C++ with OpenGL allows developers to create high-performance graphics applications using C++'s powerful features combined with OpenGL's rendering capabilities.
Here's a simple C++ code snippet to initialize a window using OpenGL and the GLFW library:
#include <GLFW/glfw3.h>
int main() {
if (!glfwInit())
return -1;
GLFWwindow* window = glfwCreateWindow(640, 480, "Hello OpenGL", NULL, NULL);
if (!window) {
glfwTerminate();
return -1;
}
glfwMakeContextCurrent(window);
while (!glfwWindowShouldClose(window)) {
glClear(GL_COLOR_BUFFER_BIT);
// Render OpenGL here
glfwSwapBuffers(window);
glfwPollEvents();
}
glfwDestroyWindow(window);
glfwTerminate();
return 0;
}
What is C++?
C++ is a powerful general-purpose programming language that is widely used in various domains, particularly in systems software and graphics programming. Renowned for its performance and efficiency, C++ provides fine-grained control over system resources and memory management. Its object-oriented features also make it a favorite for developing large-scale applications and games.
In the realm of graphics programming, C++ stands out due to its speed and the ability to manipulate hardware resources directly. This is particularly beneficial for applications needing high-performance graphics rendering, such as video games and simulation software.
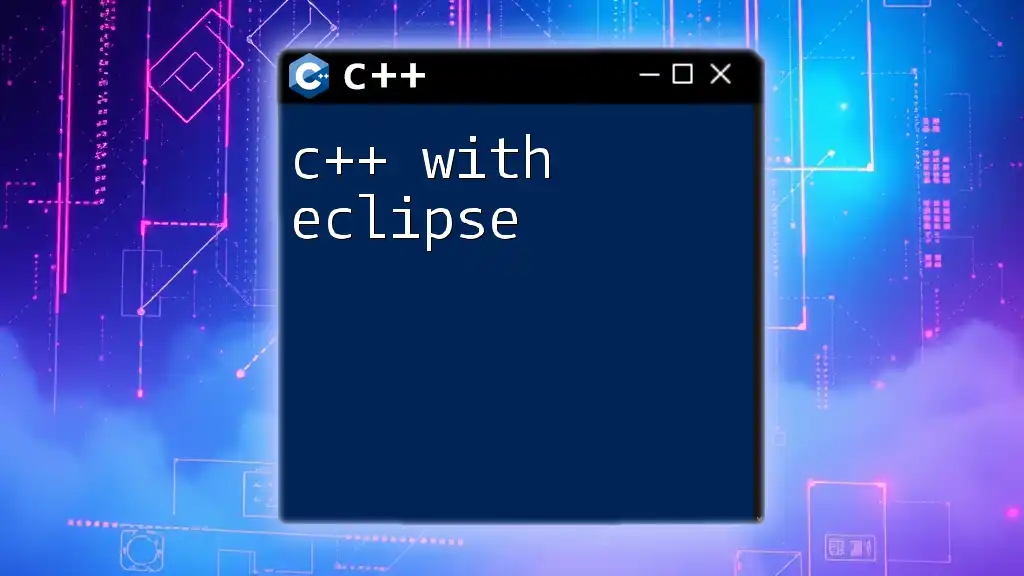
What is OpenGL?
OpenGL (Open Graphics Library) is a cross-language, cross-platform API used for rendering 2D and 3D vector graphics. It provides developers with a powerful, flexible interface to efficiently render graphics for a wide variety of applications, from games to immersive simulations.
Originally developed in the early 1990s, OpenGL has undergone numerous updates and revisions, incorporating new features and enhancements designed to keep pace with the evolving graphics hardware landscape. Some of its key features include:
- Cross-platform compatibility: OpenGL works on Windows, macOS, and Linux.
- Support for modern graphics hardware: OpenGL provides low-level access to the graphics hardware, enabling fine-tuned control over rendering processes.
- Extensibility: Developers can use extensions to add new features that are not part of the core API.
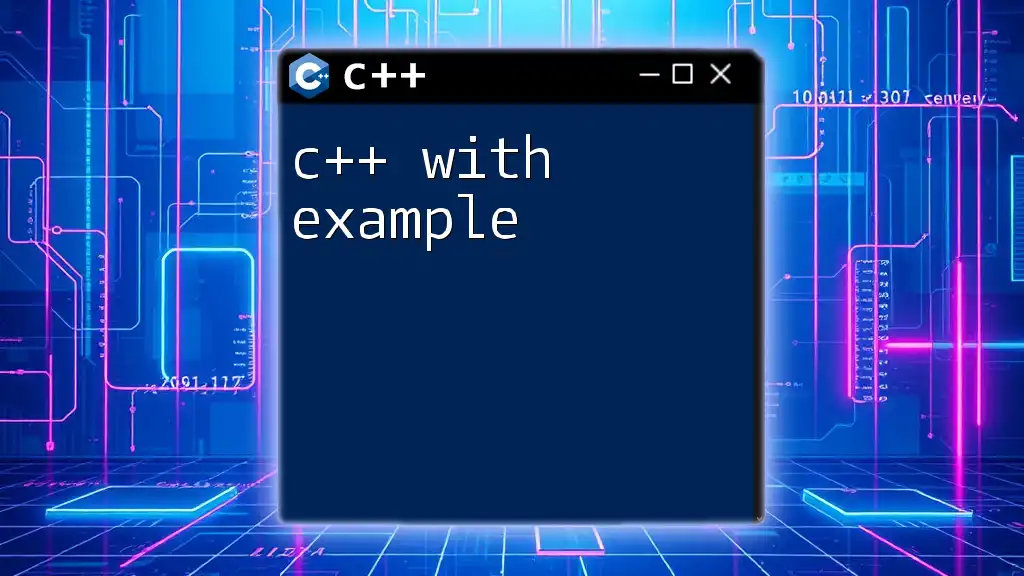
Why Use C++ with OpenGL?
Using C++ with OpenGL makes sense for several reasons:
- Performance: C++ is compiled directly to machine code, and its close-to-the-metal architecture allows developers to write performance-critical code, benefiting real-time graphics rendering.
- Control: C++ offers developers the fine control needed to manage graphics pipeline states and data. This is crucial when optimizing rendering performance.
- Industry standard: Many game engines and graphical applications, such as Unity and Unreal Engine, use C++ alongside OpenGL (or other graphics APIs). Learning this combination opens doors to career opportunities in many high-tech industries.
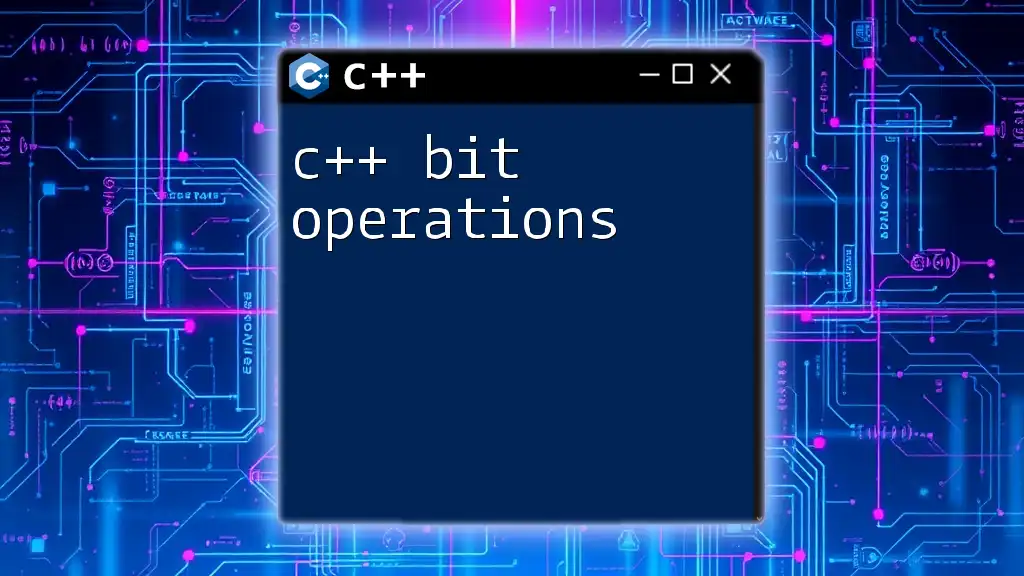
Setting Up Your Environment for C++ and OpenGL
Required Software
Before diving into C++ with OpenGL, you will need necessary software components:
- Compilers: Popular compilers include GCC for Linux and MinGW for Windows, as well as MSVC for Visual Studio users.
- IDEs: Integrated Development Environments like Visual Studio, Code::Blocks, or CLion provide excellent development support for C++.
Installing OpenGL
Installing OpenGL will vary based on your operating system. Generally, it comes pre-installed on most systems, but you will need to install development libraries like GLFW and GLEW for creating OpenGL applications.
- Windows: You can set up OpenGL using libraries available via vcpkg or downloading them directly from their respective sites.
- macOS: OpenGL is usually included, and you can access it through the macOS SDK. Additional libraries can be installed via Homebrew.
- Linux: Use your package manager to install OpenGL libraries (e.g., `sudo apt-get install libgl1-mesa-dev`).
Additional Libraries
To enhance your OpenGL experience in C++, consider integrating the following libraries:
- GLFW: A library for creating windows and handling input.
- GLEW: An extension wrangler library that helps access modern OpenGL features.
- GLM: A mathematics library tailored for graphics programming, offering a wide variety of mathematical functions required for transformations and projections.
Sample Installation Instructions
Here’s a step-by-step installation guide for GLFW and GLEW on Windows:
- Download GLFW from the official website and extract it.
- Open your compiler and configure the include and library paths to point to the GLFW directories.
- For GLEW, download the binaries and include them in your project similarly.
To verify the installation, create a simple C++ application that includes both libraries and run it.
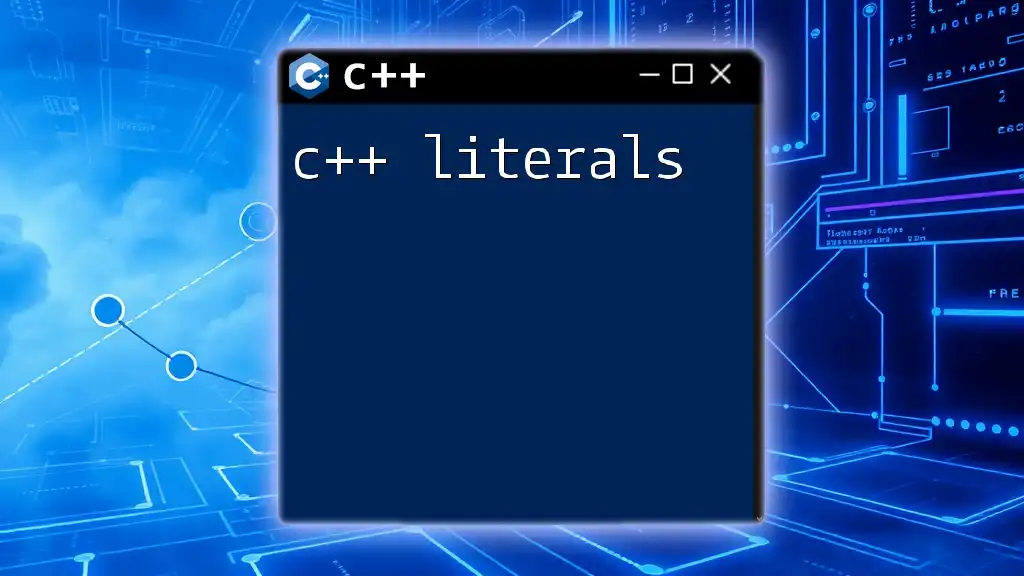
Basics of OpenGL with C++
Understanding the OpenGL Pipeline
To effectively utilize C++ with OpenGL, you must understand the OpenGL rendering pipeline. The pipeline consists of multiple stages, each responsible for processing vertex data and drawing pixels to the screen. The key stages are:
- Vertex Processing: Each vertex is transformed into screen space coordinates using vertex shaders.
- Primitive Assembly: Vertices are combined to form geometric objects like points, lines, or triangles.
- Fragment Processing: In this stage, fragment shaders determine the color and texture of each pixel.
Creating a Simple OpenGL Application
A fundamental step in learning C++ with OpenGL is creating a simple OpenGL application. The following example demonstrates a minimal OpenGL application setup:
#include <GL/glew.h>
#include <GLFW/glfw3.h>
void processInput(GLFWwindow *window) {
if (glfwGetKey(window, GLFW_KEY_ESCAPE) == GLFW_PRESS)
glfwSetWindowShouldClose(window, true);
}
int main() {
// Initialize GLFW
if (!glfwInit())
return -1;
// Create a windowed mode window and its OpenGL context
GLFWwindow *window = glfwCreateWindow(640, 480, "Hello World", NULL, NULL);
if (!window) {
glfwTerminate();
return -1;
}
glfwMakeContextCurrent(window);
glewInit();
// Main loop
while (!glfwWindowShouldClose(window)) {
processInput(window);
glClear(GL_COLOR_BUFFER_BIT);
glfwSwapBuffers(window);
glfwPollEvents();
}
glfwDestroyWindow(window);
glfwTerminate();
return 0;
}
This program initializes a window and sets up a basic loop where you can handle input and refresh the display.
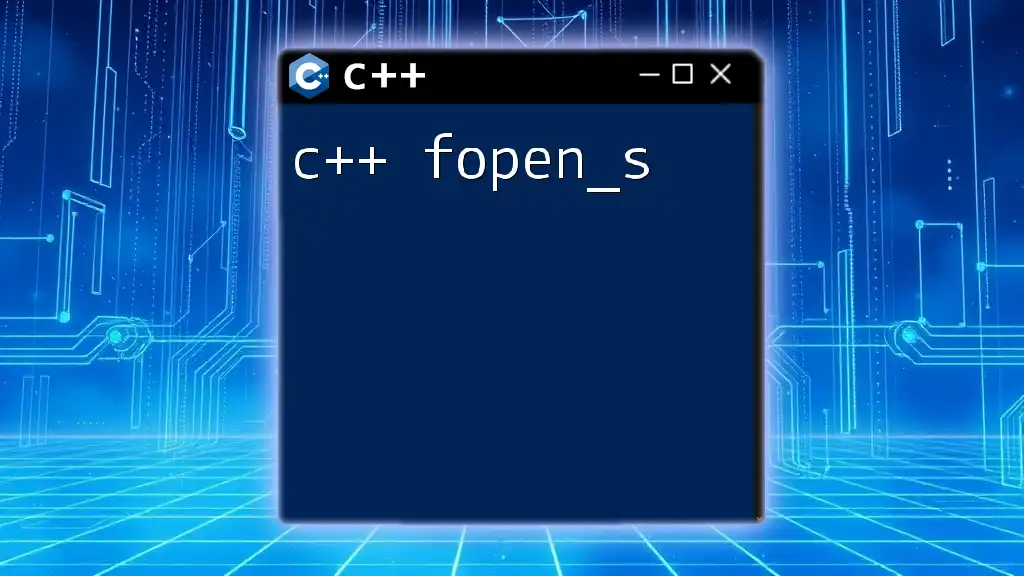
Rendering with OpenGL and C++
Basic Drawing with OpenGL
Once your OpenGL context is set up, the next step is drawing shapes. To begin, you need to handle vertex array objects (VAOs) and vertex buffer objects (VBOs), as shown in the code below:
GLuint VAO, VBO;
glGenVertexArrays(1, &VAO);
glGenBuffers(1, &VBO);
glBindVertexArray(VAO);
glBindBuffer(GL_ARRAY_BUFFER, VBO);
GLfloat vertices[] = {
// Coordinates of the triangle
0.0f, 0.5f, 0.0f,
-0.5f, -0.5f, 0.0f,
0.5f, -0.5f, 0.0f
};
glBufferData(GL_ARRAY_BUFFER, sizeof(vertices), vertices, GL_STATIC_DRAW);
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, 3 * sizeof(GLfloat), (GLvoid*)0);
glEnableVertexAttribArray(0);
glBindBuffer(GL_ARRAY_BUFFER, 0);
glBindVertexArray(0); // Unbind VAO
This example creates a triangle by defining vertex coordinates and uploading them to the GPU.
Texturing and Shading
Textures and shaders are essential for creating visually appealing graphics. Shaders are small programs that run on the GPU, controlling how vertices and pixels are processed.
A basic shader program consists of a vertex shader and a fragment shader. Here is an example of a simple shader:
// Vertex Shader
#version 330 core
layout(location = 0) in vec3 position;
void main() {
gl_Position = vec4(position.x, position.y, position.z, 1.0);
}
// Fragment Shader
#version 330 core
out vec4 color;
void main() {
color = vec4(1.0, 0.5, 0.2, 1.0); // orange color
}
These shaders process vertex positions and set the output color for rendering. Implementing shaders allows you to create more complex effects, such as lighting and material properties.
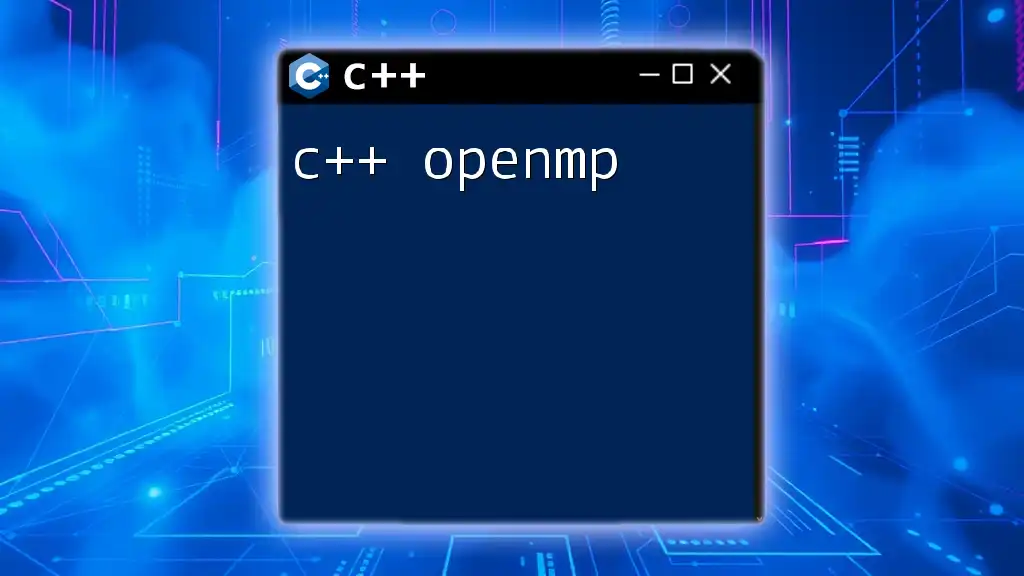
Advanced Concepts in OpenGL and C++
3D Graphics
To create a richer graphical experience, learning about 3D graphics is essential. Transformations such as translation, rotation, and scaling are applied to objects using matrices before rendering them. This is a critical concept in C++ with OpenGL, as it allows for the simulation of depth and perspective in the rendered scenes.
Lighting and Material Properties
In addition to basic drawing, incorporating lighting can significantly enhance visual fidelity. Various lighting models, like Phong and Blinn-Phong, simulate how light interacts with surfaces. By implementing these models, you can create realistic light reflections and shadows, making your graphics more immersive.
// Example of a fragment shader with Phong lighting
#version 330 core
out vec4 FragColor;
in vec3 Normal;
in vec3 FragPos;
uniform vec3 lightPos;
uniform vec3 viewPos;
uniform vec3 lightColor;
void main() {
// Ambient
float ambientStrength = 0.1;
vec3 ambient = ambientStrength * lightColor;
// Diffuse
vec3 norm = normalize(Normal);
vec3 lightDir = normalize(lightPos - FragPos);
float diff = max(dot(norm, lightDir), 0.0);
vec3 diffuse = diff * lightColor;
// Specular
float specularStrength = 0.5;
vec3 viewDir = normalize(viewPos - FragPos);
vec3 reflectDir = reflect(-lightDir, norm);
float spec = pow(max(dot(viewDir, reflectDir), 0.0), 32);
vec3 specular = specularStrength * spec * lightColor;
vec3 result = (ambient + diffuse + specular);
FragColor = vec4(result, 1.0);
}
Advanced Rendering Techniques
Once you have a grasp of the basics, consider learning advanced techniques like framebuffers. Framebuffers allow you to render to textures, enabling effects like shadow mapping and post-processing. Utilizing compute shaders can also enhance rendering efficiency by handling complex calculations in parallel on the GPU.
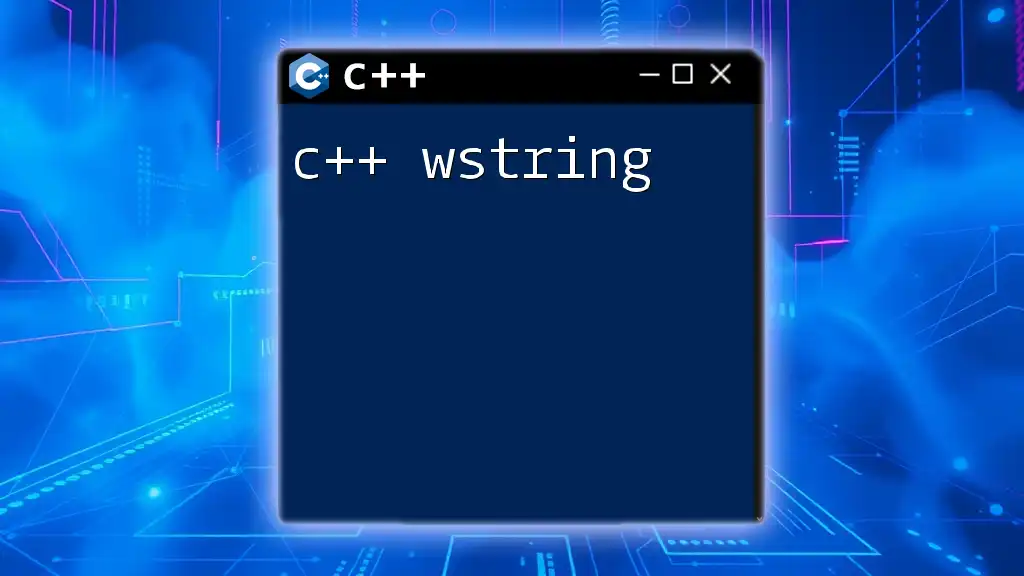
Conclusion
In summary, mastering C++ with OpenGL enables developers to create high-performance graphics applications. By understanding the intricacies of the OpenGL pipeline, setting up the development environment, and implementing basic to advanced rendering techniques, you build a solid foundation for further exploration in the graphics programming domain.
Additional Resources
To continue expanding your knowledge, consider exploring the following resources:
- Books: "OpenGL Programming Guide" and "Learn OpenGL."
- Online tutorials: Websites like LearnOpenGL.com provide excellent step-by-step guides.
- Community forums: Engage with communities on platforms such as Stack Overflow or Reddit.
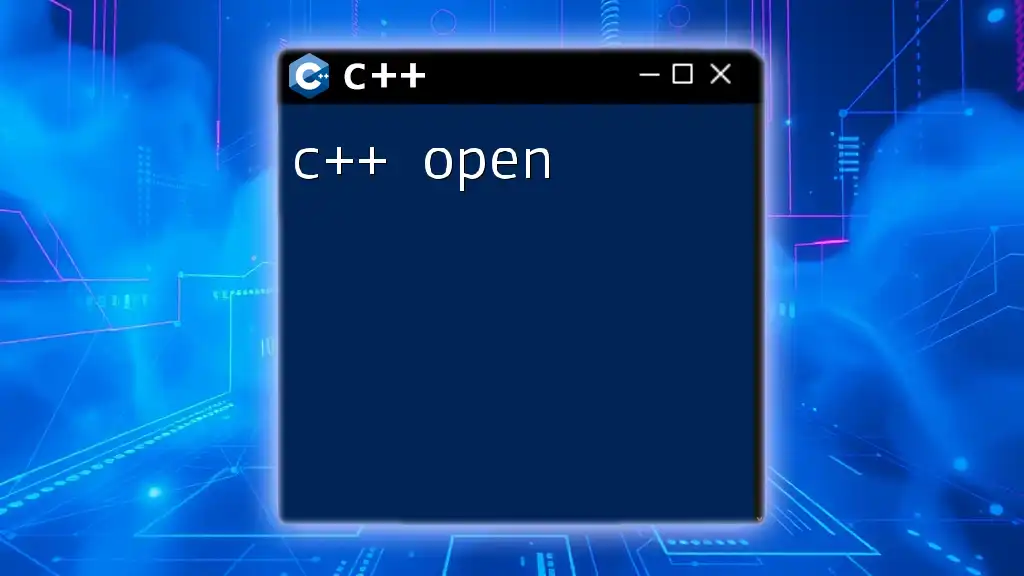
Call to Action
Get started on your journey today! Dive deep into C++ with OpenGL to create captivating graphics applications. Whether you're a beginner or looking to refine your skills, numerous resources are available to guide you on this exciting path. Links to courses and communities are just a click away!