In C++, `nullptr` is used to represent a null pointer, indicating that the pointer does not point to any valid memory location.
Here's a simple code snippet to illustrate this:
#include <iostream>
int main() {
int* ptr = nullptr; // declaring a null pointer
if (ptr == nullptr) {
std::cout << "The pointer is null." << std::endl;
}
return 0;
}
Understanding Null in C++
What is Null?
In C++, null represents the absence of a value or object. It serves as a placeholder to indicate that a pointer does not point to any meaningful data. This concept is crucial in programming, as it helps prevent errors associated with uninitialized pointers, which can lead to undefined behavior.
The Null Pointer
A null pointer is a pointer that does not point to any valid memory location. It is essential to differentiate between `null`, `nullptr`, and `NULL`. Understanding these differences can significantly improve the safety and reliability of your code.
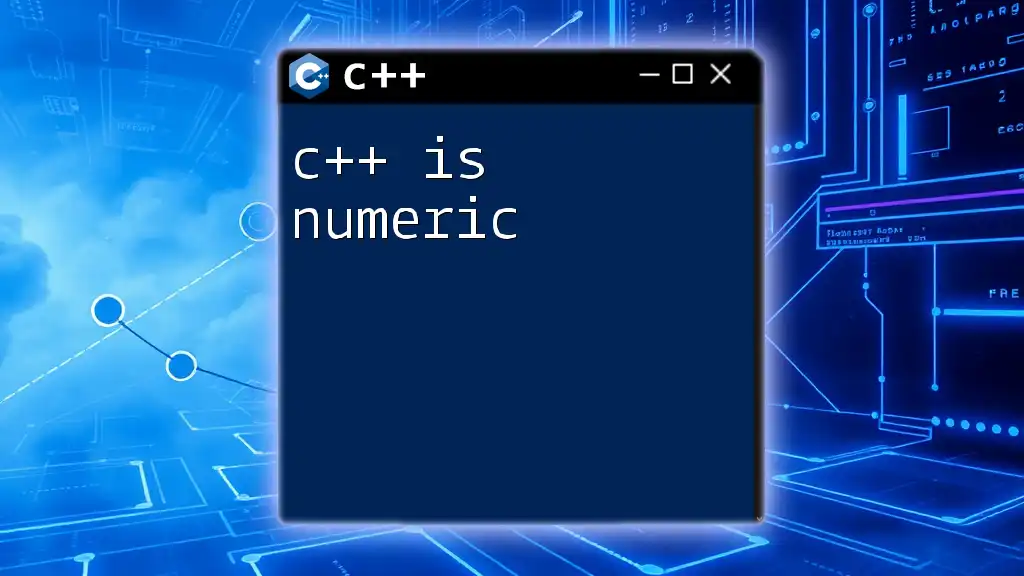
C++ Null Concepts
What is nullptr?
Introduced in C++11, `nullptr` is a type-safe null pointer constant. It is specifically designed to avoid ambiguity when dealing with overloaded functions, where using a generic null would be problematic. The key benefits of using `nullptr` include:
- Type Safety: Unlike `NULL`, which can lead to confusion in overloading scenarios, `nullptr` unambiguously represents a null pointer.
- Clear Intent: Using `nullptr` clearly indicates that you are working with a pointer.
Code Example:
int* p = nullptr; // Safer than using NULL
Legacy NULL
Before the introduction of `nullptr`, the `NULL` macro was widely used as a null pointer constant. However, its use can lead to several issues:
- Ambiguity: In overloaded functions, it can be interpreted as both an integer and a pointer, leading to unexpected behavior.
- Potential Pitfalls: Using `NULL` can make code less readable.
Code Example:
int* p = NULL; // Could lead to confusion in overloaded functions
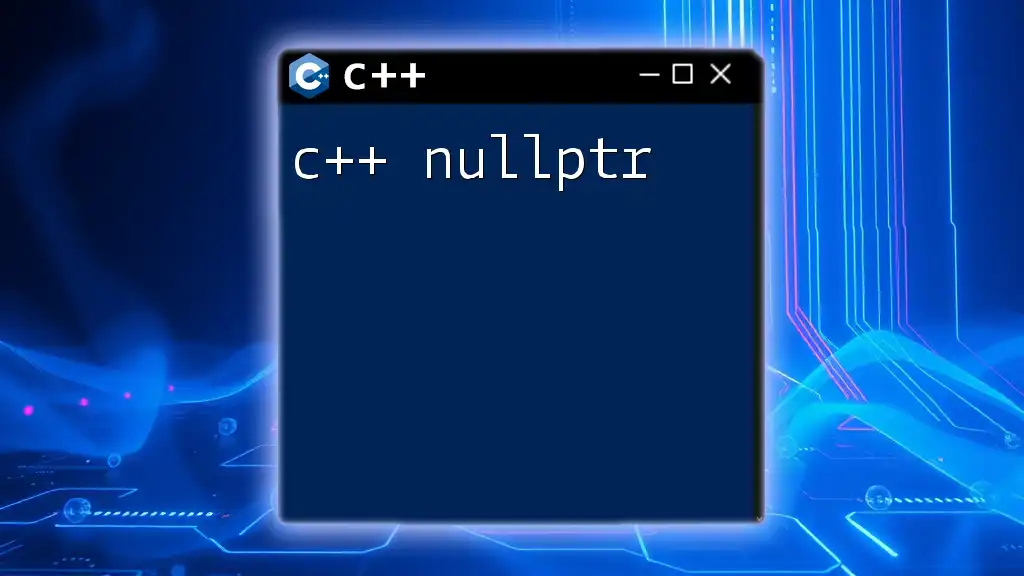
Practical Applications of Null
Checking for Null Pointers
Checking for null pointers is critical to prevent dereferencing a pointer that does not point to any valid memory. This step is essential for maintaining robust code.
Code Example:
if (p == nullptr) {
// Handle null pointer
}
Using Null in Function Parameters
When writing functions that take pointers as parameters, it’s common to accept `nullptr` as a valid argument. This allows for flexible function design and improved error handling.
Code Example:
void process(int* p) {
if (p == nullptr) {
// Handle null case
}
}
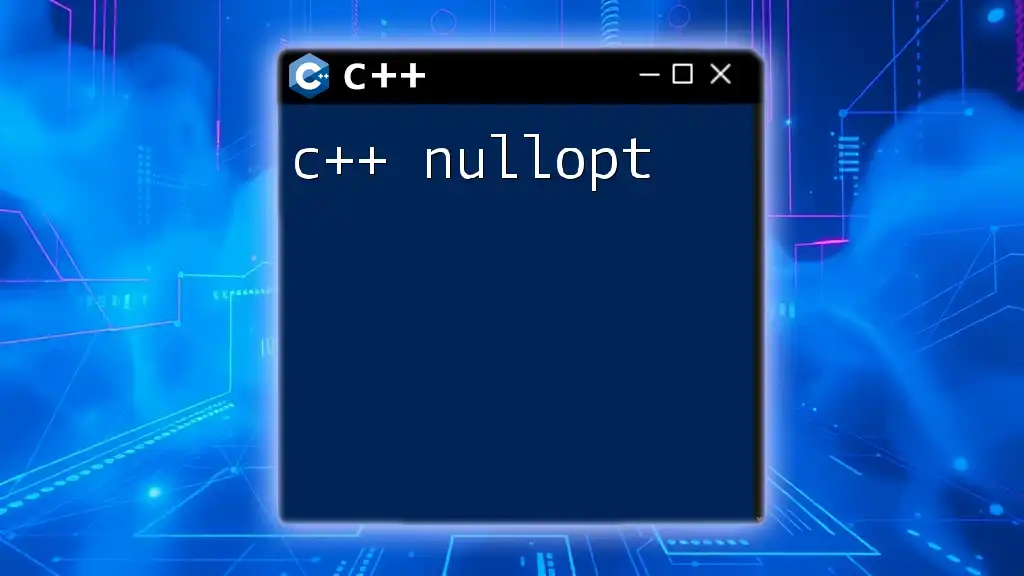
Common Errors Associated with Null
Dereferencing a Null Pointer
Dereferencing a null pointer is one of the most common mistakes in C++. Attempting to access the data that a pointer supposedly references when the pointer is null will lead to runtime errors or crashes.
Code Example:
if (p != nullptr) {
*p = 10; // Safe dereferencing
}
Memory Leaks and Null Pointers
Improper use of null pointers can also contribute to memory leaks. If you delete a pointer without checking if it is null, it can lead to undefined behavior or access violations.
Code Example:
delete p; // Make sure p is not nullptr to avoid issues
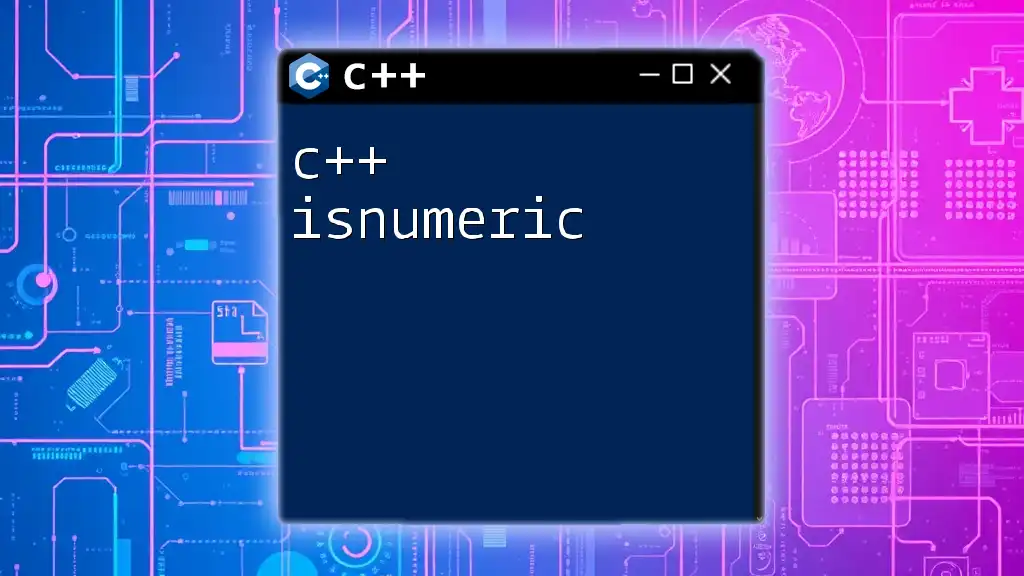
Advanced Topics
Smart Pointers and Null
To manage dynamic memory more safely, C++ offers smart pointers like `unique_ptr` and `shared_ptr`. These smart pointers help manage ownership of dynamically allocated objects and automatically handle memory when they go out of scope, making it much safer to work with pointers.
Code Example:
std::unique_ptr<int> uptr(nullptr); // Smart pointer initialized to nullptr
Nullability in C++
C++17 introduced `std::optional`, which provides a way to express an optional value. This can be particularly useful for handling cases where a value may or may not be present.
Code Example:
std::optional<int> opt = std::nullopt;
if (!opt) {
// Handle the case where opt is null
}
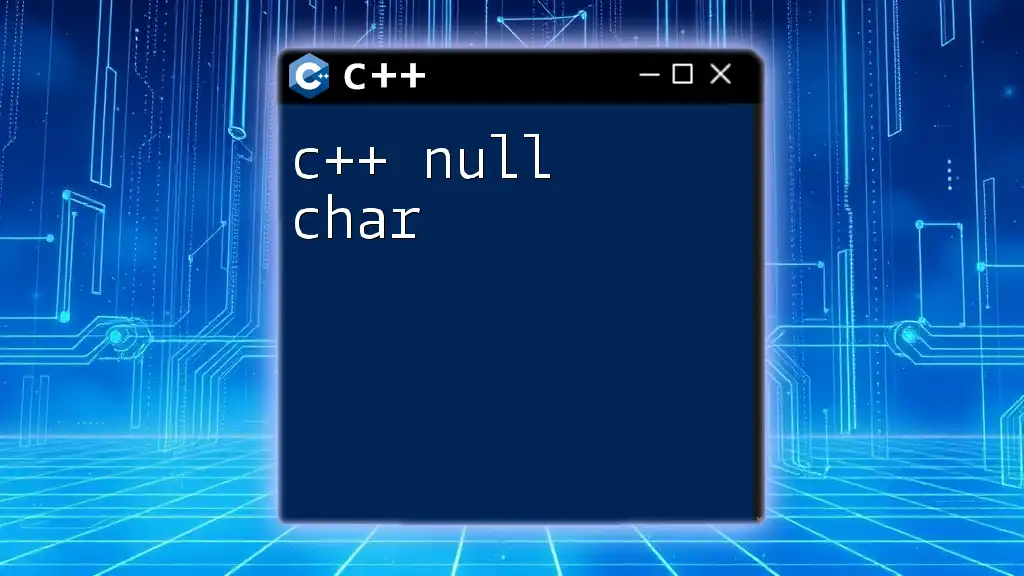
Best Practices
Tips for Handling Null Pointers
- Always initialize pointers to `nullptr`. This helps to avoid accidental dereferencing of uninitialized pointers.
- When passing pointers as function arguments, check for null pointers before dereferencing to avoid runtime errors.
- Use `nullptr` where possible for clearer and safer code.
Debugging Null Pointer Issues
Debugging null pointer exceptions can be challenging. Here are some strategies to help:
- Utilize debugging tools that can help track pointer values.
- Incorporate sanity checks throughout your codebase to assert that pointers are valid before use.
- Leverage modern IDEs that highlight potential null pointer dereference during coding to catch errors early.
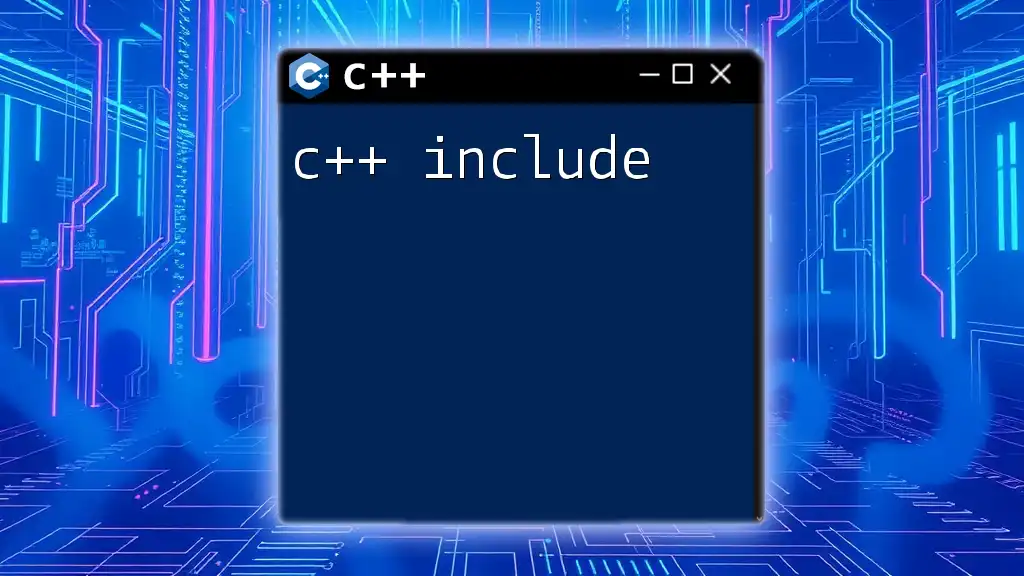
Conclusion
Understanding how C++ is null is fundamental for any C++ programmer. It is crucial not only for error prevention but also for writing clear, maintainable code. By practicing safe null handling and understanding null pointers, you can significantly enhance the quality and reliability of your C++ projects.
Additional Resources
For those looking to deepen their knowledge on this topic, consider exploring the following resources:
- C++ Standard Library documentation
- Online courses focused on memory management in C++
- Advanced C++ programming books that cover pointers in depth