C++ serialization is the process of converting an object into a format that can be easily stored or transmitted and then reconstructed later, typically using a stream.
Here’s a simple example demonstrating serialization using `std::ofstream`:
#include <iostream>
#include <fstream>
#include <string>
class Person {
public:
std::string name;
int age;
// Serialize function
void serialize(const std::string& filename) {
std::ofstream ofs(filename);
ofs << name << '\n' << age;
ofs.close();
}
// Deserialize function
void deserialize(const std::string& filename) {
std::ifstream ifs(filename);
std::getline(ifs, name);
ifs >> age;
ifs.close();
}
};
int main() {
Person p1;
p1.name = "Alice";
p1.age = 30;
p1.serialize("person.txt");
Person p2;
p2.deserialize("person.txt");
std::cout << "Name: " << p2.name << ", Age: " << p2.age << std::endl;
return 0;
}
Understanding C++ Serialization
What is Serialization?
Serialization is the process of converting an object into a format that can be easily stored or transmitted and then reconstructed later. In the context of C++, it involves transforming an object into a sequence of bytes. These bytes can then be saved to a file, sent over a network, or used in inter-process communication.
This fundamental process is crucial for enabling data persistence and seamless communication between different parts of an application or different applications altogether.
Why Use Serialization?
The benefits of C++ serialization are vast:
- Data Persistence: Serialization allows objects to be saved to files or databases, enabling applications to store and retrieve state across sessions.
- Inter-process Communication: When processes need to exchange data, serialization formats provide a common ground for object representation, ensuring both parties correctly interpret the data.
- Data Interchange Formats: Many web services and APIs use serialized data formats, such as JSON or XML, making serialization key to modern software development.

Types of Serialization Techniques
Built-in Serialization
C++ offers basic serialization functionality for built-in types. This type of serialization is straightforward and involves converting fundamental data types like `int`, `float`, and `string` into a storable format. C++ handles serialization of these types directly through simple methods.
For instance, you can serialize an integer by converting it into a string:
int num = 42;
std::string serialized = std::to_string(num); // Serializing int to string
Custom Serialization
What is Custom Serialization?
Custom serialization refers to the process of defining your serialization mechanism for more complex or user-defined types. It empowers developers to control how their objects are transformed into a storable format and vice versa.
Creating Serializable Classes in C++
To create a serializable class, you must define a way to transform its data members into a storable format. Here is a simple example showcasing a `Person` class:
class Person {
public:
std::string name;
int age;
// Serialization function
std::string serialize() {
return name + "|" + std::to_string(age);
}
// Deserialization function
void deserialize(const std::string &data) {
size_t delimiter_pos = data.find('|');
name = data.substr(0, delimiter_pos);
age = std::stoi(data.substr(delimiter_pos + 1));
}
};
In this case, the `serialize` method concatenates the name and age of the person into a single string, while the `deserialize` method splits that string back into the object's original attributes.
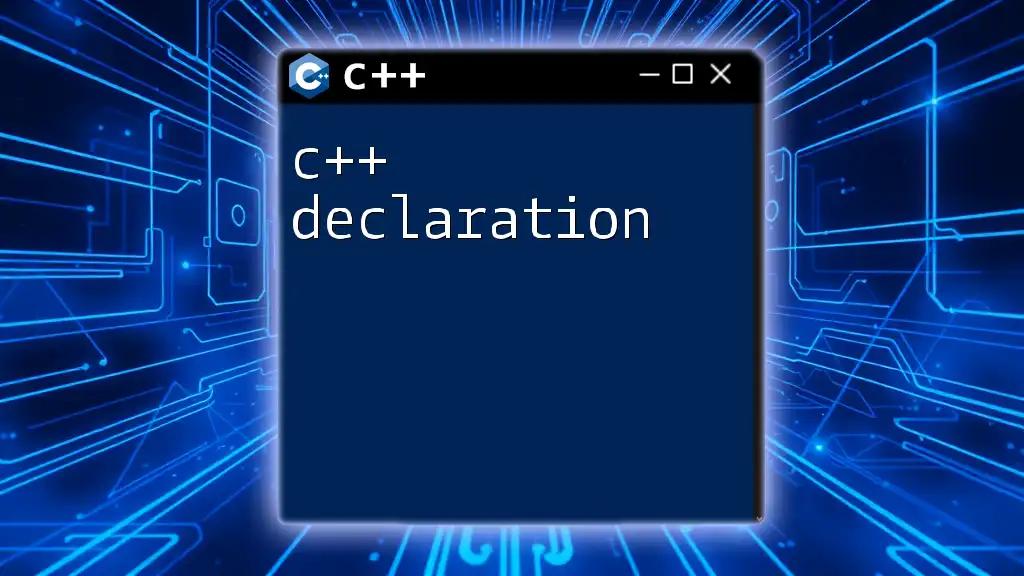
Implementing C++ Serialization
Using Boost Serialization Library
The Boost Serialization Library is a powerful tool for managing serialization in C++. Unlike manual serialization methods, Boost provides a comprehensive framework that simplifies the process.
To get started, ensure that Boost is installed in your development environment. This can typically be done through package managers or by downloading directly from the Boost website.
Example: Streaming Data with Boost
Here is an example demonstrating how to create a serializable `Person` class using Boost:
#include <boost/archive/text_oarchive.hpp>
#include <boost/archive/text_iarchive.hpp>
#include <fstream>
class Person {
public:
std::string name;
int age;
// Boost Serialization
template<class Archive>
void serialize(Archive & ar, const unsigned int version) {
ar & name;
ar & age;
}
};
By integrating Boost serialization, developers can save and load objects straightforwardly—allowing for rich data management capabilities with minimal coding overhead.
Serialization with Protocol Buffers
Protocol Buffers (protobuf) is a method developed by Google for serializing structured data. It is an efficient alternative to traditional formats such as XML and JSON, known for its speed and small size.
To use Protocol Buffers, start by defining your data structure in a `.proto` file. After generating source files using the Protocol Buffers compiler, you can write C++ code to serialize and deserialize your data.
Example of Protocol Buffers Implementation
Here’s how you might define a `Person` message in a `.proto` file:
syntax = "proto3";
message Person {
string name = 1;
int32 age = 2;
}
Once you've compiled this file, you can serialize and deserialize `Person` objects easily in your C++ application, significantly reducing the complexity compared to manual serialization techniques.
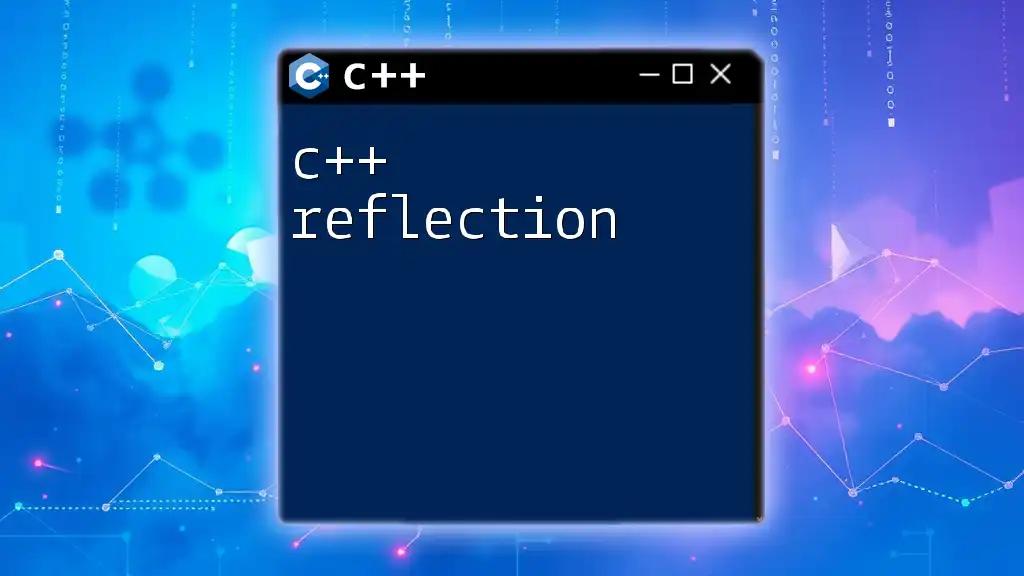
Best Practices for Serialization in C++
Efficiency Considerations
Efficiency is a critical aspect of serialization. Optimizing performance starts with choosing suitable data structures. Here are some tips:
- Utilize primitive data types as much as possible for quicker serialization.
- Avoid deep copy serializations when referencing shared resources.
Versioning Serialized Data
When evolving a software application, having a robust versioning strategy for serialized data is essential. This ensures backward compatibility and prevents potential data corruption. Utilize techniques like adding a version number to your serialized output and implementing logic to handle new and deprecated fields appropriately.
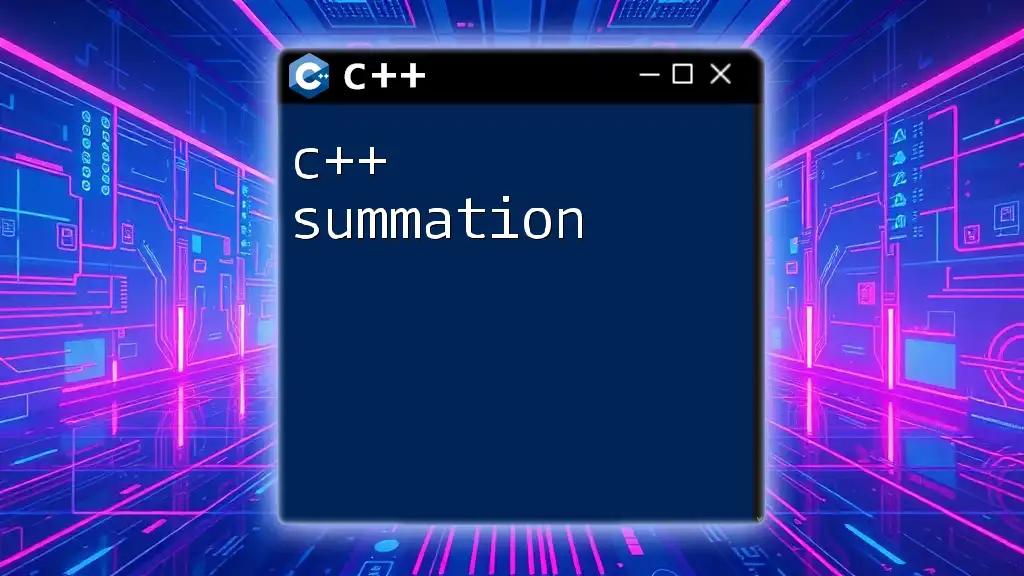
Common Pitfalls in C++ Serialization
Dealing with Pointers and Dynamic Memory
One of the most significant challenges in serialization is managing pointers and dynamically allocated memory. Serialized data does not inherently carry metadata about object types or memory locations, which can lead to misinterpretation during deserialization.
To address this, consider using smart pointers or managing the object's lifecycle rigorously. Also, avoid serializing raw pointers directly—serialize the data they point to instead.
Error Handling
Serialization can come with a host of potential errors. Some common issues include:
- Data Corruption: When the serialization format mismatches the expectations upon deserialization.
- File I/O Errors: When reading from or writing to files, errors can occur due to permission issues, read-write conflicts, etc.
Implement thorough error handling to catch these issues early, allowing for debugging and providing user-friendly feedback.
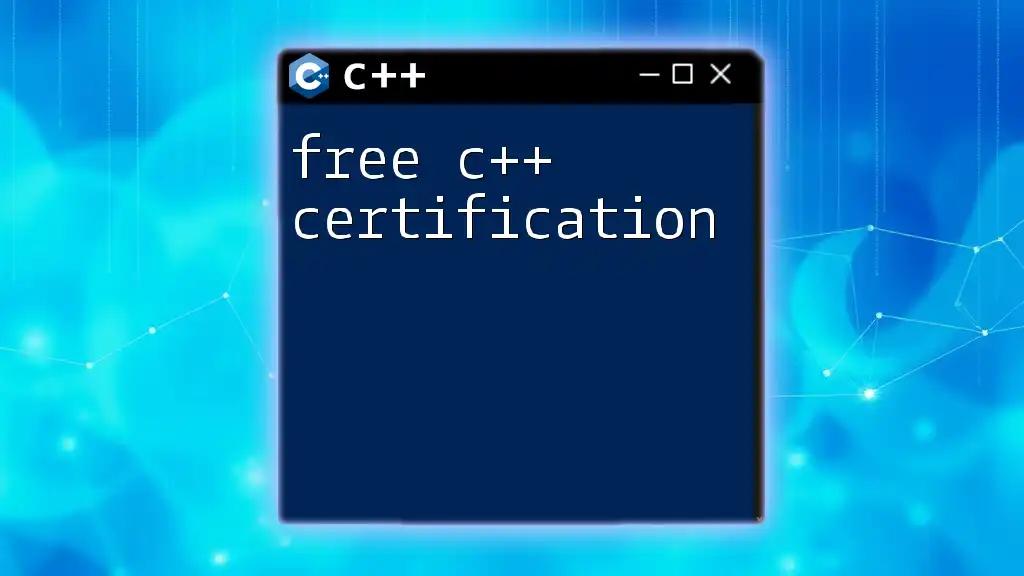
Conclusion
In summary, C++ serialization is a powerful tool that facilitates data persistence, communication, and interchange. By understanding serialization methods—ranging from built-in types to advanced libraries like Boost and Protocol Buffers—developers can efficiently handle complex data structures in their applications.
As you practice and dive deeper into serialization techniques, you’ll appreciate its significance and flexibility in C++ development. Whether you are building desktop applications, networked services, or custom data formats, mastering serialization is crucial, paving the way for innovative solutions and enhanced user experiences.