In C++, `NULL` is a macro representing a null pointer, traditionally used in older code, whereas `nullptr` is a keyword introduced in C++11 that provides a type-safe pointer representation.
Here's a code snippet illustrating the difference:
#include <iostream>
int main() {
int* ptr1 = NULL; // NULL representation
int* ptr2 = nullptr; // nullptr representation
std::cout << "ptr1 is " << (ptr1 == nullptr ? "null" : "not null") << std::endl;
std::cout << "ptr2 is " << (ptr2 == nullptr ? "null" : "not null") << std::endl;
return 0;
}
What is Null in C++?
Definition of Null
In C++, null refers to the absence of a value or the indication that a pointer does not point to any valid memory. Historically, null pointers were represented by the integer constant `0`, which could lead to various ambiguities and issues in coding practices.
The Null Pointer Constant
The classic method of creating a null pointer in C++ involves initializing a pointer with the integer value `0`. This is demonstrated in the following code snippet:
int* ptr = 0; // ptr is a null pointer
While this approach works, it can lead to confusion. For instance, the `0` can sometimes be interpreted as an integer, not solely as a pointer. This weakens type safety and makes it challenging to read and understand code at a glance.
Risks of Using Null
Using null, especially in legacy codebases, poses several risks:
-
Null Dereferencing Issues: Attempting to dereference a null pointer can lead to runtime errors and potential crashes. For example:
int* ptr = 0; int value = *ptr; // Undefined behavior: dereferencing a null pointer
-
Code Readability and Maintainability: The integer zero (0) does not convey specific meaning related to pointer initialization. This lack of clarity can make code less maintainable and harder to comprehend for developers interpreting the code in the future.
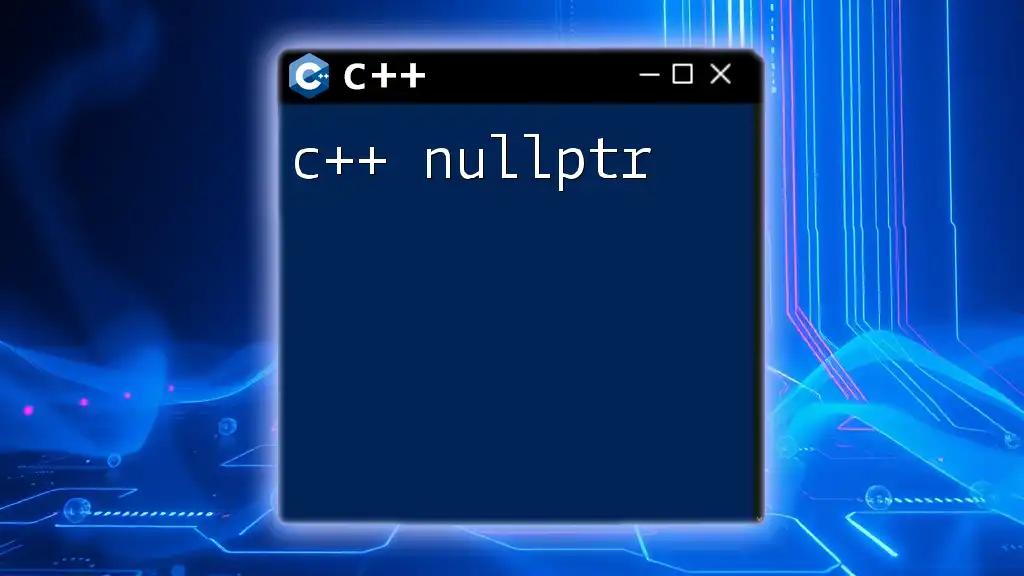
What is nullptr?
Definition of nullptr
Introduced in C++11, `nullptr` is a keyword that represents a null pointer, providing a more clear and type-safe alternative compared to 0 or `NULL`.
Advantages of Using nullptr
-
Type Safety and Clarity: Unlike the integer 0, which can be confused with integer types, `nullptr` is a pointer type. This ensures that the compiler can effectively catch errors where a pointer type is expected but an integer is given. Consider the following example of ambiguity with `0`:
void func(int* p) { /* ... */ } func(0); // This call is ambiguous: it could be interpreted as an int or a pointer
In comparison, using `nullptr` resolves this ambiguity:
void func(int* p) { /* ... */ } func(nullptr); // Clear intent, resolves correctly
-
Enhanced Compatibility with Overloaded Functions: `nullptr` can effectively be used in overloaded function scenarios without ambiguity. This makes the code cleaner and easier to debug.
nullptr in C++11 and Beyond
The introduction of `nullptr` marked a significant shift in C++ programming practices. It fits seamlessly into the language, functioning as a single, generic pointer type that can be used across different scenarios. This modernization of handling null pointers is crucial for contemporary coding, enhancing both safety and clarity.

Key Comparisons between Null and nullptr
Type Safety
A significant distinction between null and `nullptr` lies in type safety. While null pointers (historically represented by `0`) can lead to incorrect type interpretations, `nullptr` explicitly denotes a pointer type. This distinction aids developers in writing safer code.
Readability and Clarity
Using `nullptr` improves code readability. It clearly indicates intent, ensuring that anyone reading the code understands that a pointer is being initialized or compared with null. For instance:
if (ptr == nullptr) { /* Code Logic */ } // Clear check for a pointer
This contrasts with using `0`, where it might be less clear and could require additional comments to explain the context.
Performance Considerations
From a performance perspective, using `nullptr` typically does not introduce any measurable overhead compared to null. In practice, it merely acts as syntactic sugar to enhance the clarity and safety of code. However, its real value comes from preventing bugs that could arise from misinterpretation of null pointers.
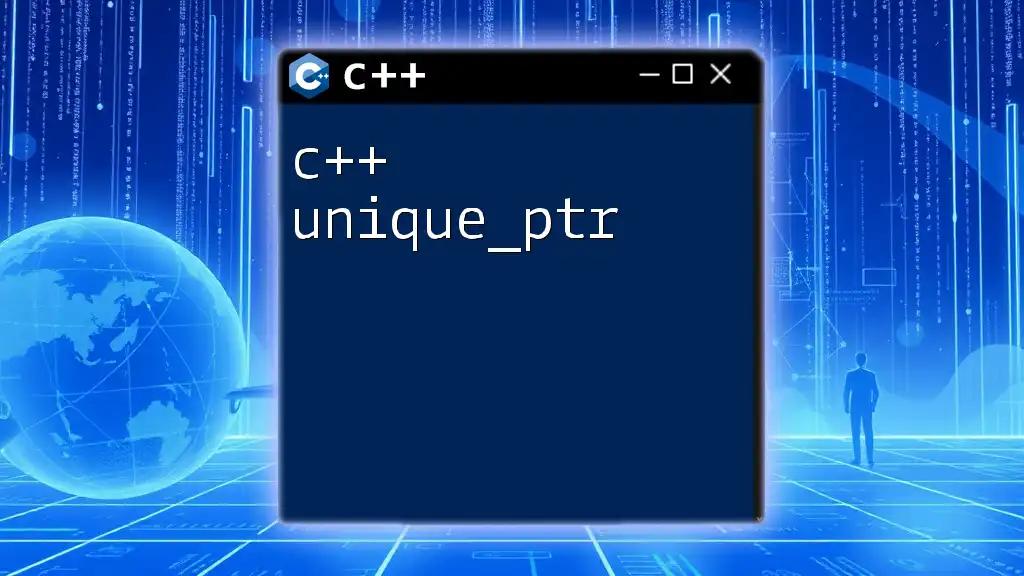
When to Use Null vs nullptr
General Recommendations
In modern C++ development, the recommended practice is to always use `nullptr` instead of null or `0`. This practice aligns with better code design patterns that emphasize clarity and type safety. Avoid using a null pointer constant and favor initializing pointers explicitly with `nullptr`.
Legacy Code Considerations
In legacy codebases where null pointers are extensively used, transitioning to `nullptr` should be approached carefully. Here’s how to manage this transition effectively:
- Evaluate existing code to identify all instances of null pointers.
- Gradually replace instances of `0` or `NULL` with `nullptr`, ensuring to run tests after changes to maintain functionality.
- Encourage and train team members to adopt `nullptr` in any new code they write as part of a coding standards process.

Conclusion
Understanding the differences between C++ null vs nullptr is essential for writing robust, maintainable, and error-free code. The shift from null (or `0`) to `nullptr` embodies a modern approach to pointer management in C++. By embracing `nullptr`, developers can produce safer and more readable code, leading to more efficient and reliable software development.
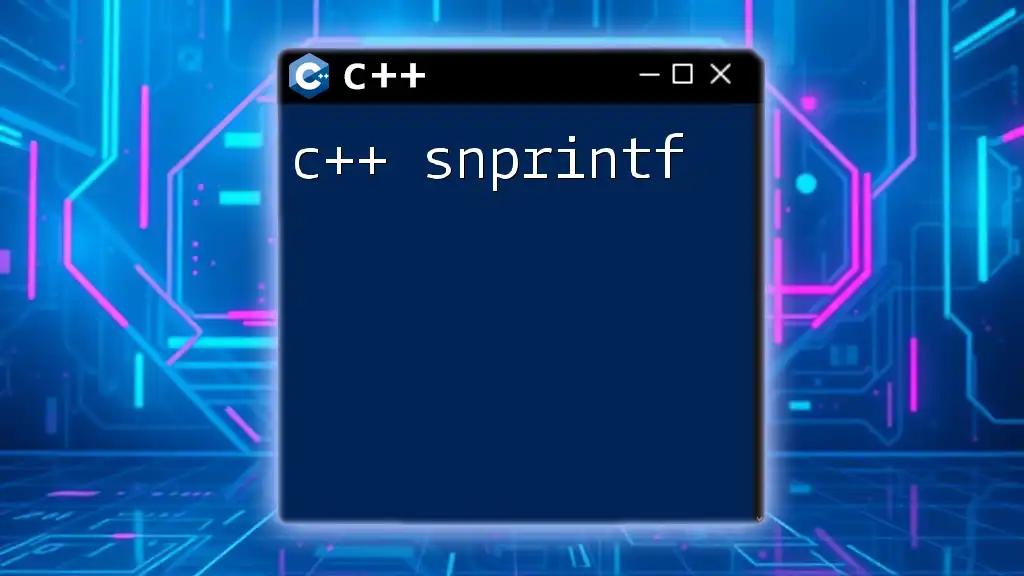
Further Resources
For those looking to deepen their understanding of pointers and memory management in C++, refer to:
- The official C++ documentation
- Recommended texts that elaborate on advanced C++ topics
- Online courses focused on modern C++ and its best practices
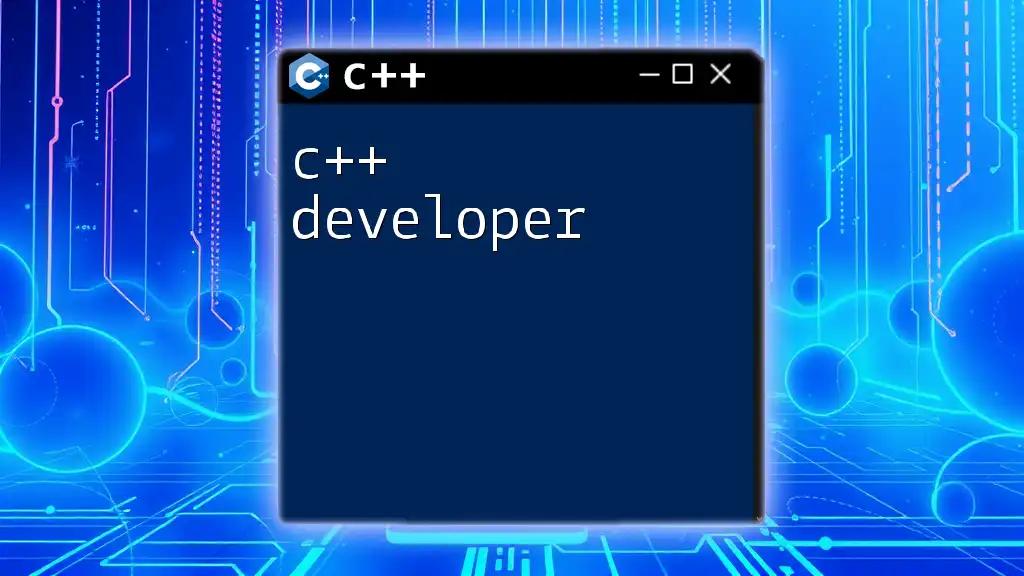
FAQs
Common Questions
What happens if I use null instead of nullptr? Using null may lead to ambiguous type interpretations, making your code less safe and harder to debug.
Can you mix nullptr with legacy code? Yes, but care must be taken to ensure that existing code remains functional after any changes.
Is nullptr standard across different compilers? Yes, `nullptr` is part of the C++11 standard and supported by all modern C++ compilers.