C++ aggregate initialization allows you to initialize an object of an aggregate type (like structs or arrays) using a braced list of initializers.
Here's a code snippet demonstrating aggregate initialization for a struct:
struct Point {
int x;
int y;
};
Point p1 = {10, 20}; // Aggregate initialization of a Point object
Understanding Aggregates in C++
Definition of Aggregate Types
In C++, aggregate types are data structures that consist of multiple members. Specifically, they are uninitialized data types such as `structs`, `arrays`, and `unions` that meet certain criteria. Notably, an aggregate does not possess any user-defined constructors, virtual functions, or any private/protected non-static data members.
Characteristics of Aggregate Types
- No user-defined constructors: Aggregate types must be initialized using aggregate initialization; they cannot have constructors that the programmer defined.
- No private or protected non-static data members: All members must be public to allow initialization without special access.
- No base classes: Aggregate types cannot derive from any class, maintaining a straightforward and clear structure.
- No virtual functions: Virtual functions introduce complexity that aggregate initialization is not designed to handle.
Understanding these characteristics is crucial as they define the constraints under which aggregate initialization operates.
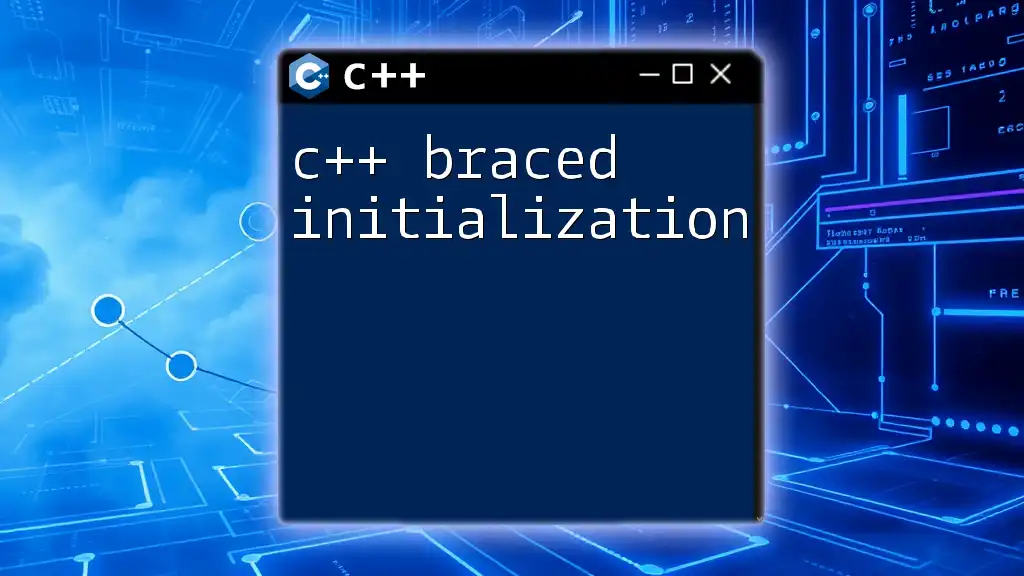
Aggregate Initialization
What is Aggregate Initialization?
Aggregate initialization is a concise way to initialize aggregate types in C++. It allows you to assign values to all members of an aggregate type in a single statement, using a curly brace syntax. This method is essential for creating readable and error-free code.
Syntax and Semantics of Aggregate Initialization
The syntax for aggregate initialization is straightforward. You list the values within curly braces, matching the order of the members in the aggregate.
Here’s a basic example:
struct Point {
int x;
int y;
};
Point p1 {10, 20}; // Using aggregate initialization
In this example, `p1` is initialized with the `x` member set to `10` and the `y` member set to `20`. This approach allows initializing multiple members at once, resulting in cleaner and more compact code.
Aggregate Initialization for Arrays
Aggregate initialization also applies to arrays in C++. You can initialize all elements in an array succinctly.
For instance:
int arr[3] {1, 2, 3}; // Initializing an array using aggregate initialization
In this case, the array `arr` is initialized with three integers. If fewer values are provided, the remaining elements are automatically assigned the value zero.
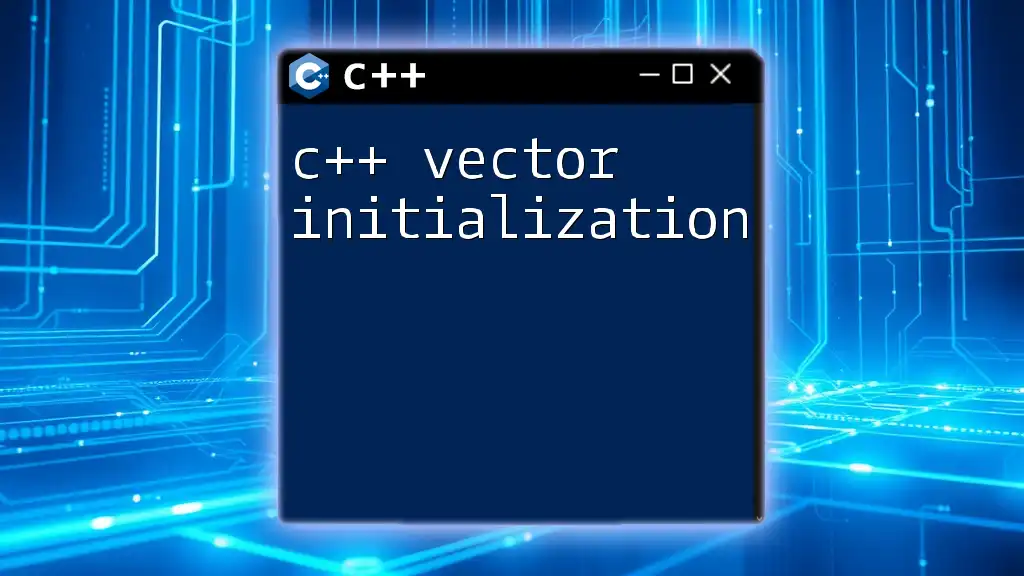
Benefits of Aggregate Initialization
Simplicity and Clarity
One of the most significant advantages of aggregate initialization is its simplicity. The curly brace syntax makes it immediately clear what values are assigned to each member or element, contributing to readability and easy maintenance. This clarity is especially helpful in long structures or large data sets.
Performance Benefits
Aggregate initialization can also offer performance advantages. Since the initialization occurs in a single operation, the compiler can optimize memory allocation and performance better than it can with multiple assignment statements. This results in faster execution and lower overhead, making aggregate initialization a preferred choice for performance-critical applications.
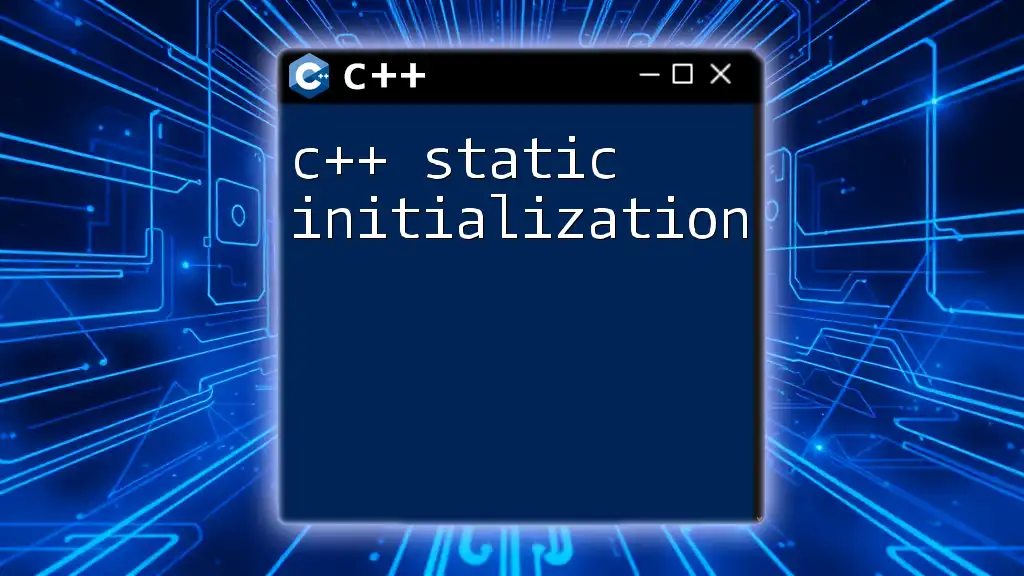
Common Use Cases of Aggregate Initialization
Using Aggregate Initialization with Structs
Structs are one of the primary use cases for aggregate initialization. When you define complex data types using structs, initializing them using aggregates reduces the likelihood of errors and ensures that all members can be set up at once.
struct Rectangle {
int width;
int height;
};
Rectangle rect1 {5, 10}; // Simple initialization
Rectangle rect2 {8, 15}; // Another example
In the code above, `rect1` and `rect2` are initialized with their respective dimensions in a clear manner.
Nested Aggregates
Aggregate initialization also supports nested structures, allowing for the concise initialization of complex types.
struct Point {
int x;
int y;
};
struct Circle {
Point center;
int radius;
};
Circle c1 { {0, 0}, 5 }; // Initializing a Circle with aggregate initialization
In this example, the `Circle` structure, which contains a `Point`, is initialized with nested braces. This flexibility allows the initialization of hierarchical data structures without sacrificing clarity.
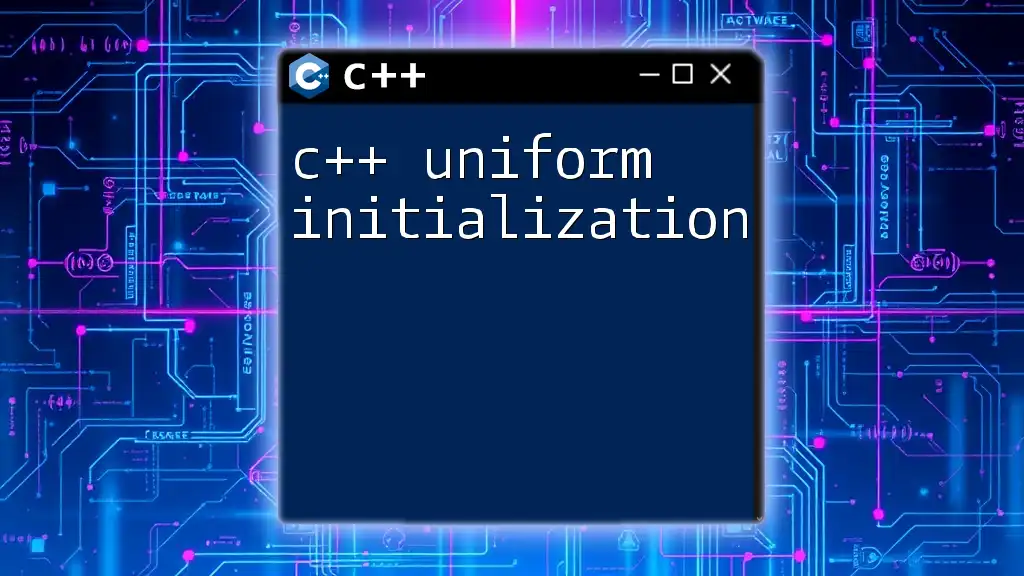
Limitations of Aggregate Initialization
When Aggregate Initialization Cannot Be Used
Although aggregate initialization is powerful, it does have limitations. You cannot use this method if your aggregate type has user-defined constructors, virtual functions, or private/protected members.
struct Invalid {
Invalid() {} // User-defined constructor
};
Invalid inv {}; // Error: Cannot use aggregate initialization
In this case, attempting to use aggregate initialization raises a compilation error because the struct has a user-defined constructor.
Understanding Brace Elision
Brace elision is a feature that allows initialization without specifying all values explicitly. If you have fewer values than elements in an aggregate, the remaining elements will default to zero.
int arr[3] = {1, 2}; // Brace elision allows for missing elements
Here, the array `arr` will contain `1`, `2`, and `0`. While this feature is convenient, it can sometimes lead to unexpected behavior, so it's essential to be cautious.
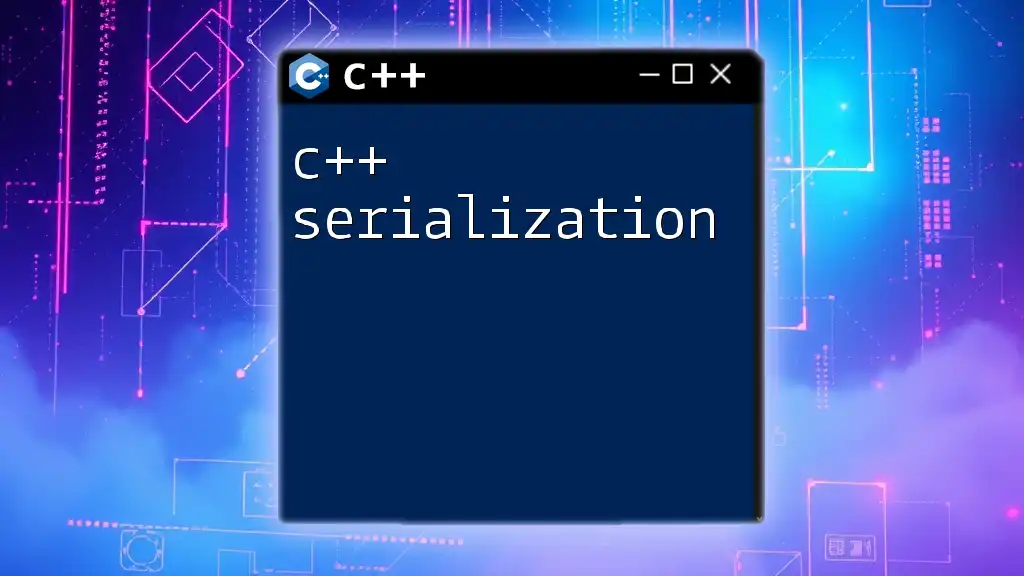
Best Practices for Aggregate Initialization
When to Use Aggregate Initialization
Aggregate initialization should be used whenever dealing with plain old data (POD) types like structs or arrays. It is especially useful in scenarios where you want to quickly create multiple instances with various initial values.
For instance, if you are working with a configuration structure, using aggregate initialization can ensure that all parameters are set correctly at the time of creation.
Tips for Writing Clear Code
When utilizing aggregate initialization, maintain clarity by ensuring that the structures are well-defined. Always provide meaningful member names and comment on your code where necessary. This practice will not only make your code easier to read but will also minimize the chances of errors as the codebase evolves.
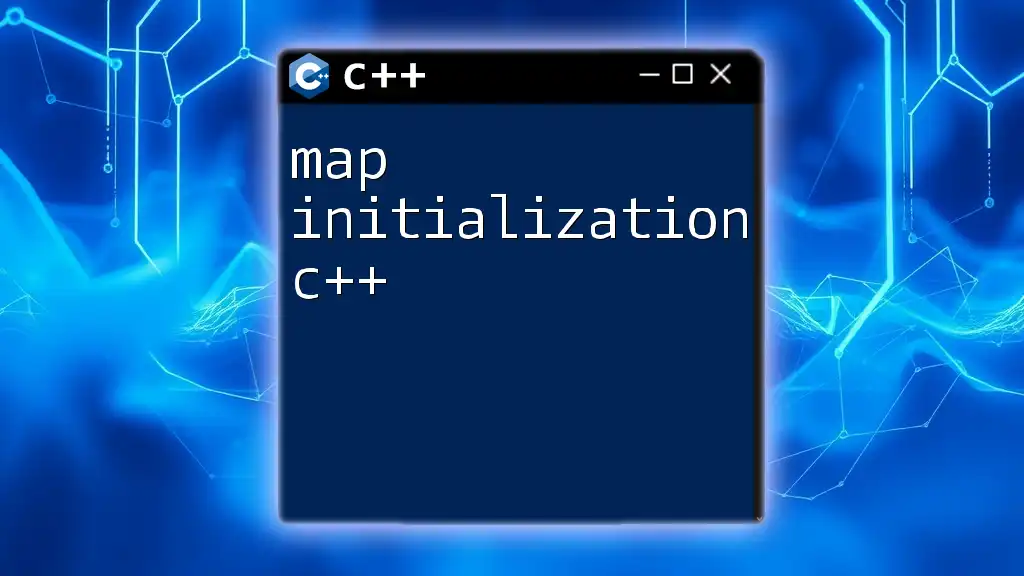
Conclusion
C++ aggregate initialization enhances both the efficiency and readability of code. By employing this technique, developers can streamline their workflow, quickly set up complex structures, and maintain clearer codebases. Understanding when and how to use this feature is essential for any C++ programmer striving for excellence.
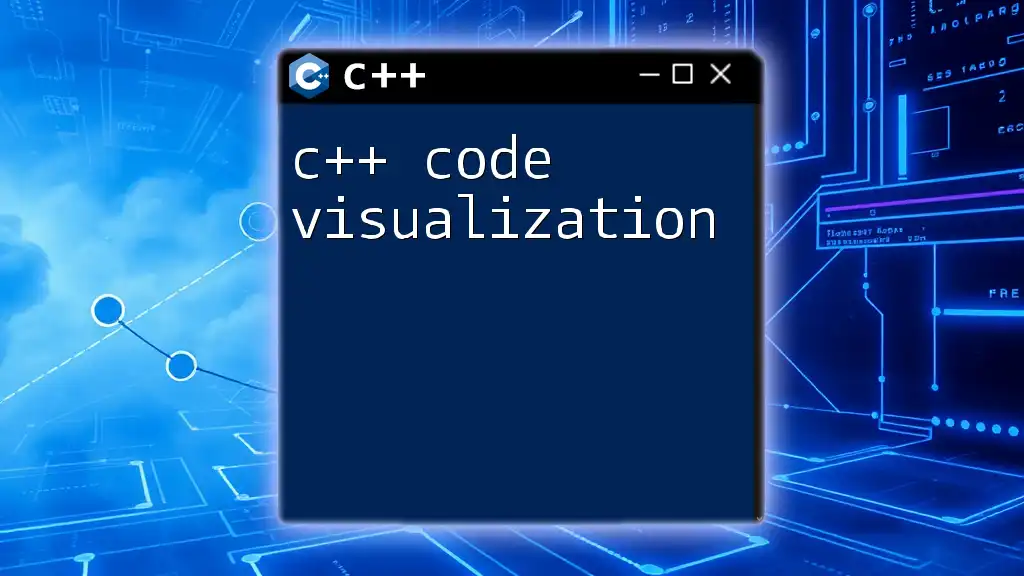
FAQs
What is the difference between aggregate initialization and list initialization?
Aggregate initialization primarily pertains to initializing aggregate types succinctly using braces. In contrast, list initialization allows for the initialization of any type using a similar syntax but may include additional features like narrowing conversions and type safety checks.
Can I initialize class types using aggregate initialization?
No, aggregate initialization is limited to aggregate types like structs and arrays. Classes that have user-defined constructors or private members cannot utilize this initialization method.
Are there any pitfalls to avoid with aggregate initialization?
Common pitfalls include attempting to use aggregate initialization for types that do not qualify (like classes with user-defined constructors) and misunderstanding brace elision behavior, leading to potentially uninitialized values in your data structures.
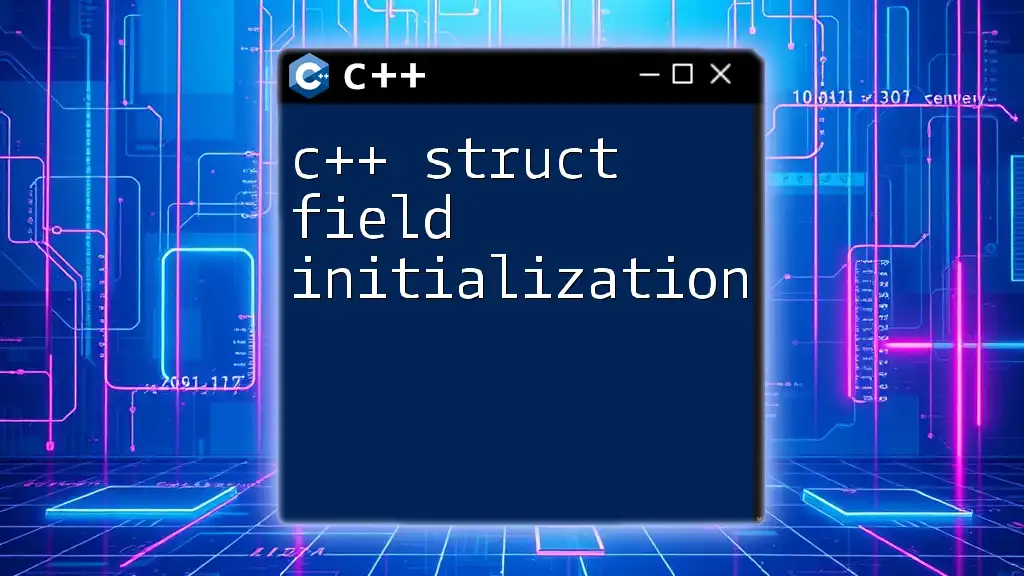
Further Reading
To deepen your understanding of C++ initialization techniques, consider exploring resources such as books on advanced C++ programming, online courses focused on C++ standards, and official C++ documentation detailing initialization methods and their applications.