In C++, "offset" typically refers to the difference in memory addresses between two pointers, which can be calculated using pointer arithmetic.
Here’s a code snippet demonstrating how to calculate an offset between two pointers:
#include <iostream>
int main() {
int arr[] = {10, 20, 30, 40};
int* ptr1 = &arr[0]; // pointer to the first element
int* ptr2 = &arr[2]; // pointer to the third element
// Calculate the offset
std::ptrdiff_t offset = ptr2 - ptr1;
std::cout << "Offset between ptr2 and ptr1: " << offset << std::endl; // Output: 2
return 0;
}
Understanding Offsets in C++
Definition of Offset
In programming, offset refers to the distance (in bytes) from a specific reference point, such as the beginning of a data structure. In C++, offsets are crucial for understanding how data is organized in memory. They play a significant role in pointer arithmetic and array manipulation, as every variable and data structure has a defined starting address. Mastering offsets enables developers to navigate and manipulate memory more effectively.
Memory Layout in C++
C++ uses two primary regions for memory allocation: the stack and the heap.
-
Stack: This is where local variables are stored. Memory is allocated and released in a Last In, First Out (LIFO) fashion. Offsets in stack memory help understand how close or far variables are from each other based on their order of declaration.
-
Heap: This region is used for dynamic memory allocation, where variables persist beyond their scope. Offsets in heap memory can be more complex due to fragmentation and variable lifetimes.
Understanding the layout of memory helps in calculating offsets accurately, allowing for efficient data access and manipulation.
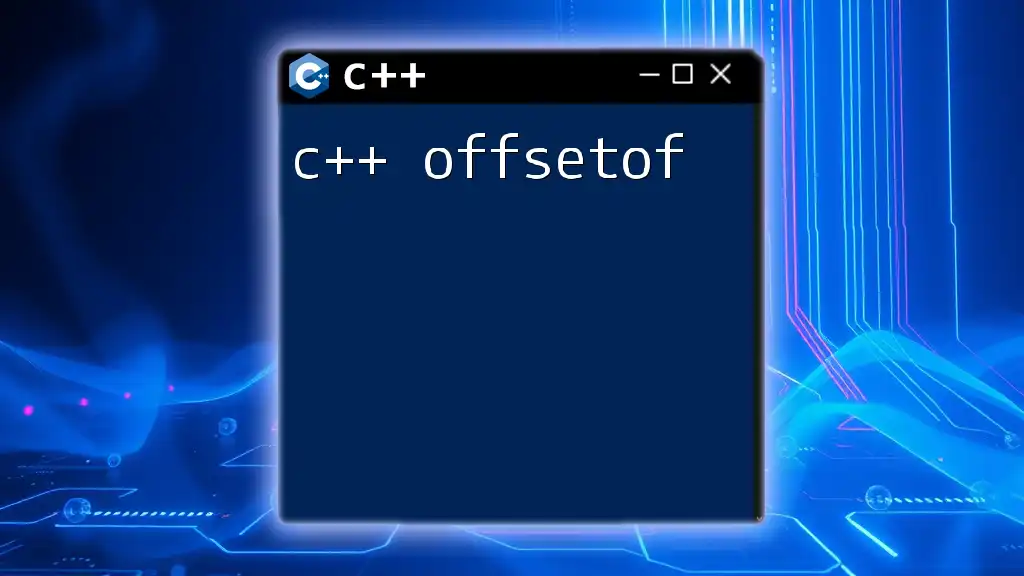
Calculating Offsets
Addressing in C++
C++ manages memory through addresses and pointers, fundamental concepts for calculating offsets. A pointer is a variable that holds the memory address of another variable.
Pointer Arithmetic allows developers to move through arrays or structures by adjusting the pointer's address. For instance, adding to a pointer increases the address it points to, effectively moving through the memory allocated for that data type.
Here’s a simple example illustrating pointer arithmetic:
void pointerExample() {
int arr[3] = {10, 20, 30};
int* ptr = arr; // points to the first element
// Accessing elements using pointer and offset
std::cout << *(ptr + 1); // Output: 20
}
Structs and Offsets
Understanding offsets is particularly important when dealing with structs. Structs bundle different data types together. The layout in memory defines how much space each member takes up and their relative positions.
Here’s how to calculate the offset in a struct:
#include <iostream>
#include <cstddef> // for offsetof
struct Example {
int a; // offset 0
double b; // offset 4 (on many systems, double might need padding)
};
int main() {
std::cout << "Offset of a: " << offsetof(Example, a) << std::endl; // Output: 0
std::cout << "Offset of b: " << offsetof(Example, b) << std::endl; // Output: 8
}
The `offsetof` macro from `<cstddef>` provides a straightforward method to determine the byte offset of a member within a struct. This is particularly useful for serialization, data mapping, and interfacing with hardware.
Using Offsets in Classes
Class layouts can be more complex due to inheritance and virtual functions. In C++, the presence of virtual functions might introduce additional overhead, as it needs to support dynamic binding. This changes the offsets for member variables based on the inheritance structure.
Consider this example:
class Base {
virtual void method1();
};
class Derived : public Base {
void method2();
};
In this case, the offset of `method2` in the `Derived` class may differ based on whether `Base` contains virtual functions. This knowledge is essential for optimizing class design and understanding performance implications.
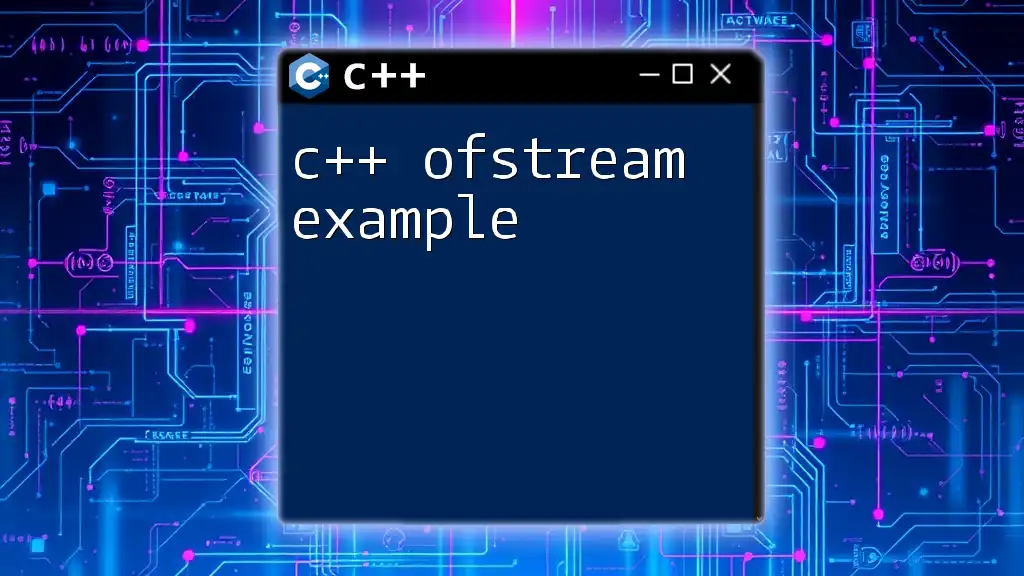
Practical Applications of Offsets
Memory Alignment and Performance
Memory alignment is the practice of arranging data in memory at addresses that are multiples of their sizes. Many modern CPUs require this alignment for optimal performance. Misalignment can lead to increased access times or even faults.
For example, an `int` might require 4-byte alignment:
struct Misaligned {
char a; // 1 byte
int b; // 4 bytes (could cause misalignment)
};
Properly aligning data types will result in offsets that match optimal access patterns and improve performance.
Low-Level Memory Manipulation
Offsets are also crucial for low-level memory manipulation. In certain situations, developers may need to adjust data directly in memory.
Here's an example:
void manipulateArray() {
int arr[3] = {1, 2, 3};
int* ptr = arr;
// Accessing using offset
std::cout << *(ptr + 1); // Output: 2
}
However, caution is vital: while powerful, manipulating memory directly can lead to unpredictable behavior if not handled properly.
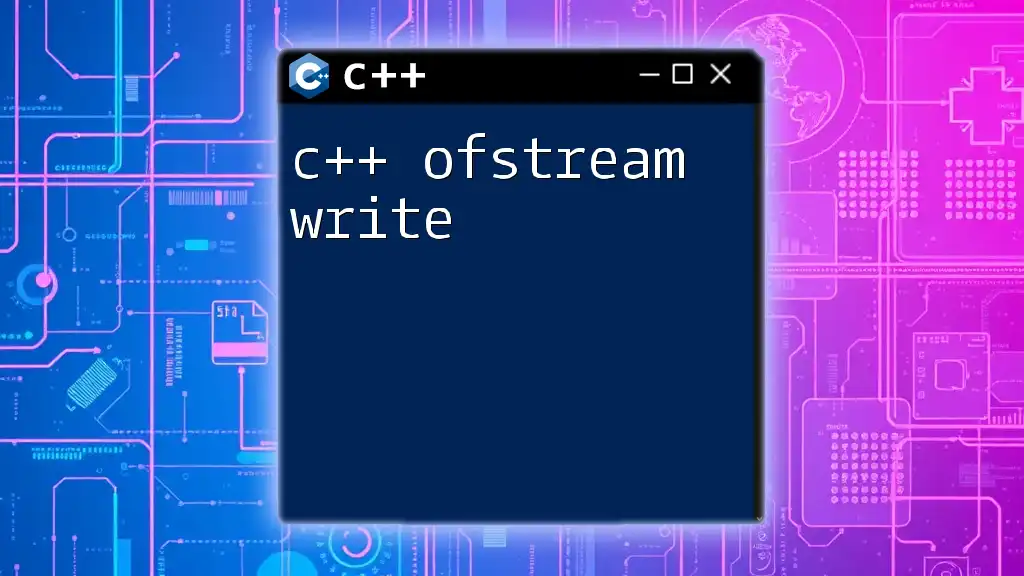
Best Practices with Offsets
Avoiding Common Pitfalls
When working with offsets, developers frequently encounter common pitfalls. A major mistake is assuming memory layouts are the same across different platforms or compiler settings. Different compilers and architectures may introduce padding and alignment, altering offsets.
Using the `offsetof` macro can mitigate these issues, providing accurate byte offsets regardless of compiler differences.
Debugging with Offsets
Debugging tools such as GDB can help visualize memory layouts and offsets. By examining memory content and structure layouts, developers can ensure that their calculations align with actual memory usage.
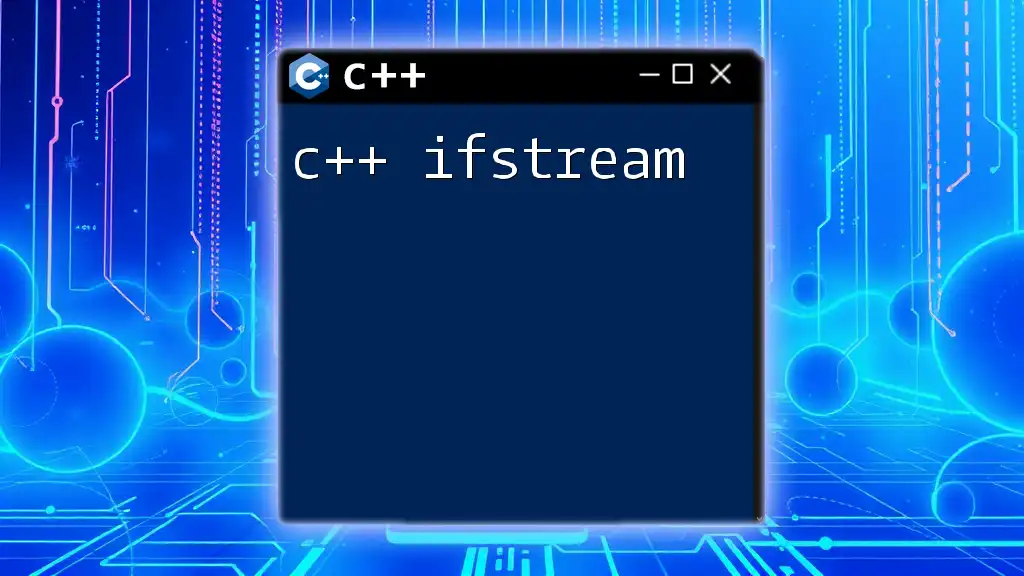
Conclusion
Understanding C++ offsets is essential for efficient programming. They provide insight into the memory layout of variables, enhance performance through proper alignment, and offer control over low-level memory management. As you begin to explore offsets in your C++ projects, practice is key. Experimenting with struct layouts, pointer arithmetic, and debugging tools can deepen your understanding.
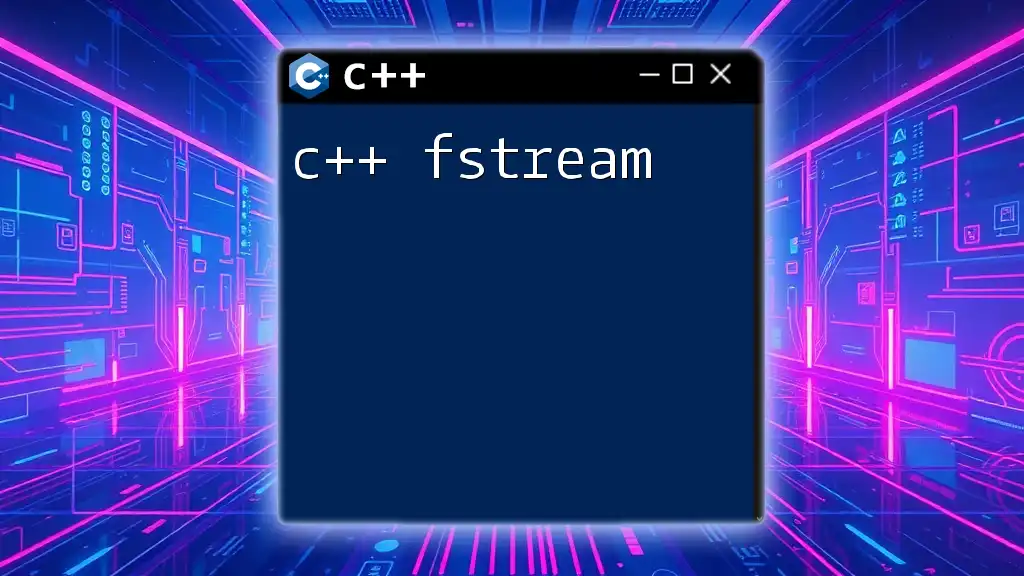
Call to Action
If you're interested in mastering more about C++ commands and best practices, consider subscribing for upcoming posts. Join our community and download a free guide on C++ offsets to kickstart your learning journey!
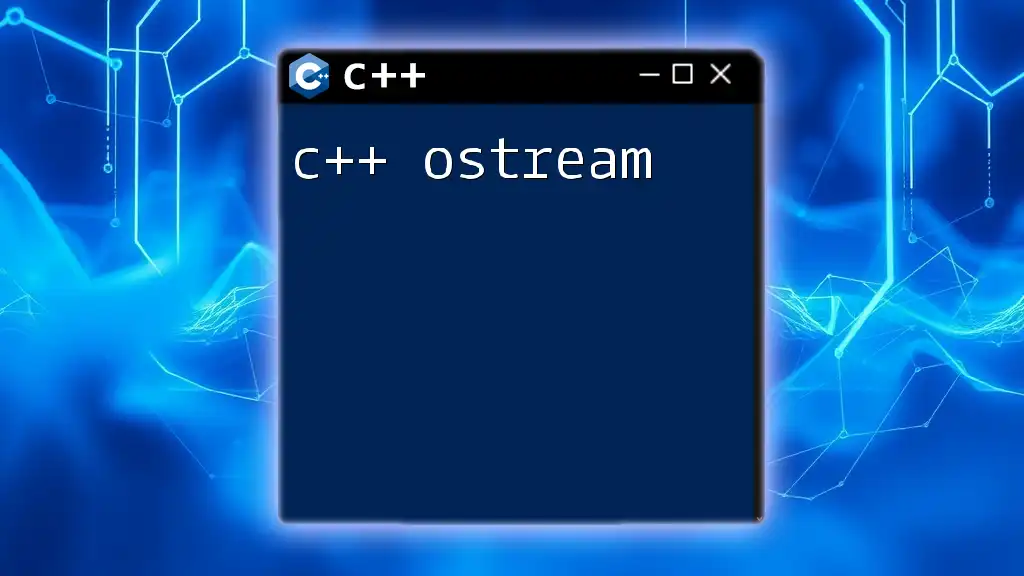
Additional Resources
For further reading on C++ offsets and memory management, consider these resources:
- “The C++ Programming Language” by Bjarne Stroustrup
- "Effective C++" by Scott Meyers
- Online documentation on standard libraries and memory management in C++
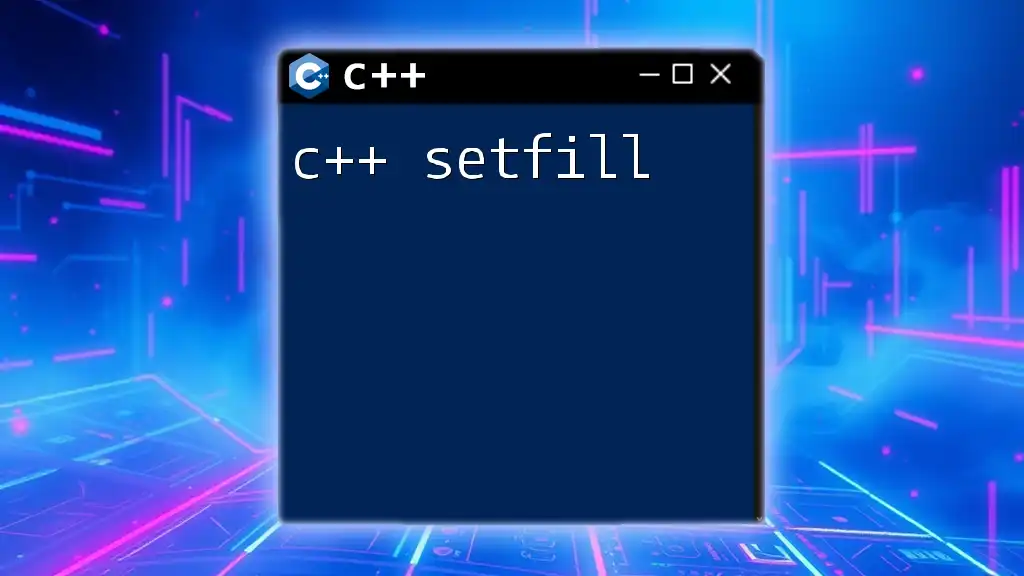
FAQs about C++ Offset
What is the difference between offset and pointer?
Offsets represent positions in memory relative to a specific reference point, while pointers hold the actual addresses of variables or data.
Why is it important to understand offsets for performance?
Offsets help ensure that data accesses align with memory structures, preventing slowdowns due to misalignment and ensuring cache efficiency.
Can offsets be negative in C++?
Offsets themselves are typically non-negative, but pointer arithmetic allows for decrementing pointers, effectively accessing earlier memory locations. However, care should be taken to avoid undefined behavior.