C++ build systems automate the process of compiling code and managing dependencies to streamline the development workflow.
// Sample Makefile for a simple C++ project
CXX = g++
CXXFLAGS = -Wall -g
all: main
main: main.o utils.o
$(CXX) -o main main.o utils.o
main.o: main.cpp
$(CXX) $(CXXFLAGS) -c main.cpp
utils.o: utils.cpp
$(CXX) $(CXXFLAGS) -c utils.cpp
clean:
rm -f *.o main
Understanding the Build Process
What is a Build?
In the context of software development, a build refers to the process of converting source code files into executable applications. This includes not only compiling the code but also linking it with libraries and resources needed for execution. Understanding this process is key to effectively using C++ build systems.
It is important to note that compiling and building are not synonymous. Compilation is specifically the transformation of source code into object files, whereas building encompasses both compilation and additional steps like linking.
Stages of Building C++
The C++ build process can be broken down into several key stages:
-
Compilation: During this stage, the C++ compiler translates your source code files (.cpp) into object files (.o). Each source file is compiled independently. For example:
g++ -c main.cpp -o main.o
-
Linking: This stage combines all object files into a single executable. If your program uses external libraries, those must also be linked here. Consider the following command:
g++ main.o utils.o -o my_program
-
Testing and Packaging: Once the executable is created, testing ensures that the application works as intended. Packaging might include creating installation scripts or bundles for distribution.
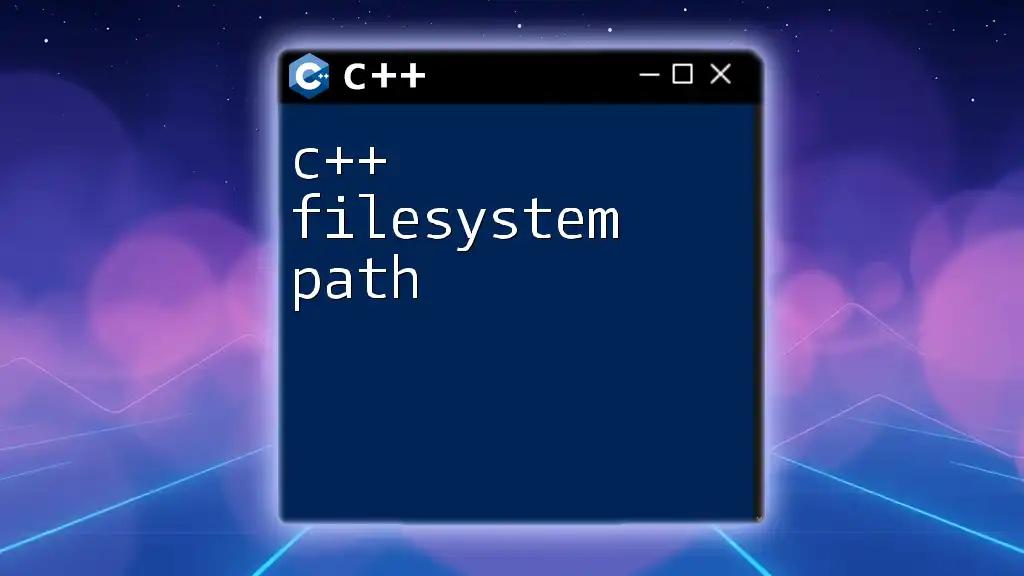
Types of C++ Build Systems
Makefiles
A Makefile is a script used by the `make` utility to manage dependencies and automate the build process. They are particularly powerful for small to medium-sized projects but can become complex for larger systems.
Basic Structure of a Makefile
Here is a simple example of how a Makefile might look:
all: main.o utils.o
g++ main.o utils.o -o my_program
%.o: %.cpp
g++ -c $< -o $@
In this example, when you run `make`, it will first compile `main.o` and `utils.o` from their corresponding source files, and then link them into an executable called `my_program`.
When to Use Makefiles
Makefiles are ideal for smaller projects or when you require fine control over the build process without introducing extra complexity. They work well in Unix-like systems but can be cumbersome in larger projects or cross-platform setups.
CMake
CMake is a versatile and powerful tool that simplifies the process of managing the build process in a variety of environments. It can create Makefiles, Visual Studio project files, or Xcode projects, making it a popular choice for cross-platform development.
CMake Files and Structure
A simple CMake configuration can look like the following:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
add_executable(my_program main.cpp utils.cpp)
With just a few lines, CMake not only identifies the sources but can also manage dependencies efficiently.
Advantages of CMake
CMake's primary advantage over Makefiles is its ability to support multiple platforms and its more straightforward syntax for complex projects. It abstracts away many boilerplate requirements, thus allowing developers to focus on their code rather than on build configurations.
Other Build Systems
Meson
Meson is a newer build system designed for speed and ease of use. It emphasizes simplicity and supports multiple programming languages, including C++.
Example of a Meson Build Configuration
A basic Meson build file would look like this:
project('my_project', 'cpp')
executable('my_program', 'main.cpp', 'utils.cpp')
This example creates an executable called `my_program` from the specified source files and is particularly user-friendly for beginners.
Bazel
Developed by Google, Bazel is a powerful build system that can handle large codebases with ease. It focuses on speed, scalability, and correctness.
Bazel Build File Example (BUILD)
A simple Bazel build configuration might look like:
cc_library(
name = "utils",
srcs = ["utils.cpp"],
hdrs = ["utils.h"],
)
cc_binary(
name = "my_program",
srcs = ["main.cpp"],
deps = [":utils"],
)
This example defines a library and a binary, showcasing Bazel's clear dependency management capabilities.
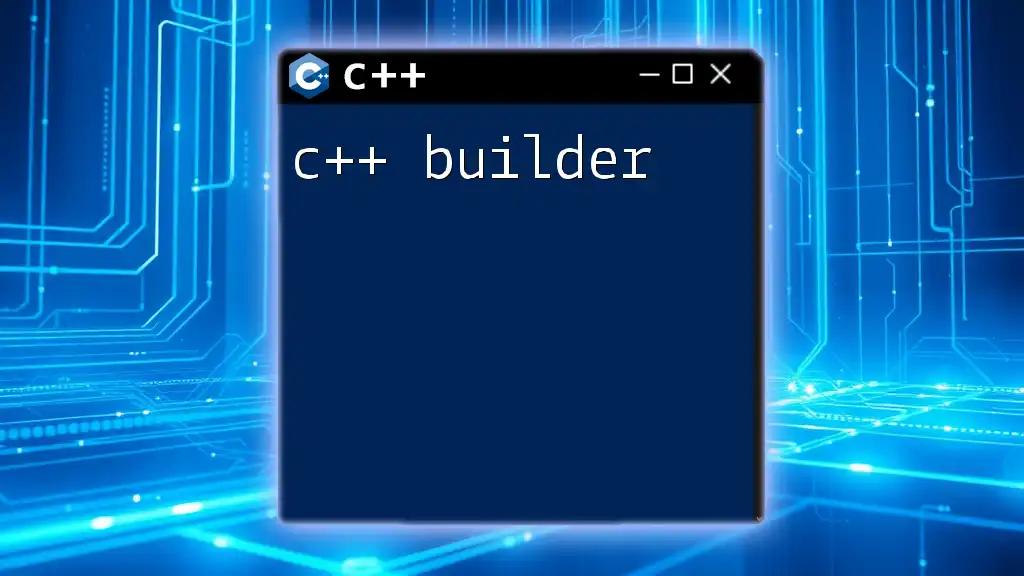
Choosing the Right Build System for Your Project
Factors to Consider
When deciding on a C++ build system, consider these factors:
- Project Size: Smaller projects may benefit from the simplicity of Makefiles, while larger projects might require the scalability of CMake or Bazel.
- Team Size: Larger teams might need a system that simplifies collaboration, such as CMake or Bazel, which can handle dependencies more effectively.
- Platform Requirements: If your application needs to run on multiple platforms, CMake or Meson might be the best options due to their cross-platform capabilities.
Pros and Cons of Popular Build Systems
Each build system has its strengths and weaknesses:
-
Makefiles:
- Pros: Simple for small projects, flexible.
- Cons: Can become unwieldy as project size grows, less suitable for cross-platform development.
-
CMake:
- Pros: Cross-platform support, strong community.
- Cons: Can have a steep learning curve for beginners.
-
Meson:
- Pros: Easy to set up and use, emphasizes speed.
- Cons: Less mainstream; smaller community compared to CMake.
-
Bazel:
- Pros: Excellent for large projects, offers scalability.
- Cons: Configuration can be complex, requires a learning period.

Integrating Build Systems Into Your Workflow
Setting Up Your Environment
Setting up your chosen build system depends on your development environment. For instance, to set up CMake with Visual Studio:
- Install CMake and ensure it's in your system's PATH.
- Create a directory for your project and navigate to it in the command line.
- Run:
cmake -G "Visual Studio 16 2019" .
- Open the generated `.sln` file in Visual Studio to begin development.
Automation and Continuous Integration
Continuous Integration (CI) systems, such as Jenkins or GitHub Actions, can help automate the testing and deployment of your C++ applications. Integrating your build system with CI can streamline the development process by automatically running builds and tests.
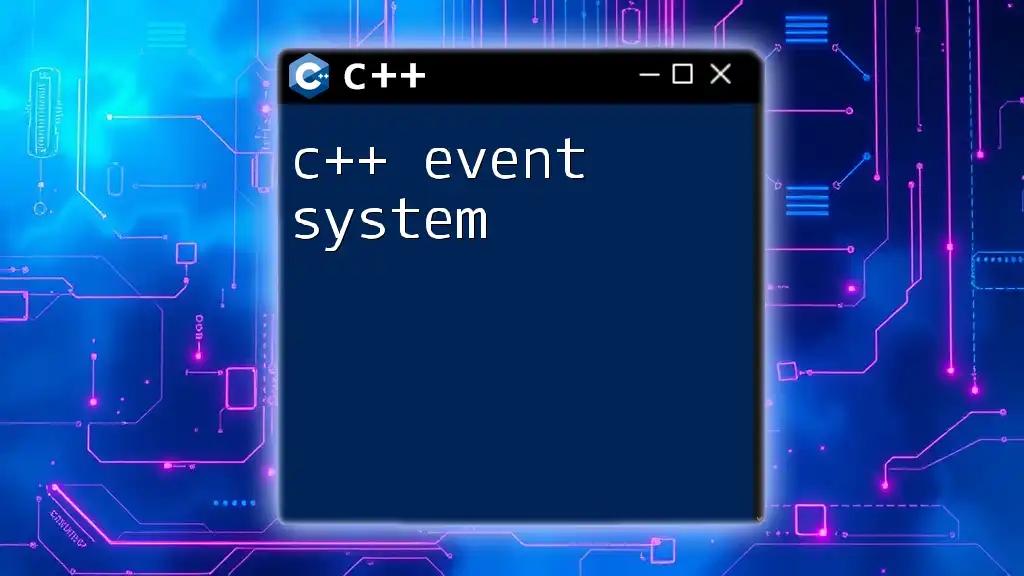
Common Issues and Solutions
Debugging Build Errors
While building C++ applications, you may encounter common errors. Understanding the error messages can save you substantial time. Coding mistakes, like missing semicolons or undeclared variables, often lead to compilation errors that can be resolved by carefully reading the compiler output.
Performance Optimization
To optimize build performance, consider techniques like using precompiled headers. A precompiled header allows you to compile commonly used headers once, reducing compile time for subsequent compilations. Here’s how a precompiled header might be included in a Makefile:
CXXFLAGS = -include precompiled_header.h
By understanding and implementing these strategies, you can enhance the efficiency of your C++ projects and streamline your workflow.

Conclusion
Choosing the right C++ build system is crucial for your development process. By understanding the nuances of each system and how they fit different project needs, you can make informed decisions that enhance your coding efficiency. Don't hesitate to try out various systems to discover which aligns best with your workflow and project goals.

Additional Resources
To continue learning about C++ build systems, consider exploring books on software development practices, online courses that focus on CMake and Meson, and the official documentation of the respective build tools. These resources can help cement your knowledge and proficiency in managing C++ projects efficiently.