The `setwidth` manipulator in C++ is used to set the minimum width of the next input/output field, allowing you to control the layout of your outputs in a formatted way.
Here's a code snippet demonstrating its use:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setw(10) << "Hello" << std::setw(10) << "World" << std::endl;
return 0;
}
What is `setw` in C++?
`setw` is a function in C++ primarily used for formatting the output of streams, particularly with `std::cout`. It is part of the `<iomanip>` library, designed to provide manipulators that adjust the properties of the output streams. The `setw` function allows you to control the width of the output for the next item to be printed. If the output is shorter than the specified width, spaces (or another specified character if combined with `setfill`) will be added to pad the output.
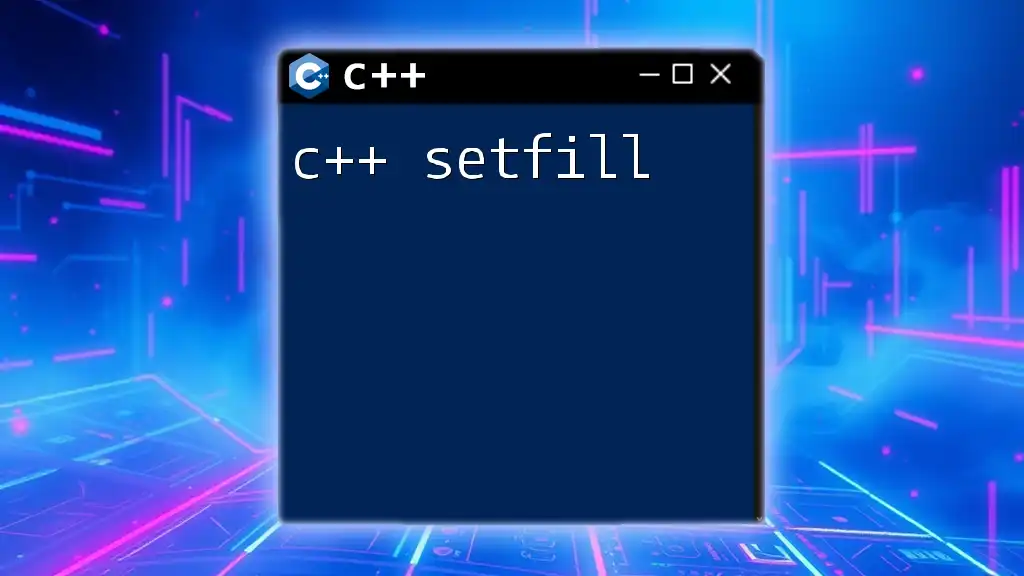
How Does `setw` Work in C++?
The `setw` function's syntax is simple:
std::setw(int width)
Here, the `width` parameter specifies the total number of characters to be used for the next output. This includes both the characters that are meant to be displayed as well as any padding needed.
To use `setw`, you must include the `<iomanip>` header in your program. This inclusion allows access to various formatting functions, including `setw`.
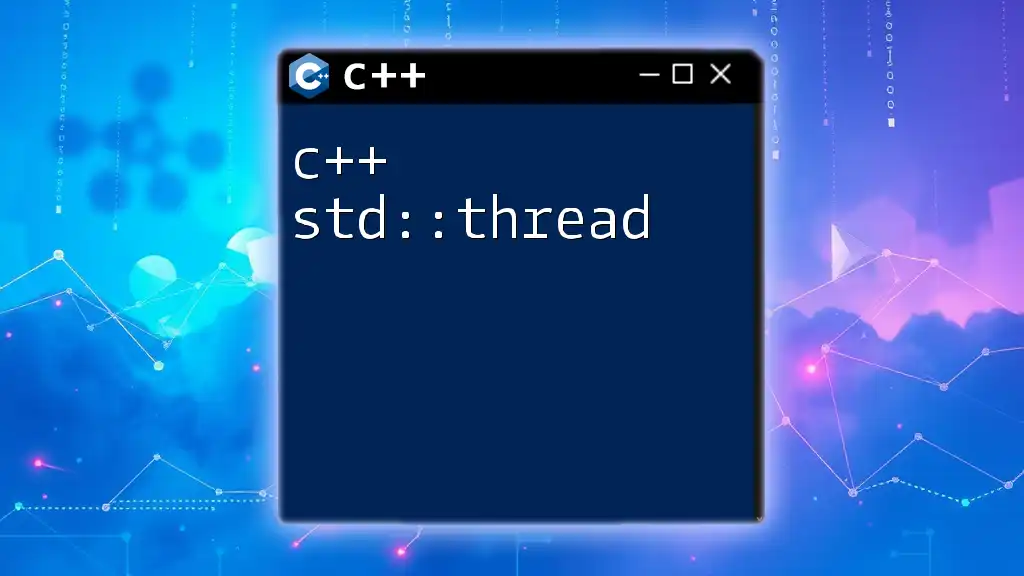
The Functionality of `setw` in C++
Basic Usage of `setw`
A common use case for `setw` is formatting strings in a structured manner, making the output more readable. Consider the following C++ code snippet:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setw(10) << "Hello" << std::endl;
std::cout << std::setw(10) << "World" << std::endl;
return 0;
}
In this example, each word, "Hello" and "World," will be outputted in a space of 10 characters. If the word has fewer characters, the output will be padded with spaces on the left, creating a neat column.
Combining `setw` with Other Formatting Functions
One of the powerful aspects of `setw` is its ability to work with other formatting functions like `setfill`, `setprecision`, etc. For instance, combining `setw` with `setfill` allows you to specify which character to use for padding.
Here’s an example:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setfill('*') << std::setw(10) << 42 << std::endl;
return 0;
}
This code utilizes `setfill` to replace the default space padding with asterisks. Thus, the output will be `*******42`, showcasing how `setw` can modify the presentation of numerical data.
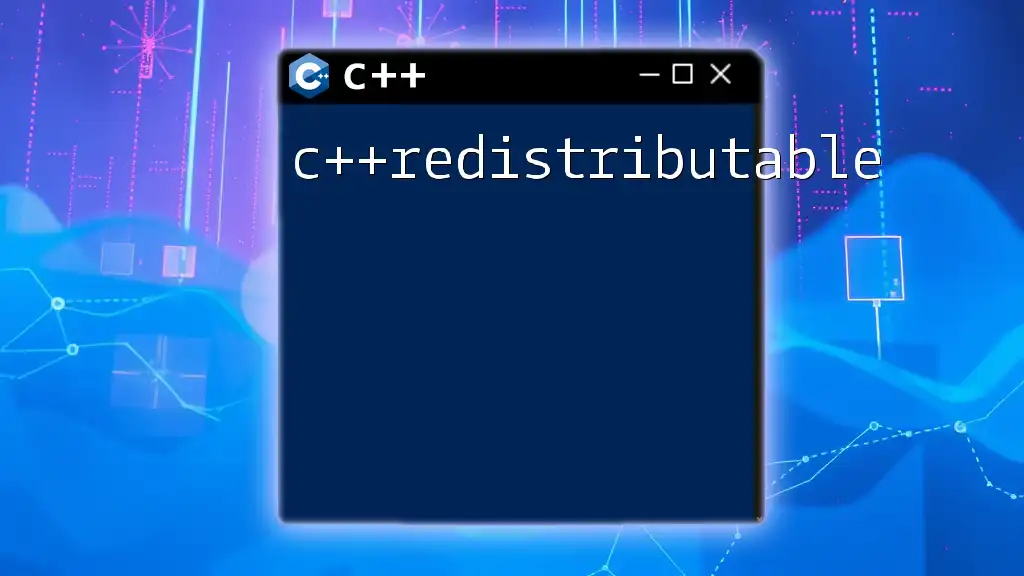
Why Use `setw` in C++?
Enhancing Readability
Output clarity is vital, especially when displaying data in a console application. The `setw` function can significantly enhance readability. It allows programmers to outline data in a structured table format.
For example, consider the following structured output:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setw(5) << "ID" << std::setw(15) << "Name" << std::setw(10) << "Score" << std::endl;
std::cout << std::setw(5) << 1 << std::setw(15) << "Alice" << std::setw(10) << 95 << std::endl;
std::cout << std::setw(5) << 2 << std::setw(15) << "Bob" << std::setw(10) << 90 << std::endl;
return 0;
}
In this case, the output aligns the ID, Name, and Score data into clear columns, making it much easier for users to read and interpret the information.
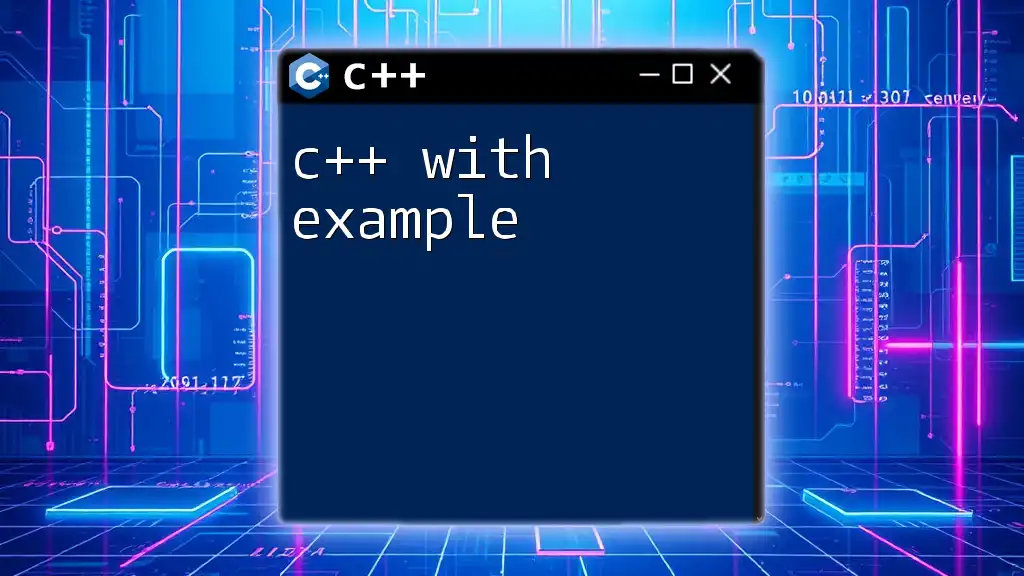
Common Mistakes with `setw`
When dealing with `setw`, beginners often encounter several common pitfalls. A typical oversight is expecting `setw` to persist across different lines or outputs. However, `setw` only applies to the next output operation. Here’s an example:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setw(10) << "Hello" << std::setw(10) << "Goodbye" << std::endl; // Outputs different width
return 0;
}
In this code, `Hello` and `Goodbye` will be padded separately, which may not align as expected. As a best practice, it's crucial to always use `setw` for each output operation that requires it.
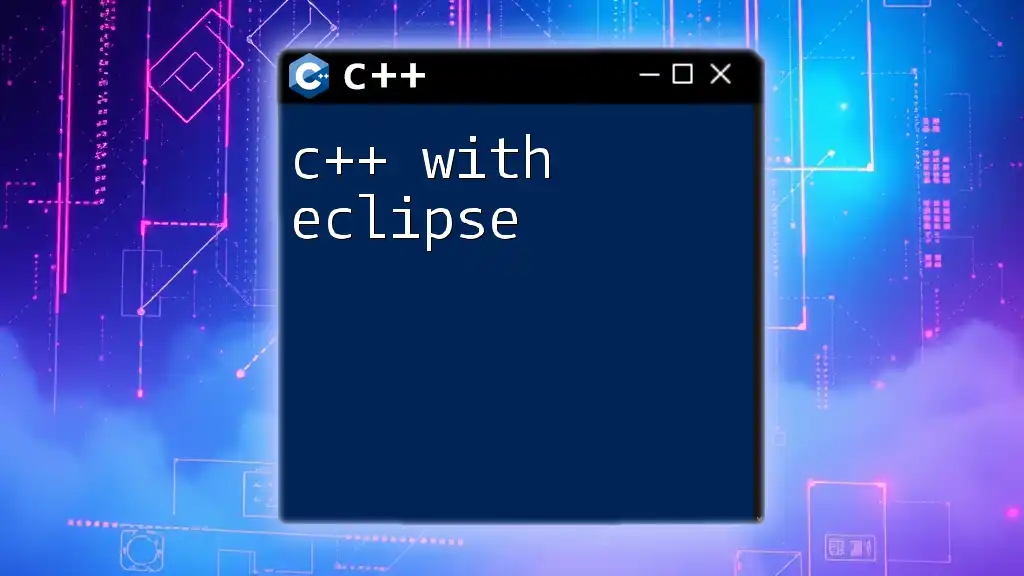
Advanced Usage of `setw` in C++
Dynamic Width Setting
For more complex applications, you might want to set the width dynamically. This can be particularly useful when the desired width is determined at runtime.
Here’s an example of how to use a variable for width:
#include <iostream>
#include <iomanip>
int main() {
int width = 15;
std::cout << std::setw(width) << "Dynamically set width" << std::endl;
return 0;
}
This flexibility enables developers to adapt their output formatting based on varying requirements and data characteristics.
Using in Loops and Complex Structures
Another powerful application of `setw` lies in loops, particularly for formatting rows of data in a table-like structure.
Consider the following example:
#include <iostream>
#include <iomanip>
int main() {
for (int i = 1; i <= 5; ++i) {
std::cout << std::setw(5) << i << std::setw(15) << "Item " + std::to_string(i) << std::setw(10) << (i * 10) << std::endl;
}
return 0;
}
This structure allows you to effectively present multi-column output in a neatly formatted table, enhancing data clarity through consistent alignment.
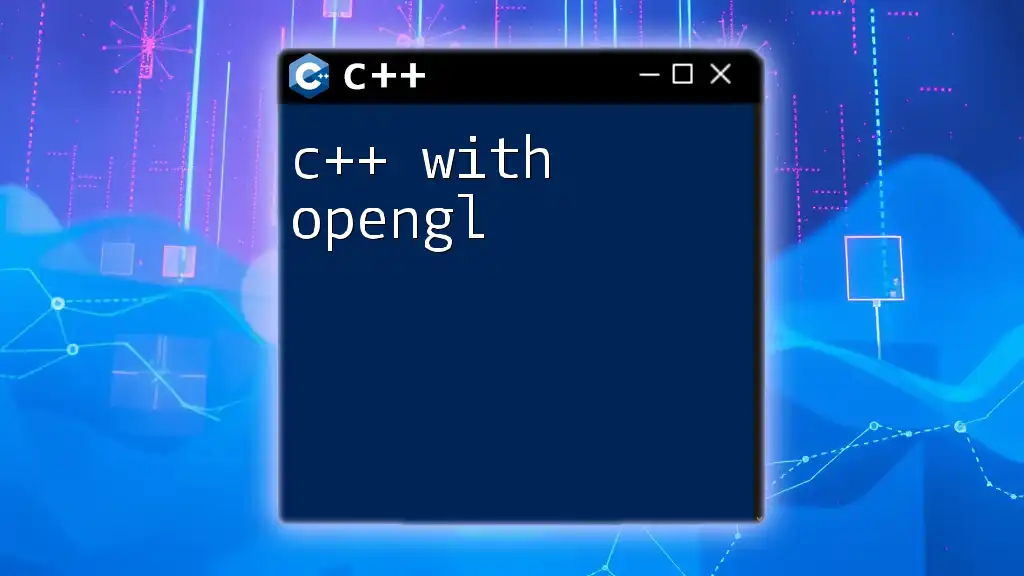
Conclusion
In summary, `setw` is a powerful tool in C++ for controlling the format of output in console applications. Understanding how to properly use `setw` allows developers to enhance readability and presentation of data effectively. Whether you are creating simple outputs or complex formatted reports, mastering `setw` can significantly improve your programming output's quality.
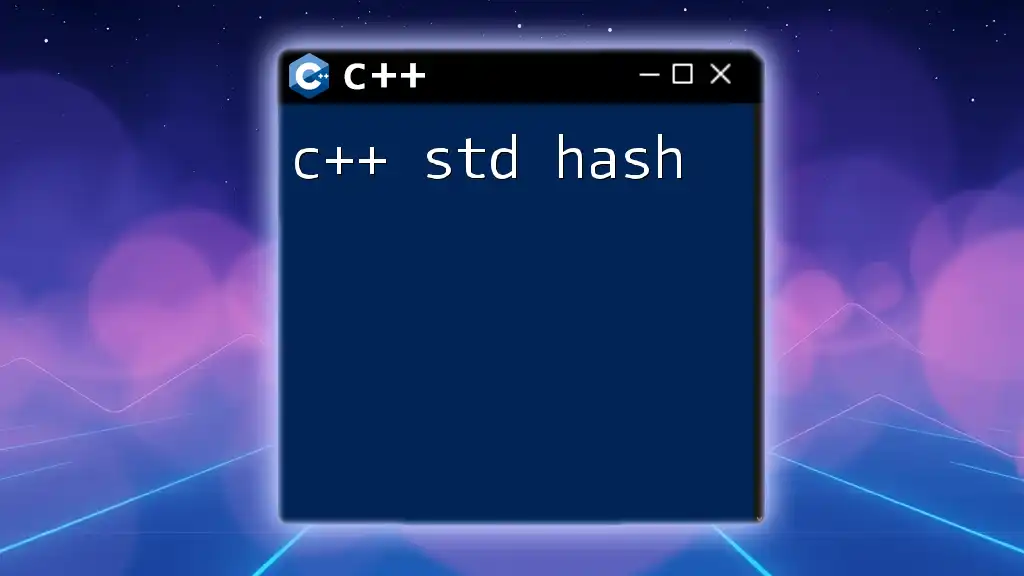
Call to Action
Now that you have a comprehensive understanding of `c++ setwidth`, we encourage you to practice and experiment with it. Feel free to share your experiences and examples with `setw` in C++! For more concise C++ tutorials, make sure to subscribe to our newsletter or follow us for the latest updates.