In C++, a recursive factorial function calculates the factorial of a non-negative integer by repeatedly calling itself until it reaches the base case of 0, returning 1.
Here's a simple implementation in C++:
#include <iostream>
using namespace std;
int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
int main() {
int number = 5;
cout << "Factorial of " << number << " is " << factorial(number) << endl;
return 0;
}
Understanding Factorial
What is Factorial?
The factorial of a non-negative integer `n`, denoted as `n!`, is defined as the product of all positive integers less than or equal to `n`. The formula for computing factorial is:
\[ n! = n \times (n-1) \times (n-2) \times ... \times 3 \times 2 \times 1 \]
An important special case to note is that \( 0! = 1 \). This definition allows the definition to hold consistently throughout mathematics.
Mathematical Properties of Factorial
Factorials have various properties that are crucial in mathematics:
-
Combinatorial Significance: Factorials are fundamental in computing combinations and permutations, where the number of ways to choose `r` elements from a set of `n` is given by the formula:
\[ C(n, r) = \frac{n!}{r!(n - r)!} \]
-
Growth Rate: Factorial grows at an exceedingly rapid rate. For instance, \( 5! = 120 \), while \( 10! = 3,628,800 \). This growth is essential when evaluating algorithms, particularly in combinatorioal problems.
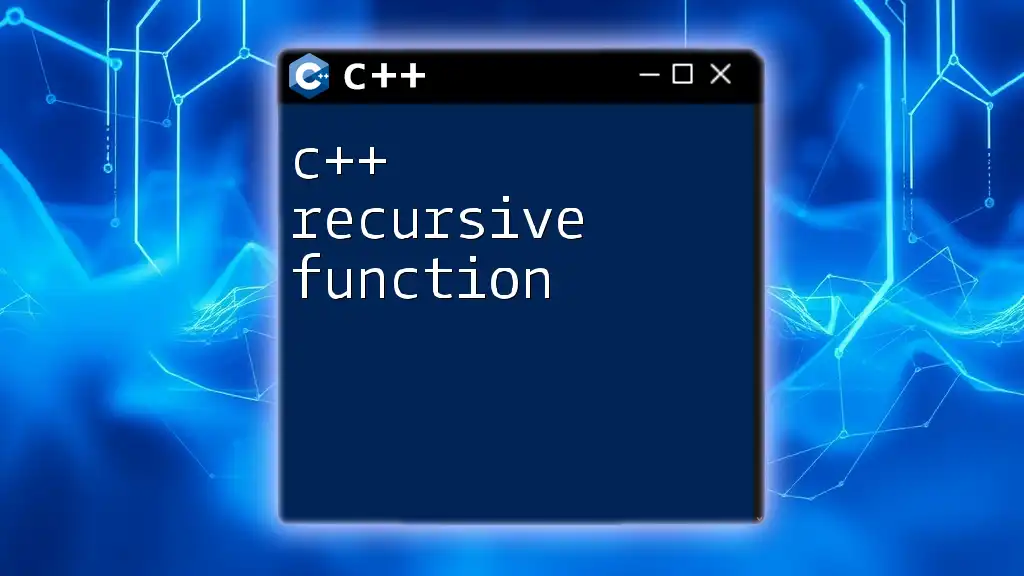
Introduction to Recursion
What is Recursion?
Recursion refers to the process of a function calling itself to solve smaller instances of the same problem. By breaking down a problem into smaller, more manageable parts, complex functions can be implemented in a straightforward manner. In contrast to iterative solutions, recursion can provide elegant solutions at the expense of performance and memory usage.
Benefits and Drawbacks of Recursion
Advantages
-
Simplicity: Recursive functions are often easier to read and understand because they mirror powerful mathematical definitions, making code clearer.
-
Reduction of Boilerplate: Recursive code eliminates the requirement for loops, leading to shorter and more maintainable code.
Disadvantages
-
Memory Consumption: Each function call increases the call stack, and deep recursions can lead to stack overflow errors if not managed properly.
-
Speed Concerns: Recursive solutions can be slower than their iterative counterparts due to overhead from multiple function calls.
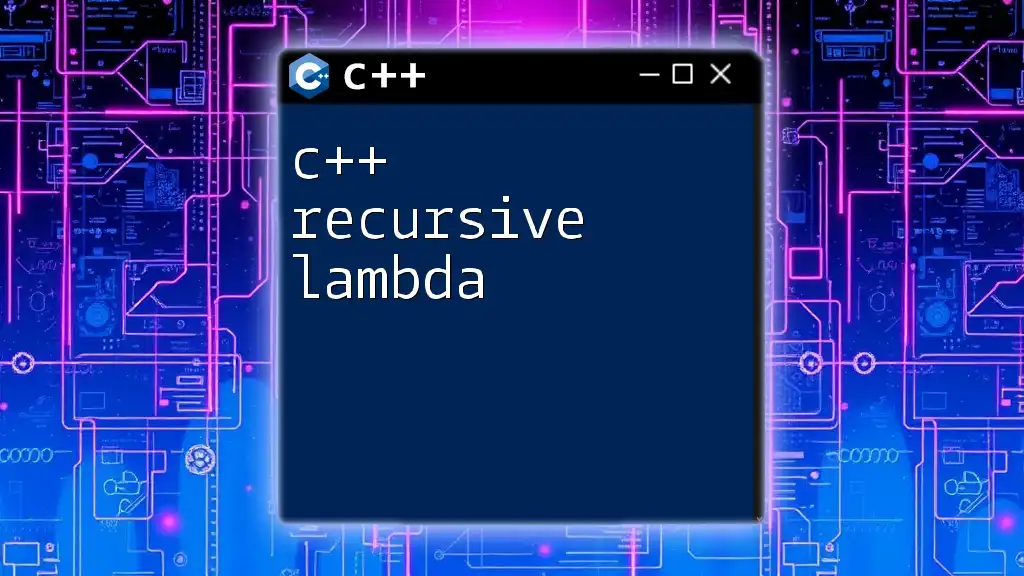
C++ Recursive Factorial
C++ Factorial Recursive: A Basic Example
Let's implement the basic recursive function to calculate the factorial of a number in C++. The code snippet below outlines this simple recursive solution:
int factorial(int n) {
if (n == 0) return 1; // Base case
return n * factorial(n - 1); // Recursive call
}
Explanation of the Code
In this code, we see an example of the recursive logic applied to compute the factorial:
-
Base Case: The base case is critical as it halts the recursive calls. When `n` reaches zero, it returns `1`. Without this base case, the function would theoretically continue to call itself indefinitely, leading to a stack overflow.
-
Recursive Call: The function calls itself with the argument `n-1`, multiplying the current `n` by the factorial of `(n-1)`. This recursive relationship is what allows us to compute larger numbers based on smaller results.
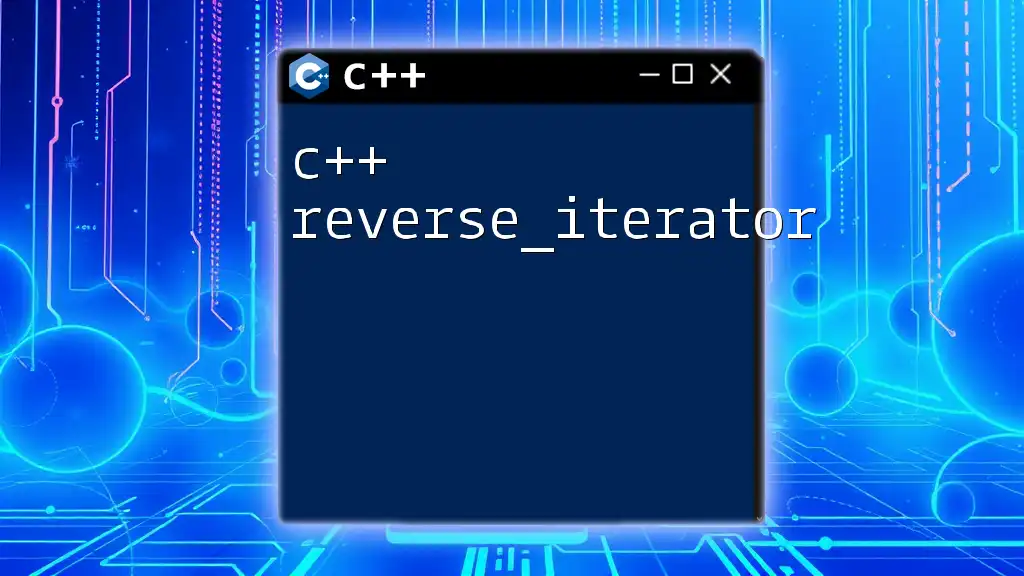
Enhancements on the Recursive Factorial
Handling Negative Numbers
It is crucial to handle edge cases, such as when a negative number is passed to the factorial function. Here’s how you can modify the function to manage such cases gracefully:
int factorial(int n) {
if (n < 0) throw std::invalid_argument("Negative numbers do not have a factorial.");
if (n == 0) return 1; // Base case
return n * factorial(n - 1); // Recursive call
}
In this enhanced implementation, we check if `n` is negative and throw an exception, preventing the function from attempting to calculate the factorial of an invalid input.
Using C++ Exception Handling
By integrating exception handling, you can ensure that your program behaves correctly under invalid conditions. This approach preserves the integrity of the program and provides meaningful feedback to the user about erroneous input.
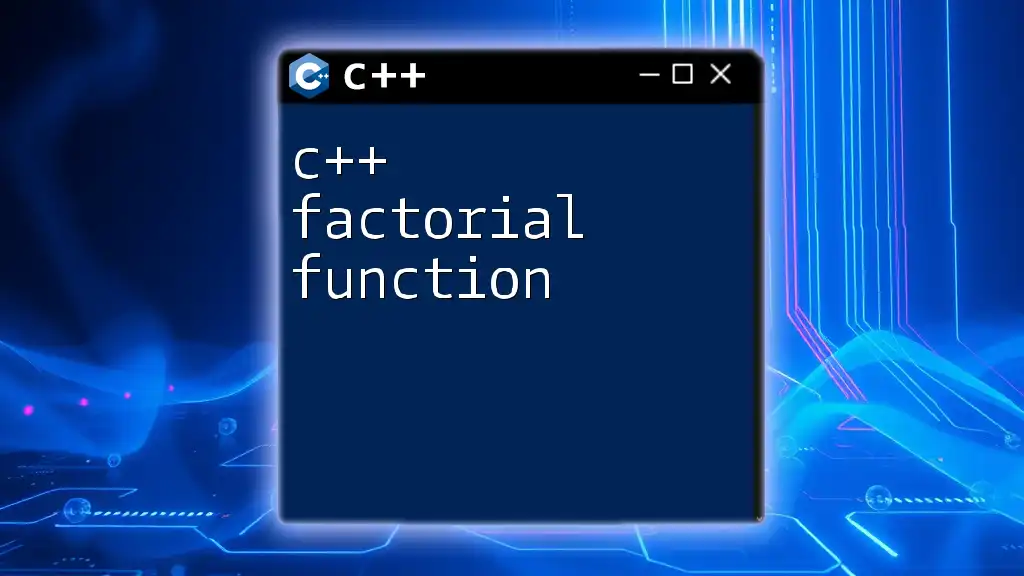
Practical Usage of Factorial in C++
C++ Factorial Recursion: Applications
Understanding the factorial function is not only pertinent in theoretical contexts; it has several practical applications in real-world computing:
- Combinatorics: Calculating permutations and combinations for problems involving arrangements of data.
- Probability: Necessary for computations involving the likelihood of certain outcomes.
- Computer Science: Algorithms that manipulate large sets of data often require factorial computations.
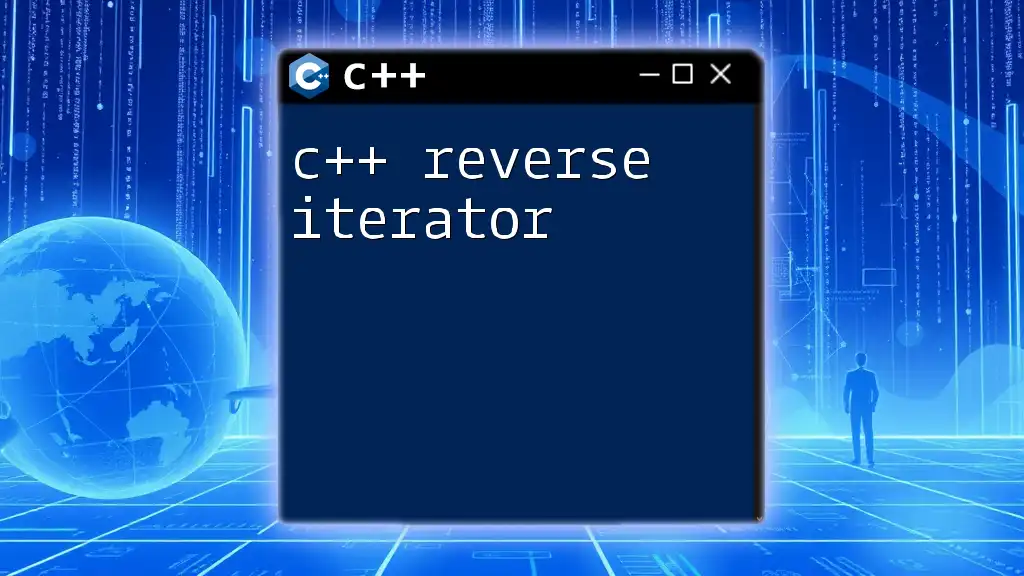
Performance Considerations
Time and Space Complexity
The time complexity of the recursive factorial function is \( O(n) \) because it makes a single recursive call for each decrement of `n`. The space complexity is also \( O(n) \) due to the depth of the call stack as each function call is queued until the base case is hit.
Tail Recursion
While simple recursion functions as straightforwardly as explained, there is also the concept of tail recursion. In tail recursion, the recursive call is the last operation in the function. This approach can optimize performance and manage memory effectively. Here’s how you can implement tail recursion for computing factorial:
int tail_factorial(int n, int accumulator = 1) {
if (n == 0) return accumulator; // Base case
return tail_factorial(n - 1, n * accumulator); // Tail recursive call
}
In this function, the `accumulator` parameter aggregates the computed result. Because the final action of the function is the recursive call, the compiler may optimize the call stack usage.
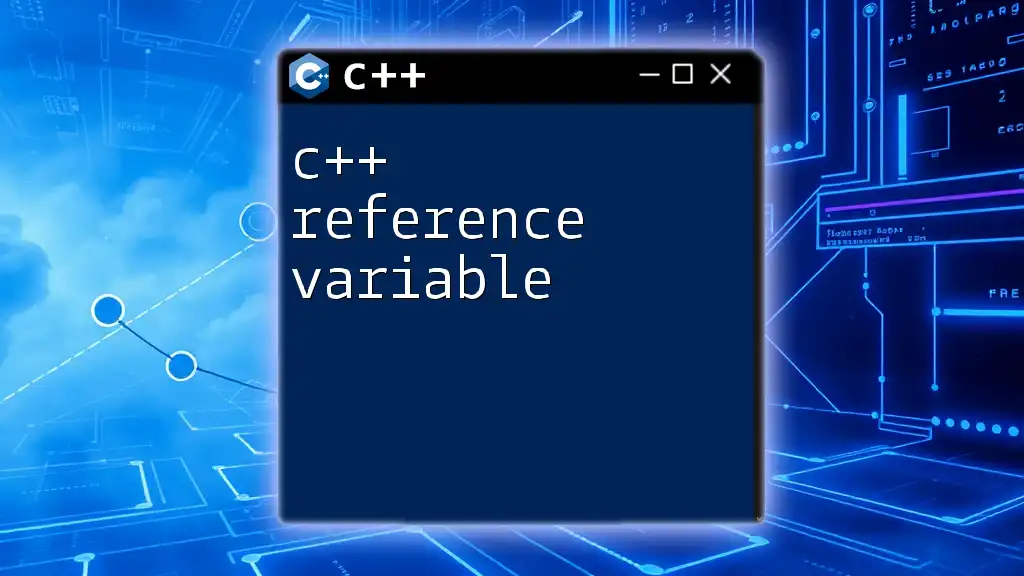
Conclusion
The `c++ recursive factorial` implementation provides a valuable insight into recursion's capabilities and its applications in mathematics and computer science. By mastering recursive approaches, programmers can write code that is not only effective but also elegant. Understanding how to manage edge cases, the nuances of performance, and different recursive strategies such as tail recursion enhances your skills as a C++ developer.
As you embark on practicing these concepts, explore additional recursive problems and challenge yourself to solidify your understanding of recursion and its potential within C++.