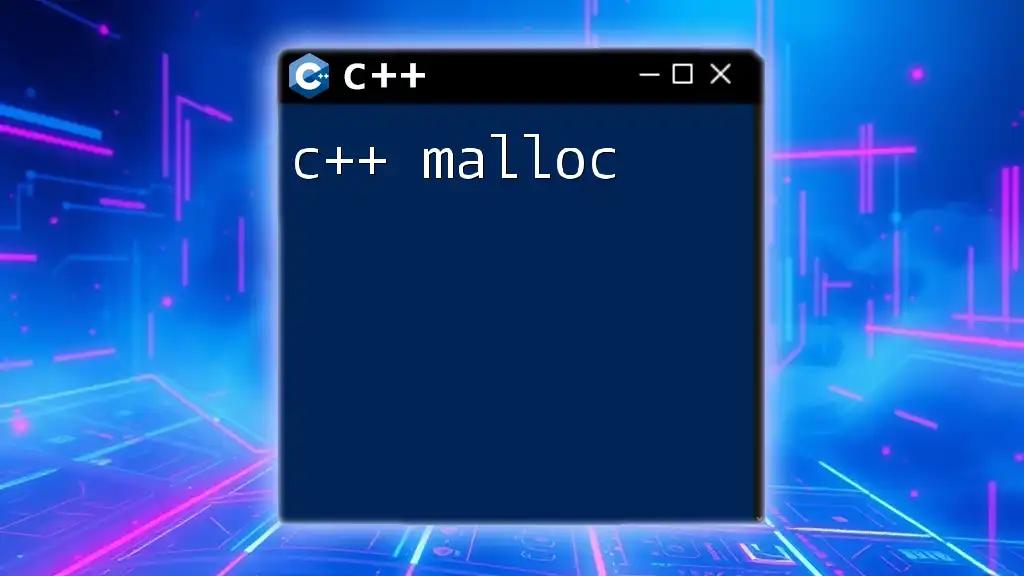
Checking for `nullopt`
Using `has_value()`
To determine whether an `std::optional` contains a value, use the `has_value()` method. This method returns `true` if the optional holds a value and `false` otherwise:
if (!optInt.has_value()) {
std::cout << "optInt contains nullopt" << std::endl;
}
Using Conversion to Boolean
One of the elegant features of `std::optional` is its implicit conversion to boolean. You can directly check if an optional holds a value as follows:
if (!optInt) {
std::cout << "optInt is not set" << std::endl;
}
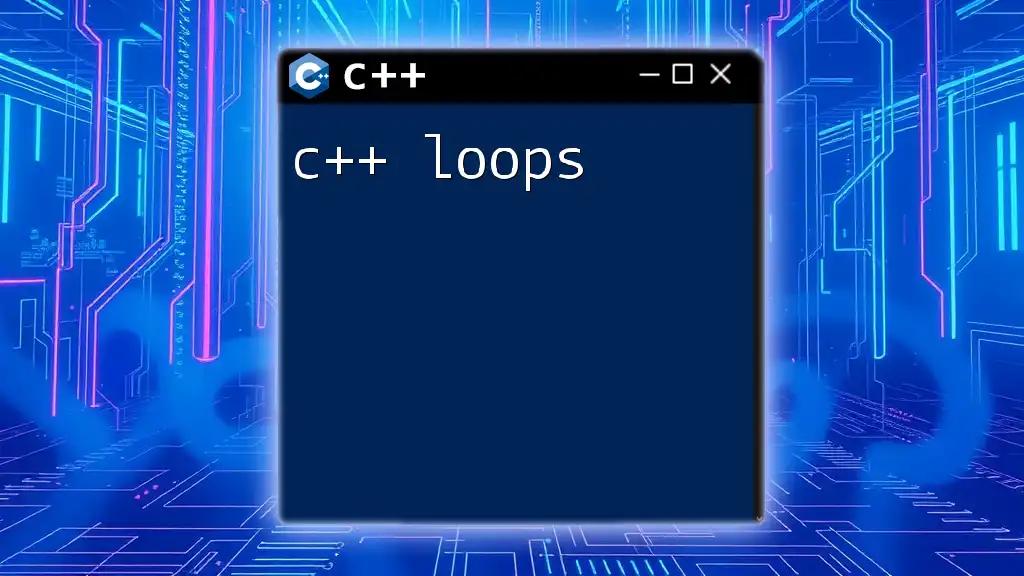
Accessing Values in `std::optional`
Using `value()`
When you are certain that an optional contains a value, you can use the `value()` method to retrieve it. However, if `nullopt` is present, it will throw an exception:
try {
int val = optInt.value(); // Throws if optInt is nullopt
} catch (const std::bad_optional_access&) {
std::cout << "No value available!" << std::endl;
}
Using `value_or()`
A safer way to access the value is through the `value_or()` method, which lets you define a fallback value in case the optional is empty:
int val = optInt.value_or(42); // Returns 42 if optInt is nullopt

Practical Examples
Example 1: Simple Use Case
Here’s a straightforward implementation of a function that returns an optional integer, utilizing `nullopt` for conditional value return:
std::optional<int> getNumber(bool returnNumber) {
if (returnNumber) {
return 42; // A predefined number if the condition is met
}
return std::nullopt; // Return nullopt if the condition fails
}
// Calling the function
std::optional<int> num = getNumber(false);
if (!num) {
std::cout << "No number returned!" << std::endl;
}
Example 2: Real-world Application
In a more complex scenario, consider a function that retrieves user information where the age might be unknown. This illustrates how `nullopt` can be employed elegantly:
struct User {
std::string name;
std::optional<int> age; // Age can be unknown (nullopt)
};
std::optional<User> findUserById(int id) {
if (id == 1) {
return User{"Alice", 30}; // Alice has a known age
}
return std::nullopt; // No user found
}
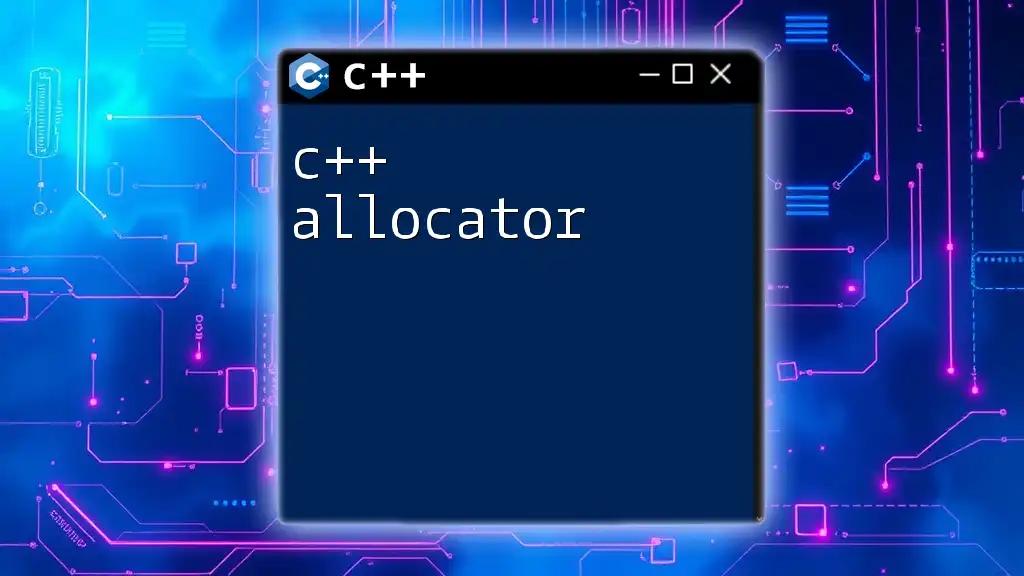
Common Mistakes to Avoid
Misunderstanding the Purpose of `std::optional`
One common mistake is confusing `std::optional` with pointers or using it where alternative solutions like exceptions may be more appropriate. Remember, `std::optional` is about representing optional values safely.
Overusing `nullopt`
While `nullopt` is immensely useful, be cautious about overusing it. It should only be applied when the represented value genuinely can be absent. Excessive reliance on `std::optional` may lead to increased overhead in performance-sensitive contexts without tangible benefit.

Conclusion
In summary, understanding C++ `nullopt` opens up powerful possibilities for dealing with optional values in a clear and type-safe manner. By employing `std::optional` and `nullopt` effectively, you can enhance your code’s safety and readability while reducing the likelihood of errors associated with handling null or uninitialized states. As C++ continues to evolve, embracing such modern features will be essential for crafting robust and maintainable code.