C++ delegates are a design pattern that allows binding functions to events or callbacks, enabling a flexible way to handle event-driven programming.
Here's a simple code snippet demonstrating the concept of delegates using function pointers:
#include <iostream>
#include <functional>
void myFunction() {
std::cout << "Hello from myFunction!" << std::endl;
}
int main() {
// Creating a delegate using std::function
std::function<void()> delegate = myFunction;
// Invoking the delegate
delegate();
return 0;
}
What are Delegates?
C++ delegates are a powerful feature that allows methods to be referenced and invoked indirectly, enhancing flexibility and allowing for better decoupling in code. By essentially acting as a reference to a function, delegates can streamline certain programming tasks, especially those involving callback mechanisms.
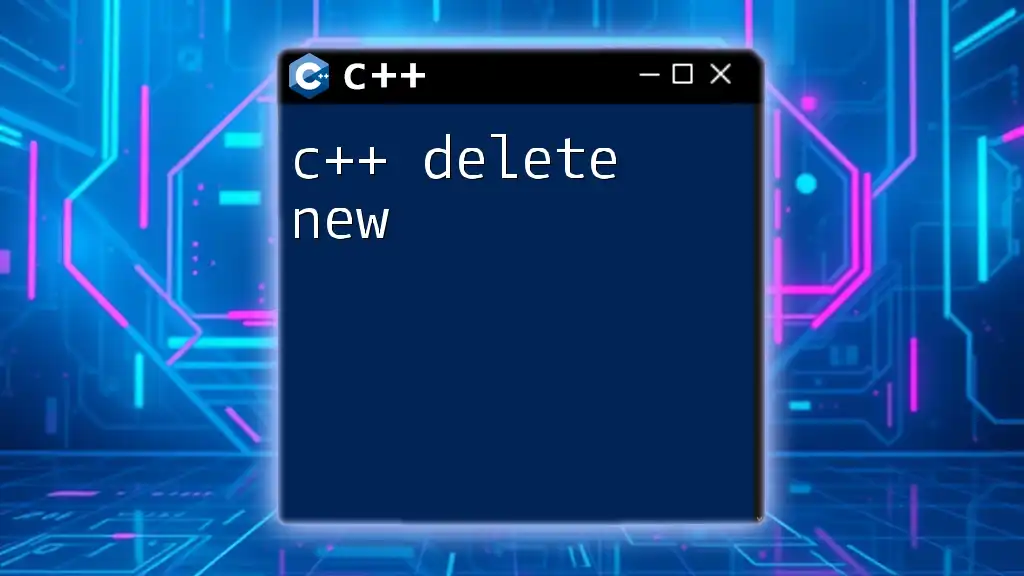
Why Use C++ Delegates?
Using C++ delegates comes with multiple benefits:
-
Flexibility: Delegates provide a layer of abstraction over traditional function calls. This allows changing the method to be invoked at runtime without modifying the caller code.
-
Decoupling: By allowing the calling code to specify which method to execute, delegates create a more modular design that reduces dependencies between components.
By comparison, function pointers lack the syntactic safety and ease of use that delegates provide, as they do not support bindings to member functions without cumbersome techniques involving the `this` pointer.
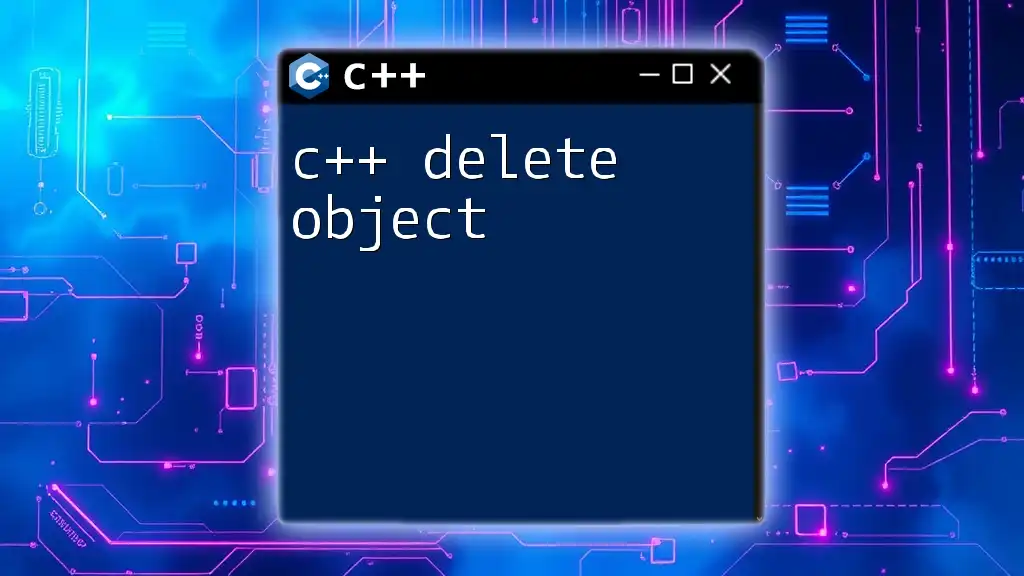
Understanding the Basics of C++ Delegates
What Makes Up a Delegate?
A delegate in C++ typically consists of a method signature and the target function or object. In practical terms, this means that you have a typed interface that allows you to call various functions that conform to that interface.
Key Components
-
Method Signature: This defines the return type and parameters of the functions that can be assigned to the delegate.
-
Target Function/Object: The actual function or member function that will be invoked when the delegate is called.
Types of Delegates in C++
Global Delegates
Global delegates are the simplest to implement. They provide a straightforward way to reference functions that are not tied to any class.
Example:
void GlobalFunction() {
// Implementation
}
Class Delegates
Class delegates require understanding of member functions, which are tied to specific object instances. To use a member function as a delegate, it’s essential to keep in mind the need for the `this` pointer.
Example:
class MyClass {
public:
void MemberFunction() {
// Implementation
}
};
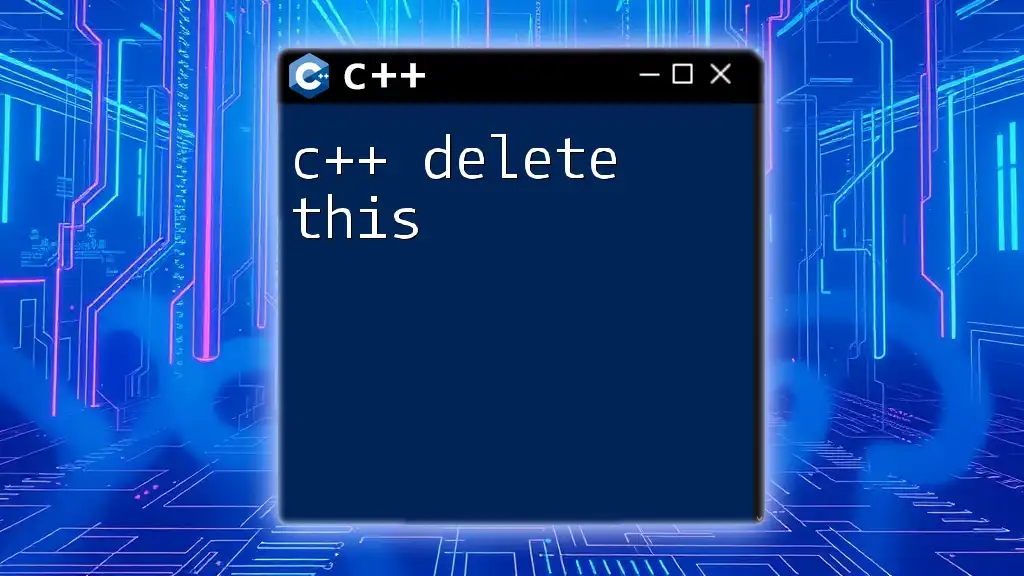
Implementing Delegates in C++
Step-by-Step Implementation
Creating a simple delegate in C++ consists of a few key steps. Below are the essentials of setting up a delegate:
Code Snippet: Creating a Delegate
#include <iostream>
#include <functional>
void GlobalFunction() {
std::cout << "Global function called!" << std::endl;
}
int main() {
std::function<void()> myDelegate = GlobalFunction; // Assign global function
myDelegate(); // Call the delegate
return 0;
}
Working with Member Functions
When working with member functions, you need to bind the delegate to an instance of the class.
Code Example: Using a Member Function Delegate
#include <iostream>
#include <functional>
class MyClass {
public:
void MemberFunction() {
std::cout << "Member function called!" << std::endl;
}
};
int main() {
MyClass obj;
std::function<void()> memberDelegate = std::bind(&MyClass::MemberFunction, &obj);
memberDelegate(); // Call the delegate
return 0;
}
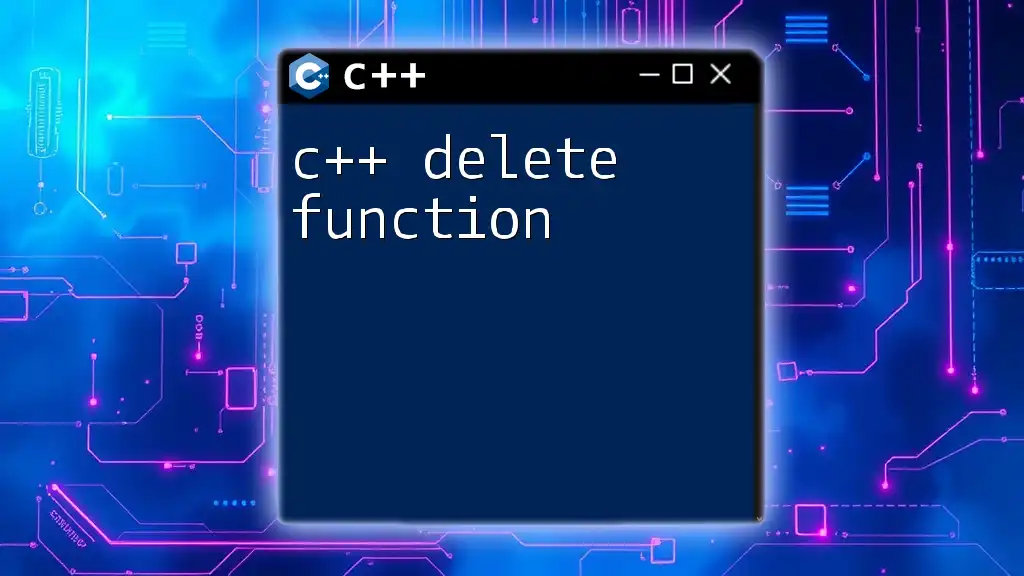
Advanced Delegate Features
Handling Multiple Delegates
You can create rich event systems using C++ delegates by managing multiple delegate references. This can be achieved through containers such as `std::vector`.
Code Example: Broadcasting Messages
#include <iostream>
#include <functional>
#include <vector>
void GlobalFunction() {
std::cout << "Global function called!" << std::endl;
}
int main() {
std::vector<std::function<void()>> delegates;
delegates.push_back(GlobalFunction);
delegates.push_back([]() { std::cout << "Lambda function called!" << std::endl; });
for (auto& del : delegates) {
del(); // Call each delegate
}
return 0;
}
Lambda Expressions with Delegates
C++11 introduced lambda expressions, enabling inline function definition that can also be assigned to delegates. This is a particularly powerful feature for event handling or callback functions.
Code Snippet: Delegates with Lambdas
#include <iostream>
#include <functional>
int main() {
std::function<void()> lambdaDelegate = []() {
std::cout << "Lambda delegate called!" << std::endl;
};
lambdaDelegate(); // Call the lambda delegate
return 0;
}
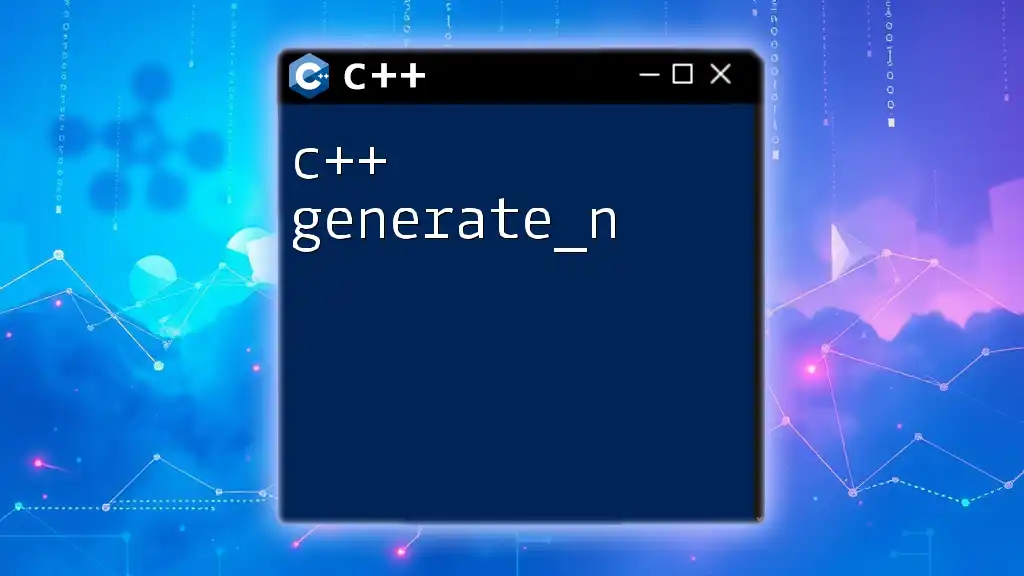
Best Practices for Using Delegates in C++
When to Use Delegates
Delegates are particularly helpful in scenarios such as implementing event handling, callback mechanisms, and decoupling applications for more flexible architectures. They shine in systems where you need to communicate between components without tight bindings.
Performance Considerations
While C++ delegates offer convenience, it is crucial to acknowledge the slight overhead introduced compared to direct function calls. Ensure that they are used judently in performance-critical code paths. Always measure and understand their impact within your specific application context.
Debugging Delegate Issues
Common issues arising from using delegates often include:
-
Invalid Function References: Ensure that the delegate is assigned correctly and points to an existing function when invoked.
-
Object Lifetimes: Be cautious of the object lifetime, especially with member function delegates. It is vital to manage the lifecycle of instances being pointed at.
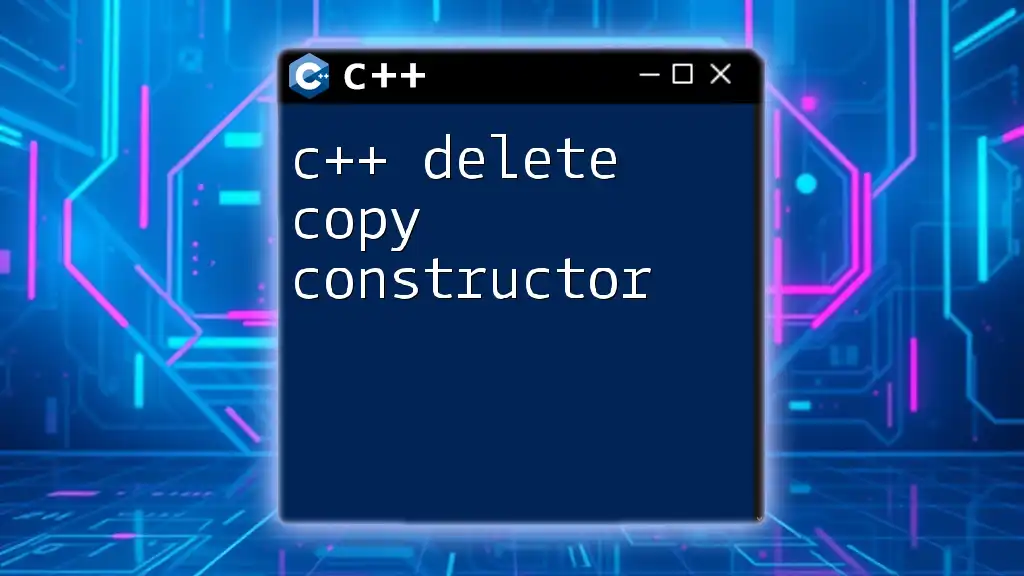
Real-World Applications of C++ Delegates
Event Handling and Callbacks
C++ delegates lend themselves perfectly to event-driven architectures. For instance, in GUIs or asynchronous programming, delegates can manage callbacks seamlessly, streamlining user interactions and operations without tightly coupling components.
GUI Development
Implementing delegates in GUI frameworks allows for elegant handling of events (like button clicks) via callback functions. This makes event handling code cleaner and more maintainable.
Asynchronous Programming
In modern C++ applications, you often rely on asynchronous tasks. Using delegates to manage callbacks for asynchronous operations helps reduce callback hell and leads to more readable and maintainable code.
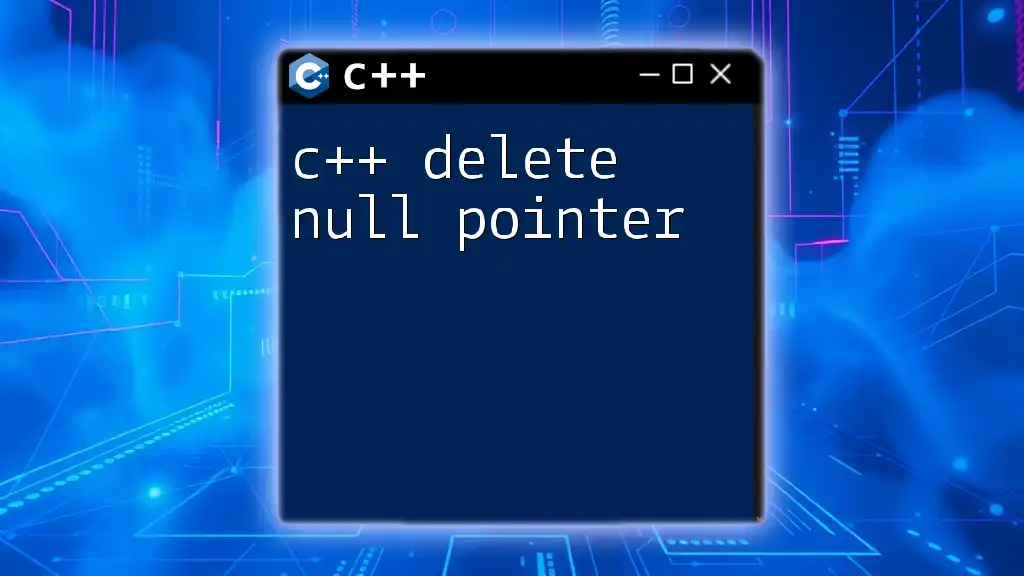
Conclusion
Understanding and implementing C++ delegates can significantly enhance your programming efficiency. As you explore the world of C++ increasingly, incorporating delegates into your code will allow for more modular, flexible, and adaptive applications. The benefits gained from employing this feature will ultimately lead to a better structured and more potent application design. Remember to practice using delegates in your projects to fully harness their potential and improve your coding repertoire.
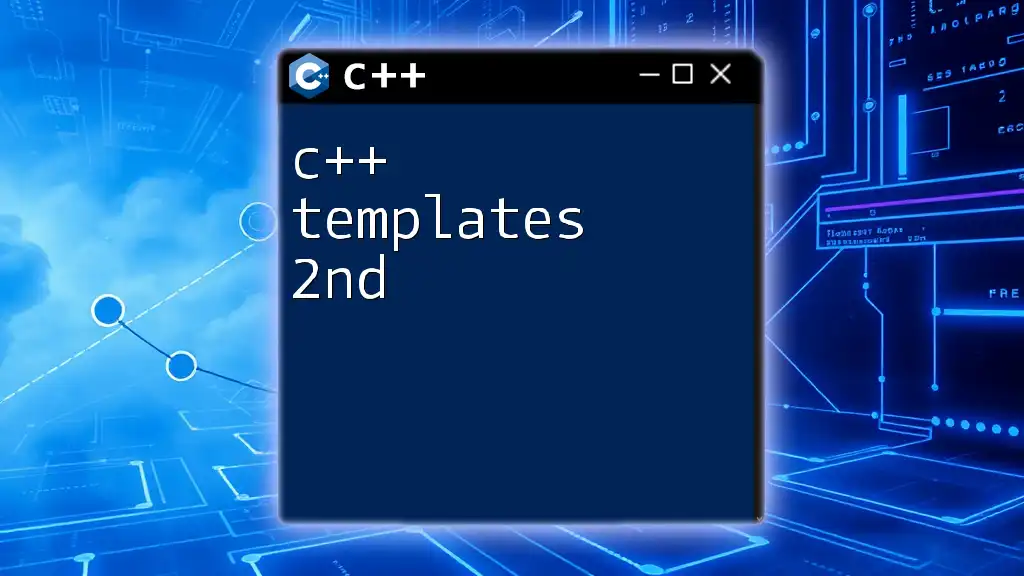
Additional Resources
For further reading on C++ and delegates, consider exploring recommended books on advanced C++ concepts or participating in online communities where you can engage with fellow developers and share your learnings.