A C++ bitfield is a way to use a specific number of bits within a data structure to efficiently store multiple boolean or integer values, allowing for more compact data representation.
struct Flags {
unsigned int flag1 : 1; // 1 bit for flag1
unsigned int flag2 : 1; // 1 bit for flag2
unsigned int flag3 : 3; // 3 bits for flag3
};
Introduction to Bitfields in C++
Definition of Bitfields: A "bitfield" in C++ is a way to pack data into a smaller space, which allows you to use a specified number of bits for a particular field in a structure or class. This is particularly useful when you need to save memory or manage flags in a compact way.
Applications of Bitfields: Bitfields have various applications, including hardware manipulation, memory management, and data communication. They’re often used in embedded programming or protocols where every bit matters.
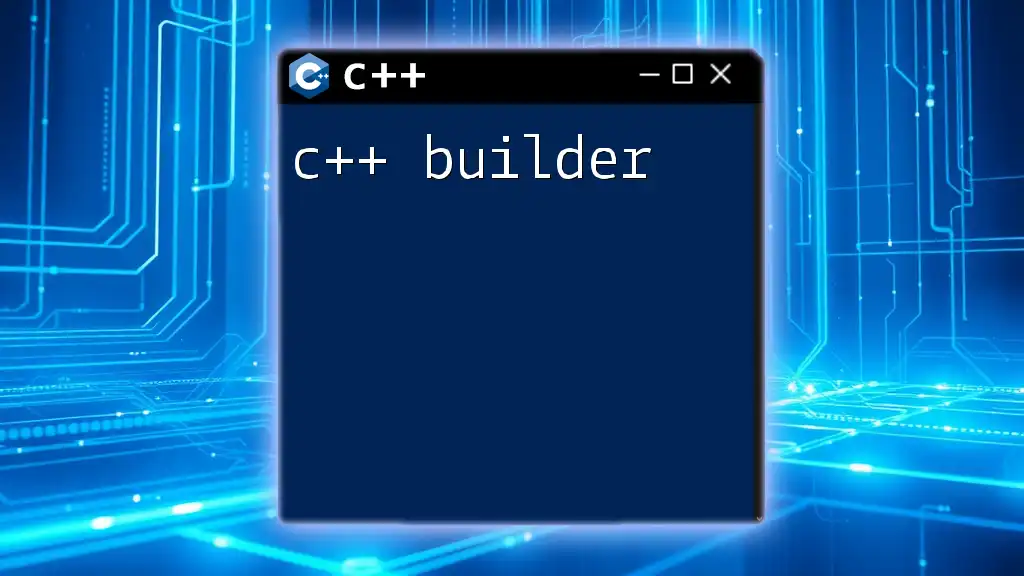
The Basics of C++ Bitfields
What are Bitfields?
Understanding bits is fundamental to grasping the concept of bitfields. A bit is the smallest unit of data in a computer, representing a state of either 0 or 1. Bitfields leverage these bits to represent multiple smaller values within a single data type.
Structure of Bitfields
The syntax for declaring a bitfield is straightforward. You define a struct or class, and within it, you specify the type and the number of bits for each field. Here’s a basic example:
struct Example {
unsigned int field1 : 3; // Uses 3 bits for field1
unsigned int field2 : 5; // Uses 5 bits for field2
};
Why Use Bitfields?
Memory Efficiency: One of the primary reasons to use bitfields is to save memory. Traditional data types can consume more space than necessary. By specifying the number of bits each field requires, you optimize memory usage significantly.
Performance Considerations: Bitfields can offer performance improvements by reducing the need for memory allocation and leading to fewer cache misses. However, it's essential to consider that not all operations benefit from this optimization, and in some instances, they may introduce overhead.
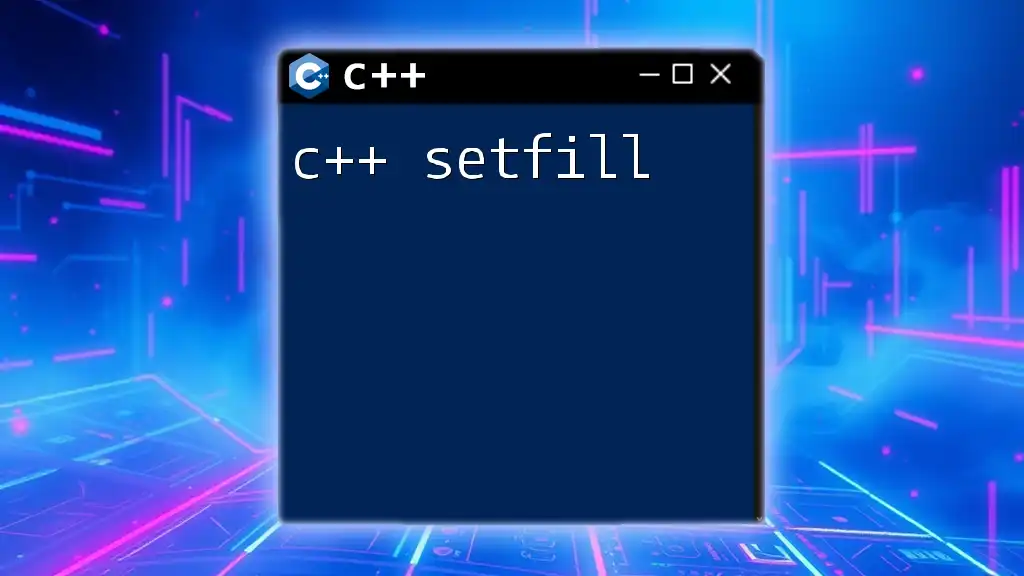
Declaring a Bitfield in C++
Syntax of Bitfields
The syntax for declaring bitfields can vary slightly based on your requirements. The general structure you're looking for is:
struct BitFieldExample {
unsigned int a : 4; // Field a will occupy 4 bits
unsigned int b : 4; // Field b will also occupy 4 bits
};
Data Types for Bitfields
It is important to choose the correct data type when declaring bitfields. The most common types used are `unsigned int` for positive values and `int` for signed integers. When your bitfield only needs to represent non-negative numbers, using `unsigned` is advisable to maximize the range of values.
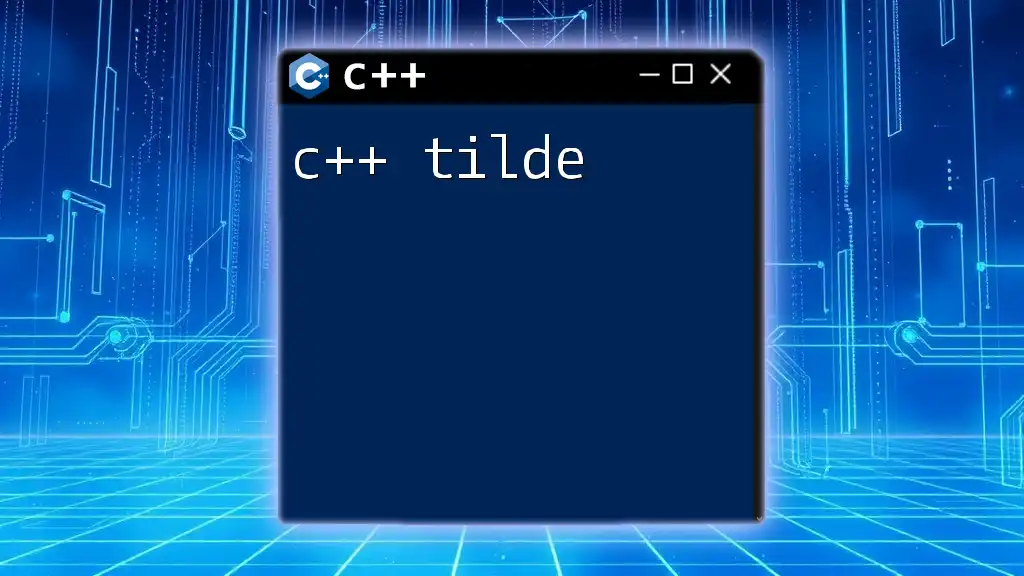
Accessing and Manipulating Bitfields
Initializing Bitfields
Initializing bitfields is seamless. You can define a structure with bitfields and set their initial values during the creation of an instance. For example:
BitFieldExample bf = {5, 6}; // Setting a to 5 and b to 6
Reading Bitfield Values
Accessing the values in a bitfield is as easy as accessing regular struct members. For instance, to print the values of the fields declared above:
#include <iostream>
int main() {
BitFieldExample bf = {5, 6};
std::cout << "Field a: " << bf.a << ", Field b: " << bf.b << std::endl;
return 0;
}
Modifying Bitfield Values
To modify a value in a bitfield, you simply assign a new value, keeping in mind the limits of the bitfield size. For example:
bf.a = 3; // Changing the value of a to 3
Be cautious when assigning values that exceed the bitfield's defined size, as this can lead to unexpected results due to overflow.
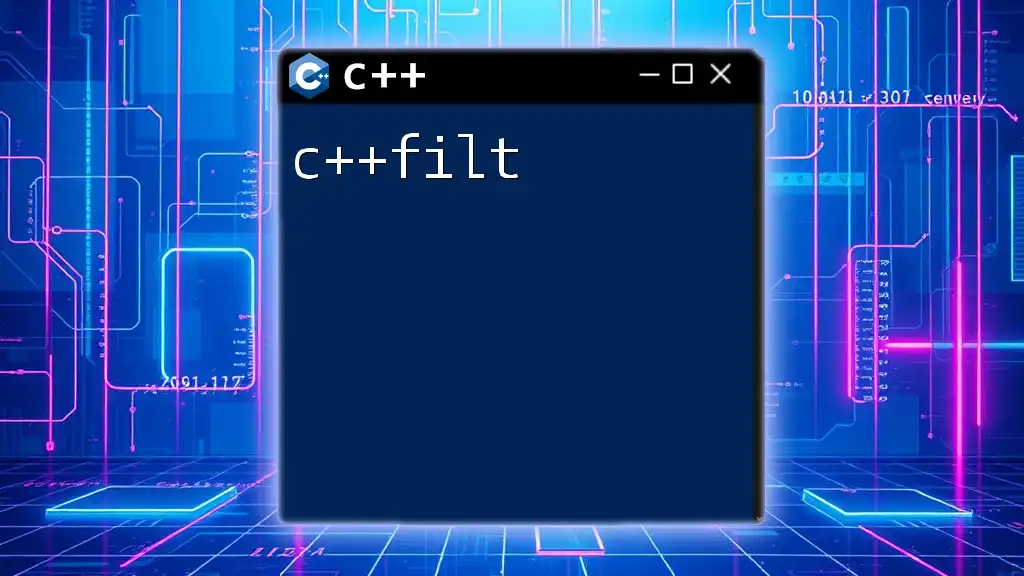
Practical Examples of Bitfields
Use Case: Flags Management
Bitfields are an excellent choice for managing flags. They allow you to pack multiple flag values into a single byte. Here’s how you might define such a structure:
struct Flags {
unsigned int isVisible : 1; // 1 bit for visibility
unsigned int isActive : 1; // 1 bit for active state
unsigned int hasFocus : 1; // 1 bit for focus state
};
In this example, each flag occupies only one bit, enabling efficient representation of the state without wasting space.
Use Case: Packing Data
Bitfields can also be used effectively for packing data. For example:
struct PackedData {
unsigned char a : 2; // 2 bits for a
unsigned char b : 3; // 3 bits for b
unsigned char c : 3; // 3 bits for c
};
This structure can store three values in a single byte (8 bits), making it very efficient for data transmission or storage.
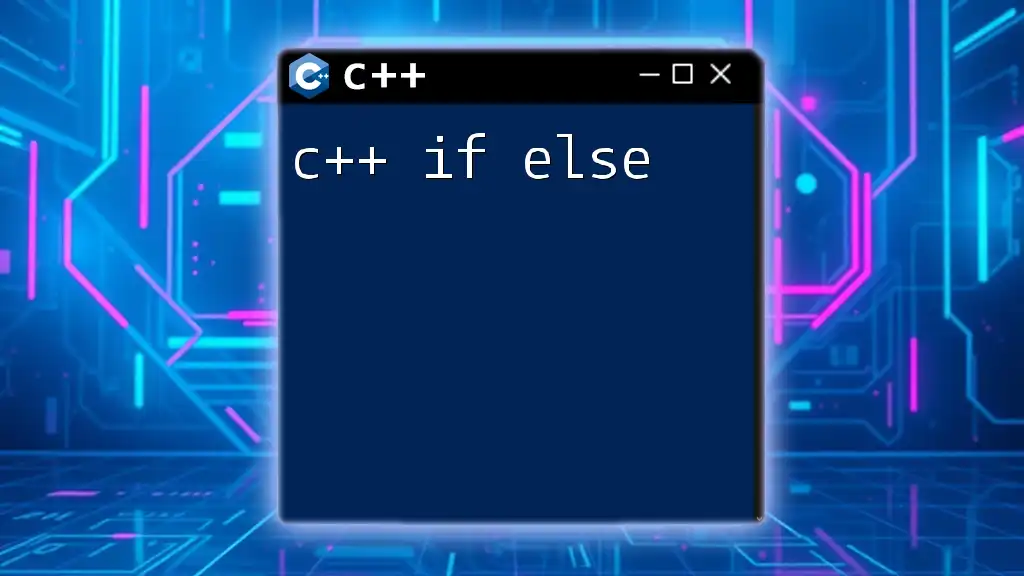
Limitations and Best Practices
Limitations of Bitfields
Using bitfields comes with certain limitations. The most significant issues arise from the fact that their layout can be hardware dependent. The arrangement of bits in memory is not guaranteed between different architectures or compilers, meaning code that works on one platform might fail on another.
Portability Issues: This non-portability can lead to significant challenges when developing cross-platform applications. It’s crucial to keep this in mind when leveraging bitfields in your C++ coding practices.
Best Practices for Using Bitfields
Guidelines for Implementation
When implementing bitfields, adhere to best practices for clarity and maintainability. Include comments to clarify the purpose of each bitfield and its range of values. Avoid using too many bitfields in one structure to keep things manageable.
Common Mistakes to Avoid
Several common pitfalls can occur, such as exceeding the defined bit limits or relying on unspecified behavior across different compilers. Being aware of these issues will help prevent bugs and improve the reliability of your code.
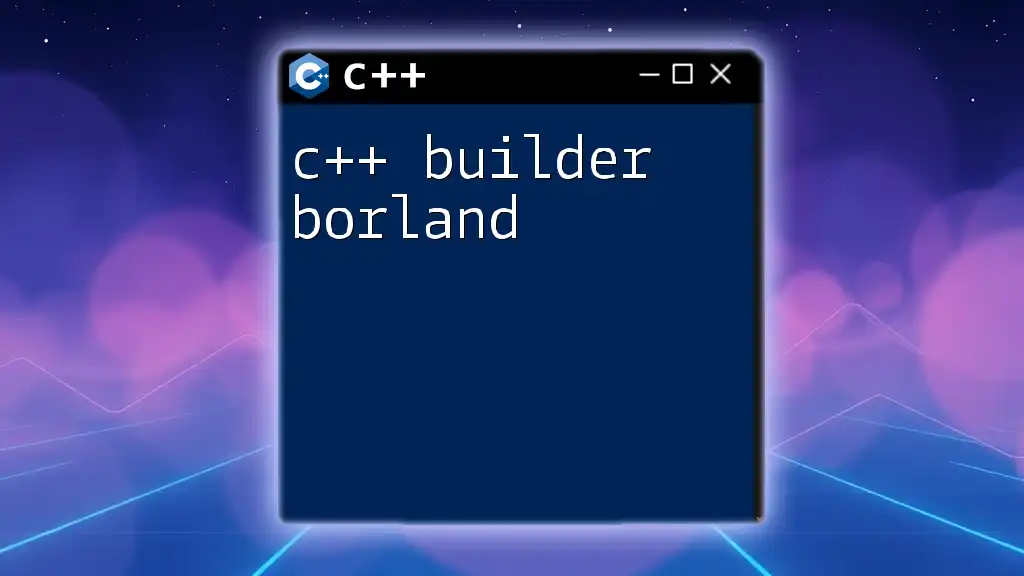
Conclusion
In summary, C++ bitfields provide a powerful tool for packing data and managing memory efficiently. With proper understanding and implementation, they can lead to better performance and optimized resource use. However, developers must be mindful of the limitations, particularly concerning portability and potential pitfalls. Utilizing bitfields correctly enhances code efficiency and leads to more streamlined programs. Embrace this feature as you explore the depths of C++ programming!
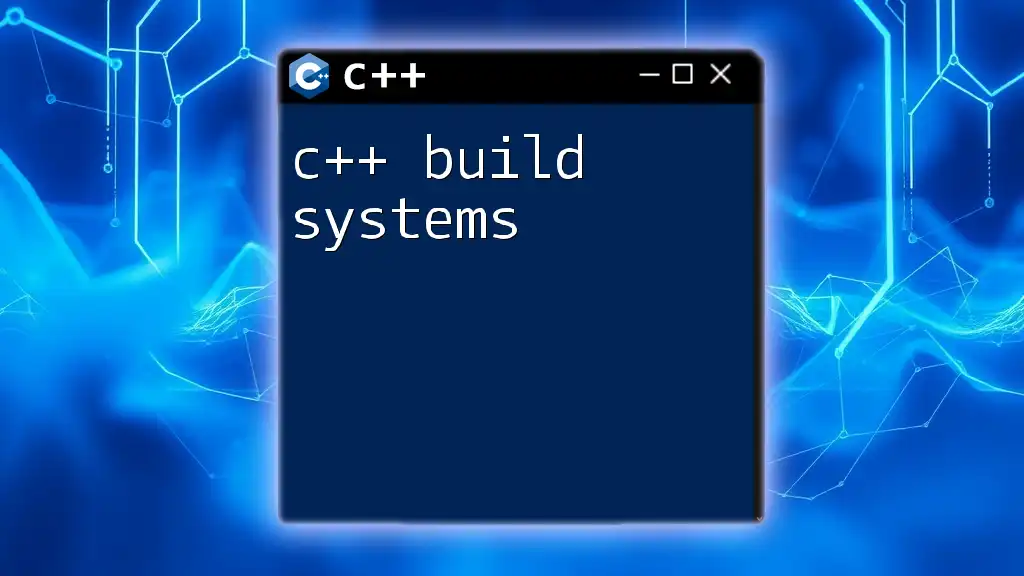
Additional Resources
For further reading on C++ bitfields, consider visiting authoritative sources such as the [C++ documentation](https://en.cppreference.com/w/cpp/language/bitfield), or join discussions on community platforms where experienced developers share their insights.