The C++ set difference can be obtained by using the `std::set_difference` algorithm from the Standard Library, which computes the difference between two sorted ranges, producing a sorted output of elements that are in the first range but not in the second.
#include <iostream>
#include <set>
#include <algorithm>
#include <iterator>
int main() {
std::set<int> setA = {1, 2, 3, 4, 5};
std::set<int> setB = {3, 4, 5, 6, 7};
std::set<int> result;
std::set_difference(setA.begin(), setA.end(), setB.begin(), setB.end(),
std::inserter(result, result.begin()));
for (int i : result) {
std::cout << i << ' ';
}
return 0;
}
What is Set Difference?
Set difference refers to the operation where one set removes the elements that are present in another set. Mathematically, it can be represented as \( A - B \), where \( A \) is the original set and \( B \) is the subset to be removed. This operation is widely used in various programming scenarios, such as filtering data, managing collections, and performing comparative analyses.
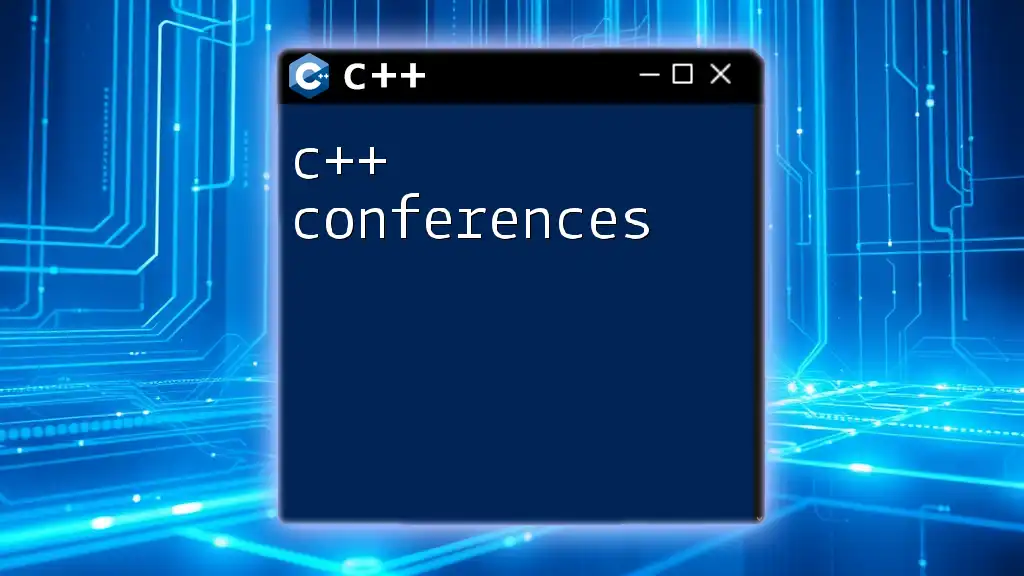
Applications of Set Difference
Understanding set difference can help in numerous programming applications. For instance, imagine you have a collection of users who have signed up for a service, and you want to find users who haven't opted for a particular feature; you could simply perform a set difference operation between the two groups. This capability is particularly useful in data analysis, where cleaning datasets and making comparisons is essential.
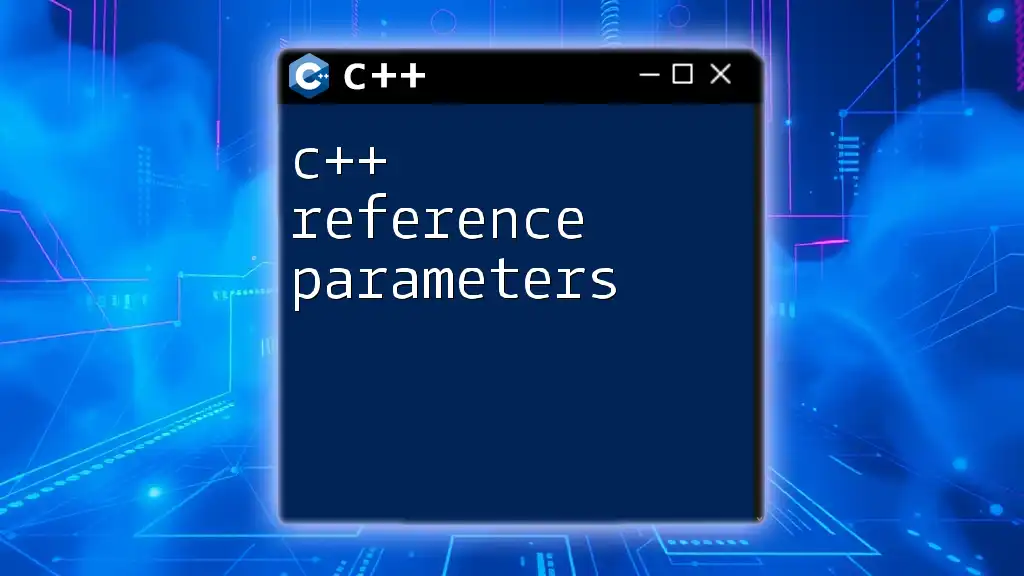
Understanding Sets in C++
Overview of Sets
In C++, a set is a data structure that stores unique elements in a specific order. This intrinsic property of sets makes them ideal for mathematical operations like intersection and difference. Unlike vectors or lists, sets automatically manage the uniqueness of elements and sort them, ensuring that no duplicates are stored.
Key Properties of Sets
- Unique Elements: Each element in a set is unique, meaning duplicates are automatically ignored.
- Automatic Sorting: Sets are stored in a balanced binary tree, allowing for automatic sorting of elements as they are added.
- Insertion and Deletion Complexities: The average time complexity for inserting or deleting an element in a set is \( O(\log n) \), thanks to the underlying tree structure.
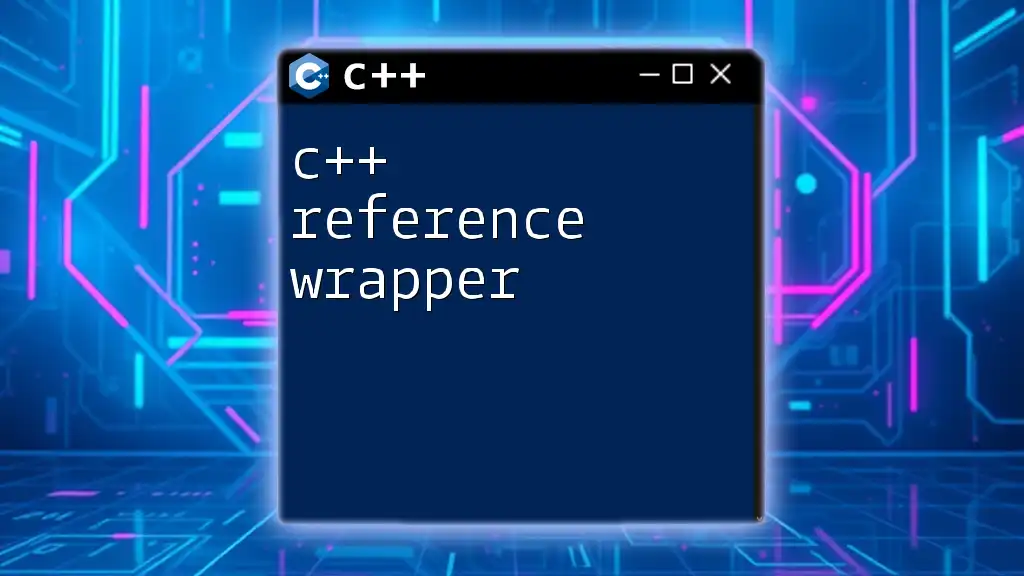
The C++ Standard Library and Sets
Introduction to `<set>` Header
To use sets in C++, you need to include the `<set>` header file. This header file provides the necessary definitions and functions for set operations, available since C++11.
Creating a Set
Declaring a set in C++ is straightforward. Here’s a simple example of how to create a set and initialize it with some values:
#include <set>
#include <iostream>
int main() {
std::set<int> mySet = {1, 2, 3, 4, 5};
return 0;
}
In this code snippet, we have created a set called `mySet` initialized with integers from 1 to 5.
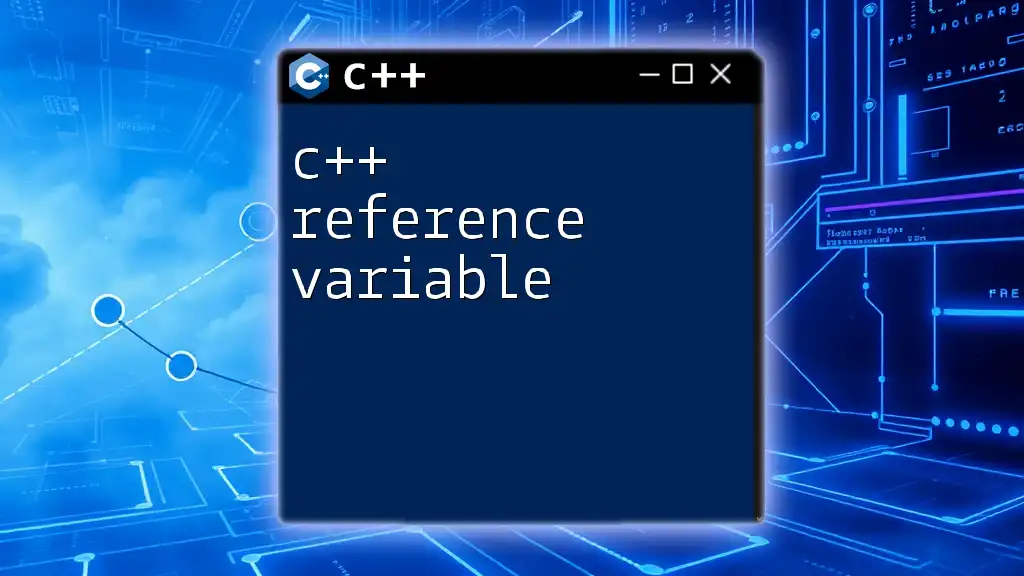
Implementing Set Difference
The Concept of Set Difference
As mentioned earlier, set difference involves removing the elements of one set from another. In practice, this means you can start with a comprehensive set and trim it down based on the elements of another set.
Using STL Algorithms for Set Difference
C++ provides a built-in algorithm called `std::set_difference`, allowing you to compute the difference between two sets easily. This algorithm takes two sorted ranges and outputs the difference into a destination range.
Example of Set Difference
Here’s how you can implement set difference using `std::set_difference`:
#include <set>
#include <algorithm>
#include <iterator>
#include <iostream>
int main() {
std::set<int> setA = {1, 2, 3, 4, 5};
std::set<int> setB = {3, 4};
std::set<int> result;
std::set_difference(setA.begin(), setA.end(),
setB.begin(), setB.end(),
std::inserter(result, result.begin()));
std::cout << "Set Difference (A - B): ";
for (int i : result) {
std::cout << i << " ";
}
return 0;
}
Explanation of the Code
In this example, we declare two sets, `setA` and `setB`. We utilize `std::set_difference` to find the elements in `setA` that are not in `setB`. The resultant set, `result`, is then populated with these elements. The `std::inserter` function allows us to insert the resulting elements into `result`. Finally, we print the elements of `result` to the console.
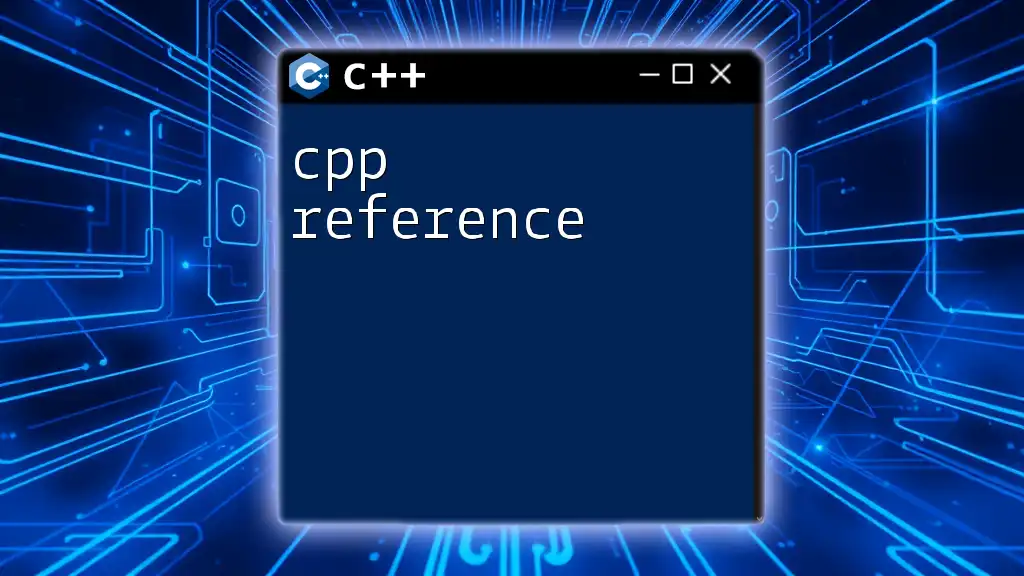
Practical Use Cases of Set Difference
Data Filtering
Set difference is particularly useful in scenarios like filtering data sets. For instance, if you are working with customer data and want to create a list of customers who have not made recent purchases compared to those who have, you could easily apply the set difference.
Comparing Two Collections
In programming, it's often necessary to compare two collections for various reasons, such as identifying common and different elements. Set difference serves as a straightforward method to determine which items are unique to one collection.
Example: Comparing Two Sets
Consider this function that compares two sets:
#include <set>
#include <iostream>
void compareSet(std::set<int> setA, std::set<int> setB) {
std::set<int> diff = setA;
std::set_difference(diff.begin(), diff.end(),
setB.begin(), setB.end(),
diff.begin());
std::cout << "Difference: ";
for (int i : diff) {
std::cout << i << " ";
}
std::cout << std::endl;
}
This function accepts two sets, computes the set difference, and prints the unique elements of `setA` that do not exist in `setB`.
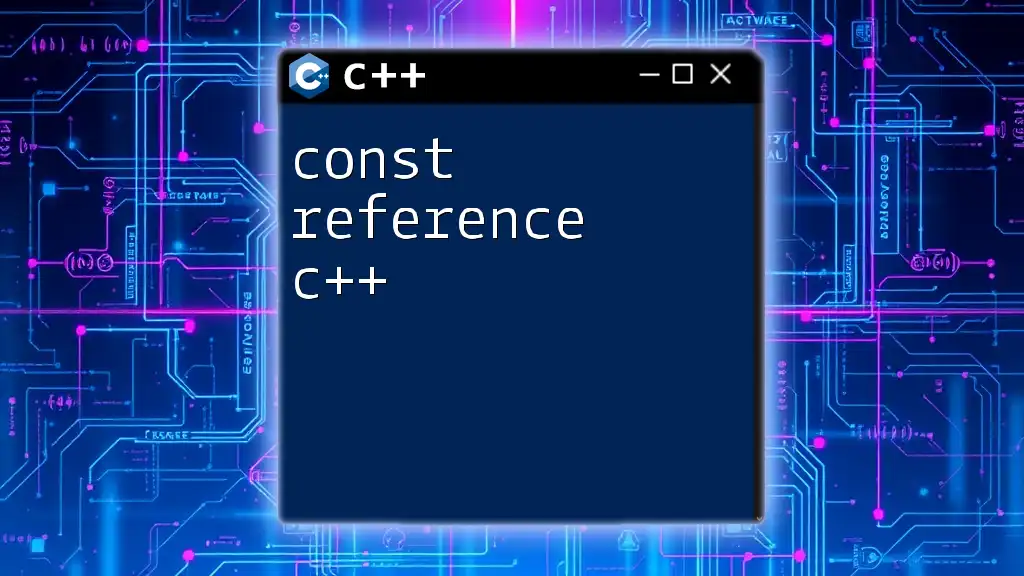
Performance Considerations
Time Complexity Analysis
The time complexity of performing set operations in C++ is generally logarithmic, \( O(\log n) \), for insertions and deletions. The nature of the underlying balanced tree structure contributes to this efficiency. However, when using algorithms like `std::set_difference`, the complexity can be approximated as \( O(n + m) \), where \( n \) and \( m \) are the sizes of the two sets involved.
Choosing the Right Data Structure for Set Operations
When working with sets, it’s important to choose the appropriate data structure based on your needs. A `std::set` is excellent when order and uniqueness are required, but if performance is a priority and ordering is not necessary, consider using `std::unordered_set`. The latter provides average constant time complexity for search, insertion, and deletion.
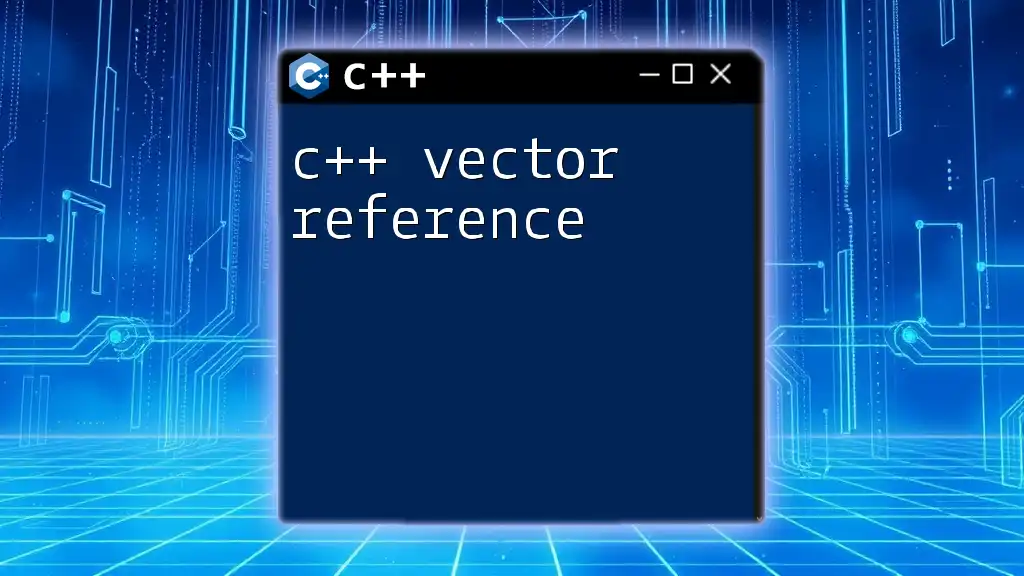
Conclusion
In conclusion, understanding C++ set difference is crucial for anyone working with collections or datasets in C++. This operation allows programmers to efficiently manage and filter data, compare collections, and perform various mathematical operations.
Further Reading and Resources
For those looking to dive deeper into C++ set operations, consider exploring official C++ documentation on sets and the `<algorithm>` header, which introduces a variety of useful algorithms for managing collections in a robust way.
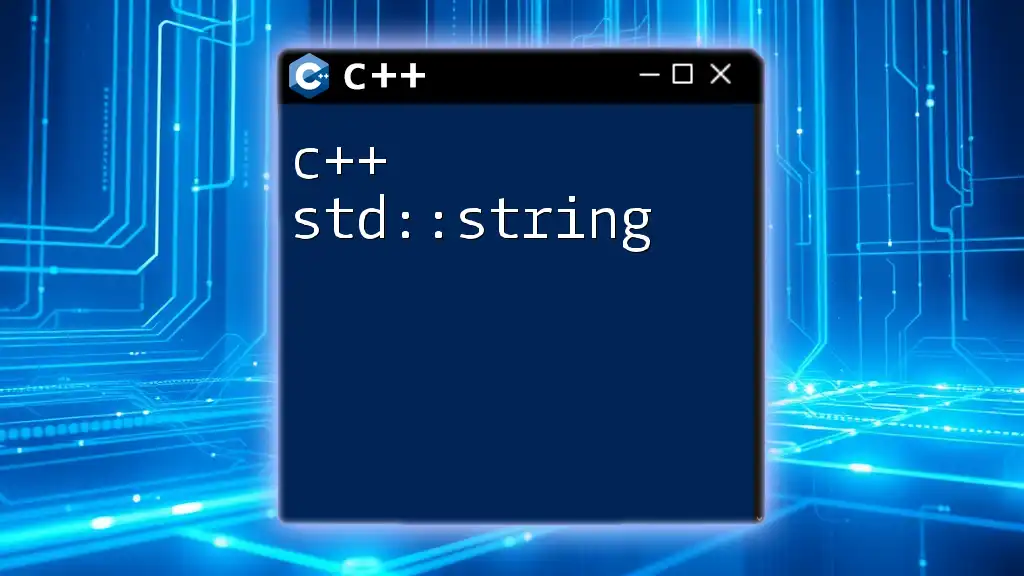
Call to Action
If you have any questions or personal experiences with C++ set difference that you'd like to share, please leave a comment below. Subscribe for more lessons and insights into C++ operations that can enhance your programming skills!