In C++, the exclusive OR (XOR) operation can be performed using the `^` operator, which returns true if one of the operands is true, but not both.
Here's a code snippet demonstrating the XOR operation:
#include <iostream>
int main() {
bool a = true;
bool b = false;
bool result = a ^ b; // Exclusive OR
std::cout << "Result of a XOR b: " << result << std::endl; // Outputs: 1 (true)
return 0;
}
What is the Exclusive Or (XOR) Operator?
The Exclusive Or (XOR) operator is a fundamental concept in boolean algebra that holds significant importance in programming, particularly in C++. The XOR operator evaluates two input conditions and yields a true (1) outcome when only one of the conditions is true. If both conditions are true or both are false, the result will be false (0). This property makes the XOR operator unique and particularly useful in various programming scenarios.
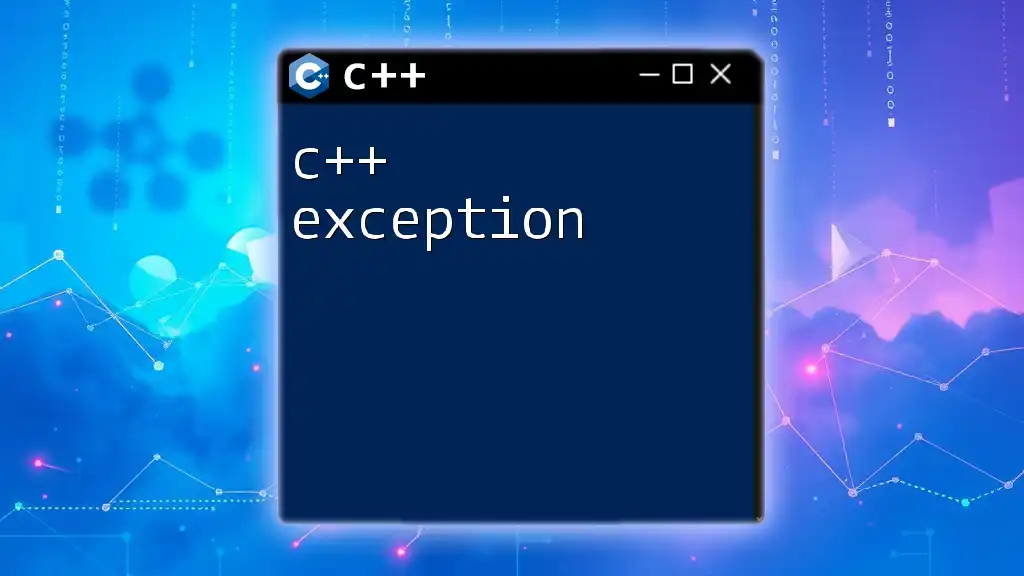
How to Use the XOR Operator in C++
The syntax of the XOR operator in C++ is simple and straightforward. The XOR operator is represented by the caret symbol (`^`).
// C++ syntax for XOR
result = a ^ b;
In this syntax, `a` and `b` are boolean or integer values. The result will contain the outcome of the XOR operation.
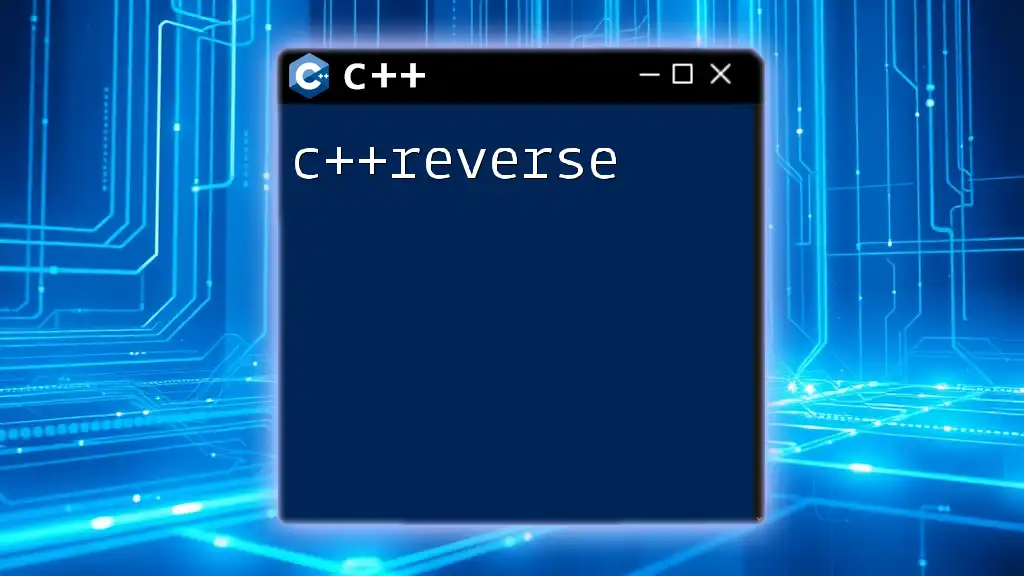
The Purpose of the XOR Operator
Logical Comparison: The XOR operator serves a distinct role in comparing binary digits. When comparing two bits, the XOR operator will return a true (1) value if the two bits are different. Conversely, if both bits are the same, it yields a false (0).
Truth Table
A truth table can help clarify how XOR operates with different inputs:
A | B | A XOR B |
---|---|---|
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
As indicated in the truth table, the XOR operator outputs true only when one of the inputs is true.
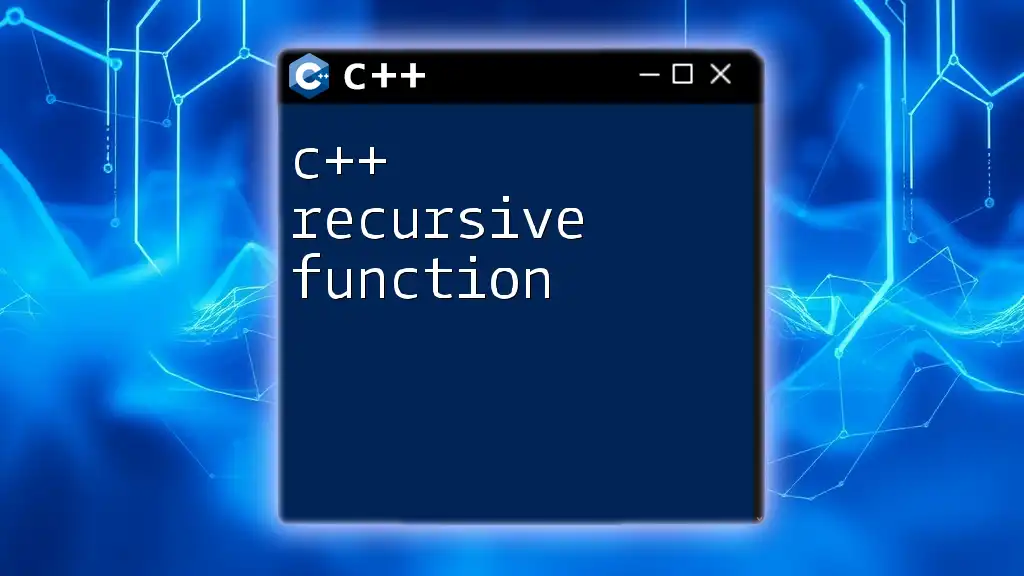
Practical Applications of the XOR Operator
Bit Manipulation
XOR is a powerful tool for bitwise manipulation due to its properties. It is often used in algorithms that deal with cryptography and data encoding.
Swapping Variables
A classic example of using the XOR operator is to swap two variables without an auxiliary variable. The following C++ code snippet illustrates this technique:
// Example of swapping using XOR
int x = 5;
int y = 10;
x = x ^ y;
y = x ^ y;
x = x ^ y;
// After execution, x is 10, and y is 5
This method leverages the unique properties of XOR, allowing a variable's values to be exchanged without needing temporary storage.
XOR in Conditional Statements
Using XOR in conditional statements can simplify specific checks. When used in conditions, the XOR operator will return true if either of the conditions is true but not both.
// Example condition
bool isAorB = (a ^ b);
if (isAorB) {
// Code to execute when either A or B is true, but not both
}
This can lead to more efficient and cleaner code.
Debugging with XOR
In the debugging phase, the XOR operator can help identify differences between two states or values. By applying XOR to two values, the resultant bit pattern will highlight areas where the values differ, making it easier to diagnose issues in logic or data.
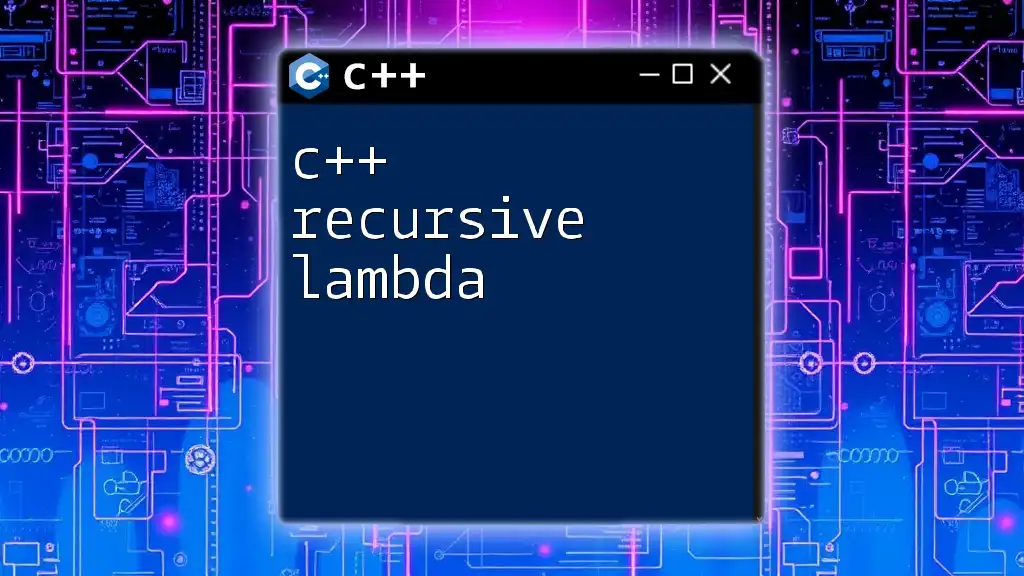
Comparisons with Other Operators
XOR vs. OR
Understanding the distinction between XOR and the standard OR operator is essential for effective programming. The logical OR operator outputs true if at least one operand is true, whereas XOR specifically requires only one operand to be true for a true outcome.
Truth Table Comparison
Here is a comparison of the truth tables for both XOR and OR operators:
A | B | A OR B | A XOR B |
---|---|---|---|
0 | 0 | 0 | 0 |
0 | 1 | 1 | 1 |
1 | 0 | 1 | 1 |
1 | 1 | 1 | 0 |
From this comparison, it is clear that while both operators return true for inputs (0, 1) and (1, 0), their behavior diverges in the case where both inputs are true.
XOR vs. AND
The AND operator serves an entirely different function, resulting in true only when both operands are true. At times, XOR and AND may be used in combination in complex logic statements, but they cater to different logic conditions.
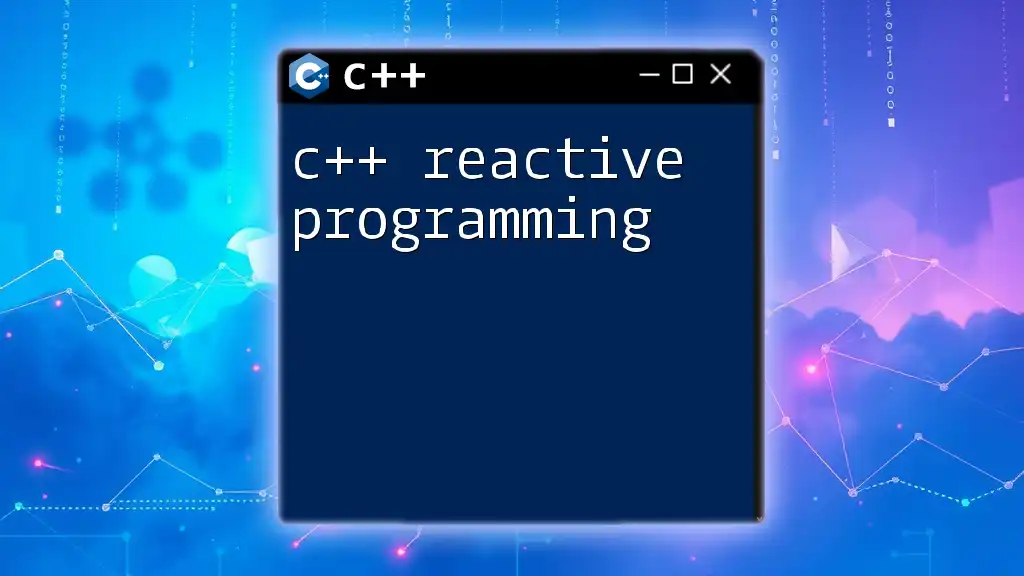
Advanced Techniques Using XOR
XOR in Cryptography
XOR is frequently utilized in creating strong encryption techniques, particularly within the concept of a one-time pad. In this encryption method, plaintext is XORed with a random key of equal length to produce ciphertext.
// Simplified example of XOR encryption
char encrypt(char a, char b) {
return a ^ b; // Returns the XOR of two characters
}
This simple technique allows for efficient and effective encryption when the key is random and used only once.
XOR and Array Manipulation
One of the remarkable applications of XOR is in finding unique numbers in an array. If you have several numbers with one appearing only once, you can efficiently identify that value with the XOR operation.
int findUnique(int arr[], int n) {
int unique = 0;
for (int i = 0; i < n; i++) {
unique ^= arr[i]; // XOR all elements
}
return unique; // Returns the unique number
}
This approach takes advantage of XOR's properties, allowing you to find the odd one out in linear time and constant space.
Performance Considerations
In terms of performance, XOR is a highly efficient operation. It’s optimized at the hardware level, meaning that using it can be computationally less expensive than other logical operations. This makes it an appealing choice in performance-sensitive applications.
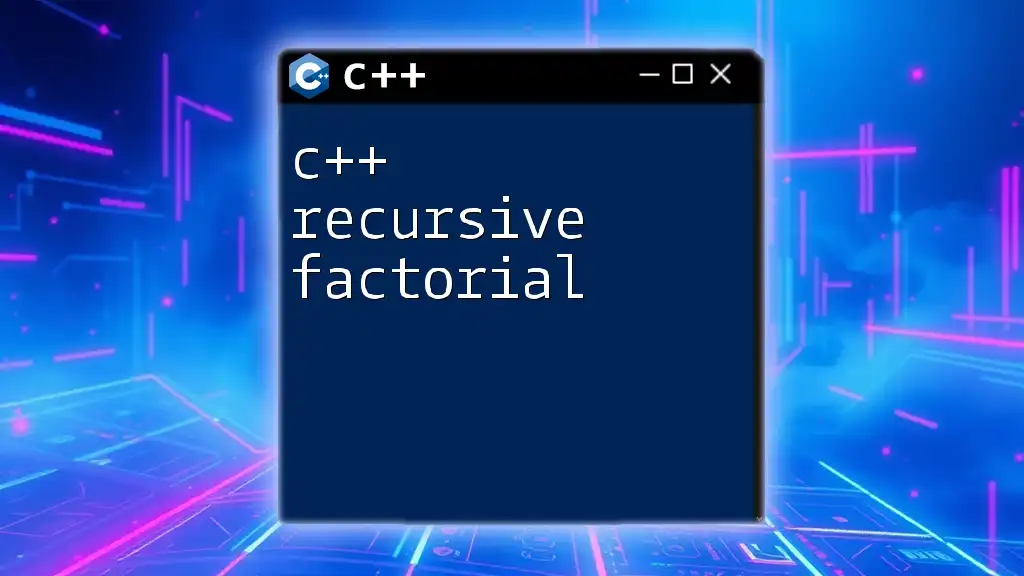
Conclusion
The C++ Exclusive Or (XOR) operator is versatile and impactful, offering unique capabilities in logical operations, debugging, encryption, and more. Understanding its functionality and applications can significantly improve your coding practices and problem-solving strategies. Whether you're swapping variables, manipulating bits, or developing cryptographic techniques, mastering the XOR operator is essential for any C++ programmer. Embrace its power and integrate it into your coding toolkit for enhanced efficiency and capability.
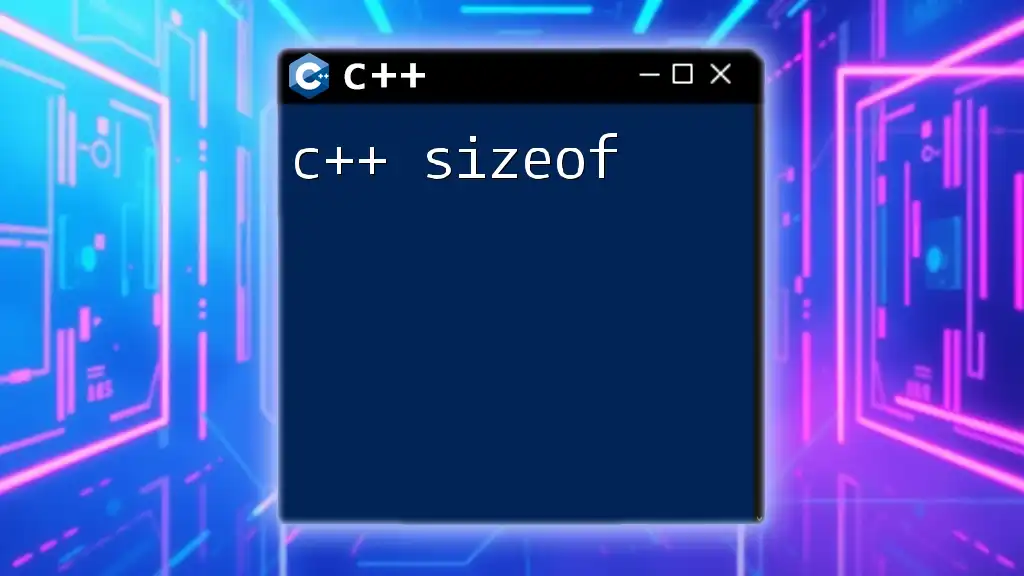
Additional Resources
For those looking to further their understanding, there are numerous resources available, including tutorials and references on C++ operators and programming techniques. Leverage these resources to deepen your knowledge and practical experience in using the XOR operator.