In C++, a decorator is a design pattern that allows behavior to be added to individual objects, either statically or dynamically, without affecting the behavior of other objects from the same class.
Here’s a simple example demonstrating the decorator pattern:
#include <iostream>
#include <string>
// Base class
class Coffee {
public:
virtual std::string getDescription() const {
return "Coffee";
}
virtual double cost() const {
return 2.0;
}
};
// Decorator base class
class CoffeeDecorator : public Coffee {
protected:
Coffee* coffee;
public:
CoffeeDecorator(Coffee* c) : coffee(c) {}
};
// Concrete decorator
class Milk : public CoffeeDecorator {
public:
Milk(Coffee* c) : CoffeeDecorator(c) {}
std::string getDescription() const override {
return coffee->getDescription() + ", Milk";
}
double cost() const override {
return coffee->cost() + 0.5;
}
};
// Another concrete decorator
class Sugar : public CoffeeDecorator {
public:
Sugar(Coffee* c) : CoffeeDecorator(c) {}
std::string getDescription() const override {
return coffee->getDescription() + ", Sugar";
}
double cost() const override {
return coffee->cost() + 0.2;
}
};
int main() {
Coffee* myCoffee = new Milk(new Sugar(new Coffee()));
std::cout << myCoffee->getDescription() << " costs " << myCoffee->cost() << std::endl;
delete myCoffee; // Clean up memory
return 0;
}
What is the Decorator Pattern?
The C++ Decorator pattern is a structural design pattern that allows behavior to be added to individual objects, either statically or dynamically, without affecting the behavior of other objects from the same class. This enhances the flexibility of the code, enabling developers to modify certain aspects of an object’s functionality without altering its core structure.
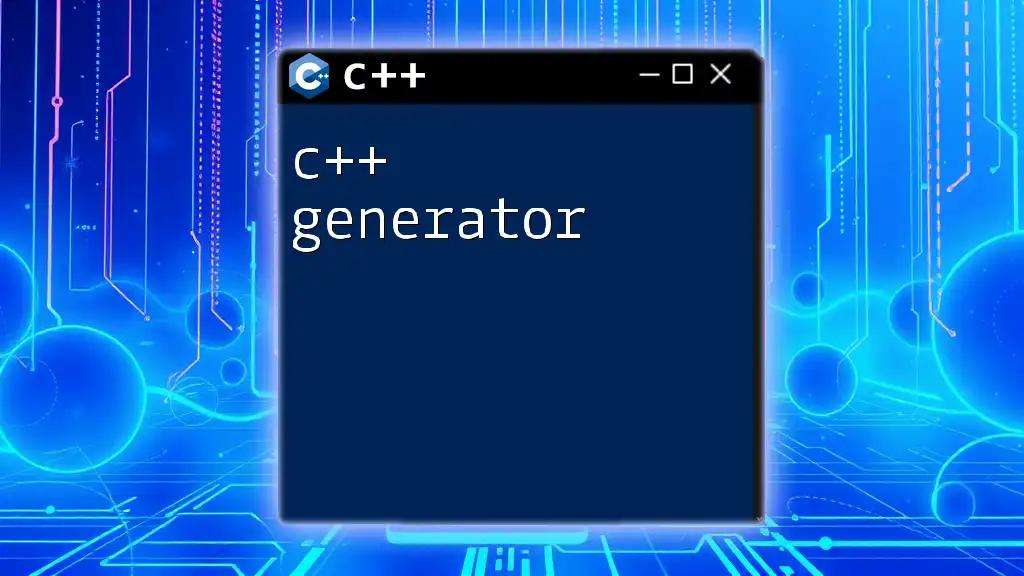
Why Use the Decorator Pattern in C++?
Using the decorator pattern comes with several advantages:
- Flexibility: You can add responsibilities to objects at runtime, allowing programming to accommodate future changes without modifying existing code.
- Adherence to the Open/Closed Principle: Classes should be open for extension but closed for modification. The decorator pattern allows you to extend functionality without changing existing class code.
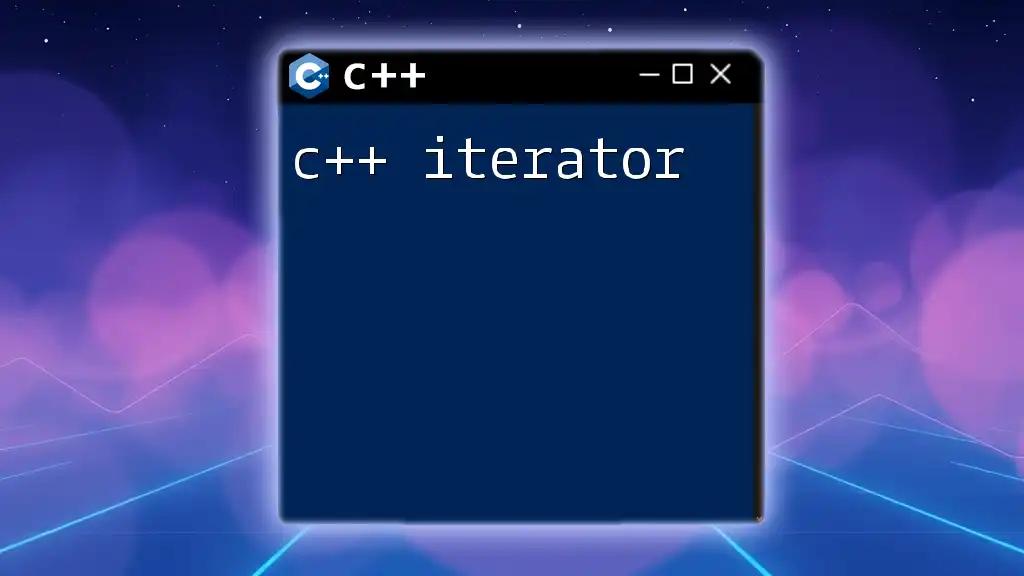
Understanding Decorators
In the context of the C++ decorator pattern, a decorator is a structural design that lets you wrap an object to provide additional functionality. The essence of the pattern is that decorators are interchangeable and can be composed to create various enhancements.
Components of the Decorator Pattern
- Base Component: This is an abstract class that defines the common interface for both the concrete components and the decorators.
- Concrete Component: This is the class that implements the base component. It provides the core functionality, which can be enhanced later.
- Decorator Class: This class follows the same interface as the base component and holds a reference to an object of the base component, allowing it to override the methods and add additional behavior.
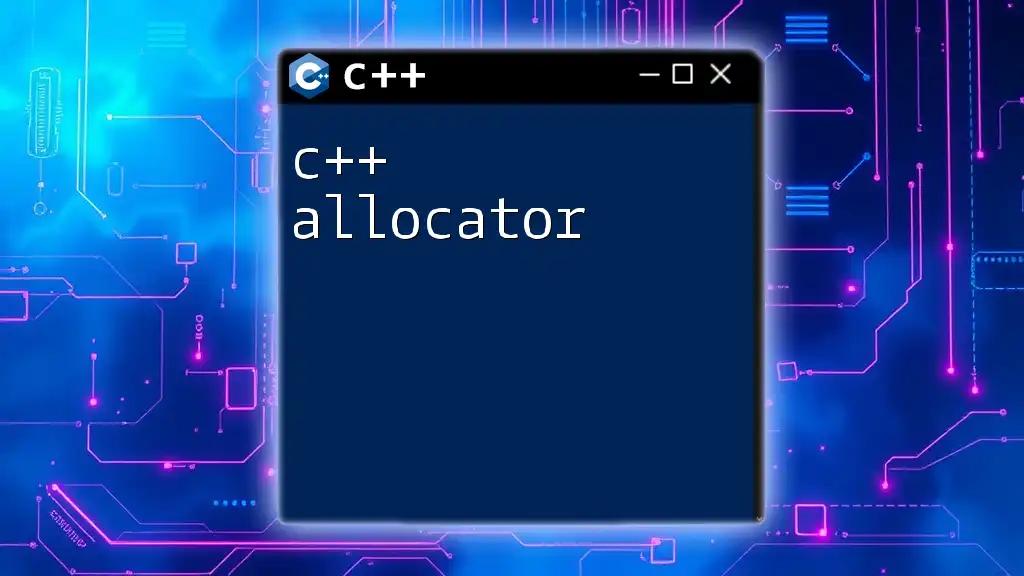
Implementing the Decorator Pattern in C++
Setting Up the Environment
Before diving into the implementation of the C++ decorator, ensure you have a C++ compiler (such as g++) installed and a basic understanding of C++ classes and inheritance. An IDE like Visual Studio, Code::Blocks, or even a text editor with a terminal will be suitable for coding.
Basic Example of the Decorator Pattern
First, we will define a simple text component. This component will serve as our base for future decorators.
class Text {
public:
virtual std::string getContent() const {
return "Hello, World!";
}
virtual ~Text() {}
};
Building a Concrete Component
Next, we create a concrete component that inherits from the Text class. This will serve as the base functionality.
class PlainText : public Text {
public:
std::string getContent() const override {
return "This is plain text.";
}
};
Creating Decorators
To build a decorator, we first create a base decorator class that also inherits from the Text.
class TextDecorator : public Text {
protected:
Text* text;
public:
TextDecorator(Text* t) : text(t) {}
~TextDecorator() { delete text; }
};
In this decorator class, we define a pointer to a Text object that allows us to refer to the object being decorated.
Concrete Decorators
Now let’s create a concrete decorator that enhances our text component by providing additional formatting. For example, we can add a bold effect.
class BoldText : public TextDecorator {
public:
BoldText(Text* t) : TextDecorator(t) {}
std::string getContent() const override {
return "<b>" + text->getContent() + "</b>";
}
};
In this class, we override the `getContent()` method to return the original content wrapped in bold tags.
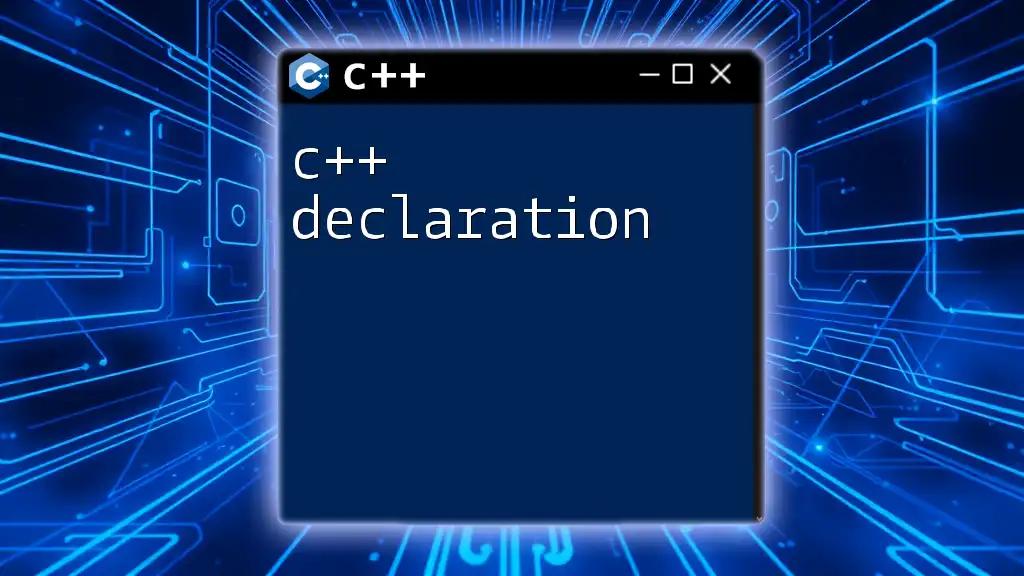
Applying the Decorator Pattern: A Real-World Example
Let’s consider a practical example of the C++ decorator pattern in a coffee-ordering system. Here, the base component will represent a coffee, and we will add features like milk and sugar as decorators.
Base Component
class Coffee {
public:
virtual double cost() const {
return 2.0; // Base cost for espresso
}
virtual ~Coffee() {}
};
Concrete Component
class Espresso : public Coffee {
public:
double cost() const override {
return 2.0; // Cost specifically for espresso
}
};
Decorators
Now, we implement decorators to add extra ingredients, such as milk and sugar.
class MilkDecorator : public Coffee {
Coffee* coffee;
public:
MilkDecorator(Coffee* c) : coffee(c) {}
double cost() const override {
return coffee->cost() + 0.5; // Adding cost for milk
}
~MilkDecorator() { delete coffee; }
};
class SugarDecorator : public Coffee {
Coffee* coffee;
public:
SugarDecorator(Coffee* c) : coffee(c) {}
double cost() const override {
return coffee->cost() + 0.2; // Adding cost for sugar
}
~SugarDecorator() { delete coffee; }
};
In this setup, you can create an espresso coffee and decorate it with milk and sugar as needed:
Coffee* myCoffee = new Espresso();
myCoffee = new MilkDecorator(myCoffee); // Adding milk
myCoffee = new SugarDecorator(myCoffee); // Adding sugar
std::cout << "Total Cost: " << myCoffee->cost() << std::endl; // Displays total cost
delete myCoffee; // Clean up memory

Advantages and Disadvantages of the Decorator Pattern
Pros of Using the Decorator Pattern
- Increased Flexibility: The decorator pattern allows functionalities to be added in layers, which means new features can be added without altering existing code, catering to the growing requirements of a project.
- Better Organization: By using the decorator pattern, you can adhere to the Single Responsibility Principle. Each class encapsulates a specific enhancement, making the overall codebase cleaner and easier to maintain.
Cons of Using the Decorator Pattern
- Complexity: The pattern can lead to a complicated class structure, especially when multiple decorators are applied to components. Understanding the flow may require more time.
- Difficulties in Debugging: The layered design may complicate debugging since the potential issue can exist across multiple decorators.
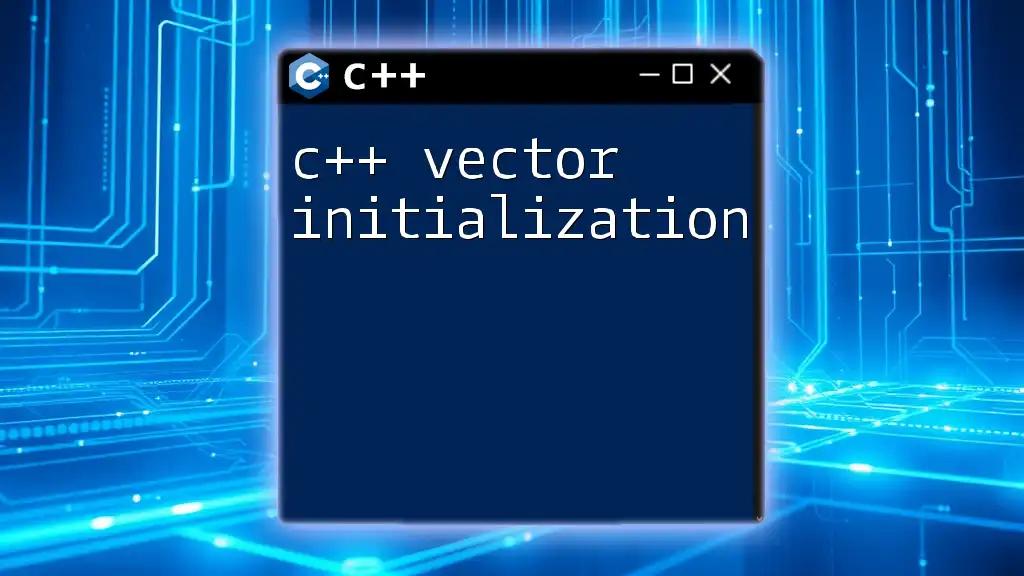
Conclusion
In summary, the C++ decorator pattern provides a powerful way to enhance the functionality of classes without modifying their structure. By employing this pattern, you can flexibly add new features to your applications while respecting the principles of object-oriented programming.
This article encourages you to experiment with the decorator pattern in your own projects. The more you practice, the deeper your understanding will become. Using decorators can lead to cleaner, more efficient, and more maintainable code.
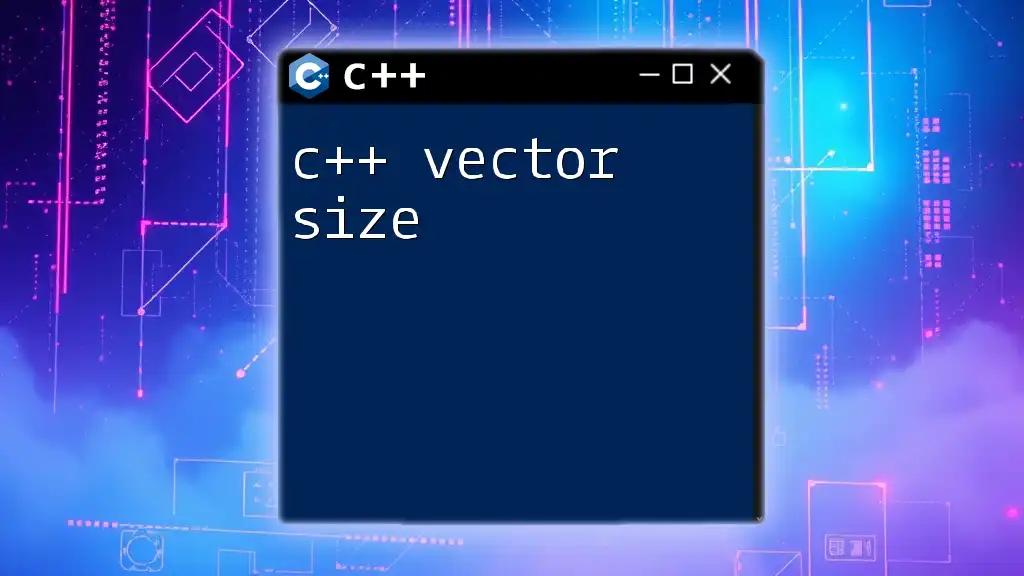
Further Reading and Resources
To expand your understanding of design patterns in C++, consider diving into the following resources:
- Books: "Design Patterns: Elements of Reusable Object-Oriented Software" by Erich Gamma et al.
- Online Resources: Websites like GeeksforGeeks and Medium often publish articles about design patterns.
- Documentation Links: The official C++ documentation can be invaluable when clarifying syntax or library usage.
By continually learning and applying these principles, you will enhance your programming skills and create robust applications that stand the test of time.