In C++, an "illegal instruction" typically occurs when the program attempts to execute a CPU instruction that is not valid for the current type of processor or is improperly formed due to corrupted or invalid code.
Here's a code snippet that demonstrates an illegal instruction by trying to dereference a null pointer:
#include <iostream>
int main() {
int* ptr = nullptr; // Pointer initialized to null
std::cout << *ptr; // This line attempts to dereference the null pointer, potentially leading to an illegal instruction
return 0;
}
Understanding Illegal Instructions in C++
What is an Illegal Instruction?
An illegal instruction is an error that occurs when a program attempts to execute a command that the CPU does not recognize or cannot perform. This can lead to undefined behavior, crashes, or abnormal halting of the program. It is essential to differentiate illegal instructions from other error types, such as segmentation faults, which involve memory access violations.
Common Causes of Illegal Instructions in C++
Understanding what leads to C++ illegal instruction errors can help prevent them. Here are the main culprits:
-
Invalid CPU Operations: These occur when the executed instruction is not supported by the CPU architecture. This can happen due to incompatibility between the code and the CPU.
-
Uninitialized Pointers: Using pointers that haven’t been assigned valid memory addresses can easily trigger illegal instructions. This results in attempts to dereference or execute invalid memory locations.
-
Outdated or Incorrect Compiler Configuration: Using outdated or improperly configured compilers can lead to the generation of code that isn't suitable for the target architecture, thus causing illegal instructions.
-
Hardware Issues: Rarely, underlying hardware problems such as a malfunctioning CPU can lead to illegal instructions being executed, although this is not a common case.
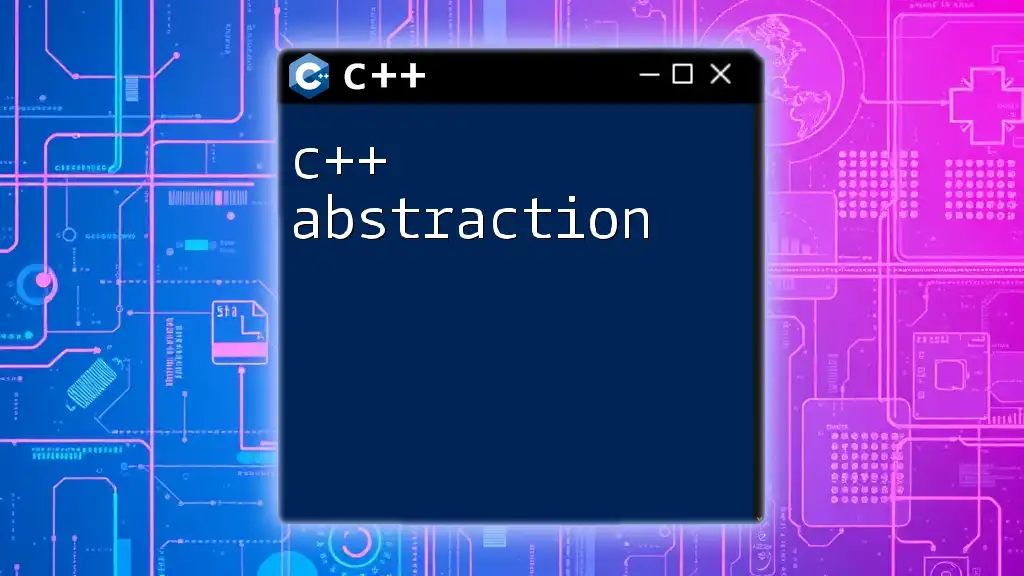
Recognizing Illegal Instruction Errors
Symptoms of Illegal Instruction Errors
When your program encounters an illegal instruction, it may exhibit specific symptoms, including:
- The program crashes immediately with an error message.
- The CPU might raise an exception signal, often resulting in messages like "Illegal instruction" on various platforms (such as SIGILL in Unix-like systems).
- In some cases, it might lead to a Core Dump, where the system saves the program's memory at the time of the crash for debugging purposes.
You might see different error messages on various operating systems, such as `Illegal instruction (core dumped)` on Linux or `Unhandled Exception: Illegal Instruction` on Windows environments.
Debugging Illegal Instructions
Debugging an illegal instruction error involves using tools and interpreting outputs effectively:
-
Using Debuggers: Tools like GDB (GNU Debugger) can help track down illegal instructions. Start your program within GDB, and when the error occurs, inspect the backtrace with the `bt` command. This will indicate where the illegal instruction was triggered.
-
Log Outputs: Make use of logging to catch unexpected behavior before crashing. Analyzing logs can help in isolating the segment in code that led to the illegal instruction.
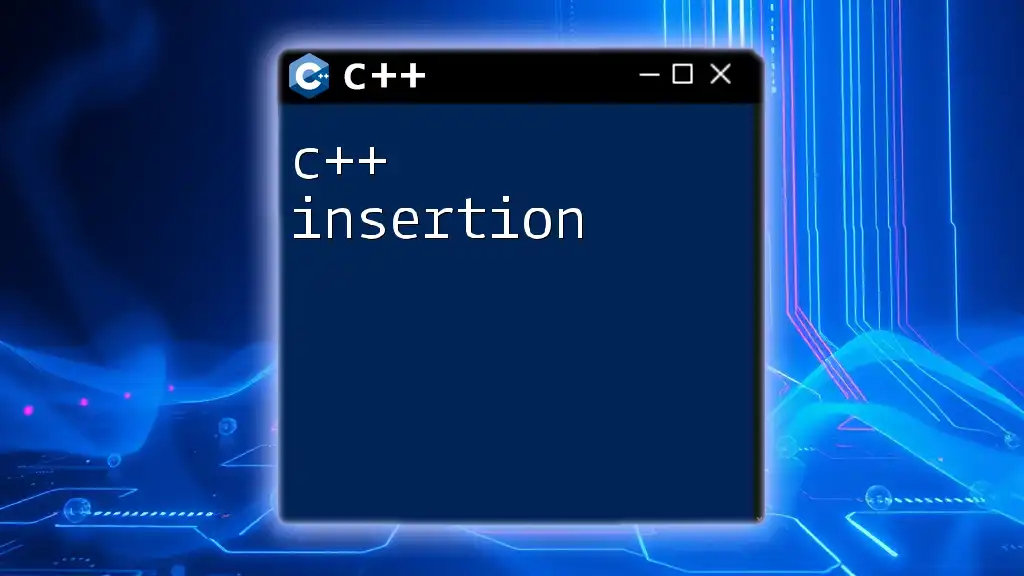
Detailed Examples
Example 1: Invalid CPU Operations
Consider the following code snippet:
#include <iostream>
int main() {
int* ptr = (int*)0xFFFFFFFF; // Deliberately setting an invalid address
std::cout << *ptr; // This can lead to an illegal instruction
return 0;
}
In this example, an invalid memory address is cast and dereferenced. This code attempts to access an address that doesn't map to any valid memory, leading to an illegal instruction when the CPU tries to execute it. It’s critical to ensure that pointer arithmetic and address manipulation are done carefully.
Example 2: Uninitialized Pointers
Another relevant example involves uninitialized pointers:
#include <iostream>
int main() {
int* ptr; // Uninitialized pointer
std::cout << *ptr; // Dereferencing with an uninitialized pointer
return 0;
}
Here, `ptr` is declared but not initialized. Dereferencing this pointer results in undefined behavior, which can include executing an illegal instruction. It’s a recommended practice to initialize pointers to `nullptr` to avoid such scenarios and ensure program stability.
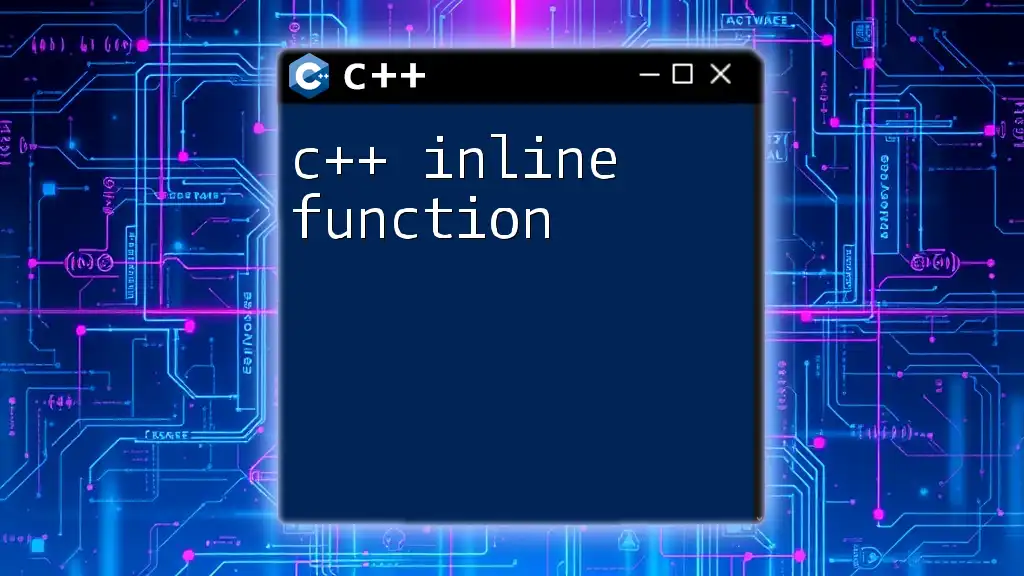
Prevention Techniques
Best Practices in C++ to Avoid Illegal Instructions
Avoiding C++ illegal instruction issues can be achieved through several best practices:
-
Always Initialize Pointers: Ensuring that pointers are properly initialized can save developers from a wide range of issues. Assigning them to `nullptr` if not set can help in identifying invalid accesses.
-
Use Smart Pointers: Leveraging smart pointers (like `std::unique_ptr` and `std::shared_ptr`) can manage memory automatically and significantly reduce the risk of illegal instructions due to erroneous memory access.
-
Proper Compiler Flags: Utilizing flags for warnings and optimizations during compilation (like `-Wall`, `-Wextra`, and `-O2` in GCC) can help catch potential issues before runtime.
Maintaining Your System
Keeping your system up to date is vital. Here are a few tips:
-
Regularly Update Software: Always use the latest versions of compilers and libraries that could affect your program's execution.
-
Check Hardware Compatibility: Ensure that the hardware you’re working with supports all the features you're using in your code.
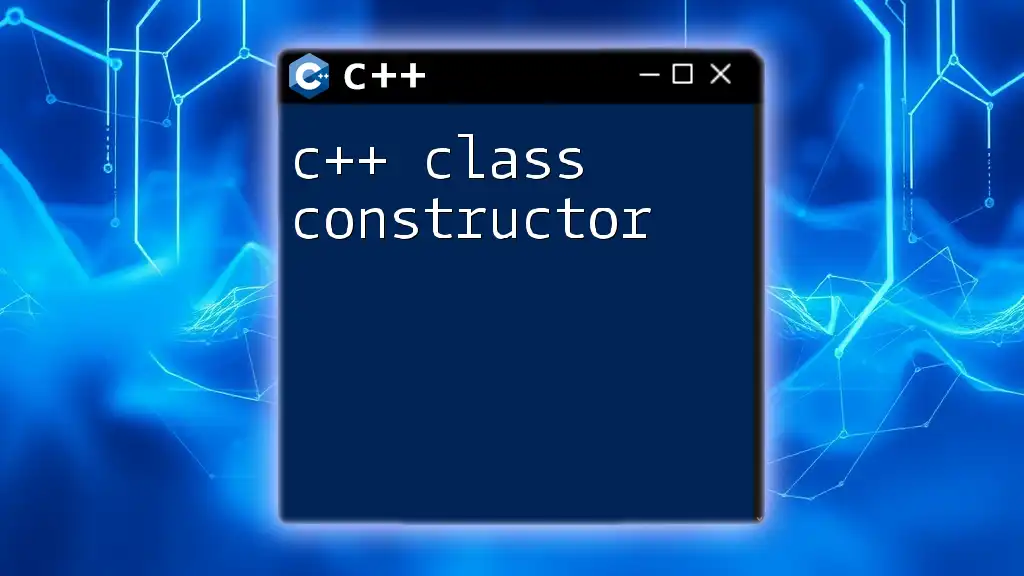
Conclusion
A clear understanding of C++ illegal instruction errors—what causes them, how to recognize them, and how to prevent and debug them—will empower developers to write more reliable and robust C++ programs. By adhering to best practices, diligently debugging, and maintaining an updated environment, programmers can reduce the likelihood of encountering these disruptive errors.