A C++ destructor is a special member function that is automatically called when an object goes out of scope or is deleted, and declaring it as virtual ensures that the derived class's destructor is invoked when an object is deleted through a base class pointer.
Here's a code snippet illustrating the use of a virtual destructor:
#include <iostream>
class Base {
public:
virtual ~Base() { std::cout << "Base destructor called" << std::endl; }
};
class Derived : public Base {
public:
~Derived() { std::cout << "Derived destructor called" << std::endl; }
};
int main() {
Base* b = new Derived();
delete b; // Calls Derived destructor followed by Base destructor
return 0;
}
Understanding Virtual Destructors in C++
What is a Virtual Destructor?
A virtual destructor is a special type of destructor that ensures proper resource management and cleanup when objects of derived classes are deleted through a base class pointer. Its primary purpose is to prevent memory leaks and undefined behaviors that can arise when polymorphism is involved in C++.
Why Use Virtual Destructors?
Using a virtual destructor is crucial in scenarios where base class pointers are used to point to derived class objects. If a base class destructor is not declared as virtual, it can lead to incomplete destruction of derived objects, leaving resources allocated by those derived classes intact. This can manifest in various ways, such as:
- Resource leaks
- Undefined behavior when accessing destructed objects
- Issues in managing dynamic memory
For example, if a derived class contains resources that need explicit cleanup, failing to use a virtual destructor in the base class will cause these resources to remain allocated after the base class destructor executes.
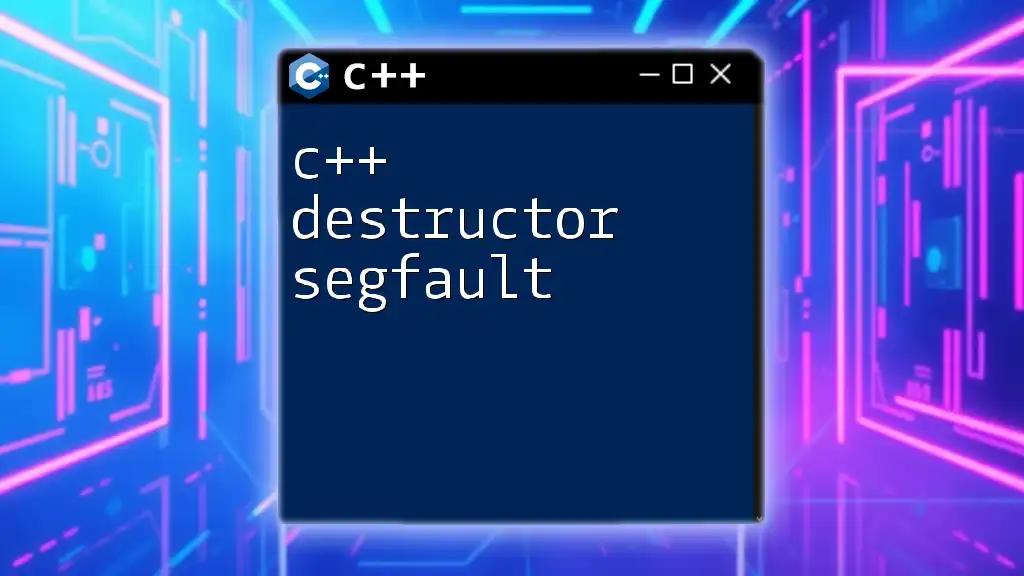
Syntax of C++ Virtual Destructors
Basic Structure
Declaring a destructor as virtual is straightforward. Here is a simple example:
class Base {
public:
virtual ~Base() {
// cleanup code
}
};
In the above code, `~Base()` is the destructor for the class `Base`, and it is declared as virtual. This tells the compiler that the destructor should be overridden in any derived class, ensuring proper cleanup.
Key Points of Syntax
When working with virtual destructors, there's a critical rule: Always declare the destructor as virtual in the base class. Failing to do so can lead to serious problems, especially in a polymorphic context. Additionally, when using multiple inheritance, be very mindful of how destructors behave, as they can have interactions that can complicate resource management.
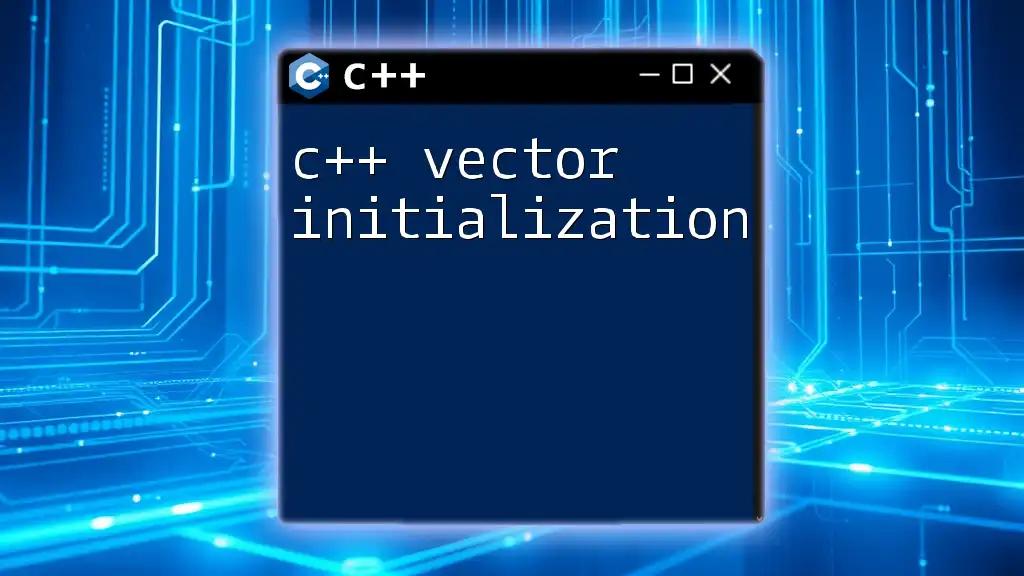
How Virtual Destructors Work
Under the Hood: Mechanism of Virtual Destructors
Behind the scenes, the C++ compiler manages virtual functions through something called a vtable (virtual table). Each class with virtual functions has a vtable. When a class inherits from a base class with a virtual destructor, the vtable entries are set up to call the correct destructor.
Example to Illustrate Behavior
To illustrate the behavior of virtual destructors, consider this code:
class Base {
public:
~Base() { std::cout << "Base Destructor\n"; }
};
class Derived : public Base {
public:
~Derived() { std::cout << "Derived Destructor\n"; }
};
// Demonstrate with and without virtual destructor
void example() {
Base* b = new Derived();
delete b; // Which destructor gets called?
}
In this example, if the destructor in `Base` were not declared as virtual, executing `delete b` would lead to only the `Base` destructor running, and the `Derived` destructor would not be called. This results in the message "Base Destructor" appearing while the resources managed by `Derived` remain unreleased, thus risking memory leaks.

Common Pitfalls and Best Practices
Common Mistakes in Using Virtual Destructors
- Not declaring the destructor as virtual: This is perhaps the most common pitfall and can lead to various forms of undefined behavior.
- Unintentional memory leaks: If derived classes allocate memory without a proper virtual destructor, those resources remain inaccessible after the parent’s delete operation.
Best Practices for Defining Virtual Destructors
- Always declare a virtual destructor in any class designed to be a base class.
- Utilize smart pointers like `std::unique_ptr` or `std::shared_ptr` where possible. These can handle resource deallocation safely, reducing the risk of memory leaks and making manual destructor management less of a concern.
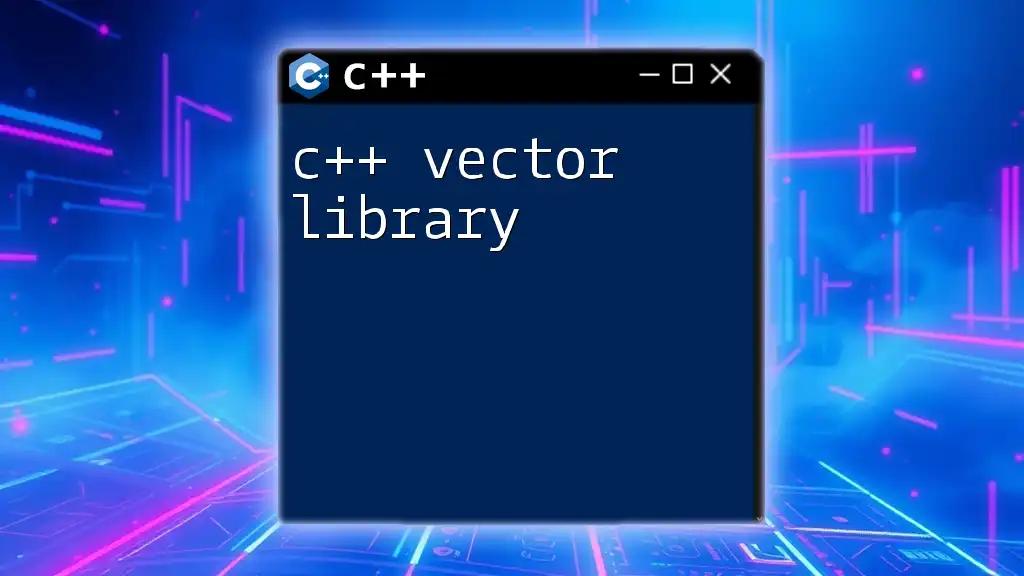
Performance Considerations
Impact on Performance
It is worth noting that virtual destructors introduce a small amount of overhead due to the need to maintain vtables and perform dynamic dispatch when destructors are called. However, in most applications, this overhead is negligible compared to the risk posed by not using a virtual destructor in polymorphic hierarchies.
Balancing Safety and Performance
When performance is critical, and polymorphism is unnecessary, consider using non-virtual destructors. Nonetheless, for any class that might be extended, the safety of a virtual destructor usually outweighs the performance costs.

Conclusion
Understanding and correctly implementing C++ destructor virtual is fundamental for resource management. It plays a critical role in ensuring that memory and other resources are properly released, particularly when dealing with polymorphism. Developers must be diligent in their approach to virtual destructors to prevent resource leaks and ensure program stability and integrity over time.

Additional Resources
For those looking to deepen their understanding of C++ destructors and other advanced concepts, consider exploring books on C++ programming, reputable online courses, and code repositories illustrating practical implementations.

Call to Action
Follow our company for further insights, tutorials, and resources aimed at mastering C++ programming techniques. Share this article to help others learn about the importance of virtual destructors in managing class hierarchies!