A C++ translation unit is the result of the compilation process for a single source file, which includes the source code along with the headers and other files it includes, and it is ultimately compiled into object code.
Here's a simple code snippet example of a translation unit:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Translation Units
What is a Translation Unit?
A translation unit (TU) in C++ is the fundamental building block of the compilation process. It is defined as the source code resulting from the preprocessing of a source file. A translation unit includes the original source code file, any included header files, and any preprocessor directives.
Understanding TUs is essential, as they play a crucial role in how the C++ compiler processes and compiles code. Each translation unit is treated independently during the compilation phase, which is why C++ allows for modular programming.
Components of a Translation Unit
A translation unit primarily comprises three components:
- Source File Contents: This includes the actual code written in C++ (.cpp files).
- Header Files: Header files (.h or .hpp) often contain declarations of functions, classes, or templates, which can be reused across multiple translation units.
- Preprocessor Directives: These are commands that direct the compiler's preprocessor to include files or define macros (e.g., `#include`, `#define`, etc.).
Understanding these components helps in organizing code effectively and ensures that the compilation process runs smoothly.
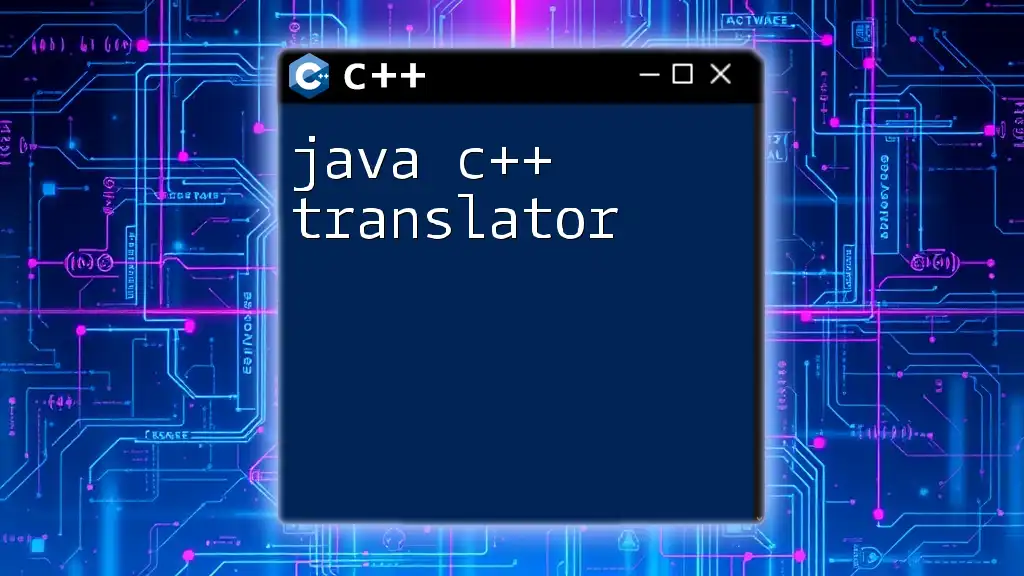
The Role of Translation Units in the Compilation Process
The Compilation Phases
The C++ compilation process can be broadly categorized into four phases:
-
Preprocessing: This phase handles all preprocessor directives. The preprocessor expands macros, includes header files, and performs conditional compilation.
-
Compilation: During this phase, the compiler translates the preprocessed code into assembly language specific to the target architecture.
-
Assembly: Here, the assembler converts the assembly code into machine code (binary).
-
Linking: In the final phase, the linker combines all the object files generated from each translation unit into a single executable.
How Translation Units Fit in Each Phase
A translation unit plays a significant role particularly during the preprocessing and compilation phases. During preprocessing, the source file along with any included header files is merged into a single file, which then becomes the input for the compilation phase.
For instance, consider the following example:
// main.cpp
#include "myheader.h"
int main() {
return add(5, 3);
}
Here, `main.cpp` is a translation unit that includes `myheader.h`. In the preprocessing phase, the content of `myheader.h` will be merged into `main.cpp`, creating a single translation unit. The compiler then processes this merged content to produce object code.
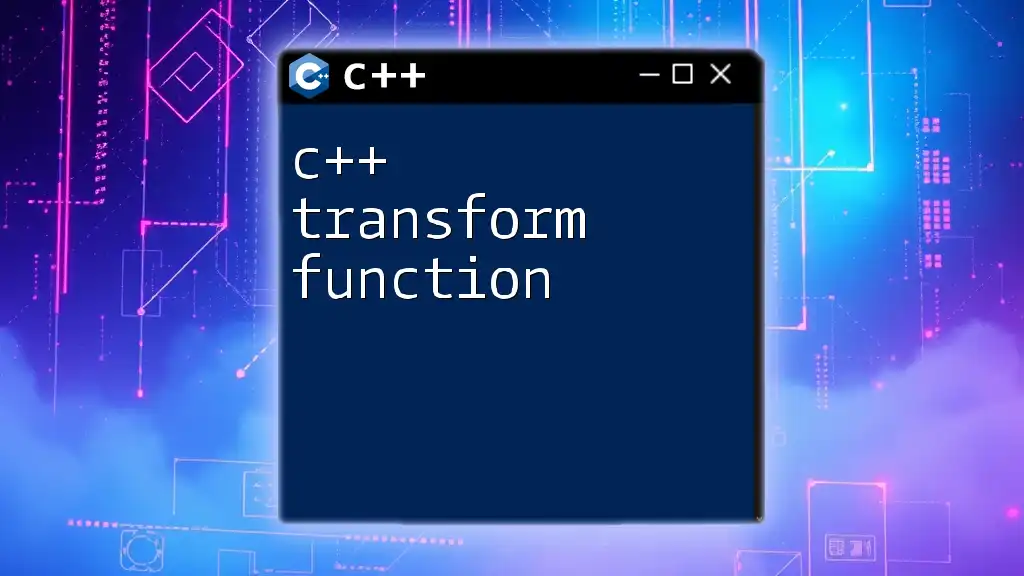
Creating a Translation Unit
Writing a Basic Source Code File
To create a translation unit, the process begins with writing a C++ source code file. Let's look at a simple example:
// example.cpp
#include <iostream>
void greet() {
std::cout << "Hello, World!" << std::endl;
}
In this case, `example.cpp` serves as a translation unit. When compiled, it becomes an independent entity, generated from the source code present in the file.
Including Header Files
Header files are an essential part of translation units. By including header files, you can share common declarations across multiple translation units.
For example, consider the following `myheader.h` file:
// myheader.h
#ifndef MYHEADER_H
#define MYHEADER_H
int add(int a, int b) {
return a + b;
}
#endif // MYHEADER_H
When you include this header file in another source file, the functions defined in it become part of that translation unit, facilitating code reusability.
Preprocessor Directives and Their Effects
Preprocessor directives can significantly impact translation units. Common directives such as `#define` and `#ifdef` allow for the inclusion or exclusion of code portions based on certain conditions.
For example:
#define PI 3.14
#ifdef DEBUG
#include <iostream>
#endif
Here, `#define PI 3.14` creates a macro that will replace instances of `PI` with `3.14` throughout the translation unit, while `#ifdef DEBUG` allows for conditional inclusion of debugging code.
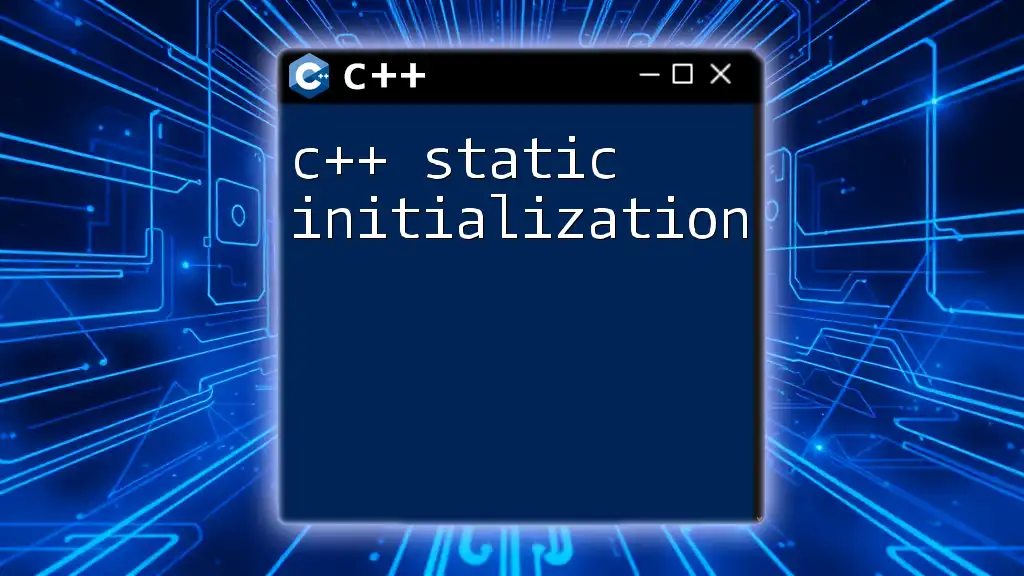
Compilation Considerations
Handling Multiple Translation Units
C++ programs often consist of multiple translation units, which can enhance modularity. Each source file can be compiled independently and later linked together. This approach means that you can work on individual files without recompiling the entire project.
For example, consider the structure of a simple project:
project/
├── main.cpp
├── utils.cpp
└── utils.h
This layout shows a project with three translation units: `main.cpp`, `utils.cpp`, and `utils.h`. Modules are encapsulated effectively, promoting better organization and maintainability.
Managing Declarations and Definitions
The management of declarations and definitions is vital in avoiding common pitfalls. In C++, if the same function or variable is defined multiple times across translation units, it leads to linker errors. To manage this, the `extern` keyword can be used to declare a variable without defining it.
For example:
// declaration in utils.h
extern int globalVar;
// definition in utils.cpp
int globalVar = 5;
In this scenario, `globalVar` is declared in the header file (thus making it visible across translation units) but is defined only once in `utils.cpp`, adhering to the One Definition Rule (ODR).
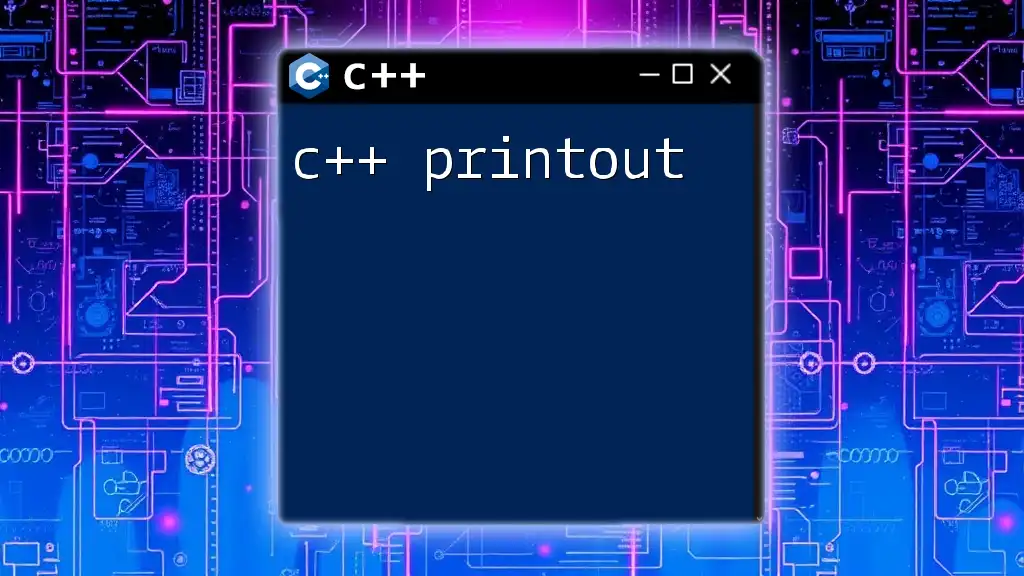
Debugging and Analyzing Translation Units
Tools for Analyzing Translation Units
Various tools are available for analyzing translation units, including `gcc` and `clang`.
For example, you can use the `gcc -E` command to view the preprocessed output of a translation unit:
gcc -E example.cpp -o preprocessed_output.cpp
This command allows you to inspect how the preprocessed translation unit looks, revealing how header files and macros are resolved before the actual compilation phase begins.
Common Issues with Translation Units
Handling translation units can sometimes lead to complications, such as duplicate definitions. A common issue arises when the same function or object is accidentally defined in multiple translation units, leading to ambiguity during linking. Careful structuring of headers and using include guards can prevent these errors.
For example, include guards prevent multiple inclusions of header files:
#ifndef MYHEADER_H
#define MYHEADER_H
// Header content
#endif // MYHEADER_H
By adopting good practices, you can minimize the likelihood of encountering linker errors related to translation units.
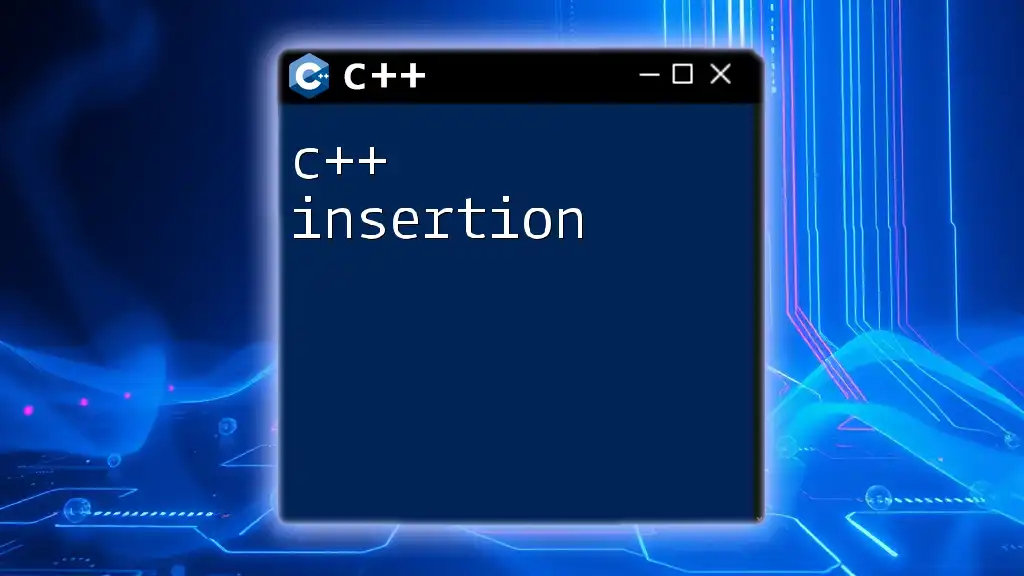
Best Practices for Working with Translation Units
Organizing Code into TUs
Organizing your code into well-structured translation units improves readability and maintainability. It’s advisable to keep related classes and functions together while separating distinct functionalities into different files.
Maintaining Header Files
Writing and maintaining header files is crucial for smooth compilation. Always ensure you use include guards and that each header file contains only declarations, not definitions. This practice allows for multiple inclusion without redefining variables or functions.
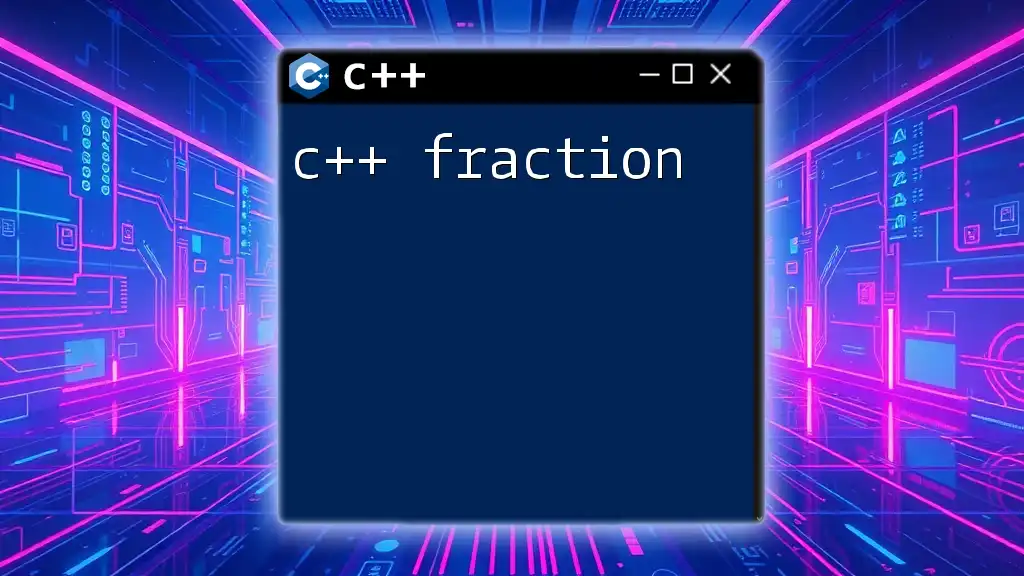
Conclusion
Understanding the concept of a C++ translation unit is fundamental to mastering the compilation process in C++. By knowing how TUs work, how to create them, and how they function within the broader compilation framework, you can write more efficient and modular C++ code. As you implement best practices, you will find that managing translation units can enhance your programming experience. For further exploration, consider diving into more advanced resources and tools dedicated to C++ development.