The C++ `transform` function is a powerful algorithm that applies a specified operation to a range of elements, transforming them into a new range based on a provided function.
Here's a simple example using the `transform` function to convert a vector of integers into their squares:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::vector<int> result(vec.size());
std::transform(vec.begin(), vec.end(), result.begin(), [](int x) { return x * x; });
for (int val : result) {
std::cout << val << " ";
}
return 0;
}
What is the Transform Function in C++?
The C++ transform function is a powerful algorithm included in the C++ Standard Library, specifically within the `<algorithm>` header. It is designed to transform elements in a range by applying a specified operation. While it aligns with the principles of functional programming, it can be utilized in various programming paradigms by facilitating element-wise transformations.
This function is particularly useful when enhancing code clarity and reducing the need for explicit loops. By encapsulating transformation logic into a single call, it not only improves readability but also promotes a more efficient coding style.
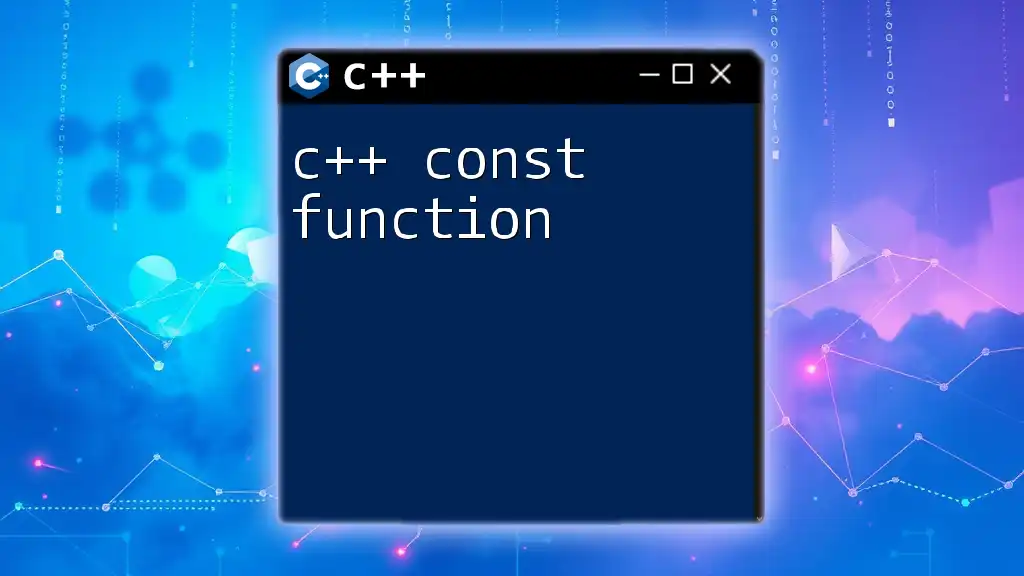
Syntax of the Transform Function
The syntax for the C++ transform function is elegantly crafted to accommodate both unary and binary operations:
std::transform(InputIt first1, InputIt last1, OutputIt d_first, UnaryOperator op);
std::transform(InputIt first1, InputIt last1, InputIt first2, OutputIt d_first, BinaryOperator op);
Explanation of Parameters
- InputIt first1, last1: These parameters define the beginning and end of the input sequence. They tell the function which elements to transform.
- OutputIt d_first: This is the starting point for where the results will be stored. Whether it's a vector, list, or any other output container, this iterator needs to point to a valid position where results can be written.
- UnaryOperator op: For unary transformations, this is a function or function object applied to each element in the input range. Common examples can include lambda expressions or pre-defined functions.
- BinaryOperator op: This parameter is relevant for binary transformations and represents the function or operation combining two corresponding elements from two ranges.
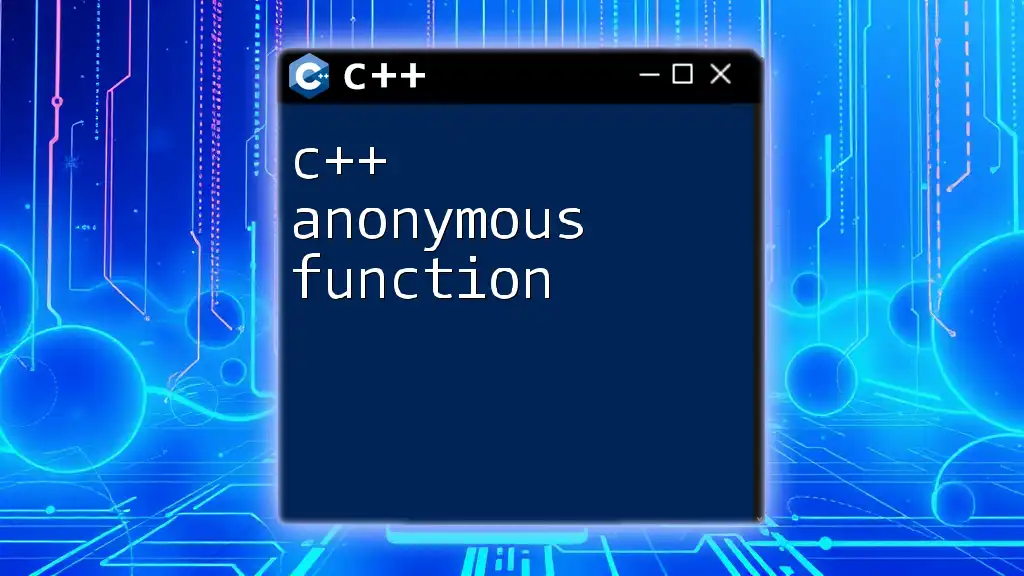
Using the Transform Function: A Step-by-Step Guide
Simple Example of Unary Transform
Let’s begin with a basic example where we aim to square each element in a vector. This showcases the C++ transform function in action.
Code Example:
#include <iostream>
#include <vector>
#include <algorithm>
int square(int x) {
return x * x;
}
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::vector<int> result(vec.size());
std::transform(vec.begin(), vec.end(), result.begin(), square);
for(int i : result) {
std::cout << i << " "; // Output: 1 4 9 16 25
}
return 0;
}
In this example, we define a simple function `square` that takes an integer and returns its square.
- We start with an original vector, `vec`, initialized with integers.
- A `result` vector of the same size is created to store the transformed values.
- The transform function is invoked, applying the `square` operation across the original vector, yielding a new vector containing the squared values.
- Finally, we output the transformed values in the console.
Example of Binary Transform
Now, let’s explore a scenario where we combine two vectors element-wise using the C++ transform function. This illustrates how we can perform operations that depend on multiple inputs.
Code Example:
#include <iostream>
#include <vector>
#include <algorithm>
int add(int a, int b) {
return a + b;
}
int main() {
std::vector<int> vec1 = {1, 2, 3};
std::vector<int> vec2 = {4, 5, 6};
std::vector<int> result(vec1.size());
std::transform(vec1.begin(), vec1.end(), vec2.begin(), result.begin(), add);
for(int i : result) {
std::cout << i << " "; // Output: 5 7 9
}
return 0;
}
In this scenario, we have two vectors: `vec1` and `vec2`, each containing integers. We want to produce a new vector `result` that holds the sums of corresponding elements from the two vectors.
- The function `add` sums two integers and is used as the binary operation in `std::transform`.
- The resulting `result` vector contains the sums of the pairs (1+4, 2+5, and 3+6), which is then printed out.
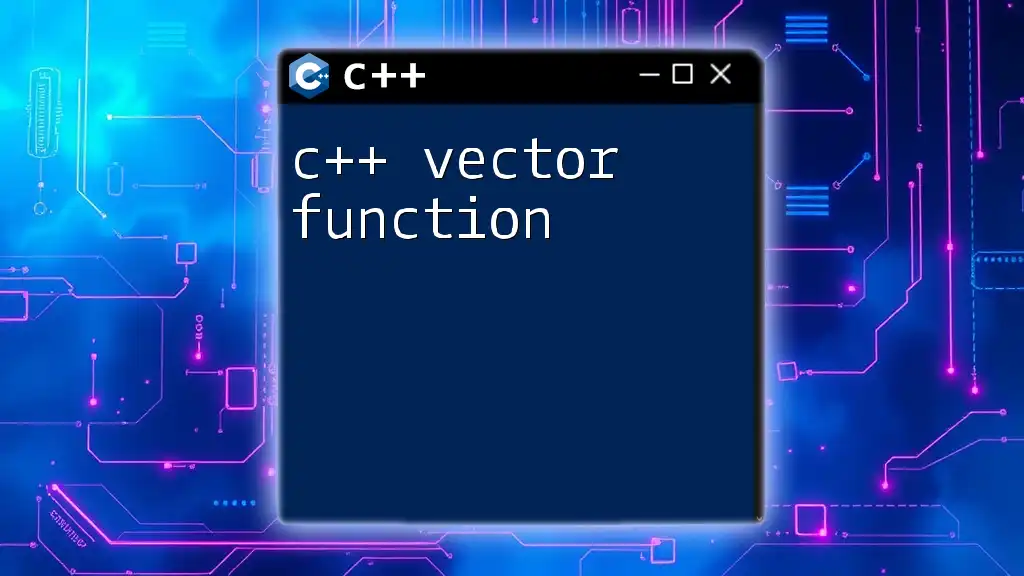
Performance Considerations
When using the C++ transform function, it's important to consider its efficiency:
- The time complexity of the transform function is O(n), where n is the number of elements being transformed. This means that it scales linearly with the input size, making it efficient for a wide range of data sizes.
- Memory considerations also come into play. In scenarios where the output vector isn’t needed separately, you can use in-place transformations using the same vector, saving additional memory allocation overhead.
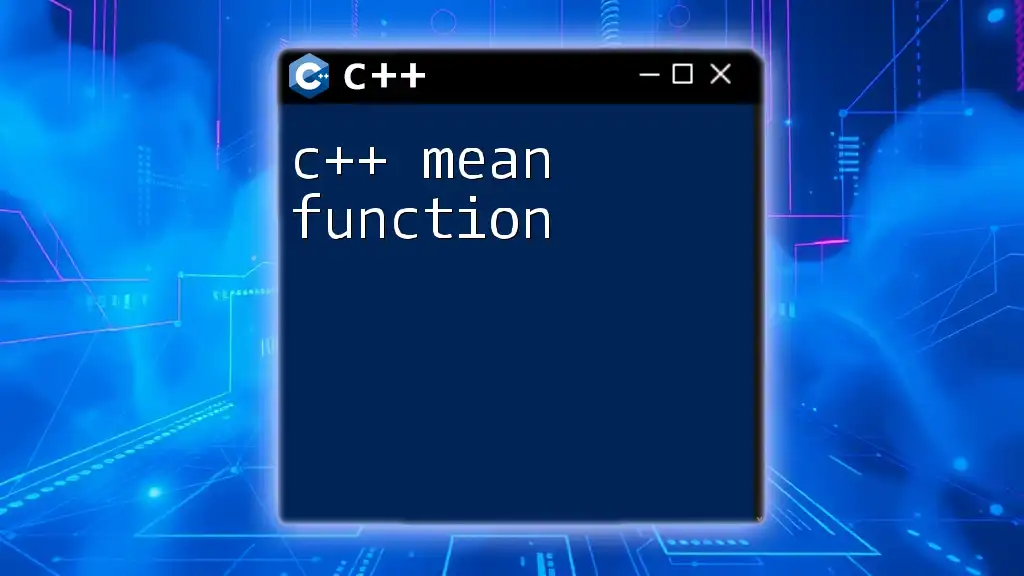
When to Use Transform Function
There are numerous situations where the C++ transform function shines:
- Unary Transformations: These are particularly useful when you need to apply a single operation to each element in a collection, such as applying a scale factor or adjusting values.
- Binary Transformations: Ideal for combining data from two associated datasets, such as syncing properties or merging two lists of updates. This allows for cleaner, more expressive code compared to traditional loops.
Common Mistakes to Avoid
While using the C++ transform function, programmers often make a few common mistakes:
- Forgetting to Resize the Output Vector: Ensure that your output vector is appropriately sized to hold the results. If your output iterator points to an uninitialized area, the code can throw runtime errors.
- Confusing Unary and Binary Transforms: Be clear on whether your operation requires one or two input sequences; mixing these configurations can lead to unexpected behavior and compile-time errors.
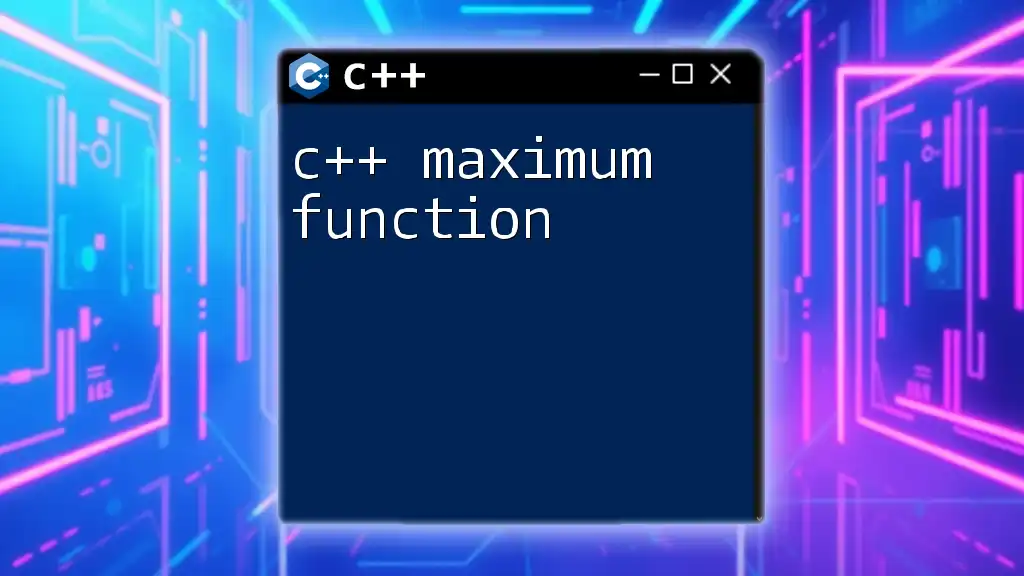
Conclusion
In this guide, we delved into the C++ transform function, examining its syntax, applications, and best practices for efficient usage in code. Mastering this function not only enhances your coding skills but also equips you with tools to write cleaner, more maintainable code.
To further deepen your understanding, consider experimenting with real-world scenarios and transformations, exploring increasingly complex use cases in your projects. The C++ transform function is an invaluable asset in any C++ programmer's toolkit.
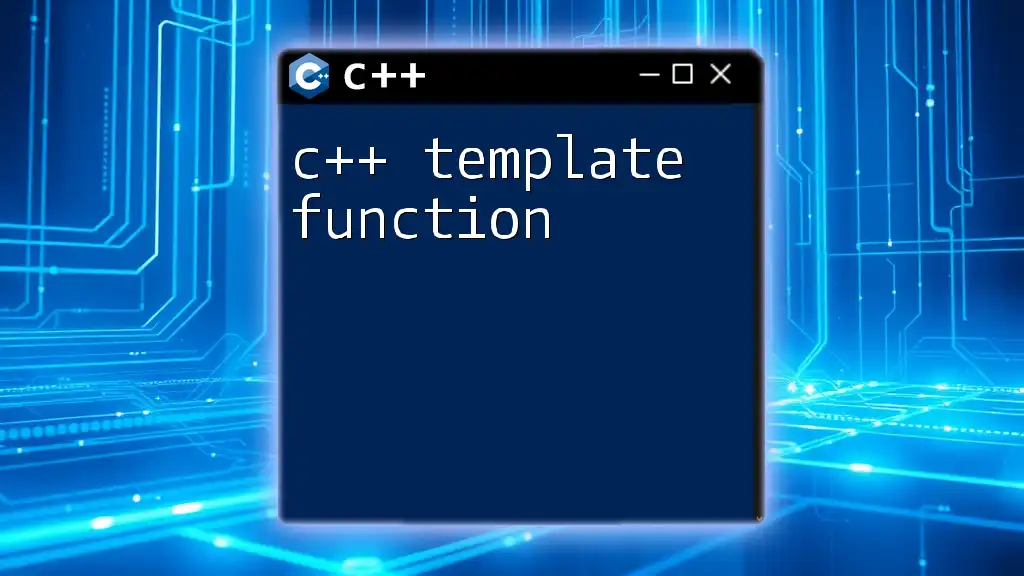
Additional Resources
For those eager to explore the topic further, refer to the C++ Standard Library documentation and various reputable books and tutorials focused on C++. Engage with online communities and forums for practical insights and advice from experienced C++ practitioners.