In C++, the `if-else` statement allows you to execute different code blocks based on a condition's truth value.
#include <iostream>
int main() {
int number = 10;
if (number > 0) {
std::cout << "The number is positive." << std::endl;
} else {
std::cout << "The number is not positive." << std::endl;
}
return 0;
}
Understanding the If-Else Statement
What Is an If-Else Statement?
The if-else statement is a fundamental construction in C++ that allows developers to control the flow of their programs based on specific conditions. It enables decision-making by executing particular blocks of code when a condition evaluates to true and alternative code when it evaluates to false. This means that you can steer your program's behavior in a logical direction as per the needs of your application.
Syntax of the If-Else Statement
The basic structure of the if-else statement in C++ is as follows:
if (condition) {
// code to be executed if condition is true
} else {
// code to be executed if condition is false
}
This simple syntax allows you to define a condition (the expression inside the parentheses) and specify what should happen if that condition holds true versus false. It’s essential to ensure that the condition is a boolean expression, which evaluates to either true or false.
Example of a Simple If-Else Statement
Consider a basic example that checks whether a number is positive or negative:
int a = 5;
if (a > 0) {
std::cout << "Positive number";
} else {
std::cout << "Negative number or zero";
}
In this snippet, the program checks whether the variable `a` is greater than zero. If it is, it outputs "Positive number"; otherwise, it outputs "Negative number or zero". This example demonstrates the practical application of a simple if-else statement.

Types of If-Else Statements
Simple If Statement
A simple if statement evaluates a condition and executes code only if that condition is true.
if (num > 10) {
std::cout << "Number is greater than 10";
}
Here, if `num` exceeds 10, the message is displayed. If not, the program simply continues without taking any action.
If-Else Statement
In the case of an if-else statement, there are two possible outcomes: one for true and one for false.
if (num % 2 == 0) {
std::cout << "Even number";
} else {
std::cout << "Odd number";
}
This construction checks if `num` is even or odd. The code in the first block executes for even numbers, while the else block handles odd numbers, providing clear feedback based on the nature of `num`.
If-Else If Ladder
The if-else if ladder allows for evaluating multiple conditions in a structured way.
if (score >= 90) {
std::cout << "Grade A";
} else if (score >= 80) {
std::cout << "Grade B";
} else if (score >= 70) {
std::cout << "Grade C";
} else {
std::cout << "Grade D";
}
In this example, the program evaluates `score` and outputs a corresponding grade. Each condition is checked sequentially, allowing complex decision-making with multiple outcomes.
Nested If Statements
Nested if statements allow for evaluating conditions within another if statement, creating a hierarchy of conditions.
if (age > 18) {
if (age < 30) {
std::cout << "Young adult";
} else {
std::cout << "Adult";
}
}
In this case, the outer `if` checks if `age` is greater than 18, while the inner `if` further categorizes the age into young adult or adult. This nesting adds layers to your logic, enabling more nuanced control.
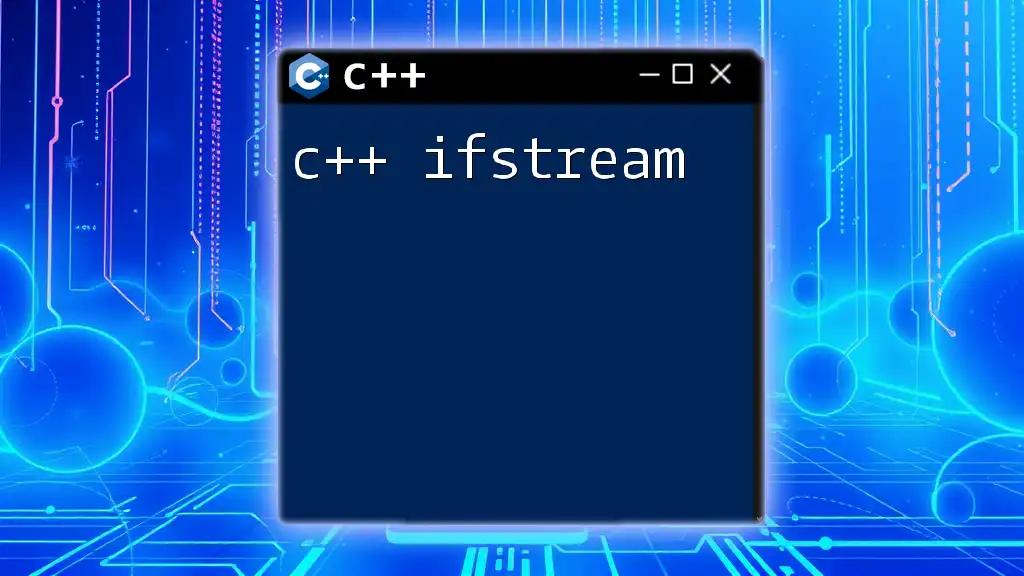
Best Practices Using If-Else Statements
Keep Conditions Simple
Clarity is key in programming. Use straightforward conditions to improve readability. Complex expressions can lead to confusion for anyone maintaining your code, including yourself.
Maintain Code Readability
Formatting your code properly is essential. Use indentation consistently and keep your lines short. Readable code not only helps others understand your logic but also makes it easier to identify and fix errors later.
Avoid Deep Nesting
While nested if statements are powerful, excessive nesting can make your code cumbersome. Aim for a balance between simplicity and functionality. If you find yourself nesting multiple layers deep, consider refactoring your code or using a switch statement for clarity.
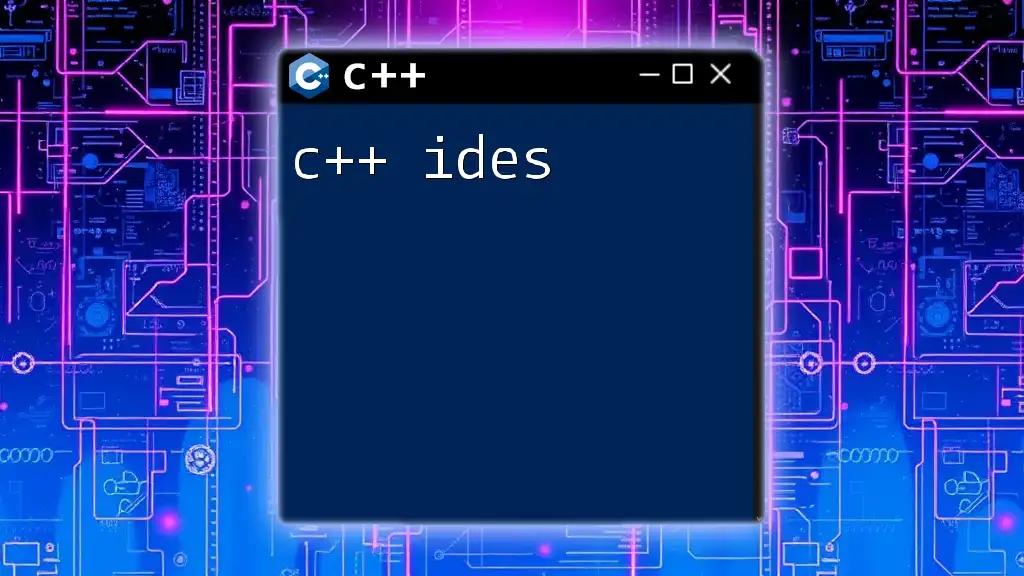
Common Mistakes to Avoid
Using Assignment Instead of Comparison
One common error is using an assignment operator (`=`) instead of a comparison operator (`==`). This mistake won't trigger a syntax error but will lead to unexpected behaviors.
// Incorrect
if (a = 5) { // This assigns 5 to a instead of checking equality
// code
}
This code snippet will always evaluate to true, leading to unintended outcomes. Ensure you use `==` when you want to check for equality.
Forgetting Braces
Always use braces `{}` to contain the code blocks, especially when writing conditional statements that involve multiple lines of code. Even if the code block is a single line, braces help prevent logical errors.

Conclusion
The C++ if else statement is an essential construct for managing program flow through decision-making. Mastering its use enables developers to create dynamic and responsive applications. By practicing the examples and adhering to the best practices outlined, you can enhance your proficiency with conditional statements in C++.
Make the most of resources and tutorials available online to expand your understanding and become adept with C++. Each time you implement an if-else statement, you add a new layer of logic to your programming skill set.