C++ uniform initialization allows you to initialize variables using a consistent syntax, typically using curly braces, which helps prevent narrowing conversions.
int a{5};
double b{3.14};
std::vector<int> vec{1, 2, 3, 4, 5};
What is Uniform Initialization in C++?
C++ uniform initialization provides a consistent syntax for initializing objects, arrays, and other data types. It was introduced in C++11 to remedy the inconsistencies found in previous initialization methods. The primary goal is to provide better type safety, help avoid narrowing conversions, and maintain consistency across different types.
Key Features of C++ uniform initialization include:
- Type Safety: Uniform initialization helps ensure that the intended type is preserved after initialization. This reduces the chances of unexpected behavior during runtime.
- Avoiding Narrowing Conversions: By using braces, C++ prevents narrowing conversions, which occur when a value is converted to a type that cannot represent all possible values of the original type.
- Consistency Across Types: Uniform initialization employs the same syntax for all types of objects. This makes the code more readable and maintainable since the reader can easily identify initialization patterns.
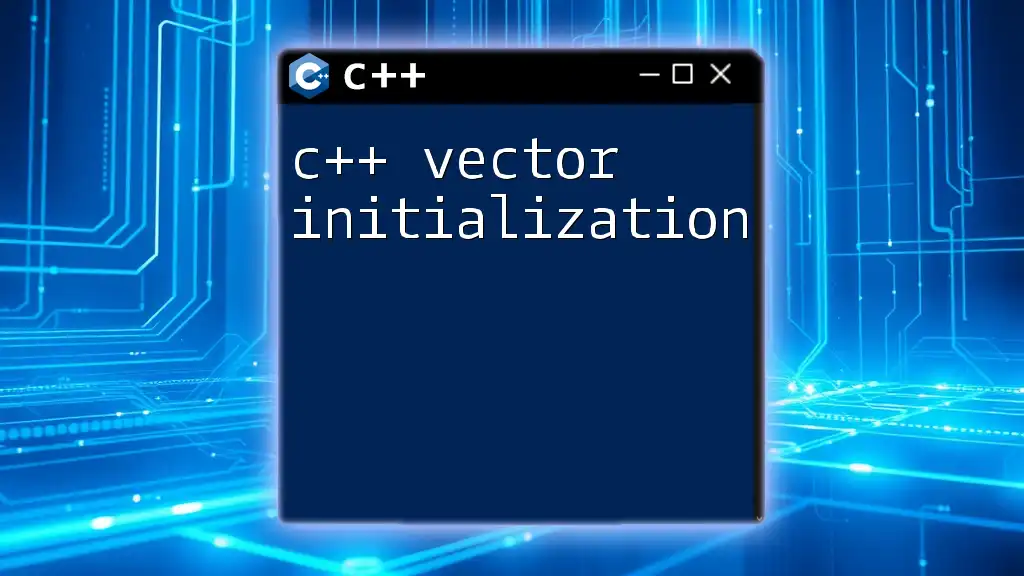
The Syntax of Uniform Initialization
Brace Initialization
The most significant aspect of uniform initialization is brace initialization. This syntax is straightforward: you use curly braces `{}` to enclose the values you wish to use for initialization.
Here’s how it looks for various data types:
int x{10}; // Integer initialization
double y{3.14}; // Double initialization
std::string str{"Hello World"}; // String initialization
This syntax can also be used for aggregate types, such as arrays and structs. Consider the following example:
struct Point {
int x, y;
};
Point p{1, 2}; // Struct initialization
int arr[]{1, 2, 3, 4}; // Array initialization
Aggregate Initialization
Uniform initialization can be particularly useful when working with aggregates (arrays or classes with no user-defined constructors). It offers a concise way to initialize and set values in these types, ensuring that all fields are appropriately assigned.
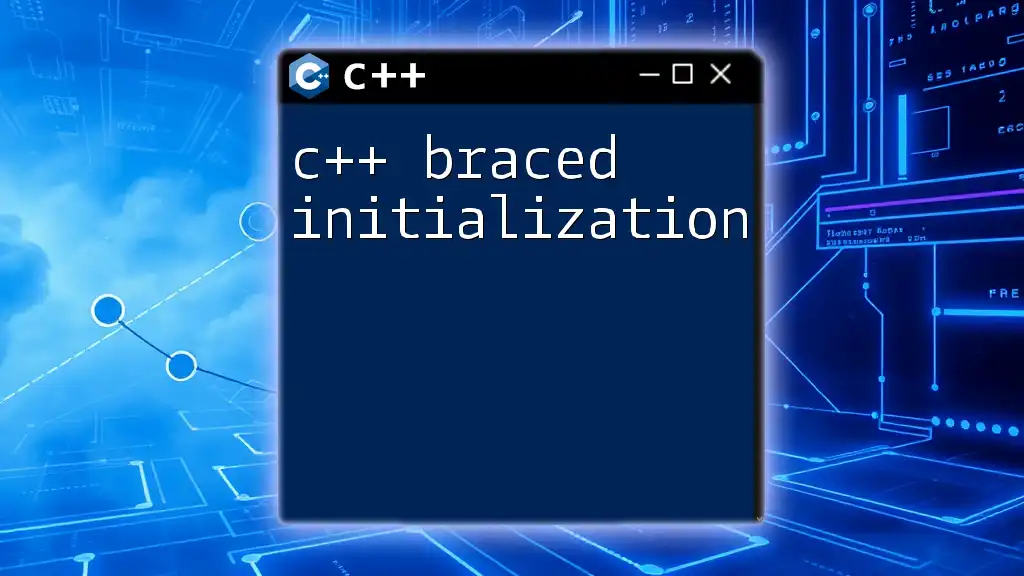
Comparison with Traditional Initialization Methods
Traditional Initialization Techniques
Before the adoption of uniform initialization in C++11, programmers relied on more traditional initialization methods. These approaches have their own syntax and potential quirks.
Here are some traditional initialization examples:
int a(10); // Traditional parentheses initialization
int b = 20; // Assignment initialization
Key Differences
Uniform initialization provides an advantage by preventing narrowing conversions. In previous initialization methods, developers had to be careful not to assign values that could lead to loss of data. For example, assigning a floating-point number to an integer could lead to undesired results:
int n(3.14); // Could cause issues or unexpected data loss.
With uniform initialization, the compiler enforces stricter type constraints:
int n{3.14}; // Error: narrowing conversion
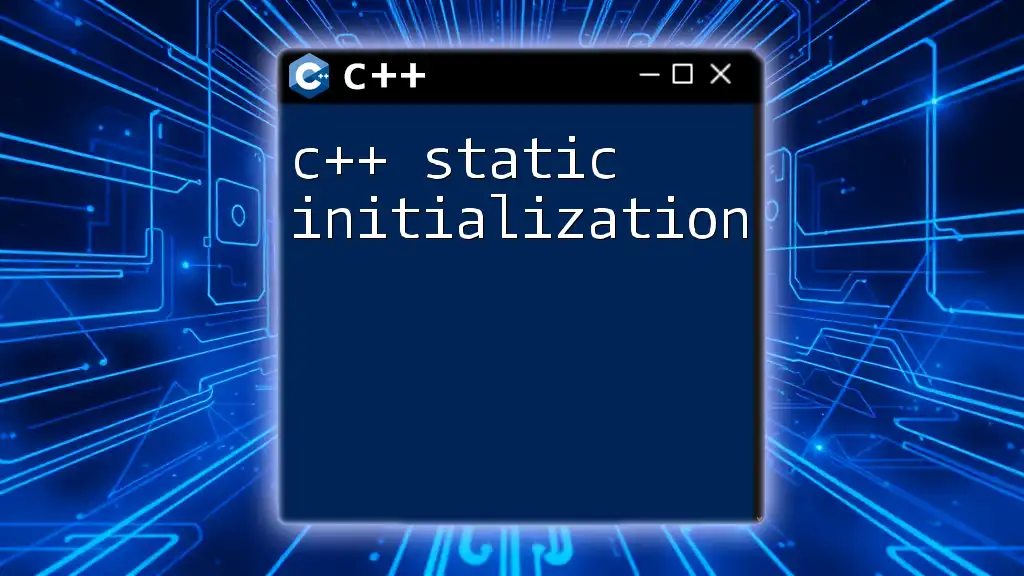
Benefits of Using Uniform Initialization
Type Safety
One of the standout benefits of using C++ uniform initialization is the enhanced type safety it offers. The syntax helps programmers avoid accidentally assigning incompatible types. This is crucial in large projects where bugs can be difficult to trace back to their origins.
Reduced Compiler Ambiguities
Using traditional initialization methods can sometimes lead to ambiguities in type determination. For instance, using parentheses may confuse the compiler regarding whether you are initializing an object or declaring a function. Uniform initialization removes this ambiguity by unifying the initialization syntax.
Cleaner Code
Lastly, adopting uniform initialization results in cleaner, more understandable code. When reading through source files, programmers can quickly grasp how objects are initialized without deciphering various initialization methods. This improves overall readability and maintenance.
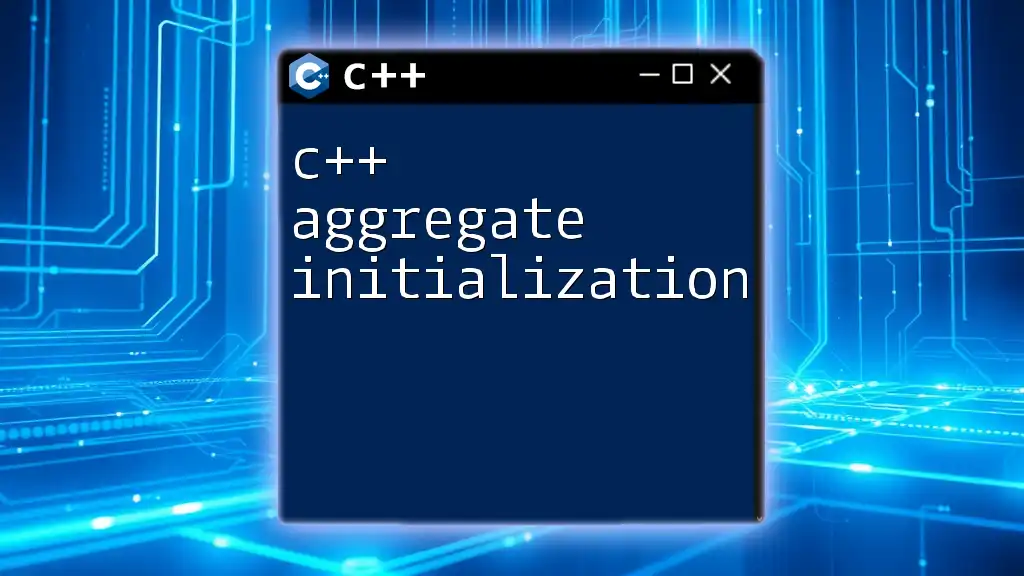
Common Pitfalls and Mistakes
Narrowing Conversions
While uniform initialization minimizes issues with narrowing conversions, developers must still be aware of this problem. A narrowing conversion occurs when a value from a wider type is converted to a narrower type, and data could be lost.
For example:
int x{3.14}; // Error: narrowing conversion
The compiler will raise an error, which helps in spotting potential issues before execution.
Ambiguities in Initialization
Another challenge lies in ambiguities that can arise with braces. For instance, initializing an array while trying to declare a pointer can lead to confusion:
int *p{nullptr}; // Creates a pointer initialized to nullptr
int arr[]{0}; // Creates an array with a single element
To avoid confusion, it is essential to use braces intentionally and recognize how they affect type resolution.
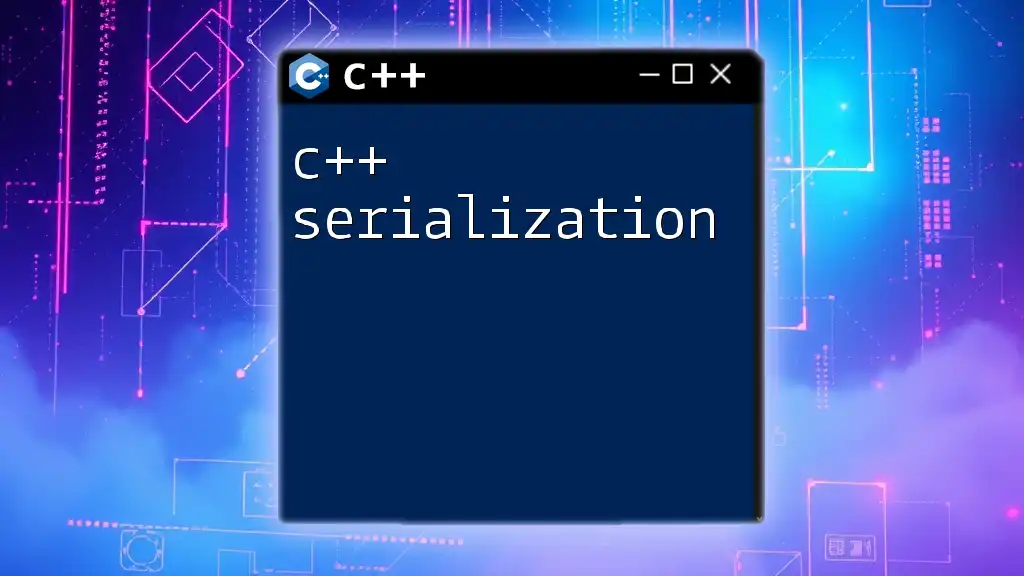
Advanced Usage of Uniform Initialization
Using Uniform Initialization with STL Containers
C++ uniform initialization is also highly beneficial when working with Standard Template Library (STL) containers. Here’s how you can initialize vectors and maps:
#include <vector>
#include <map>
#include <string>
std::vector<int> vec{1, 2, 3, 4}; // Initializing a vector
std::map<std::string, int> myMap{{"Alice", 30}, {"Bob", 25}}; // Initializing a map
Using this syntax not only streamlines the initialization process but also enhances readability.
Initializer Lists
Uniform initialization pairs well with initializer lists. These allow you to initialize classes using lists, improving usability and clarity:
class MyClass {
public:
MyClass(std::initializer_list<int> list) {
// Implementation
}
};
MyClass obj{1, 2, 3, 4}; // Creates an object using an initializer list
This capability lends itself to more flexible and extensible class designs.
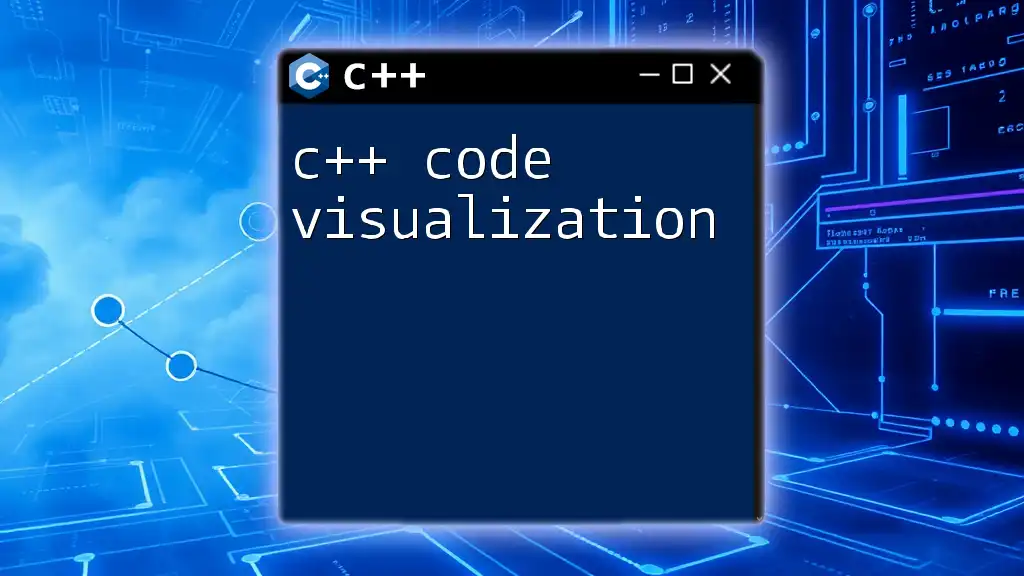
Conclusion
C++ uniform initialization represents a significant evolution in the C++ language, providing a robust mechanism to ensure safer and more consistent object initialization. By employing brace initialization, developers can avoid many common pitfalls associated with traditional methods, such as narrowing conversions and ambiguities.
Switching to uniform initialization not only enhances code readability but also reduces the likelihood of introducing elusive bugs. As programming practices continue to evolve, embracing C++ uniform initialization is a step toward writing cleaner, safer, and more maintainable code.