C++ IDEs (Integrated Development Environments) provide a comprehensive platform for writing, debugging, and executing C++ code efficiently, facilitating a smoother coding experience.
Here's a simple "Hello World" program written in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is an IDE?
An Integrated Development Environment (IDE) is a comprehensive software application that consolidates essential tools for software development. IDEs provide a user-friendly interface for writing, testing, and debugging code, simplifying the development process significantly.
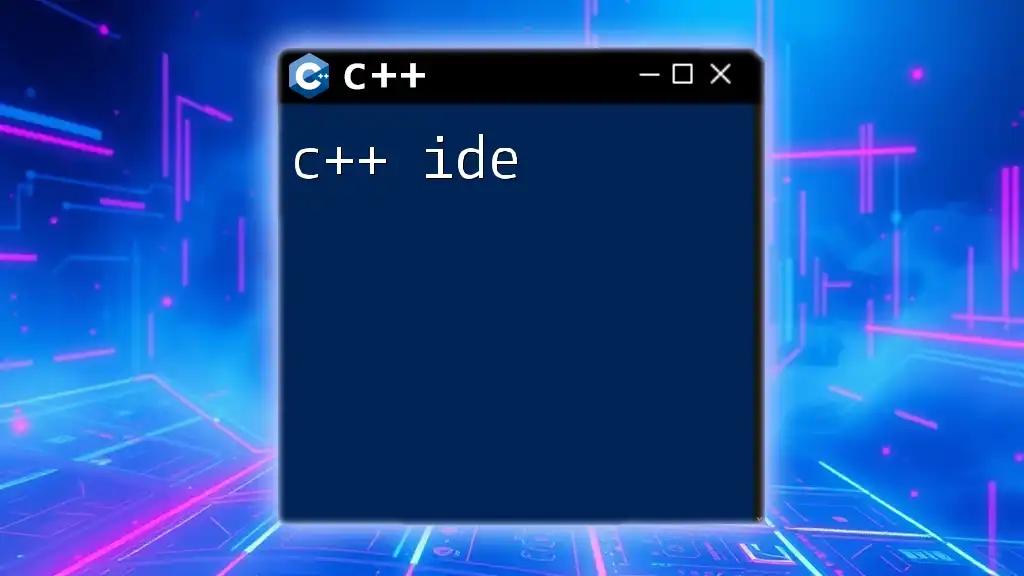
Why Choose a C++ IDE Over a Text Editor?
While text editors can be useful for C++ coding, IDEs offer numerous advantages that enhance productivity. IDEs typically include robust features such as syntax highlighting, code completion, and integrated debugging tools. This integration allows developers to focus on coding rather than managing multiple tools, making the development workflow smoother and more efficient.
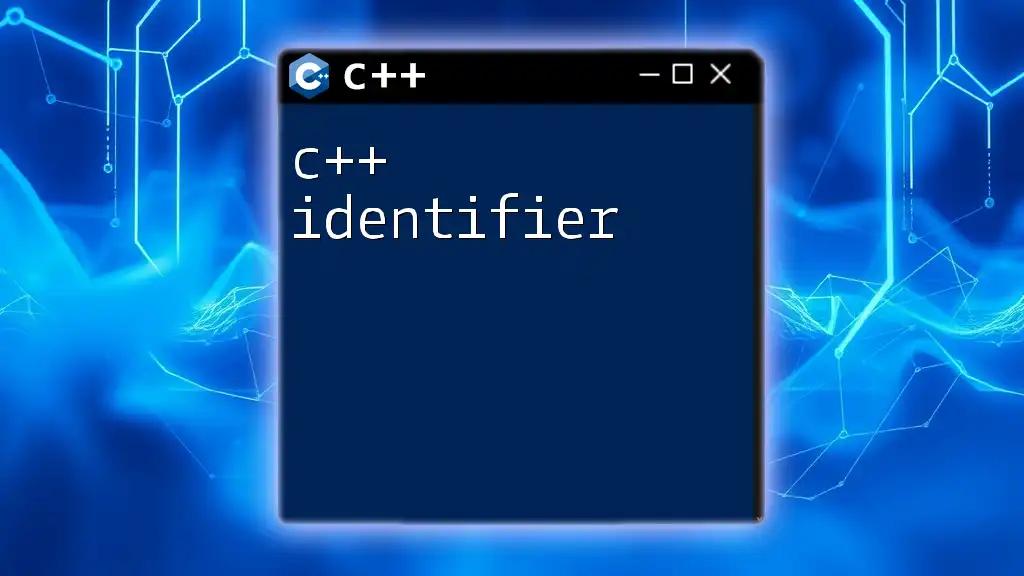
Key Features to Look for in a C++ IDE
Code Editing Features
High-quality code editing features are essential. An effective C++ IDE should have:
- Syntax Highlighting: Color-coding helps to easily differentiate between keywords, variables, and other components of your code. This enhances readability and can prevent errors.
- Code Completion: Known as intelligent code suggestions, this feature speeds up coding by suggesting possible completions for partially typed code. This is particularly helpful for remembering function names and parameters.
- Refactoring Tools: An IDE should provide facilities for refactoring code, which includes renaming variables and restructuring code without changing its functionality.
Debugging Capabilities
Debugging is a critical aspect of programming. An efficient C++ IDE should include:
- Breakpoints: Allowing you to pause program execution at a certain line of code enables you to inspect variables and control flow at that point.
- Step-through Debugging: This feature allows you to execute your code line by line, giving you insight into how the application runs and facilitating easier bug identification.
- Variable Inspection: A good IDE allows you to examine the current state of variables, which is crucial when tracking down bugs.
Build and Compilation Tools
Building and compiling your code quickly is pivotal for efficient development. Look for an IDE that offers:
- Integrated Build Systems: Features like Makefile support or CMake integration help streamline the build process, simplifying project configuration.
- Compilation Error Highlighting: Immediate feedback on syntax errors or compilation problems aids in rapid development and continuous improvement.
Version Control Integration
Version control is vital for tracking changes in code and collaborating with others. An IDE should seamlessly support:
- Git or SVN Support: Integrated GUI tools for managing branches, commits, and merges within your IDE can save you time and effort.
- Branch Management and Conflict Resolution: A solid IDE can simplify the process of managing changes and resolving conflicts in collaborative settings.
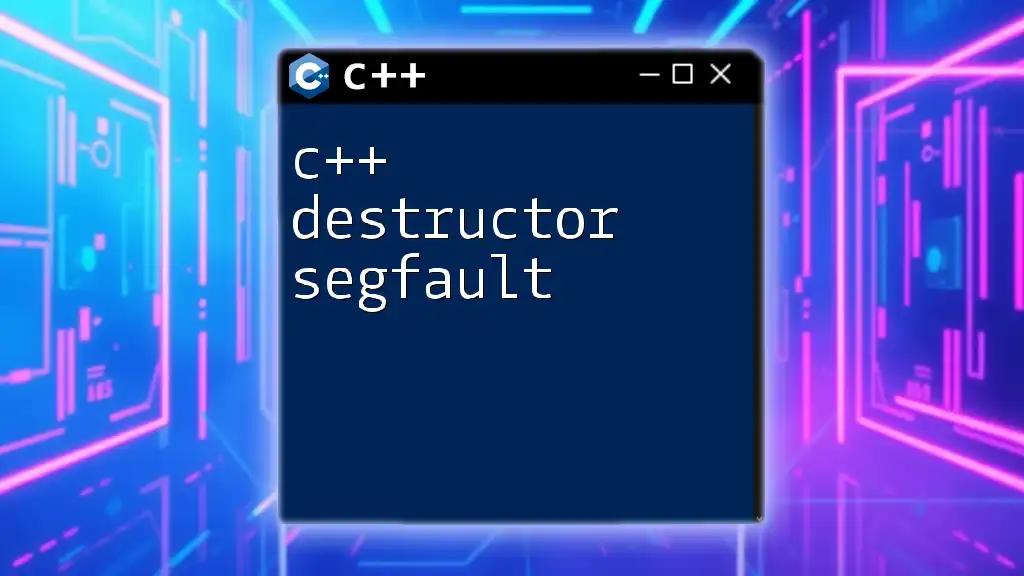
Popular C++ IDEs
Visual Studio
Overview: Visual Studio is a powerful IDE developed by Microsoft, known for its deep integration with Azure and a wide array of development tools. It is especially popular among Windows developers.
Pros and Cons:
- Advantages:
- IntelliSense: Offers great code completion and error detection.
- Built-in Debugger: Comprehensive debugging capabilities make it a favorite among developers.
- Drawbacks:
- Resource Consumption: Can be demanding on system resources, making it less suitable for older machines.
Code::Blocks
Overview: A free and open-source IDE that caters to both beginners and experienced developers. Code::Blocks is lightweight and highly configurable.
Pros and Cons:
- Flexibility: Easy to extend via plugins.
- Simplicity: A straightforward user interface with basic features.
- Limitations: Might lack some advanced features found in other more robust IDEs.
JetBrains CLion
Overview: CLion is a cross-platform IDE specializing in C and C++. It offers advanced code analysis and is designed to work well with CMake.
Pros and Cons:
- Smart Code Completion: Offers powerful completion and navigation features.
- Continuous Integration Support: Integrated testing and deployment tools.
- Cost: Subscription-based model might be a drawback for some.
Eclipse CDT
Overview: Eclipse is a widely-used open-source IDE featuring a plug-in architecture. The C/C++ Development Tooling (CDT) extension allows C++ development.
Pros and Cons:
- Strong Community Support: A wide range of plugins and extensive documentation.
- Heavy Setup: Can be time-consuming to configure properly for C++ development.
Xcode (for macOS users)
Overview: Apple's official IDE for macOS and iOS development, which supports C++ among other languages.
Pros and Cons:
- Native macOS Support: Great for developers targeting Apple platforms.
- Limited Cross-Platform Features: Less ideal if developing for non-Apple systems.
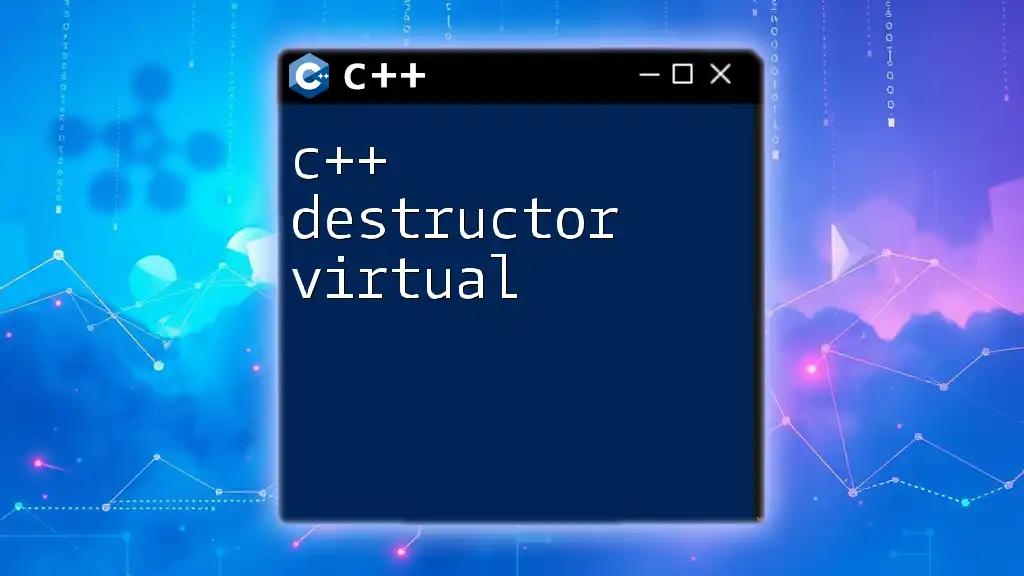
How to Choose the Best CPP IDE for Your Needs
Consider Your Project Type
Different projects have different requirements. Small projects may not need the extensive features of a heavy IDE, while large-scale applications might require powerful tools for managing complexity.
Evaluate Your Skill Level
Your experience level not only affects which IDE will be comfortable to use but also how effectively you can utilize its features. Beginners might prefer simpler environments, while advanced users would benefit from extensive debugging and version control features.
Compatibility with Platforms
Consider whether you need a cross-platform IDE that runs across Windows, macOS, and Linux, or if you can opt for something that is more specific to a single operating system.
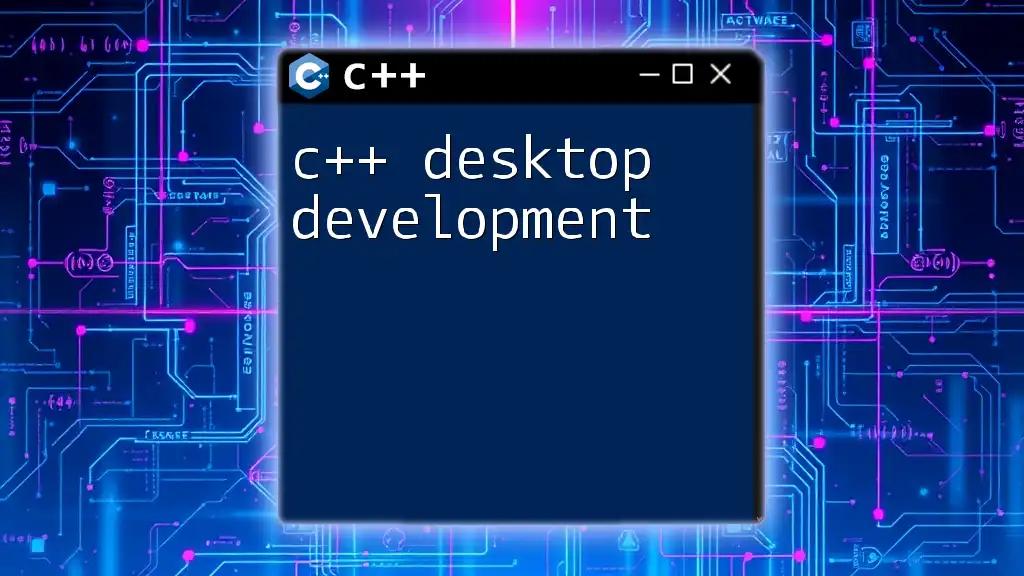
C++ Coding Environments: Beyond Just an IDE
Command Line Tools
For advanced users, command line tools like G++ and Make are invaluable. Using terminal commands can lead to a deeper understanding of the building and execution processes, allowing you to optimize your workflow.
Online IDEs
In recent years, online IDEs such as Repl.it and OnlineGDB have gained popularity. They provide quick and easy ways to experiment with C++ coding in the browser, although they may lack some of the robust features of desktop IDEs. Considerations for online IDEs include:
- Accessibility: No installation required.
- Collaboration Tools: Many allow real-time coding with peers.
- Performance Limitations: May not be suitable for larger projects.
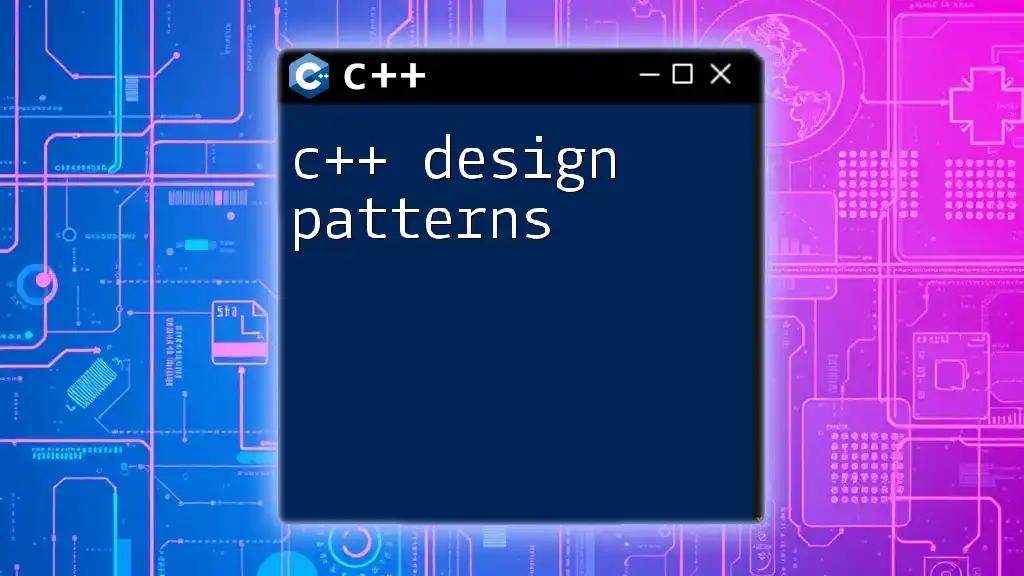
Setting Up Your IDE for C++ Development
Installation Guide
Visual Studio: Download and run the installer. Choose the "Desktop development with C++" workload to get started.
Code::Blocks: Download the installer, which often includes the MinGW compiler bundle for ease of setup. Follow the prompts to complete the installation.
Configuring Build Settings
Setting up build configurations will vary by IDE. In Visual Studio, you can create a new project and specify settings such as compiler options directly in the project properties window. For Code::Blocks, make sure to define your build targets correctly to ensure the proper compiler is invoked.
Extensions and Plugins
Most IDEs have ecosystems of plugins that can enhance functionality. For instance, in Visual Studio, you might want to explore extensions that enhance code formatting or provide better integration with Git.
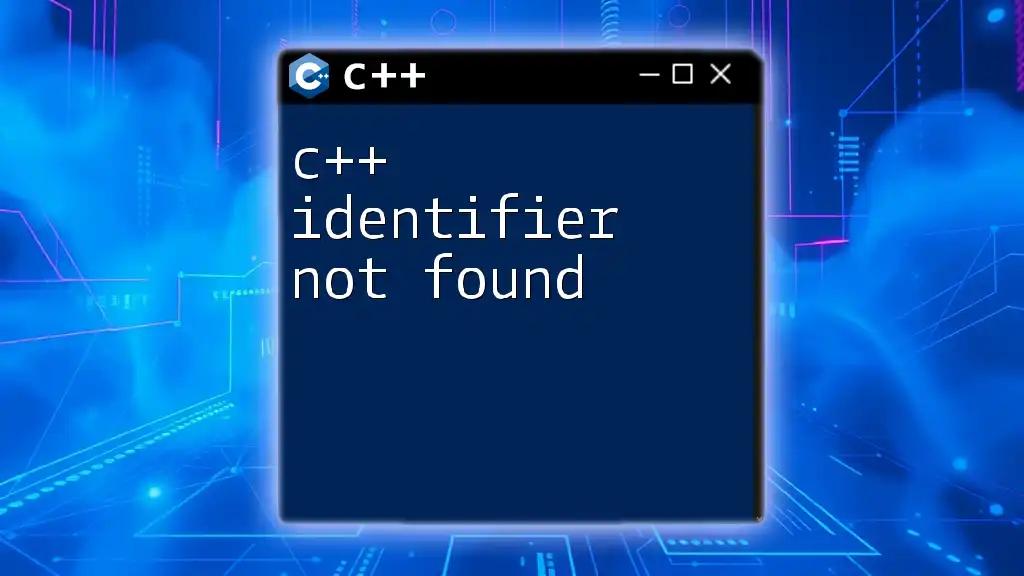
Examples and Code Snippets
Sample Project Structure
Organizing your project files can significantly impact your development efficiency. A typical structure might look like this:
/MyProject
/src
main.cpp
/include
myheader.h
/lib
external_lib.a
Makefile
Writing and Compiling Your First C++ Program
To get started with C++ coding, begin with a simple program. Here’s an example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile this in an IDE, create a new project, add the `main.cpp` file, and run the build command. Most IDEs make this as easy as hitting a “Run” button.
Debugging a C++ Application
Assume you have the following code with a potential bug:
#include <iostream>
int main() {
int x;
std::cout << "Enter a number: ";
std::cin >> x;
std::cout << "The number entered is: " << x << std::endl;
return 0;
}
Using Visual Studio, you would set a breakpoint on the line `std::cin >> x;`, run the program in debug mode, and inspect the value of `x` to ensure proper behavior.
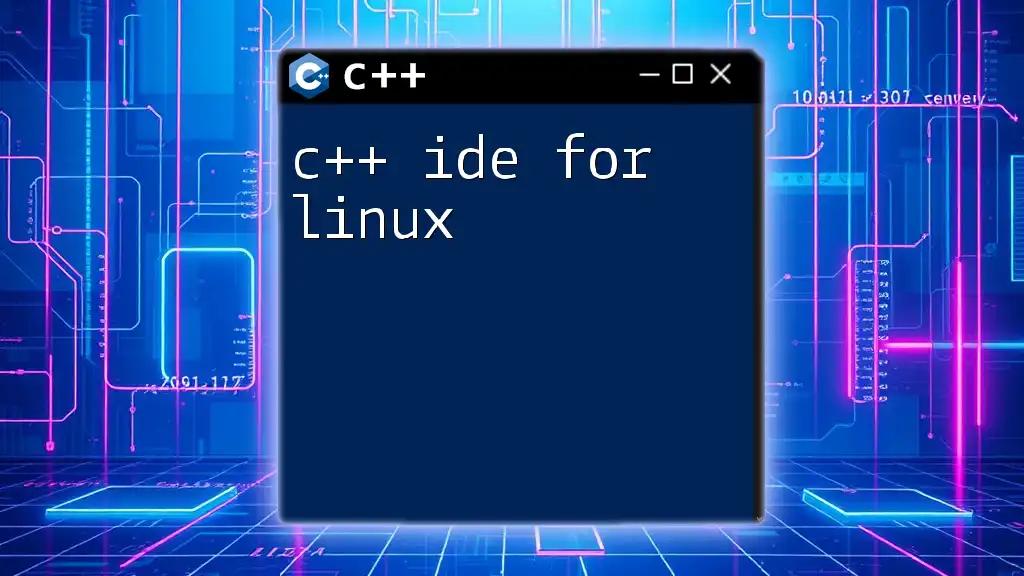
Conclusion
C++ IDEs are crucial tools that can significantly improve your programming productivity. By understanding key features, evaluating your needs, and experimenting with various options, you can identify the right C++ IDE that aligns with your development style and project requirements.
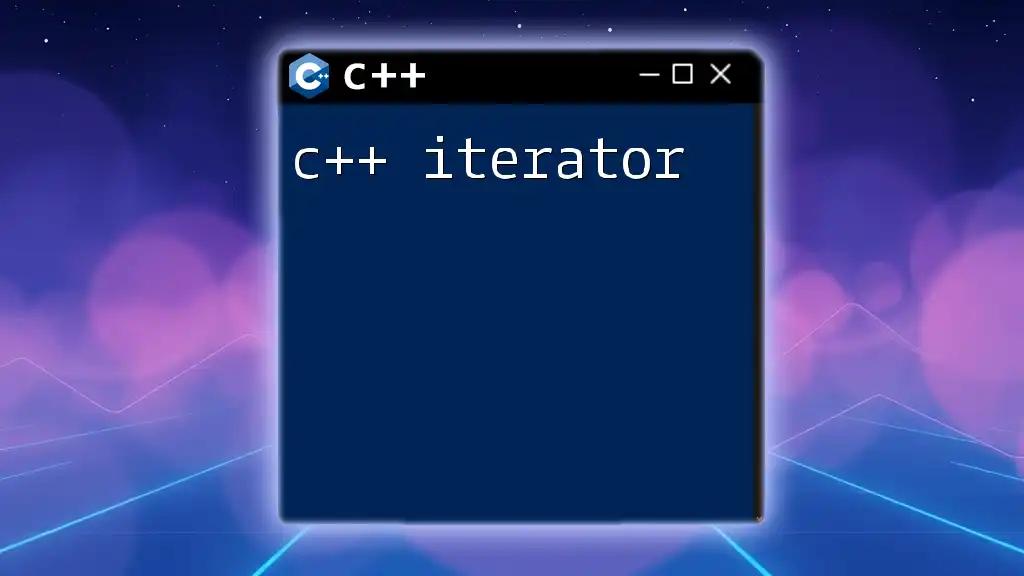
Call to Action
Get Started Today! Explore and compare different C++ IDEs to enhance your coding environment and boost your productivity.
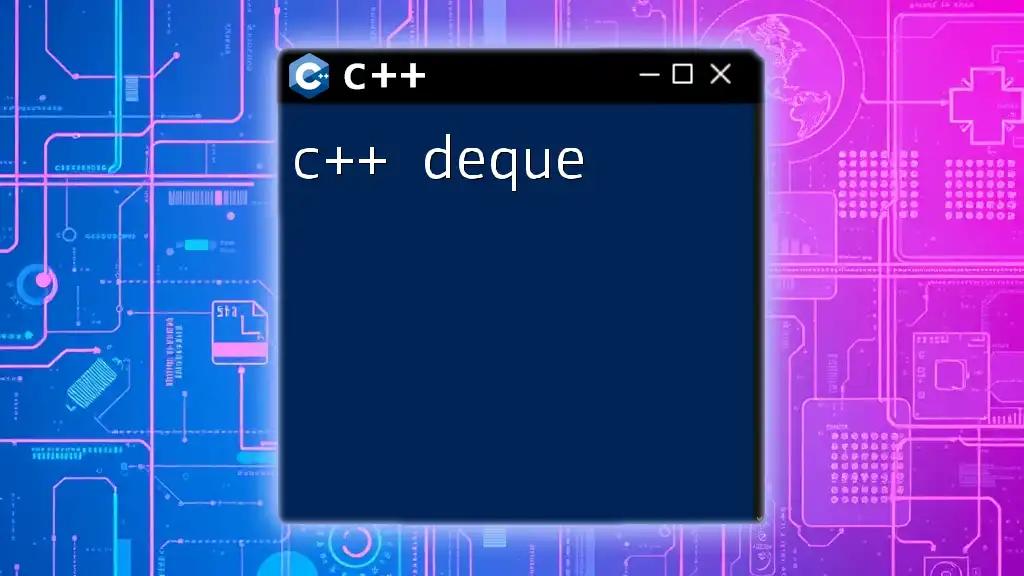
FAQs
What is the best C++ IDE for beginners?
For beginners, Code::Blocks or Eclipse CDT is often recommended for their straightforward interfaces.
Are there free C++ IDEs available?
Yes, there are several options such as Code::Blocks, Eclipse, and GNU Emacs that are open-source and free to use.
Can I use multiple IDEs for a single project?
Absolutely! Many developers choose multiple IDEs depending on the specific tasks they are working on—whether it’s debugging, writing, or compiling.
What features do advanced users prioritize in a C++ IDE?
Advanced users typically look for robust debugging capabilities, code analysis tools, and version control integration to streamline their development process.