The `#elif` directive in C++ is used in preprocessor conditionals to specify an additional condition if the preceding `#if` or `#elif` condition evaluates to false.
#include <iostream>
#define CONDITION_1 0
#define CONDITION_2 1
int main() {
#if CONDITION_1
std::cout << "Condition 1 is true." << std::endl;
#elif CONDITION_2
std::cout << "Condition 2 is true." << std::endl;
#else
std::cout << "Neither condition is true." << std::endl;
#endif
return 0;
}
The Basics of `#ifdef` and `#ifndef`
Conditional directives are essential in C++ for controlling the compilation of code based on defined conditions. Two fundamental directives that you will encounter frequently are `#ifdef` and `#ifndef`.
Definition and Purpose
`#ifdef` (if defined) checks if a particular macro is defined. It allows you to include or exclude sections of code depending on whether certain conditions are met. On the other hand, `#ifndef` (if not defined) checks if a macro is not defined, providing a way to include code only if the macro has not been previously defined.
Code Snippets and Examples
For instance, consider the usage of `#ifdef`:
#define DEBUG
#ifdef DEBUG
std::cout << "Debug mode is enabled." << std::endl;
#endif
In the above code, the message will be printed only if `DEBUG` is defined.
In contrast, here’s an example of `#ifndef`:
#ifndef MY_HEADER_H
#define MY_HEADER_H
// Header content here
#endif
This construct is commonly used in headers to prevent multiple inclusions of the same header file.
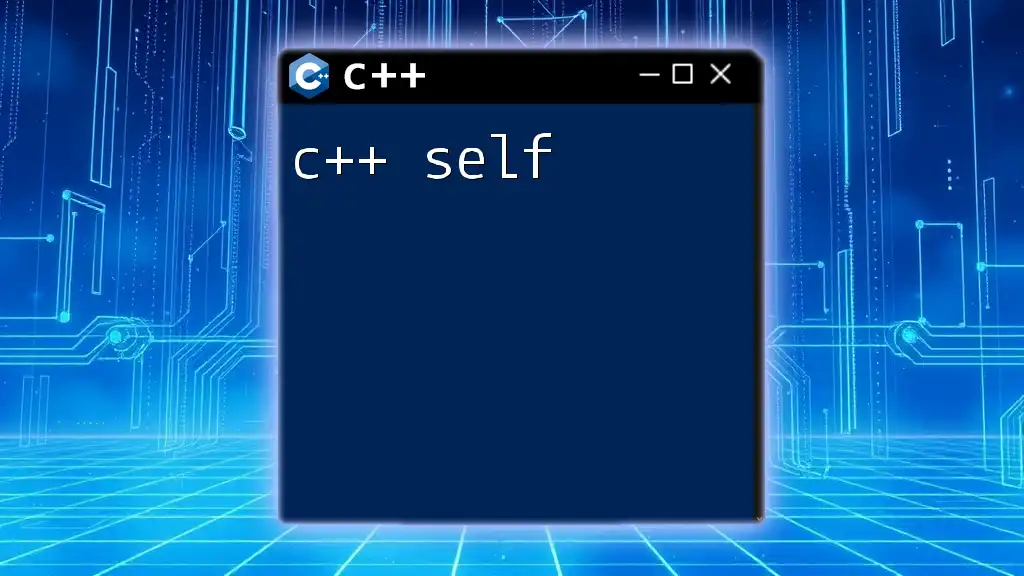
Introduction to `#elif`
`#elif` in C++ serves as a shorthand for "else if" in preprocessor directives. It offers a clean way to chain multiple conditions together, allowing for more organized and readable code.
Syntax of `#elif`
To use `#elif`, it’s important to understand its syntax within a series of directives:
#if condition1
// code block
#elif condition2
// code block
#else
// code block
#endif
This structure enables you to evaluate multiple conditions sequentially, culminating in a single `#endif`.
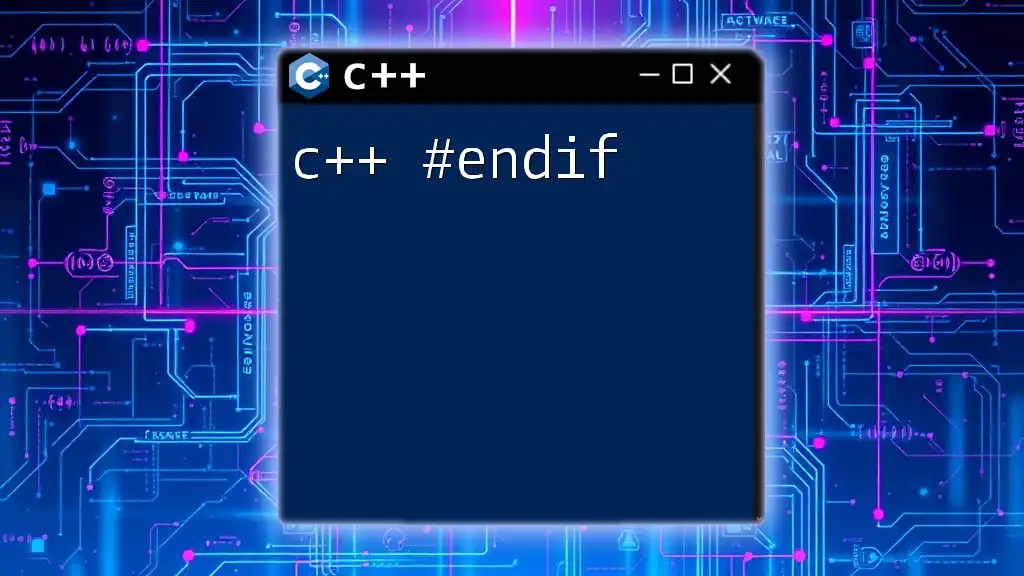
How to Use `#elif` in C++
Basic Usage
When should you use `#elif`? This directive is particularly useful in situations where you have multiple conditions to check. Instead of nesting multiple `#if` statements, which can become cumbersome, `#elif` provides a more streamlined approach.
Example of Using `#elif`
Consider this example, which demonstrates how to use `#elif` for platform-specific instructions:
#define OS_WINDOWS
#if defined(OS_WINDOWS)
std::cout << "Running on Windows." << std::endl;
#elif defined(OS_LINUX)
std::cout << "Running on Linux." << std::endl;
#elif defined(OS_MAC)
std::cout << "Running on macOS." << std::endl;
#else
std::cout << "Unknown operating system." << std::endl;
#endif
In this scenario, the preprocessor checks each defined condition in order, printing the corresponding output based on the operating system defined.
Explaining the Example
The example above clearly illustrates how `#elif` manages to check multiple conditions without complicating the code. This clarity is essential, especially in larger projects where maintainability is key.
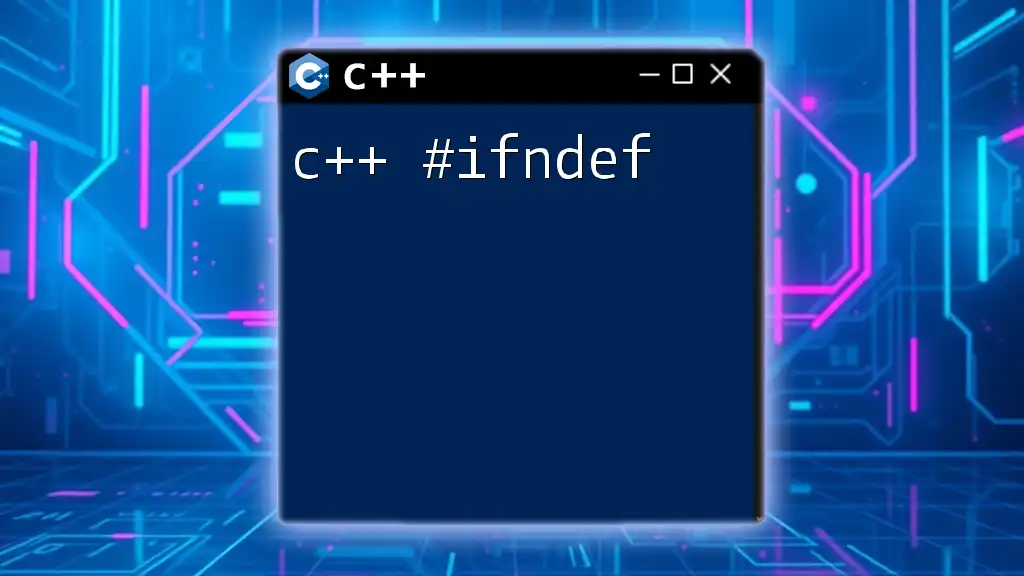
Best Practices for Using `#elif`
Maintaining readability and maintainability is vital when using `#elif`. Here are a few tips:
Readability and Maintainability
To enhance readability, ensure that each condition is self-explanatory. Keeping conditions short and relevant makes it easier for future developers (or yourself) to understand the logic quickly.
Avoiding Complexity
How many `#elif` conditions should you have? It’s good practice to limit the number of checks; otherwise, your code can become unwieldy. If you find yourself using too many `#elif` statements, it may be time to reassess your design or consider simplifying the logic.
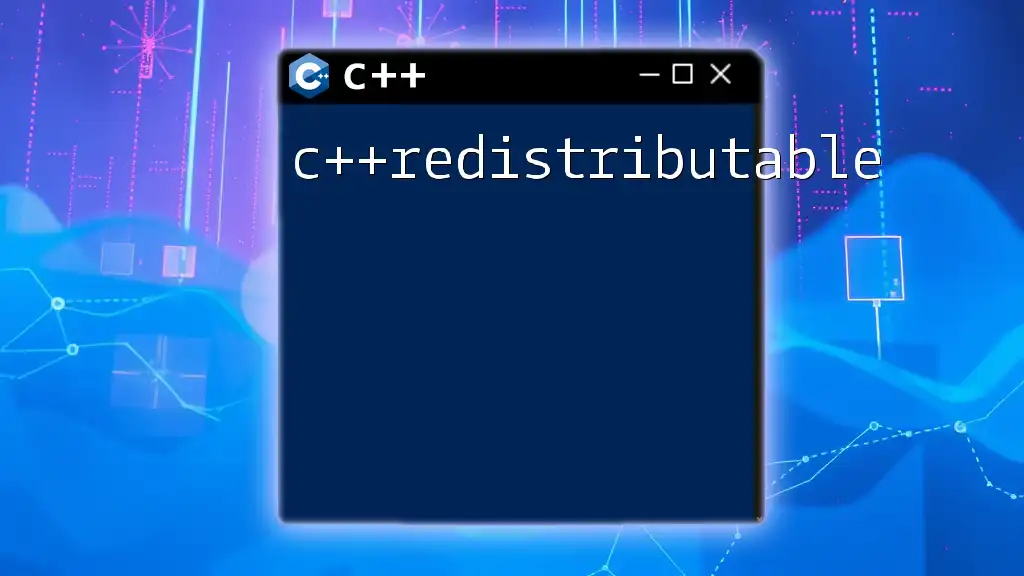
Common Mistakes with `#elif`
Missing `#endif`
A frequent pitfall is forgetting to include `#endif` after your conditional blocks. This omission can lead to compilation errors that may be frustrating to debug.
Overusing `#elif`
While `#elif` is a powerful tool, using too many conditions can obscure the logic of your program. Always strive for a balance that maintains both functionality and readability.
Not Accounting for All Possible Conditions
Failing to account for all possibilities may leave your code susceptible to errors. It's crucial to include a default case (via the `#else` clause) to manage unexpected conditions effectively.
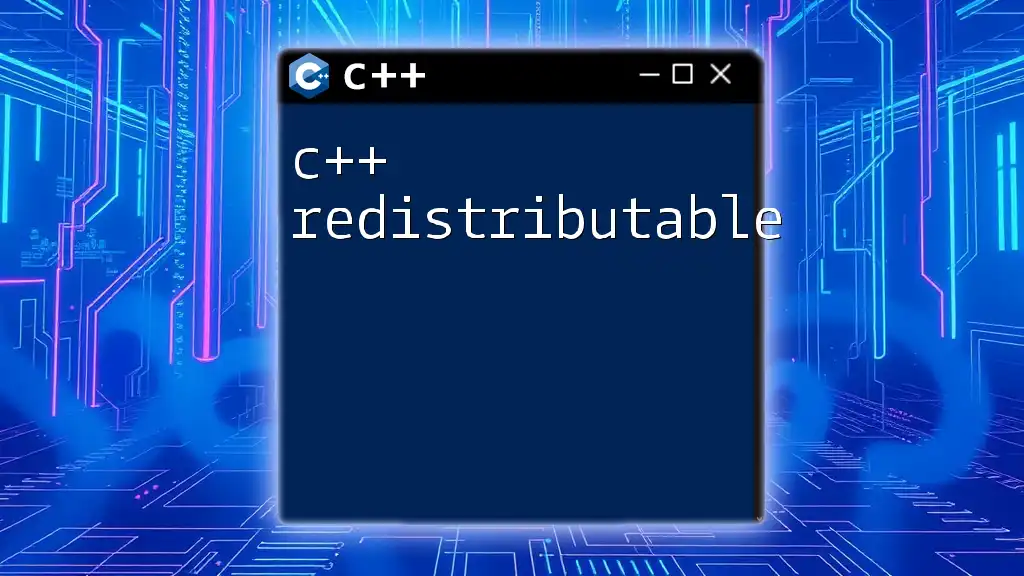
Comparing `#elif` with Other Conditional Directives
Understanding the distinctions between `#elif`, `#if`, and `#else` can be essential for writing clean code.
Key Differences and Use Cases
- `#if`: Use when you have a single condition that may evaluate to true or false.
- `#elif`: Ideal for handling multiple conditions in a clear and concise manner.
- `#else`: Acts as a final fallback when none of the preceding conditions are satisfied.
Example Comparison
Here’s a code snippet illustrating the differences:
#define VALUE 2
#if VALUE < 1
std::cout << "Less than 1" << std::endl;
#elif VALUE < 3
std::cout << "Less than 3" << std::endl; // This will be executed
#else
std::cout << "3 or greater" << std::endl;
#endif
In this case, using `#elif` captures distinct conditions that each yield different outputs based on the value of `VALUE`.
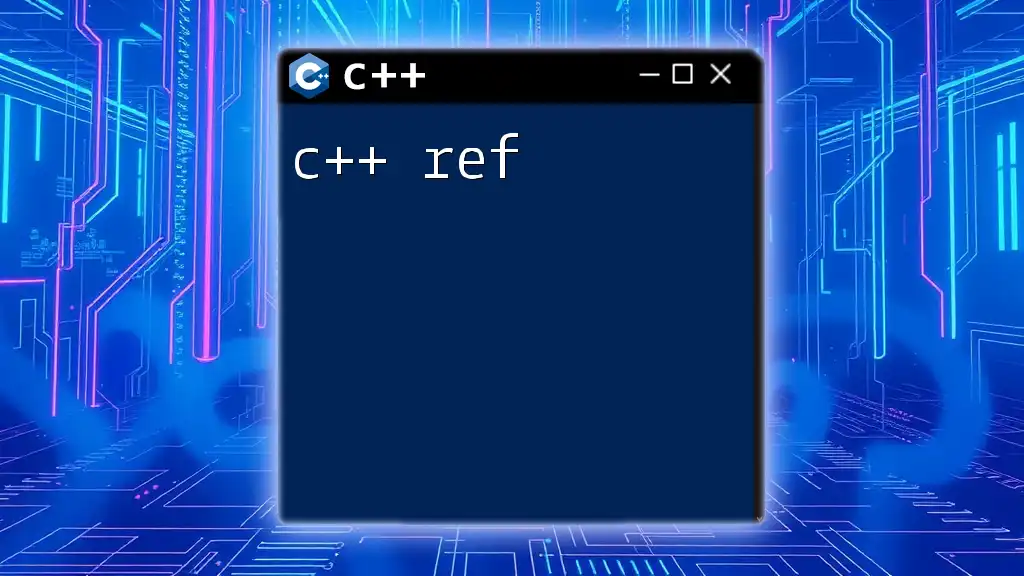
Real-world Applications of `#elif`
Use Cases in Professional Development
In actual software development scenarios, `#elif` becomes invaluable for managing platform-specific code or conditional compilation for debugging.
Code Snippet Example in a Large Project
#define DEBUG_MODE
#if defined(DEBUG_MODE)
// Debug specific code
#elif defined(DEPLOY_MODE)
// Deployment code
#else
// Normal execution
#endif
This example illustrates how `#elif` can be employed in larger codebases to handle different operational modes, enabling developers to compile only the relevant blocks of code based on predefined conditions.
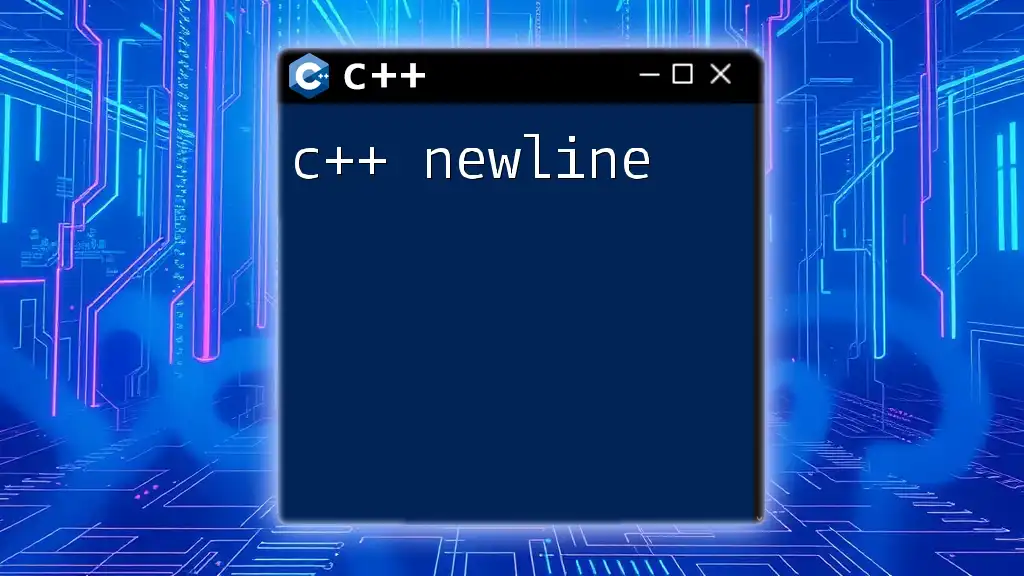
Conclusion
In summary, mastering the C++ `#elif` directive is pivotal for writing efficient and maintainable code. By leveraging this powerful tool, you can enhance your code's clarity and functionality, making it easier to debug and collaborate on. Always remember the importance of structuring your conditions logically and keeping your codebase clean. Embrace `#elif` as you refine your C++ programming skills!
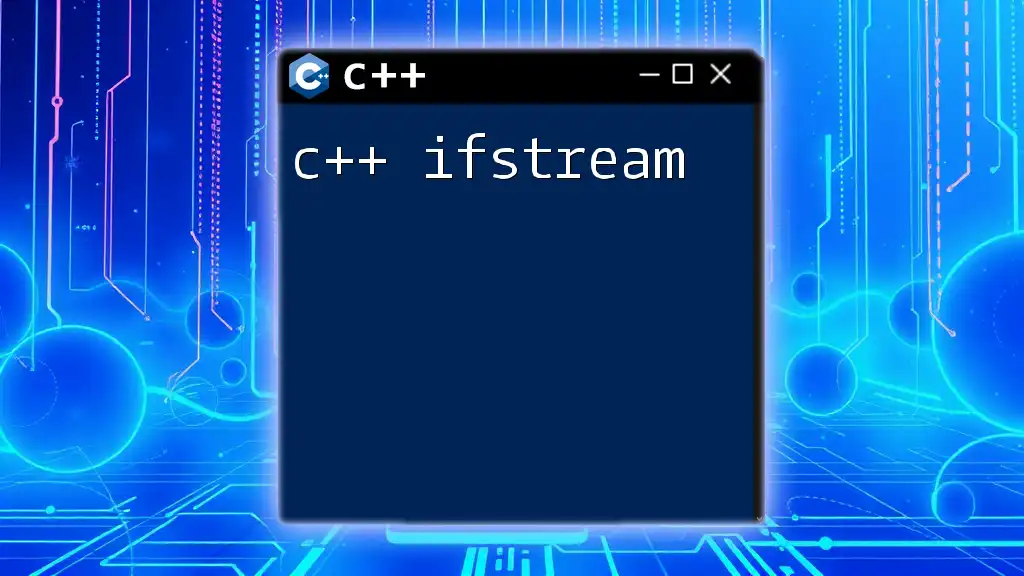
Additional Resources
For further learning, consider exploring online tutorials, official documentation, and books focused on C++ programming. Engaging with a community of programmers can provide insights and examples that deepen your understanding of preprocessor directives like `#elif`.