In C++, struct field initialization allows you to assign values to the fields of a struct when creating an instance of that struct, as shown in the following example:
struct Point {
int x;
int y;
};
Point p1 = {10, 20}; // Initialize p1 with x=10 and y=20
Understanding C++ Structures
What is a Struct in C++?
A `struct` (short for structure) in C++ is a user-defined data type that allows for the grouping of variables, often of diverse types, under a single umbrella. This can facilitate organization, readability, and maintenance of code.
One significant distinction between a struct and a class in C++ is access control. By default, struct members are public, while class members are private. This means that you can access struct variables without needing to create accessor methods, making structs an excellent choice for simple data structures.
Declaring a Struct
To declare a struct, you use the `struct` keyword followed by the struct name and its member variables wrapped in curly braces. Below is a simple example:
struct Person {
std::string name;
int age;
};
In this example, we create a struct called `Person` that contains two member variables: `name` and `age`. This method of aggregating data makes it easier to manage related information.
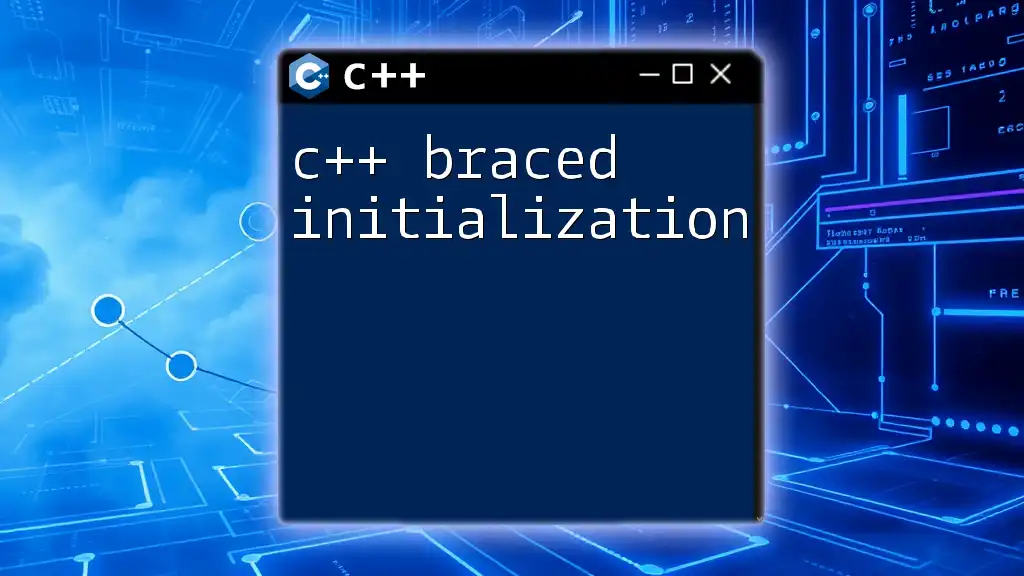
C++ Struct Field Initialization
What is Struct Initialization?
Struct initialization refers to the process of assigning initial values to a struct's member variables. Proper initialization is crucial as it helps avoid using garbage values that can lead to unpredictable behavior.
Different Methods to Initialize a Struct
Default Initialization
When you declare a struct variable without providing explicit values, it undergoes default initialization. For basic data types, this means that their values remain uninitialized and can lead to undefined behavior. Here’s a demonstration:
struct Person {
std::string name;
int age;
};
Person person; // Default initialization
In this case, `name` is left as an empty string, while `age` may have a garbage value. Therefore, always opt for initializing your structs to ensure reliability.
List Initialization
Introduced in C++11, list initialization allows you to instantiate a struct using curly braces. Here’s an example:
Person person{"Alice", 30}; // List initialization
In this instance, `name` is initialized to `"Alice"` and `age` to `30`. This approach is concise and clear and is generally preferred for initializations involving multiple fields.
Aggregate Initialization
Structs can also be initialized as aggregates. An aggregate is a struct that contains no user-defined constructors, no private or protected non-static data members, and no base classes. When initializing an aggregate, you can specify values without explicitly naming the members:
struct Point {
int x;
int y;
};
Point p{10, 20}; // Aggregate initialization
In the above example, `p` is initialized with `x` set to `10` and `y` set to `20`. This method is particularly effective when dealing with simpler structs.
Using Constructors for Struct Initialization
What Are Constructors?
A constructor is a special member function that is called when an object or struct is instantiated. Constructors can initialize member variables during creation, ensuring that the object begins its life in a valid state.
Constructor Initialization
To create a constructor in a struct, simply define a function with the same name as the struct. Here’s how you can do this:
struct Person {
std::string name;
int age;
// Constructor
Person(std::string n, int a) : name(n), age(a) {}
};
Person person("Bob", 25); // Constructor initialization
In this example, the `Person` struct contains a constructor that initializes `name` and `age` based on the provided arguments. This method not only simplifies initialization but also enhances code maintainability.
Initializing a Struct in C++ with Default Values
You can assign default values to struct members directly within the struct declaration. Here's an example:
struct Person {
std::string name = "Unknown";
int age = 0;
};
In this case, if you create a `Person` object without providing values, `name` will default to `"Unknown"` and `age` to `0`. This practice can enhance code robustness by ensuring sensible default states for members.
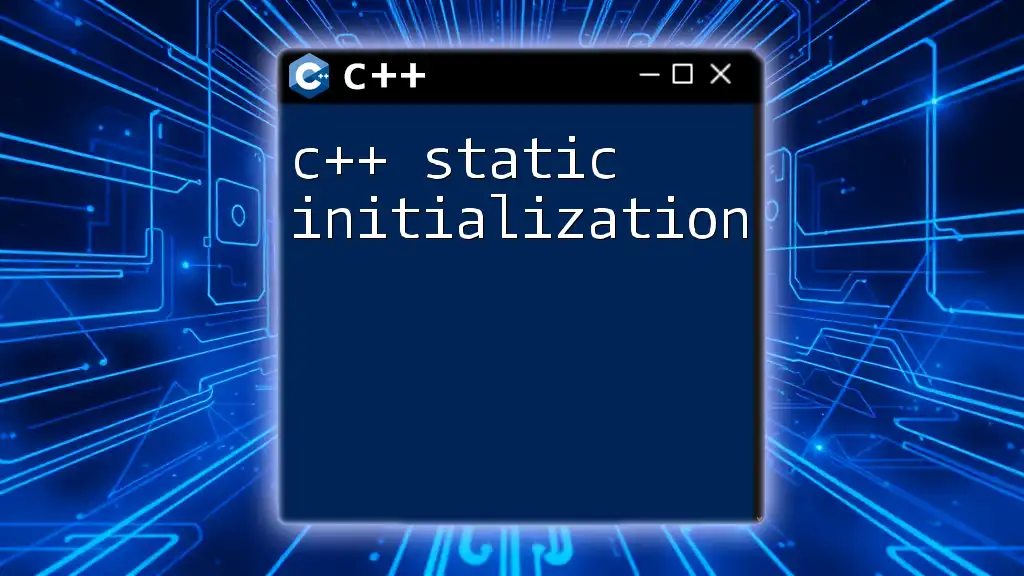
Practical Examples
Example 1: Simple Struct Initialization
Here, we see how you can initialize a struct directly:
struct Rectangle {
int width;
int height;
};
Rectangle rect{5, 10}; // Simple initialization
In this example, `rect` is initialized with a width of `5` and a height of `10`. This straightforward method increases readability and eliminates potential confusion.
Example 2: Complex Struct Initialization
When dealing with nested structs, initialization can be slightly more complex. Here’s how you can initialize a struct within another:
struct Address {
std::string city;
std::string state;
};
struct Employee {
std::string name;
Address address;
};
// Initializing the outer struct along with the nested struct.
Employee emp{"Alice", {"San Francisco", "CA"}};
In the above code, we initialize `Employee` with `name` and provide an `Address` object directly within the initialization.
Example 3: Structs with Dynamic Memory
Structs can also involve dynamic memory management. Below is an example of how you might initialize a struct that incorporates dynamic memory, such as an array:
struct DynamicArray {
int* array;
size_t size;
// Constructor that allocates memory for the array
DynamicArray(size_t s) : size(s) {
array = new int[size]; // Allocating dynamic memory
}
~DynamicArray() {
delete[] array; // Cleanup
}
};
// Initializing the struct
DynamicArray arr(5);
This example demonstrates how to manage memory dynamically within a struct to hold an array of integers. Properly handling memory ensures that you avoid memory leaks when the struct goes out of scope.
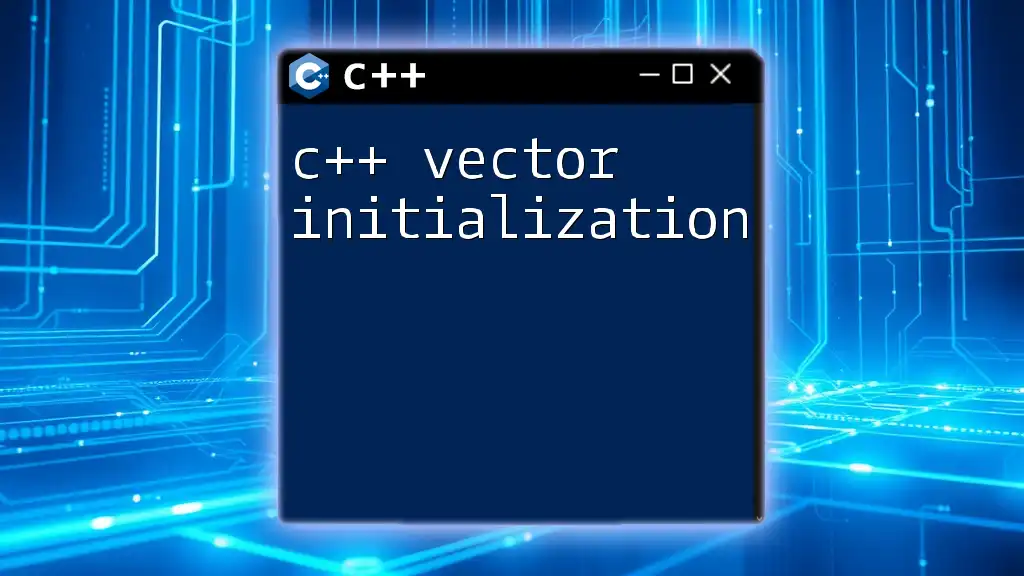
Common Mistakes in Struct Initialization
Forgetting to Initialize Members
One of the most common pitfalls is neglecting to initialize struct members. This can lead to using garbage values. Always verify that every data member is initialized, especially if you don't utilize constructors or initialization lists.
Misusing Initialization Methods
Another frequent mistake involves choosing the wrong initialization method for the situation. For example, using default initialization when you actually need aggregate initialization can result in unnecessary complexity and bugs. Familiarizing yourself with each method empowers you to make informed decisions.
Initialization Order in Structs
In structs, the order of initialization can affect the outcome of your code. The member variables are initialized in the order they are declared, which can lead to unexpected results if the initialization logic relies on other member variables. Here’s an example:
struct Example {
int x;
int y;
// Constructor relying on the order of initialization
Example(int a) : y(x + 1), x(a) {} // x is not initialized yet when y is assigned
};
In this case, `y` uses `x` before it is assigned, potentially leading to unexpected behavior.
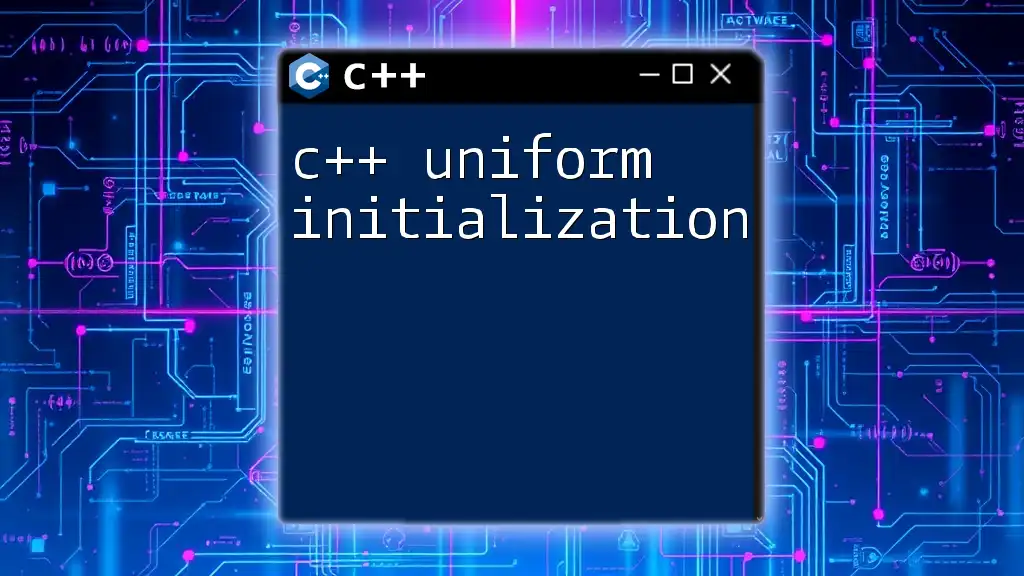
Best Practices for Struct Initialization in C++
Keep it Simple
Maintain clarity in struct definitions. Favor straightforward, uncomplicated designs that promote readability. This helps both new and experienced developers understand the code with ease.
Use Constructor Initialization When Necessary
Where appropriate, utilize constructors to enforce initialization rules and guarantee that all struct members are set to valid states right from object creation. Constructors can dramatically reduce boilerplate code while enhancing maintainability.
Use of Initializer Lists
Leverage initializer lists for their conciseness and clarity. They allow for quick, error-free initialization of all struct members without the clumsy assignment syntax.
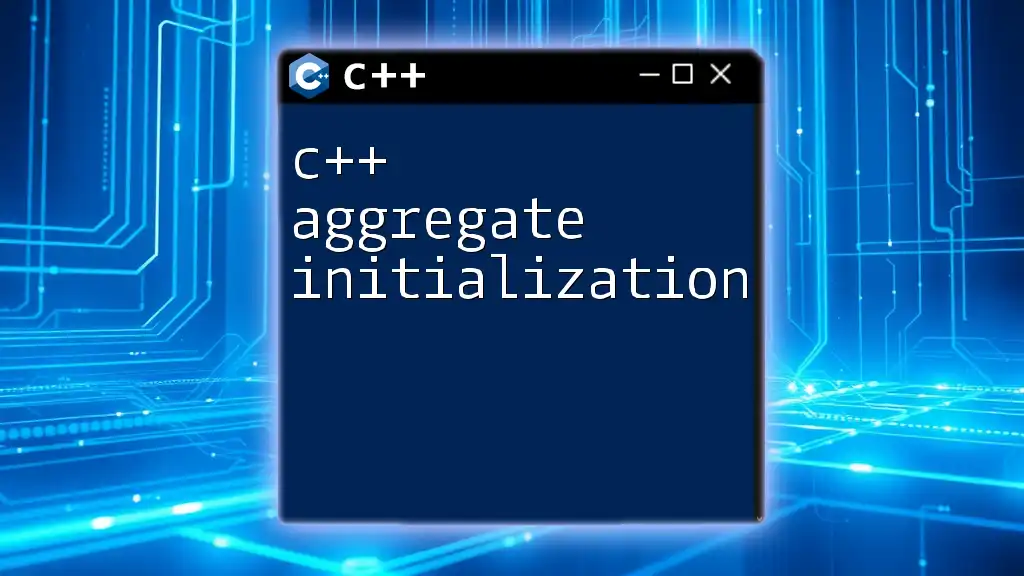
Conclusion
C++ struct field initialization is a vital aspect of programming that ensures your data structures are correctly defined before use. By choosing the right initialization method, you can prevent bugs, enhance code readability, and maintain the integrity of your applications. Understanding the nuances of struct initialization will empower you as a C++ developer, making your code more efficient and reliable.
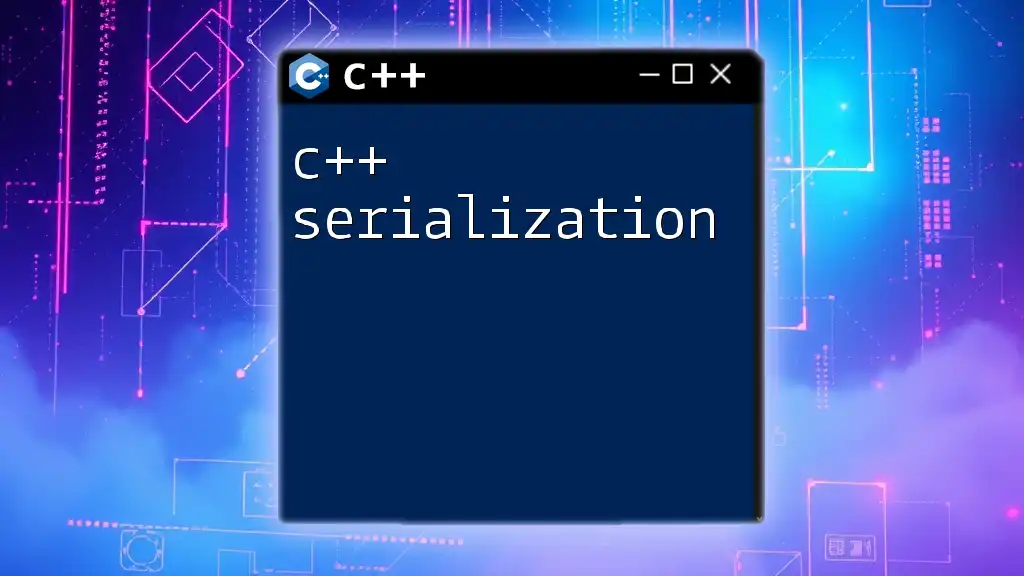
Further Reading
Explore additional resources and literature on C++ programming and struct initialization to deepen your understanding and hone your skills in creating robust software solutions.