The `else if` statement in C++ allows for multiple conditional branches, enabling the execution of different code blocks based on various conditions.
#include <iostream>
int main() {
int num = 10;
if (num > 10) {
std::cout << "Number is greater than 10";
} else if (num == 10) {
std::cout << "Number is equal to 10";
} else {
std::cout << "Number is less than 10";
}
return 0;
}
Understanding the C++ If Else If Structure
What is the `if else if` Statement in C++?
The `if else if` statement in C++ is a control structure that allows you to execute different code blocks based on multiple conditions. It provides a way to handle multiple true/false scenarios in an organized manner. When using `if else if`, the program evaluates conditions in order and executes the block of code corresponding to the first true condition. If none of the conditions are true, the `else` block (if provided) is executed.
Syntax Breakdown of C++ Else If Statements
The syntax of the `else if` statement is straightforward, offering a clean approach to managing complex condition checks. Here’s a general structure to illustrate:
if (condition1) {
// Code block if condition1 is true
} else if (condition2) {
// Code block if condition2 is true
} else {
// Code block if none of the above conditions are true
}
In this syntax, `condition1` and `condition2` are Boolean expressions that will be evaluated. The code block executes for the first condition that evaluates to `true`, allowing for efficient control flow in your C++ programs.
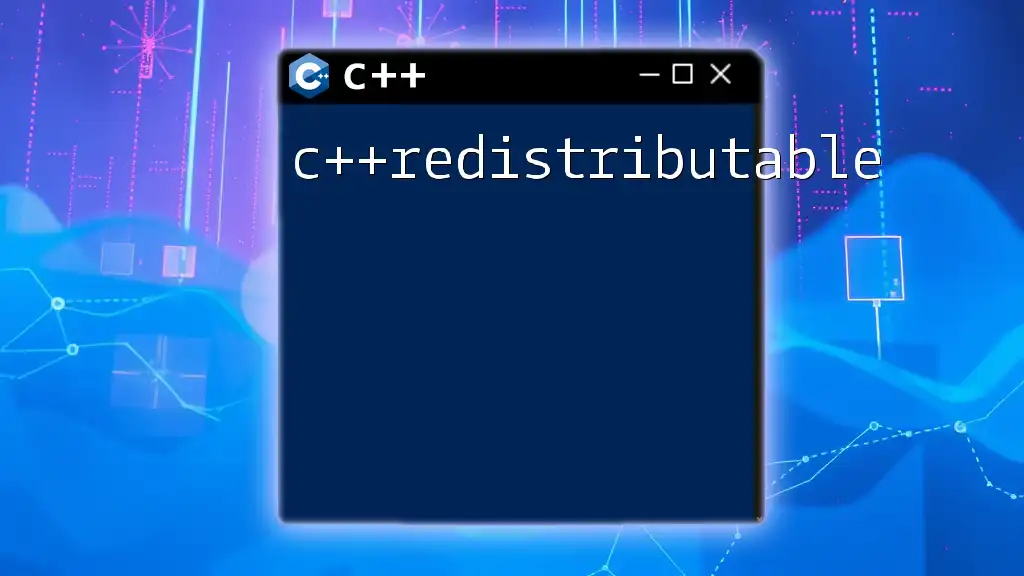
How to Use Else If in C++
Implementing Else If in C++
To effectively implement `else if` statements in your C++ code, start by identifying the conditions you wish to evaluate. The logic flows as follows:
- Begin with an `if` statement that evaluates a condition.
- Follow it with one or more `else if` statements to check additional conditions if the previous ones were false.
- Optionally conclude with an `else` statement to capture any cases not handled by the previous conditions.
Important Considerations for Using Else If
When deploying `else if` statements, consider the following:
- Order Matters: The program evaluates the conditions in top-to-bottom order. Ensure that more specific conditions are checked first to avoid premature evaluations.
- Clarity vs. Complexity: If you find that your `else if` chain is becoming lengthy, consider whether a different structure (like a `switch` statement or even a different control flow method) might reduce complexity and improve readability.
- Avoiding Redundant Conditions: Make sure that the statements do not overlap unless intended. Overlapping conditions can lead to confusion about which block will execute.
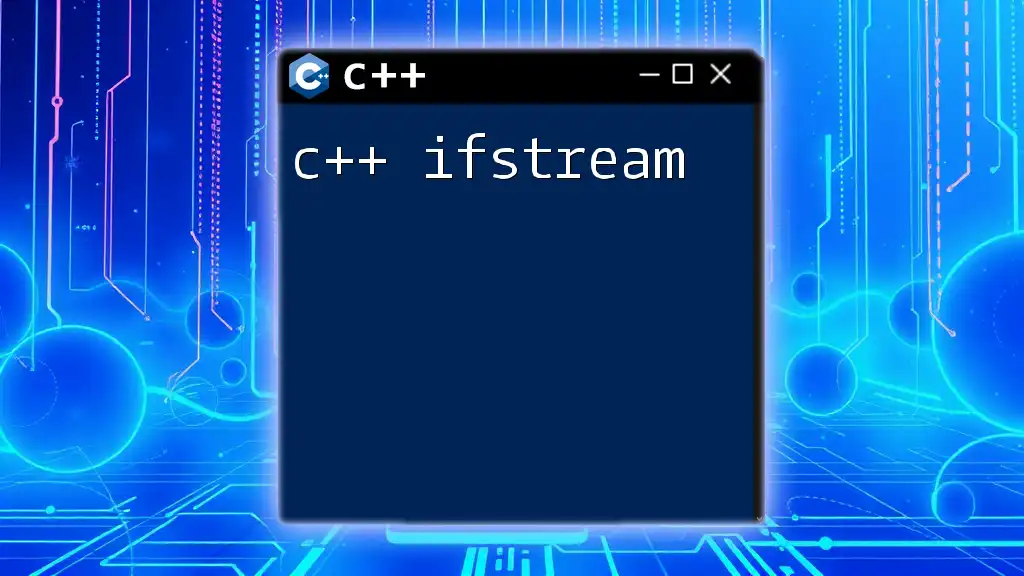
Practical Examples of Else If Statements in C++
Example: Basic Else If Statement
To demonstrate the mechanics of `else if`, consider a scenario that evaluates a number to determine if it's positive, negative, or zero:
int number = 10;
if (number > 0) {
std::cout << "Positive Number";
} else if (number < 0) {
std::cout << "Negative Number";
} else {
std::cout << "Zero";
}
In this example, the code checks the value of `number`. If it's greater than zero, it prints "Positive Number." If it's less than zero, it prints "Negative Number." If neither of those conditions holds true, it defaults to printing "Zero."
Example: Multiple Conditions with Else If
Let’s expand on this by evaluating letter grades and providing feedback based on different criteria:
char grade = 'B';
if (grade == 'A') {
std::cout << "Excellent!";
} else if (grade == 'B') {
std::cout << "Well done!";
} else if (grade == 'C') {
std::cout << "Good!";
} else {
std::cout << "Grade not recognized";
}
Here, the program assesses the variable `grade` and responds with targeted messages based on the input. Each ensuing condition is checked only if the previous ones were false.
Example: Nested If Else If Statements
More complex evaluations may require nesting of `if else if` statements. Here’s an example that determines grades based on score thresholds:
int score = 85;
if (score >= 90) {
std::cout << "Grade A";
} else if (score >= 80) {
if (score >= 85) {
std::cout << "Grade B+";
} else {
std::cout << "Grade B";
}
} else {
std::cout << "Grade C or lower";
}
In this instance, the outer `if` checks if the score is `90` or higher. If that condition is false, it evaluates whether the score is `80` or higher, invoking further nested logic to determine if it merits a `B+` or a `B` grading notation.
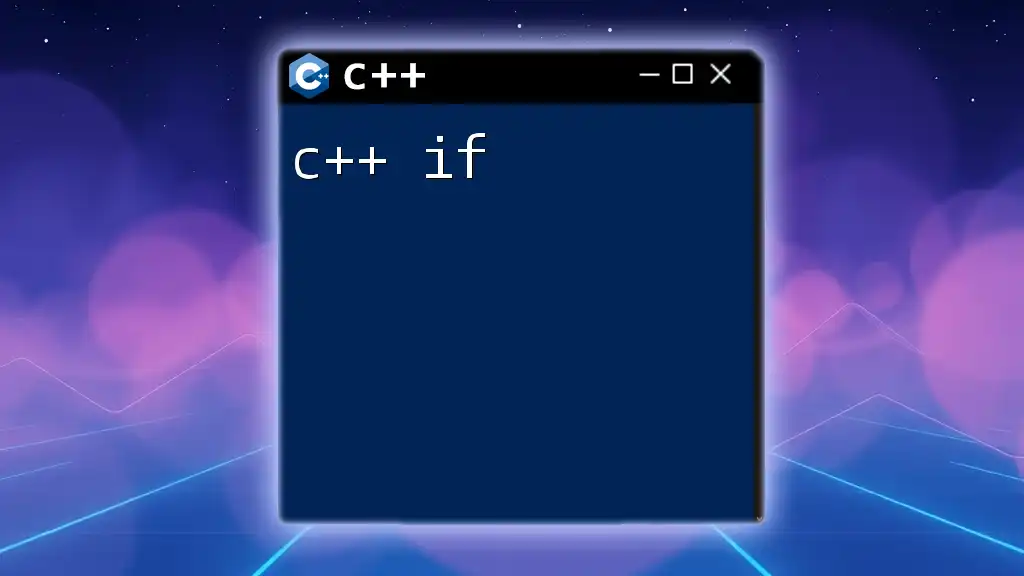
Common Mistakes with Else If in C++
Misunderstanding Condition Evaluation
One common mistake programmers make is misunderstanding how conditions are evaluated in `else if` chains. Remember that once a true condition is found, the following conditions are no longer evaluated. This behavior can sometimes lead to unintended results:
int value = 5;
if (value > 0) {
std::cout << "Value is positive";
} else if (value == 5) {
std::cout << "Value equals five"; // This will be ignored
}
In this scenario, although `value` is equal to `5`, the second condition won’t trigger because the first condition evaluates to true.
Forgetting the Last Else Statement
Another common oversight is omitting the final `else` statement. Including it serves as a fallback for unexpected cases, ensuring that your program doesn't silently fail:
int number = 0;
if (number > 0) {
std::cout << "Positive Number";
} else if (number < 0) {
std::cout << "Negative Number";
}
// Missing else for the case when number is zero
Always strive to address all potential cases to avoid unexpected behavior in your programs.
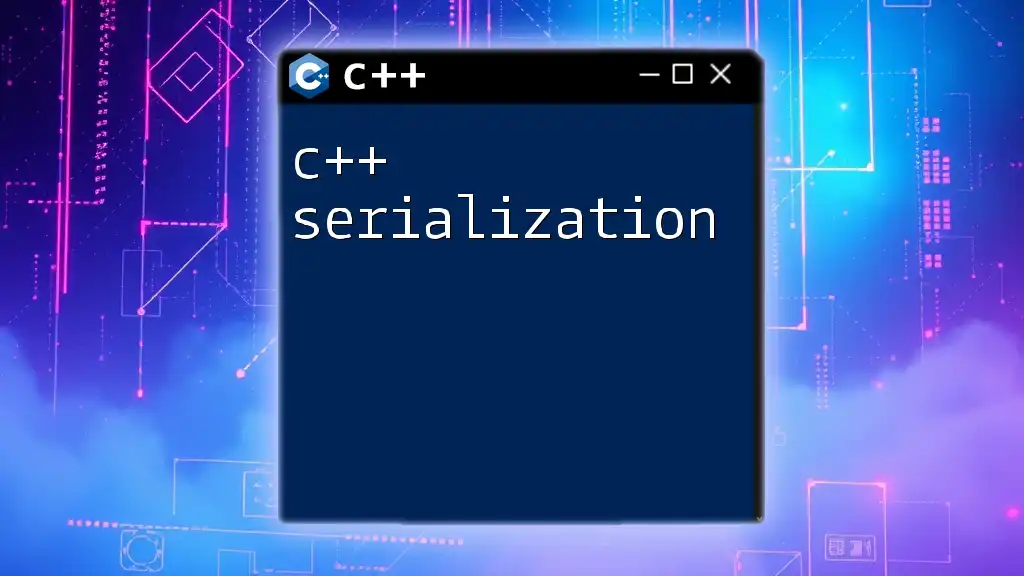
Combining Else If with Other Control Statements
Using Else If with Switch Statements
While `else if` is powerful, there are scenarios where a `switch` statement may be more appropriate, especially when checking the same variable against numerous constant values.
char grade = 'B';
switch(grade) {
case 'A':
std::cout << "Excellent!";
break;
case 'B':
std::cout << "Well done!";
break;
case 'C':
std::cout << "Good!";
break;
default:
std::cout << "Grade not recognized";
}
Here, the switch statement simplifies the code, making it clearer and potentially more efficient for certain cases.
If and If Else Statement in C++
It’s also essential to understand the distinctions between `if` and `if else` versus the `else if` pattern. While `if else` implies a binary choice, the `else if` construct enables you to check for multiple conditions sequentially:
if (condition1) {
// Code for condition 1
} else {
// Code for all other conditions
}
This succinct construction can often suffice when you only have two possible paths, simplifying your logic and enhancing readability.
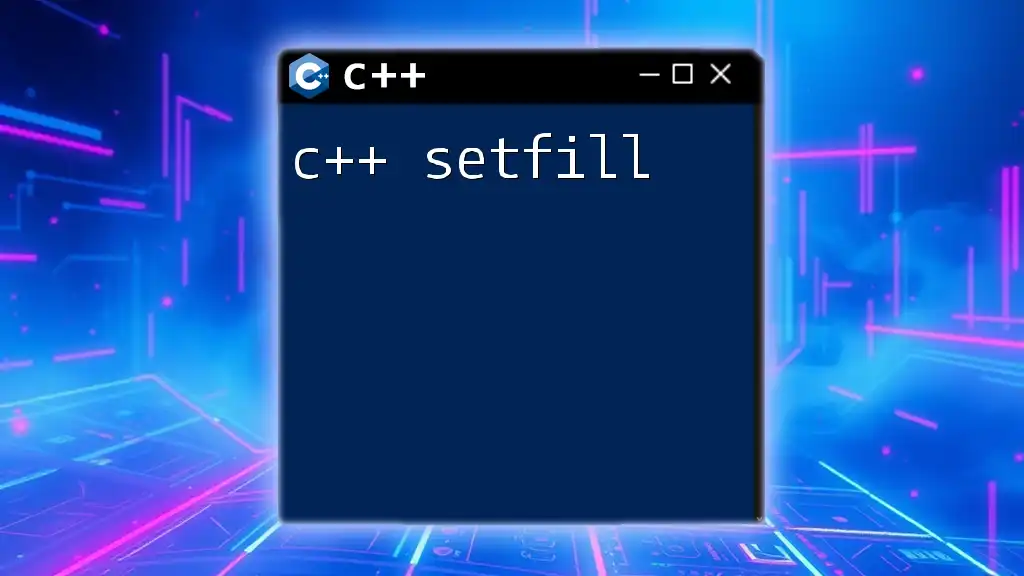
Conclusion
The `c++ else if` command is a cornerstone of control structures within the C++ programming language. Mastering its use allows for elegant and efficient decision-making within your applications. As you explore and practice with `else if`, remember to consider the order of conditions, use clear and structured logic, and avoid redundancy to ensure your code remains maintainable and easy to understand.
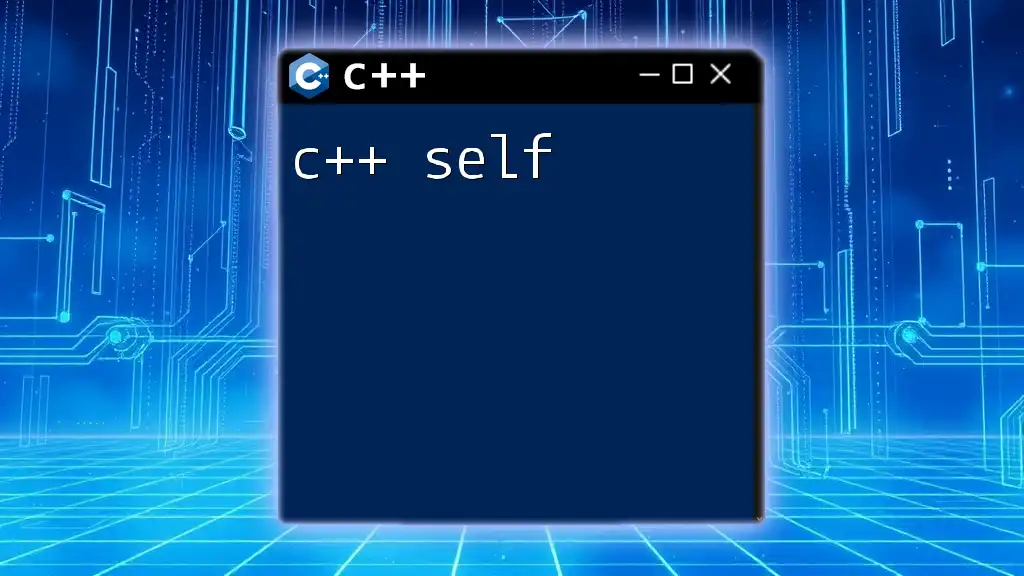
Additional Resources
For those looking to deepen their understanding of `if else if` statements and control structures in C++, resources are plentiful. Explore documentation, join forums, participate in C++ communities, and review recommended literature to enhance your expertise further.
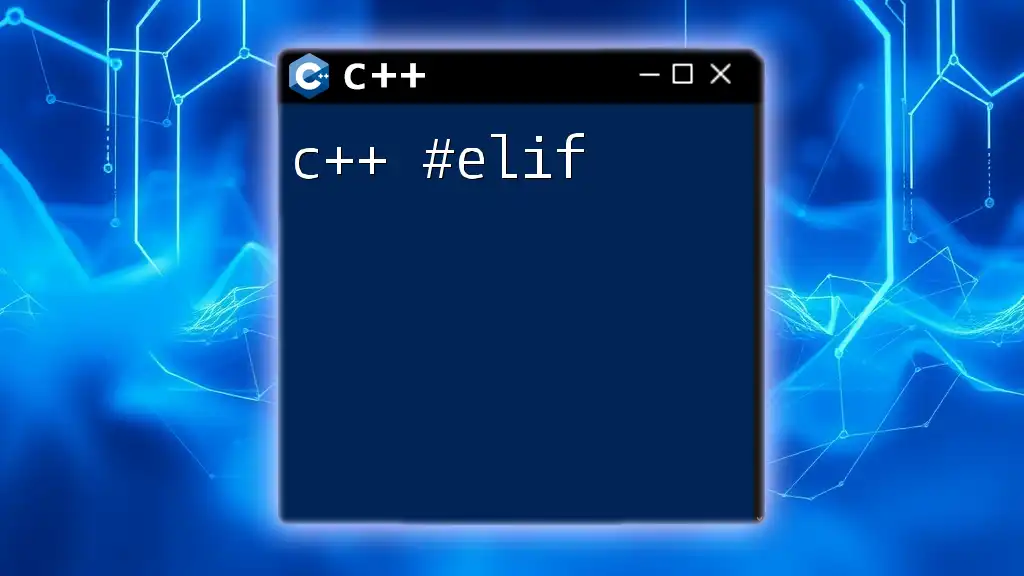
Call to Action
We encourage you to experiment with your own `else if` examples. Share your experiences, challenges, and solutions in the comments section below. Happy coding!