An anonymous function in C++ is a function that has no name and is often used in conjunction with algorithms or as arguments to other functions, allowing for concise definition and execution of short pieces of code.
Here's a simple code snippet demonstrating an anonymous function in C++ using a lambda expression:
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::for_each(numbers.begin(), numbers.end(), [](int n) {
std::cout << n * n << " ";
});
return 0;
}
Introduction to Anonymous Functions
C++ anonymous functions, commonly known as lambda functions, represent a powerful feature in modern C++ programming. They allow developers to create function-like entities without the need for explicitly naming them, thus enabling a more concise and flexible coding style. This guide explores the nuances of these functions and how to effectively utilize them in your C++ projects.
Why Use Anonymous Functions?
Utilizing anonymous functions in your code comes with numerous benefits:
- Conciseness: They allow you to define functions in place where they are needed, reducing the need for separate declarations.
- Readability: Code that employs anonymous functions can be easier to understand at a glance since the function's implementation often accompanies its use.
- Flexibility: Anonymous functions make it easy to create small, one-off functions for use in algorithms or as callbacks, steering clear of redundant function definitions.
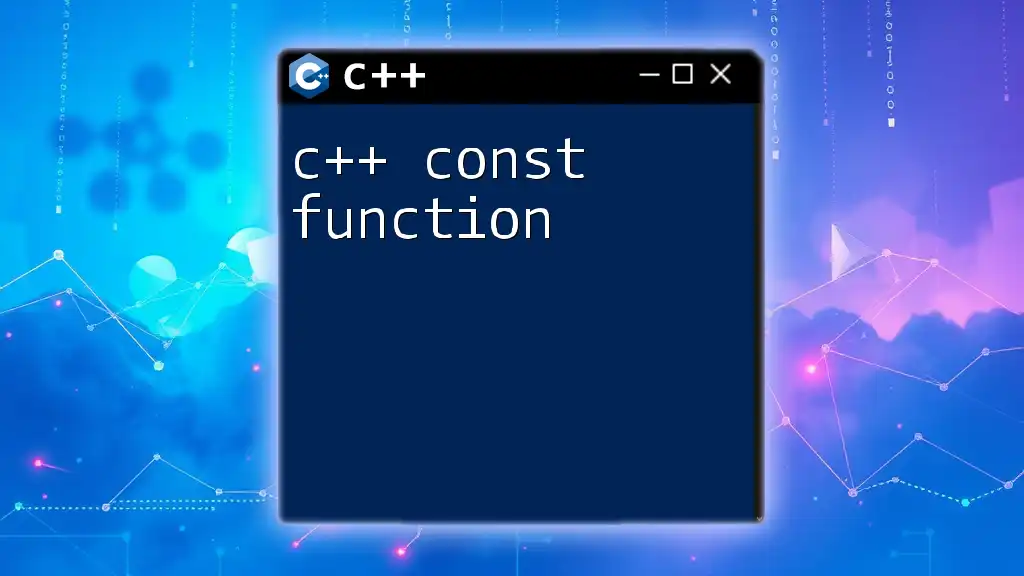
Understanding the Syntax of C++ Lambda Functions
Basic Syntax
To appreciate C++ anonymous functions, it’s essential to understand their syntax. A lambda function's structure can be broken down as follows:
[capture_clause](parameters) -> return_type { function_body }
Each component serves a specific role in defining the behavior of the lambda.
Components of a Lambda Function
Capture Clause
The capture clause determines how the lambda function accesses variables from its surrounding scope. There are two main ways to capture variables:
-
By Value: This creates a copy of the variable.
int x = 10; auto lambda1 = [x] { return x + 1; }; // captures x by value
-
By Reference: This allows the lambda to manipulate the original variable.
int y = 20; auto lambda2 = [&y] { return ++y; }; // captures y by reference
This distinction is crucial because it affects both the functionality of the lambda and the performance of your program.
Parameters
Next, the parameters section specifies any inputs the lambda function requires. For example:
auto add = [](int a, int b) { return a + b; }; // a and b are parameters
This allows you to pass arguments to the lambda just like you would with any function.
Return Type
In most cases, the return type can be inferred by the compiler. However, you may need to specify it explicitly in certain scenarios, particularly for complex calculations:
auto multiply = [](double a, double b) -> double { return a * b; };
Assigning a return type aids in maintaining clarity, especially in the context of more complex lambdas.
Function Body
Finally, the function body contains the logic of the lambda. You’d implement this just as you would in any standard function, allowing for any valid expressions or statements.
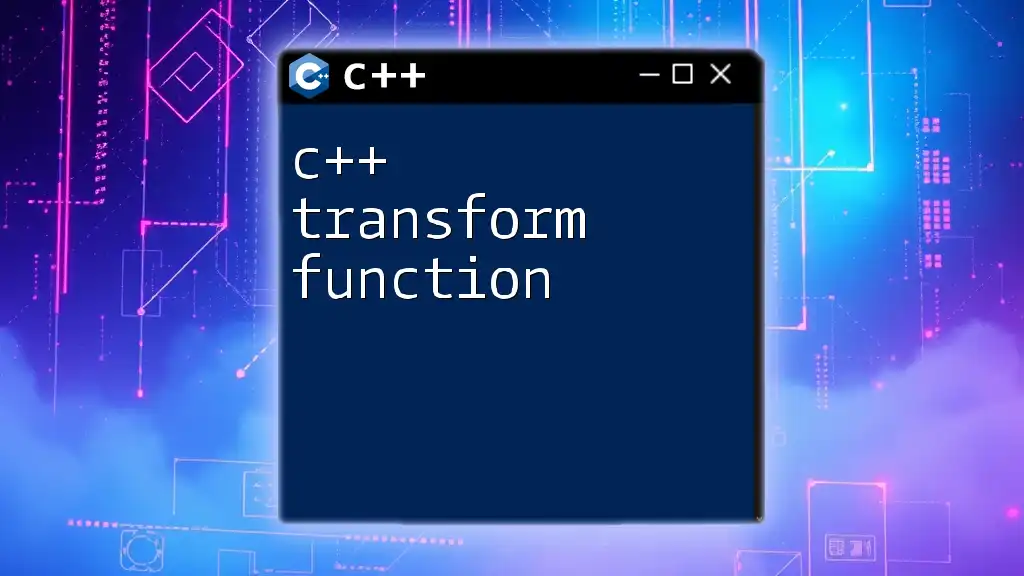
Practical Applications of Anonymous Functions
Using Anonymous Functions with STL Algorithms
The Standard Template Library (STL) in C++ offers various algorithms where lambdas can provide significant utility. For instance, using a lambda with `std::sort` enables succinct sorting without needing to resort to named functions:
std::vector<int> vec = {5, 3, 8, 1};
std::sort(vec.begin(), vec.end(), [](int a, int b) { return a < b; });
Here, the lambda function serves as a custom comparison operator directly defined in the sorting call, enhancing code clarity and effectiveness.
Callbacks and Event Handling
Anonymous functions can also act as callbacks, allowing them to be passed to APIs or event handlers seamlessly. For instance:
auto callback = [](int value) { std::cout << "Value: " << value << std::endl; };
callback(42);
This approach is particularly useful in GUI programming or asynchronous operations, where functions need to be executed in response to certain events.
Creating More Readable and Maintainable Code
Implementing lambda functions can lead to cleaner and more straightforward code by encapsulating logic within the context of its use. For example:
auto isEven = [](int n) { return n % 2 == 0; };
std::cout << isEven(4); // Outputs: 1 (true)
Here, the `isEven` function is defined inline, contributing to a more readable context in which it is used.
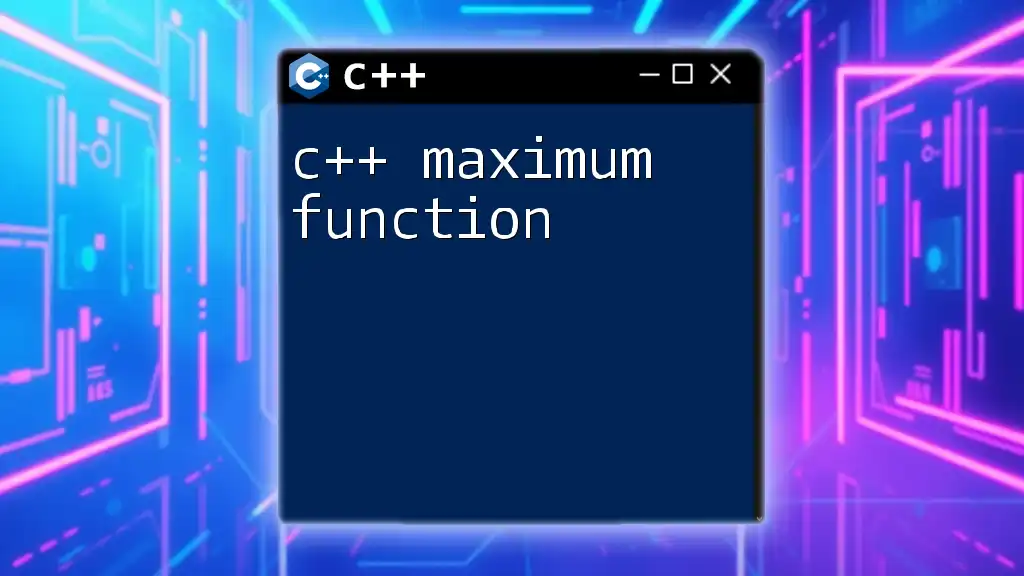
Advanced Usage of Lambda Functions
Mutable Lambdas
In some cases, you might need to modify captured variables. This is where mutable lambdas come in handy, allowing changes to the captured values:
int a = 10;
auto mutableLambda = [a]() mutable { return ++a; };
std::cout << mutableLambda(); // Outputs: 11
By enabling the mutation of captured variables, lambdas become significantly more versatile.
Lambda Functions Returning Functions
Lambdas can also return other lambdas, enabling powerful functional programming techniques. Consider the following example:
auto createMultiplier = [](int factor) {
return [factor](int value) { return value * factor; };
};
auto timesTwo = createMultiplier(2);
This pattern of returning lambdas makes it easy to generate function factories, where you define a function based on parameters.
Using Lambdas with `std::function`
Lambdas can be assigned to `std::function`, which abstracts the type to store any callable entity. This capacity is particularly useful when you need a flexible interface:
std::function<int(int, int)> func = [](int x, int y) { return x + y; };
std::cout << func(2, 3); // Outputs: 5
This allows you to interchangeably use lambdas and other callable types within your applications, ensuring flexibility in function handling.
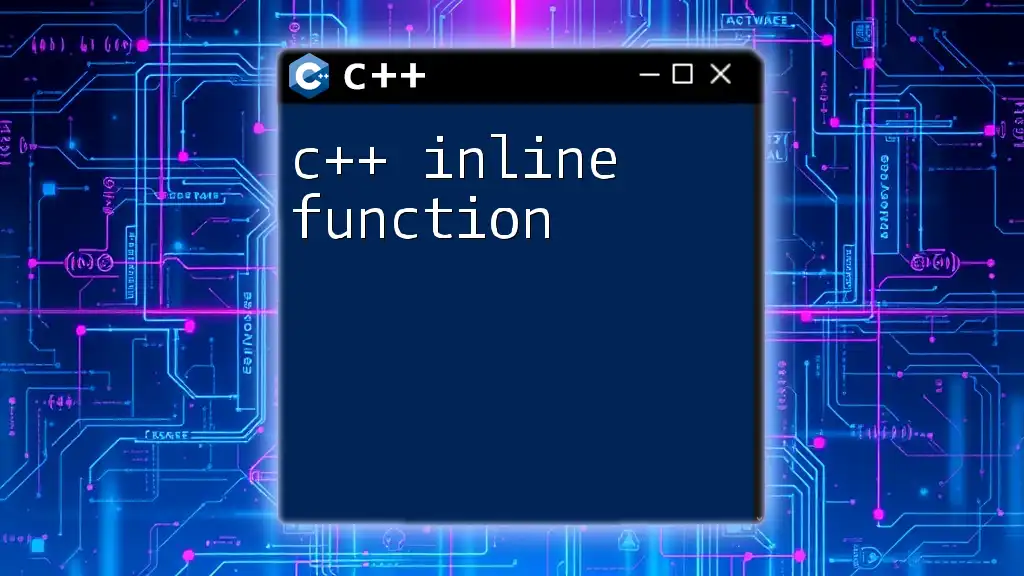
Conclusion
In summary, C++ anonymous functions (lambda functions) are an important tool for modern C++ development. They allow for cleaner, more expressive code that enhances readability and usability across various scenarios, from STL algorithms to callback functions. As you practice and implement anonymous functions in your own projects, you will undoubtedly find that they not only simplify your coding process but also improve the overall quality of your software solutions.
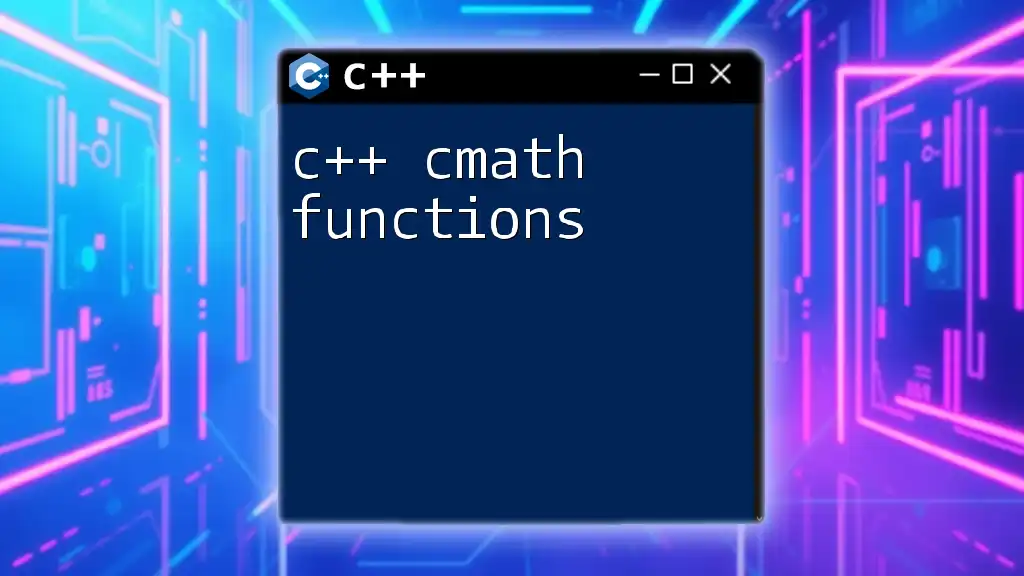
Additional Resources
To dive deeper into C++ anonymous functions and other advanced topics, consider exploring the following resources:
- Books on C++ programming, particularly those focused on the latest standards (C++11 and beyond).
- Online tutorials that offer hands-on coding exercises.
- Official C++ documentation and community forums for best practices and advice.