The `if` statement in C++ allows you to execute a block of code conditionally based on whether a specified expression evaluates to true.
Here's a basic example:
#include <iostream>
using namespace std;
int main() {
int number = 10;
if (number > 5) {
cout << "Number is greater than 5" << endl;
}
return 0;
}
Understanding the Syntax of If Statements in C++
Basic Syntax of If Statement in C++
The if statement is a fundamental conditional statement in C++ that allows you to execute a block of code only if a specified condition evaluates to true. The basic structure of an if statement looks like this:
if (condition) {
// code to execute if condition is true
}
Condition refers to a boolean expression (one that returns true or false). When the condition is true, the block of code within the braces `{}` will run. If the condition is false, the code inside the block will be skipped.
If Statement in C++ Syntax Breakdown
An if statement consists of three main components:
- The Keyword: `if`
- The Condition: placed inside parentheses `()`, which is evaluated to determine if the block should execute.
- The Code Block: enclosed in braces `{}`, which contains the code that runs if the condition is true.
It’s important to remember:
- Always use parentheses `()` around your condition.
- The braces `{}` are optional if only one statement is executed, but it is considered good practice to always use them for readability and maintainability.
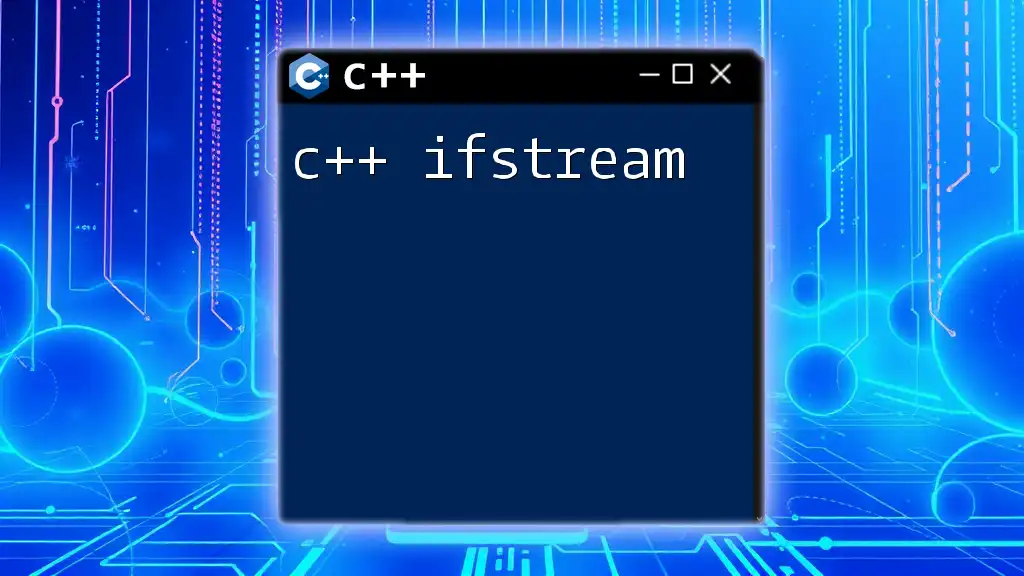
How to Write an If Statement in C++
Crafting Your First C++ If Statement
Writing your first if statement is straightforward. Here’s how you can do it in practice:
int x = 10;
if (x > 5) {
std::cout << "x is greater than 5.";
}
In this example, the condition `x > 5` is true, so the message will be printed. If the value of x were 4, for example, the message wouldn’t be displayed.
Using Else and Else If Statements in C++
To handle more than two conditions, you can use else and else if statements to provide additional logic. Here's how that works:
if (x > 5) {
std::cout << "x is greater than 5.";
} else if (x == 5) {
std::cout << "x is equal to 5.";
} else {
std::cout << "x is less than 5.";
}
In this code, three different outcomes can be printed based on the value of `x`. Each condition is checked in the order they appear, and once a true condition is found, the corresponding code block executes.
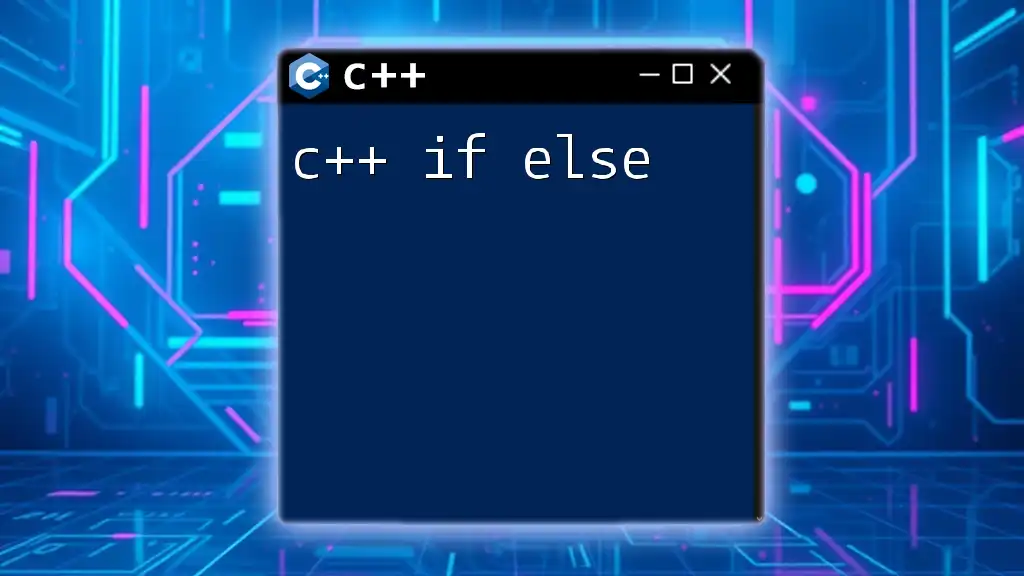
Exploring If Then Statements in C++
Definition of If Then Statements in C++
The term if then refers to the logic where actions are taken only if certain conditions are met. In C++, this is effectively implemented through the if statement. It defines scenarios where something needs to happen based on a condition:
Example of If Then in C++
Here’s a simple example illustrating an if statement:
int y = 20;
if (y < 25) {
std::cout << "y is less than 25.";
}
In this case, since `y` is indeed less than 25, the message will be displayed, demonstrating the clear "if then" logic.
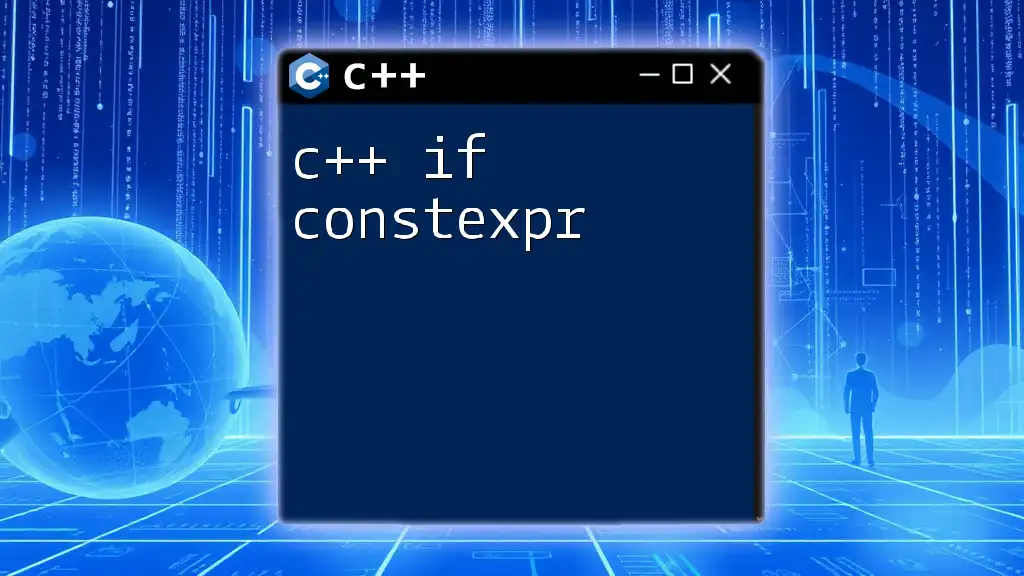
Advanced If Statement Techniques
Nested If Statements in C++
Nested if statements allow for more complex decision-making by placing an if statement inside another if statement. This feature is useful when multiple conditions must be checked in relation to each other.
int z = 15;
if (z > 10) {
if (z < 20) {
std::cout << "z is between 10 and 20.";
}
}
Here, the outer if checks if `z` is greater than 10. If true, it checks the inner condition, providing an additional layer of logic.
Using Logical Operators with If Statements in C++
C++ provides logical operators such as AND (`&&`) and OR (`||`) to combine multiple conditions within a single if statement.
if (x > 5 && y < 25) {
std::cout << "Both conditions are true.";
}
In this example, both conditions must be true for the block of code to execute. Logical operators can help refine your decision-making processes and streamline your code.
C++ If Or: Multiple Conditions
If you want to execute a block of code when at least one of multiple conditions is true, you can use the OR operator `||`.
if (x < 5 || y < 25) {
std::cout << "At least one condition is true.";
}
In this case, if either `x` is less than 5 or `y` is less than 25, the message will be printed.
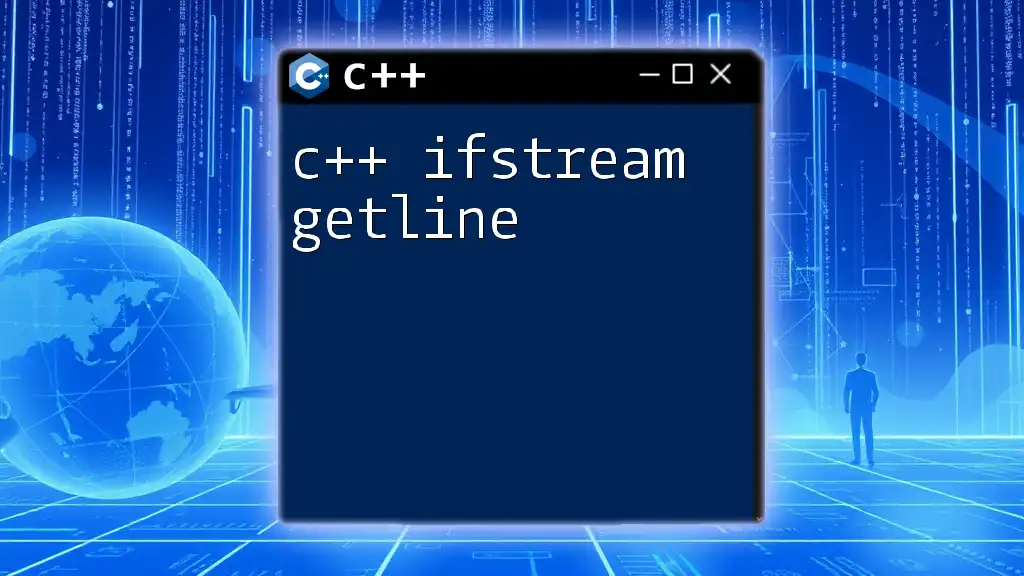
Special Cases of If Statements in C++
The C++ #if Directive
In addition to runtime if statements, C++ also has compile-time conditions with the `#if` preprocessor directive. This is helpful for conditional compilation based on defined constants.
#define CONDITION 1
#if CONDITION
std::cout << "Condition is true.";
#endif
Using the `#if` directive, you can include or exclude code snippets conditionally, which is particularly useful in library development or settings where different configurations are needed.
If Loop in C++
While if statements control the flow of execution based on conditions, they should not be confused with loops. Loops iterate through a block of code multiple times depending on a condition, while if statements make decisions about executing code once. Understanding this distinction is essential for writing effective C++ programs.
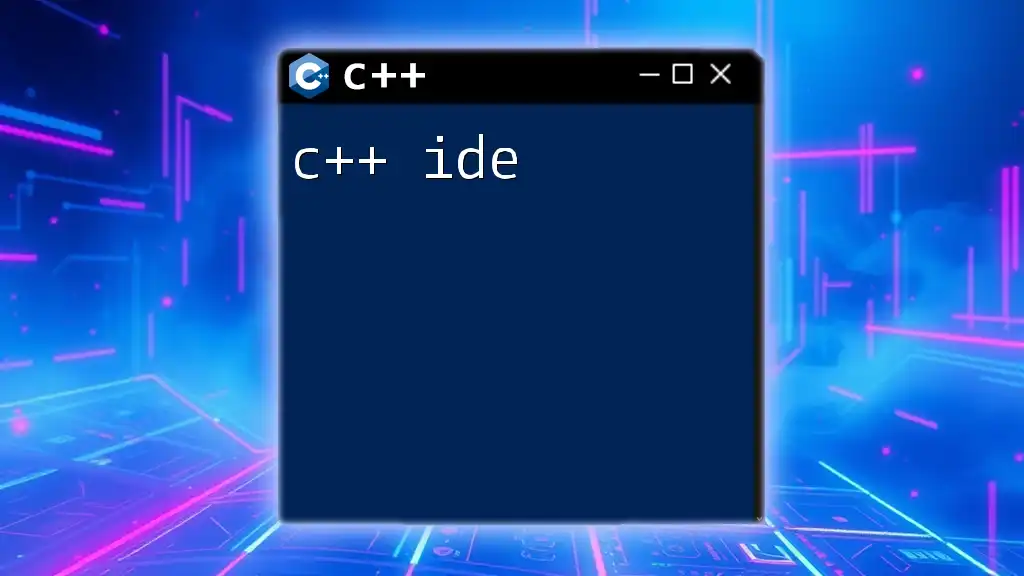
Practical If Statement Examples
Real-life C++ If Statement Example
Real-life scenarios often require user input to make decisions. Here's an example that assesses a user's age:
int age;
std::cout << "Enter your age: ";
std::cin >> age;
if (age >= 18) {
std::cout << "You are an adult.";
} else {
std::cout << "You are a minor.";
}
In this instance, the program evaluates the user's age and displays a message based on whether they are an adult or a minor.
Combining If Statements with Cases
Sometimes, you might want to handle different cases based on a single condition. This is where if statements can serve as an alternative to switch-case statements in C++.
char grade = 'A';
if (grade == 'A') {
std::cout << "Excellent!";
} else if (grade == 'B') {
std::cout << "Well done!";
}
// ... more cases
Here, different messages are printed based on the value of the variable `grade`. This usage emphasizes the versatility of if statements within your coding practices.
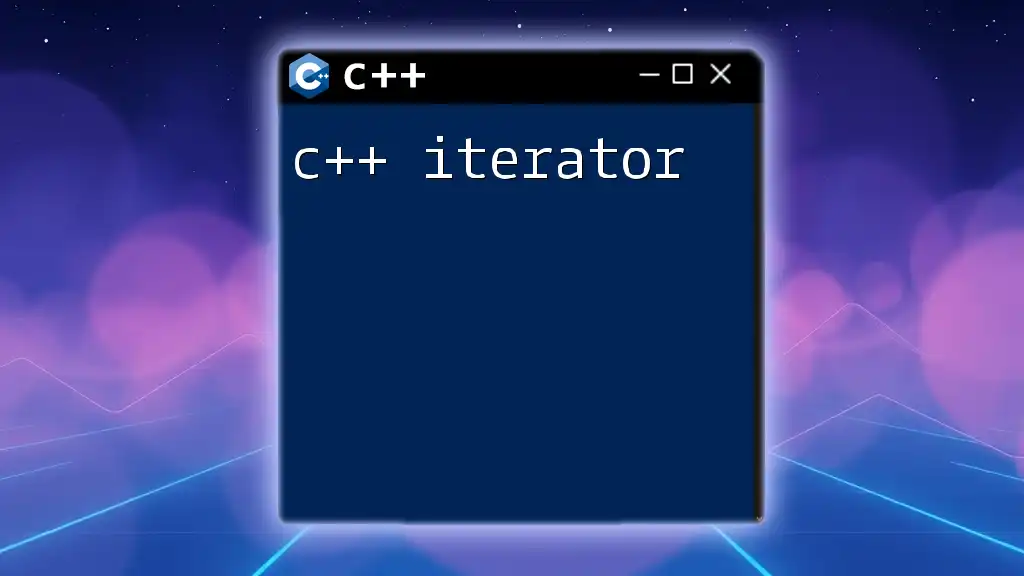
Conclusion
The C++ if statement is a powerful tool for controlling the flow of execution in your programs. By understanding its syntax and capabilities, you can make informed decisions within your code, leading to cleaner and more maintainable programming practices. Whether through simple conditions or more complex nested structures, mastering if statements is crucial for any aspiring C++ developer.
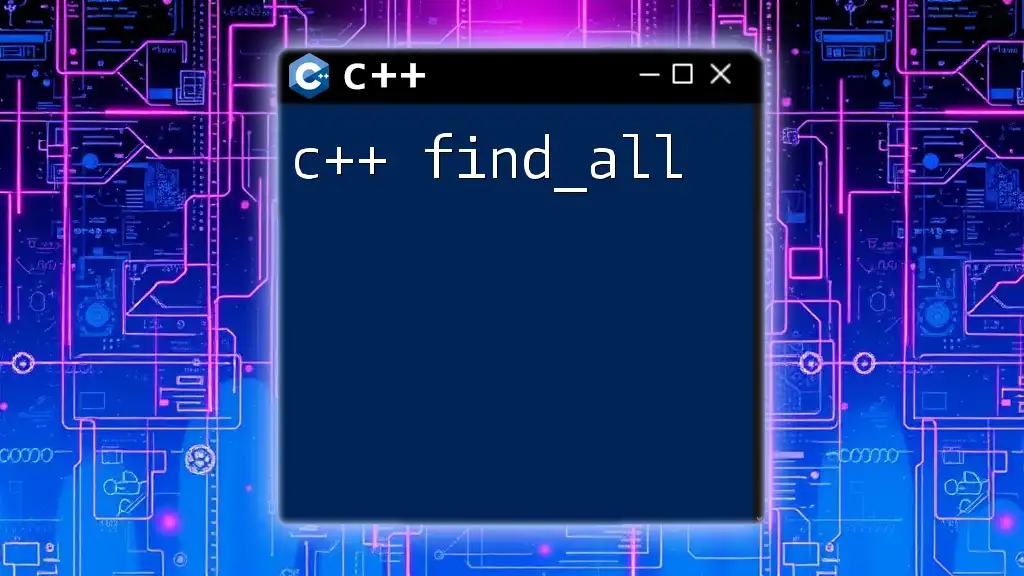
Final Thoughts and Next Steps
For those looking to deepen their knowledge of C++, mastering if statements is just the beginning. With practices on crafting robust and efficient conditions in your code, following up with references on loops, functions, and classes will further solidify your understanding of this versatile programming language. Stay tuned for more articles that explore C++ and programming best practices!