A C++ recursive function is a function that calls itself in order to solve a problem by breaking it down into smaller, more manageable subproblems.
Here's a simple example of a recursive function that calculates the factorial of a number:
#include <iostream>
using namespace std;
int factorial(int n) {
if (n <= 1) return 1; // Base case
return n * factorial(n - 1); // Recursive case
}
int main() {
cout << "Factorial of 5: " << factorial(5) << endl; // Output: 120
return 0;
}
Understanding Recursion in C++
What is Recursion?
Recursion is a fundamental concept in programming where a function calls itself in order to solve a problem. Each call to the function works on a smaller subset of the initial problem until it reaches a base case, at which point the function stops calling itself.
The Importance of Recursive Functions
Recursive functions bring several benefits to programming. They allow for easier understanding and elegance in problem-solving, especially for tasks that can be defined in terms of smaller instances of the same problem. For example, computing factorials or traversing complex data structures like trees can often be done more effectively using recursion rather than traditional iterative methods.
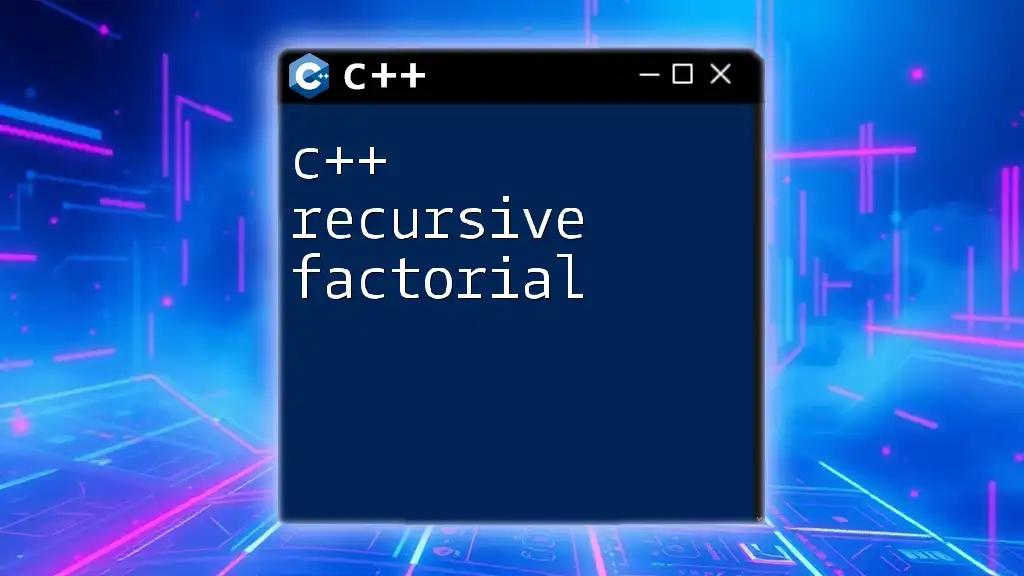
Basic Structure of Recursive Functions
Anatomy of a Recursive Function
Every recursive function must have two key components:
- Base Case: The condition under which the recursion ends. It serves as a stopping point to prevent infinite calls.
- Recursive Case: The part of the function where it calls itself with modified arguments, driving the problem towards the base case.
Visualizing the call stack can help in understanding what's happening during recursive calls. Each function invocation waits for the next one to return before it can finish executing.
Example Code Snippet: A Simple Recursive Function
#include <iostream>
using namespace std;
void countdown(int n) {
if (n < 0) return; // Base case
cout << n << endl; // Action
countdown(n - 1); // Recursive case
}
int main() {
countdown(5);
return 0;
}
In the `countdown` function above, the base case is when `n` is less than 0. The function prints the current value of `n` and then calls itself with `n - 1`, gradually reducing `n` until the base case is met.

Types of Recursive Functions
Direct vs Indirect Recursion
Direct recursion occurs when a function directly calls itself. Indirect recursion takes place when a function calls another function, which then calls the first function.
Example of Direct Recursion
int factorial(int n) {
if (n <= 1) return 1; // Base case
return n * factorial(n - 1); // Recursive case
}
Example of Indirect Recursion
void functionA(int n);
void functionB(int n);
void functionA(int n) {
if (n > 0) {
cout << n << " ";
functionB(n - 1); // Indirect call
}
}
void functionB(int n) {
if (n > 0) {
cout << n << " ";
functionA(n - 1); // Indirect call back to function A
}
}
In the indirect recursion example, both `functionA` and `functionB` call each other, creating a complex flow but ultimately still stemming from a recursive approach.
Tail Recursion vs Non-Tail Recursion
Another distinction is between tail recursion and non-tail recursion. A tail-recursive function is one where the recursive call is the last operation performed before the function finishes. This can be optimized by the compiler for better performance, as it can reuse the current function’s stack frame.
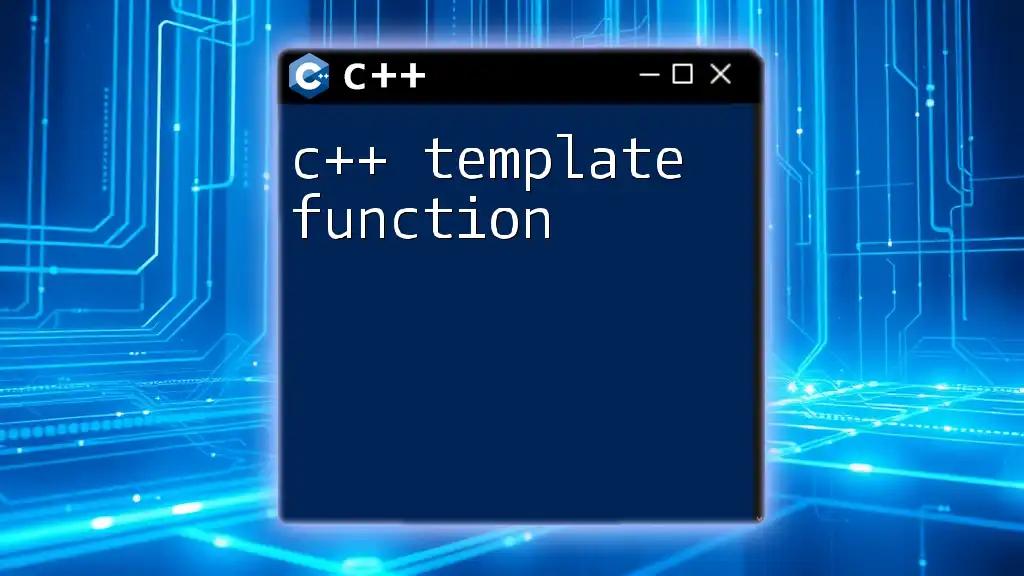
Characteristics of Recursive Functions
Memory Usage
Recursive functions use stack memory for each function call. Infinite recursion can lead to stack overflow errors if the base case is not defined properly or is never reached. It’s essential to understand how recursive calls stack up to avoid exceeding memory limits.
Time Complexity
The time complexity of recursive functions can vary. For example, the naive recursive solution for computing Fibonacci numbers can take exponential time due to repeated calculations. Understanding the efficiency of different approaches is crucial for effective programming.
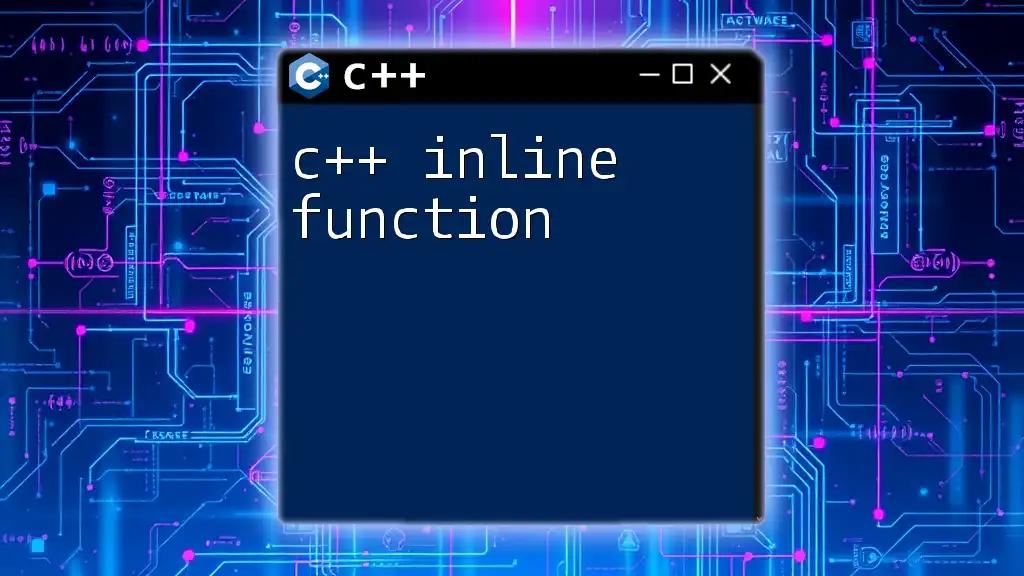
Common Use Cases for Recursive Functions
Factorial Calculation
Calculating the factorial of a number is a classic example of a problem that can be elegantly solved using recursion.
Example Code Snippet: Factorial Function
int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1); // Recursive case
}
Fibonacci Sequence
Fibonacci is another popular problem that can be solved recursively. However, naive recursion here is inefficient as it recalculates the values multiple times.
Example Code Snippet: Fibonacci Function
int fibonacci(int n) {
if (n <= 1) return n; // Base case
return fibonacci(n - 1) + fibonacci(n - 2); // Recursive case
}
The call stack grows rapidly for larger `n`, leading to performance issues due to recalculation.
Other Practical Examples
Towers of Hanoi is a classic problem that can be effectively solved with recursion.
Example Code Snippet: Towers of Hanoi Algorithm
void towersOfHanoi(int n, char from_rod, char to_rod, char aux_rod) {
if (n == 1) {
cout << "Move disk 1 from rod " << from_rod << " to rod " << to_rod << endl; // Base case
return;
}
towersOfHanoi(n - 1, from_rod, aux_rod, to_rod); // Move n-1 disks to auxiliary rod
cout << "Move disk " << n << " from rod " << from_rod << " to rod " << to_rod << endl; // Move nth disk
towersOfHanoi(n - 1, aux_rod, to_rod, from_rod); // Move n-1 disks from auxiliary to target
}
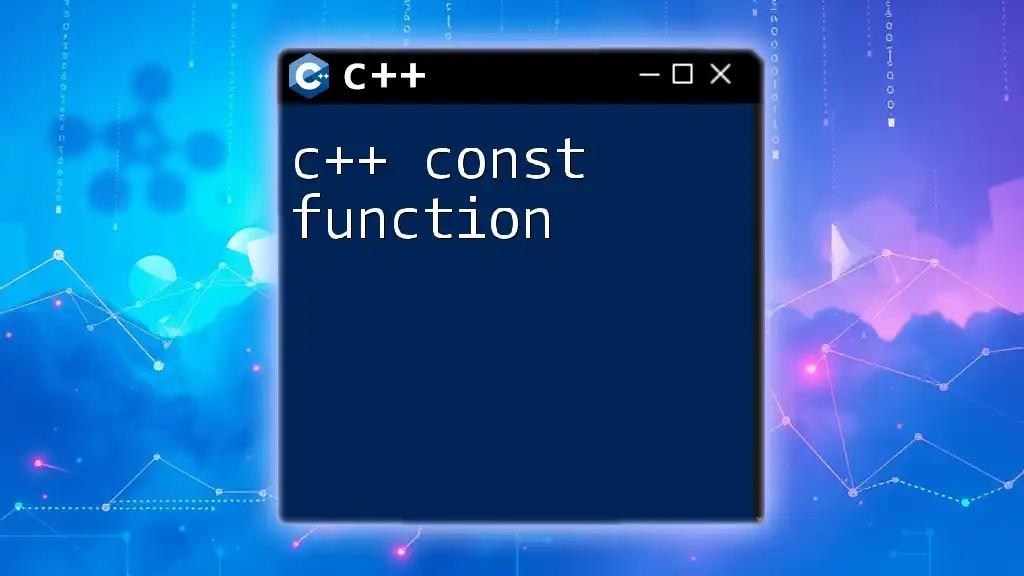
Best Practices for Writing Recursive Functions
Ensure Base Cases Are Defined
Every recursive function must have a well-defined base case. This is crucial for preventing infinite recursion and ensuring that the recursion ultimately terminates.
Optimize for Performance
Implement techniques like memoization to improve performance. Memoization stores previously computed results to avoid redundant calculations.
Example Code Snippet: Memoized Fibonacci
#include <unordered_map>
int fibonacciMemo(int n, std::unordered_map<int, int>& memo) {
if (memo.find(n) != memo.end()) return memo[n]; // Check cache
if (n <= 1) return n; // Base case
memo[n] = fibonacciMemo(n - 1, memo) + fibonacciMemo(n - 2, memo); // Recursive call with caching
return memo[n];
}
Avoiding Stack Overflow
To avoid stack overflow with deeply nested recursion, consider using iterative solutions or techniques like tail recursion when applicable.
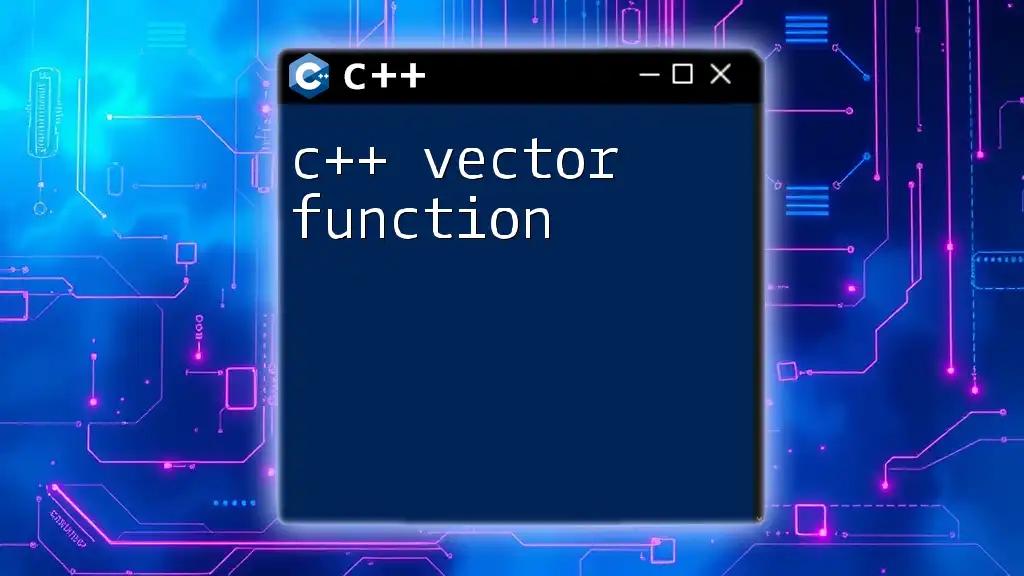
Conclusion
Understanding and utilizing C++ recursive functions can be a powerful tool in a programmer's arsenal. By mastering recursion, you will be able to devise elegant solutions for complex problems more efficiently. Experimenting with recursive functions through coding challenges can deepen your understanding and enhance your coding proficiency.
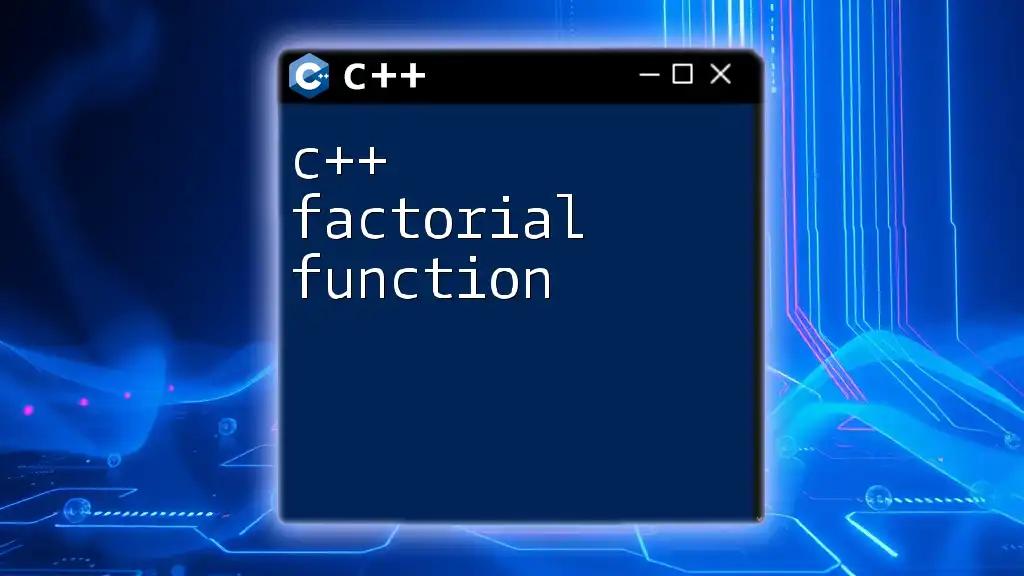
Resources for Further Learning
For those seeking to expand their knowledge further, consider looking into various programming books, online courses focused on data structures and algorithms, and engaging with programming communities to explore practical applications of recursion and other techniques.