A Mac C++ IDE is a powerful integrated development environment specifically designed to facilitate writing, debugging, and compiling C++ code on macOS systems.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a C++ IDE?
An Integrated Development Environment (IDE) is a software application that provides comprehensive facilities to programmers for software development. When it comes to C++, an IDE typically features a code editor, a compiler, a debugger, and project management tools, all integrated into a single platform.
A good C++ IDE should support:
- Code Editing: Syntax highlighting, auto-completion, and error detection to enhance coding efficiency.
- Compiler Integration: Seamless compilation of code without leaving the IDE environment.
- Debugger Support: Tools for debugging code, allowing for step-through execution and variable inspection.
- Project Management: Features that help organize files, dependencies, and configurations effectively.
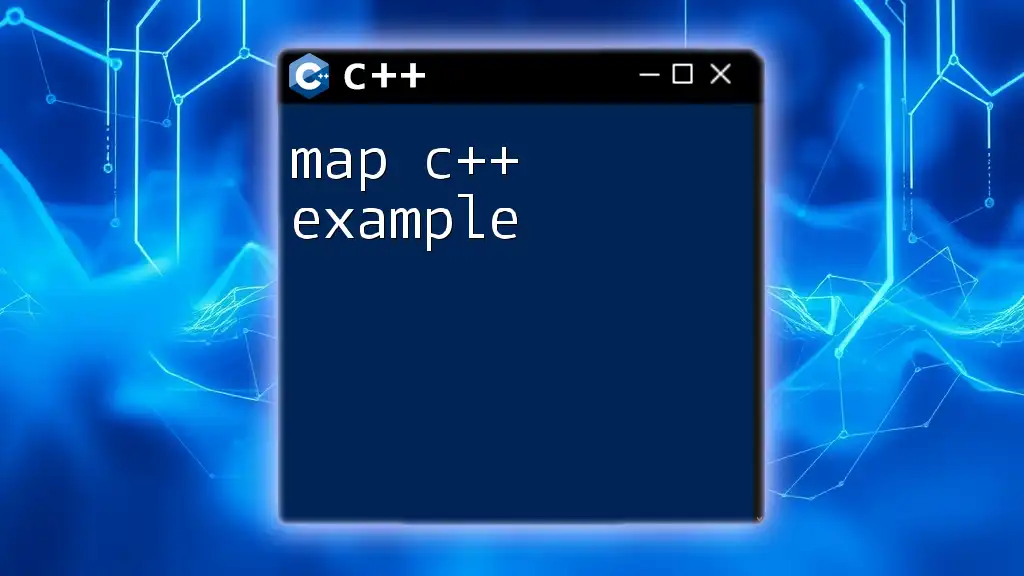
Top C++ IDEs for Mac
Overview of Popular C++ IDEs on macOS
For Mac users, several C++ IDEs provide different features and capabilities. Choosing the right IDE depends on your particular needs, whether you are a beginner, intermediate, or professional developer. Important factors to consider include ease of use, integration with other tools, and specific project requirements.
Xcode: The Premier IDE for C++ on Mac
Xcode is Apple’s official IDE for macOS and is often considered the best option for C++ development on Mac. This IDE is equipped with numerous features for developing C++ applications, including:
- User-friendly interface: Intuitive design that simplifies navigation.
- Powerful debugging: A robust debugger integrated into the IDE that allows you to inspect variables and control execution flow.
To install Xcode, simply download it from the Mac App Store. Once installed, you can create a new C++ project by selecting File > New > Project and choosing a C++ template.
Here’s how a basic C++ “Hello, World!” program looks in Xcode:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Pros of Xcode include seamless integration with macOS features and excellent support for project management. On the downside, its size and complexity can be overwhelming for new users.
CLion: A Powerful IDE for Professional Developers
CLion, developed by JetBrains, is another excellent mac C++ IDE. It supports CMake, which makes it highly portable across various platforms. Key features include:
- Smart code completion: Offers context-aware suggestions as you type.
- Integrated testing support: Facilitates unit testing and helps streamline the debugging process.
To get started with CLion, download and install it from the JetBrains website. After activation, select New Project and choose a CMake template. Here’s a simple code example:
#include <iostream>
int main() {
std::cout << "Hello, from CLion!" << std::endl;
return 0;
}
Pros of CLion include powerful code assistance and excellent support for C++ frameworks. However, it may require a subscription for continued use, which might not be suitable for everyone.
Qt Creator: The Cross-Platform Choice
Qt Creator is best known for developing graphical user interface (GUI) applications but also serves as a competent C++ IDE. Important features include:
- Multiplatform development: Write and test code for various platforms from a single interface.
- Integrated deployment tools: Streamlined tools for deploying applications across different environments.
To install Qt Creator, download it from the Qt website and follow the installation instructions. Creating a simple Qt application looks like this:
#include <QCoreApplication>
#include <iostream>
int main(int argc, char *argv[]) {
QCoreApplication a(argc, argv);
std::cout << "Welcome to Qt Creator!" << std::endl;
return a.exec();
}
Pros of Qt Creator include its versatile project management and extensive library support. The main cons are its focus on GUI development, which may not be necessary for all projects.
Visual Studio Code: A Lightweight Alternative
Although primarily known as a code editor, Visual Studio Code (VSCode) can be transformed into a powerful C++ IDE through various extensions. With features like:
- Lightweight environment: Quick to load, suitable for smaller projects.
- Rich plugin ecosystem: Enhances functionality with compilers, debuggers, and linting tools.
To configure VSCode, install the C/C++ extension from Microsoft via the extensions marketplace. A simple C++ program in VSCode can be written as follows:
#include <iostream>
int main() {
std::cout << "Hello, VSCode!" << std::endl;
return 0;
}
The pros of using VSCode include its customizability and speed, while some cons might be the need for manual configuration for complex features.
Code::Blocks: A Flexible Option
Code::Blocks is an open-source IDE that can be tailored to individual needs. Notable features include:
- Modular architecture: Users can extend functionality through plugins.
- Multi-compiler support: Compatible with various compilers, offering flexibility in building applications.
Installation is straightforward; download from the official Code::Blocks website and follow the prompts. Creating a basic C++ project might look like this:
#include <iostream>
int main() {
std::cout << "Code::Blocks is set up!" << std::endl;
return 0;
}
The pros of Code::Blocks are its adaptability and user-friendly interface, while its cons include a dated interface that may not appeal to everyone.
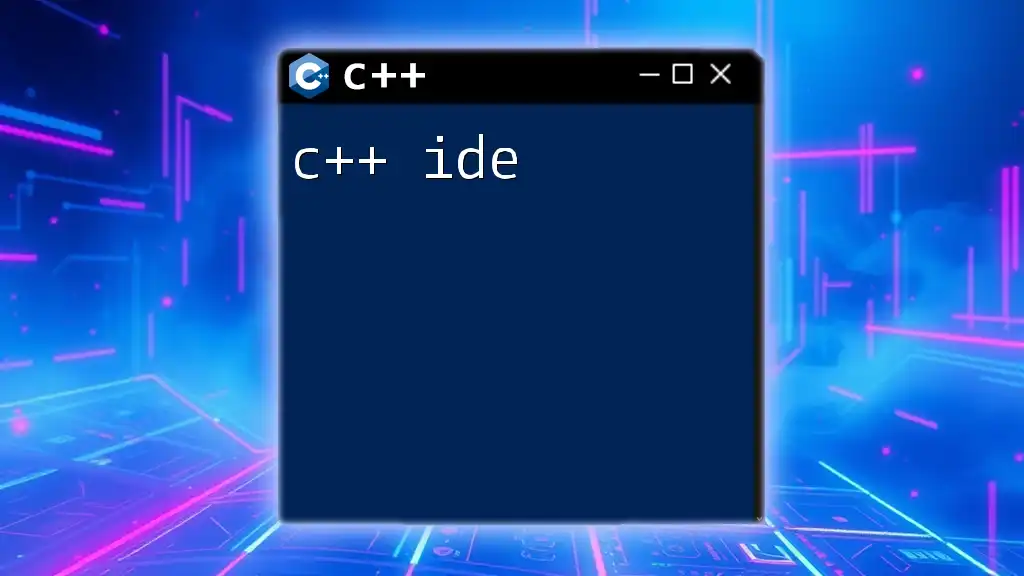
Specialized C++ IDEs for Specific Needs
Eclipse CDT: For Those Familiar with Eclipse
Eclipse CDT is ideal for developers who are already accustomed to the Eclipse IDE environment. It includes features such as:
- Rich set of development tools: Built-in debugging, profiling, and project management tools.
Installation involves downloading Eclipse for C/C++ Developers and setting up the CDT package.
Here’s an example C++ program to run in Eclipse CDT:
#include <iostream>
int main() {
std::cout << "Eclipse CDT is configured!" << std::endl;
return 0;
}
Pros of using Eclipse CDT include its extensive features and customizability, while cons often involve slower performance compared to more streamlined IDEs.
JetBrains ReSharper C++: Boost Your Productivity
While not a standalone IDE, ReSharper C++ is a productivity plugin for IDEs like Visual Studio that provides enhanced tools for C++ development. Key features include:
- Intelligent code analysis: Helps identify potential issues in code.
- Refactoring tools: Streamline code editing and enhance quality.
This tool is perfect for developers looking to enhance their existing IDE workflow but is subscription-based, which may deter some users.
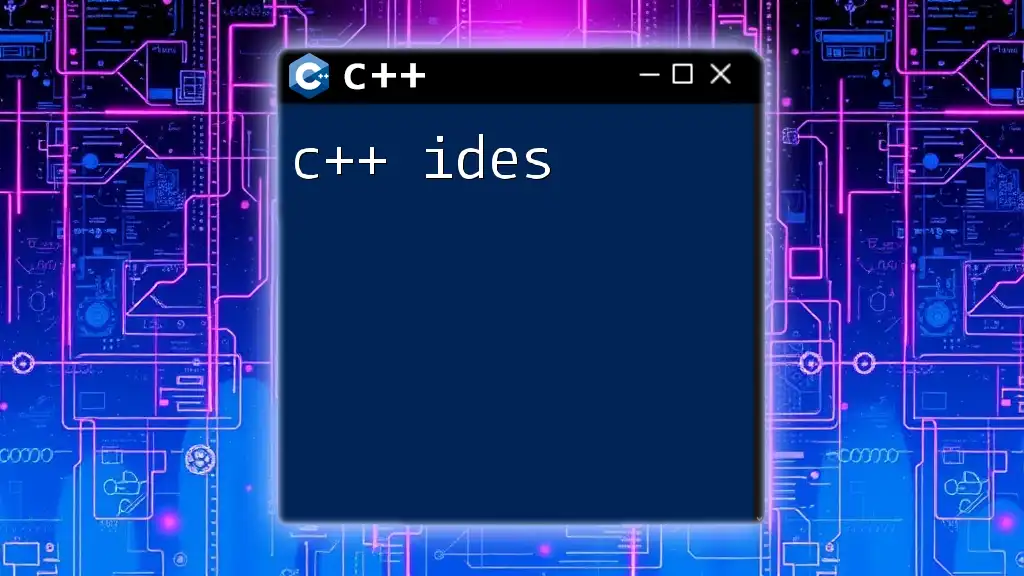
Comparison of Features Among Mac C++ IDEs
When comparing IDEs, consider their features through aspects such as debugging, ease of setup, and community support. Below is a table illustrating what each option provides:
IDE | Debugging | Compile Support | User-friendliness | Customizability |
---|---|---|---|---|
Xcode | Yes | Swift, C++ | High | Low |
CLion | Yes | CMake | Medium | Medium |
Qt Creator | Yes | Qt Toolkit | Medium | Medium |
Visual Studio Code | Yes | Various | High | High |
Code::Blocks | Yes | Multi-compiler | High | High |
Each IDE has unique strengths, and identifying the one that aligns best with your project types and personal preferences is crucial.
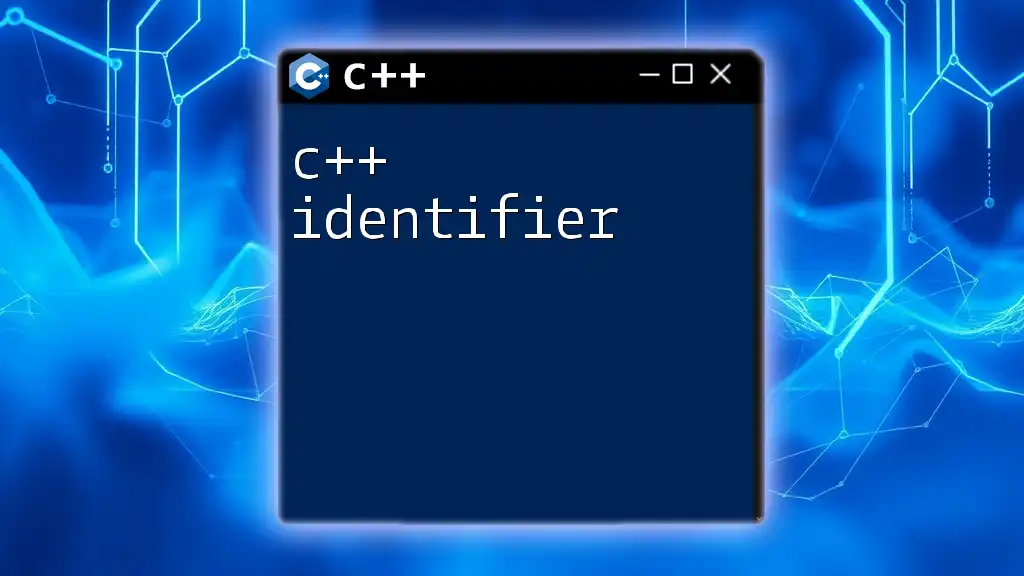
Tips for Choosing the Right C++ IDE on Mac
When deciding on a C++ IDE for Mac, consider the following:
- Project Type: Identify whether your project is console-based or GUI-focused — some IDEs cater specifically to one or the other.
- Development Experience: Look for an IDE that matches your proficiency level. Beginners may prefer simpler interfaces, while experienced developers might appreciate advanced tools.
- Community and Support: Opt for IDEs with a strong community presence for easier troubleshooting and additional resources.
- Future Needs: Ensure that the IDE you choose can grow with you, supporting new features and compliance with the latest C++ standards.
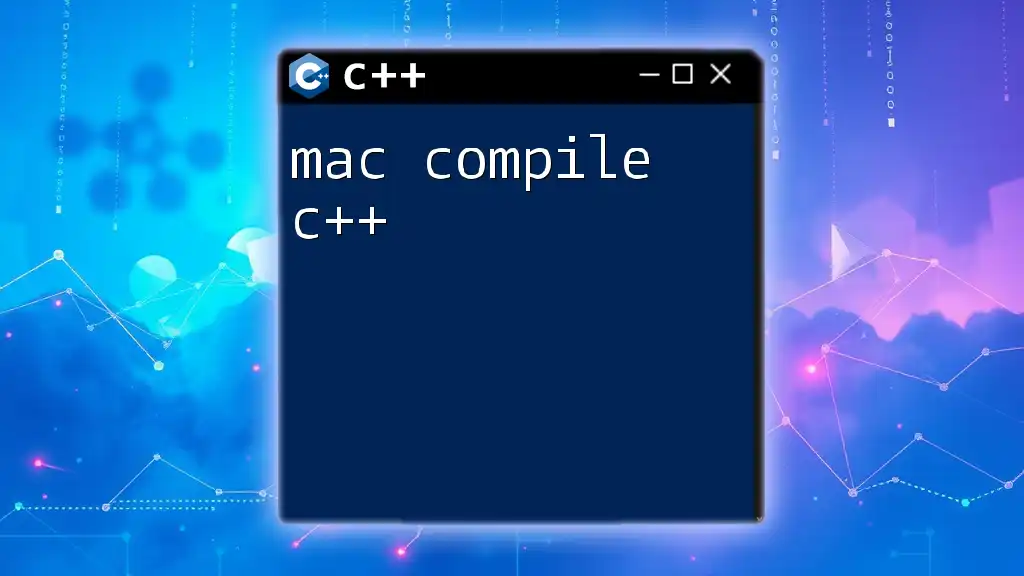
Conclusion
Selecting the right mac C++ IDE is a foundational step in your journey to becoming a proficient C++ developer. Whether you choose Xcode for its native capabilities, CLion for advanced features, or Visual Studio Code for lightweight development, each IDE offers unique benefits. Take the time to explore each option, adjust to your workflow, and enhance your coding experience. The right choice will ultimately make your coding journey smoother and more productive.
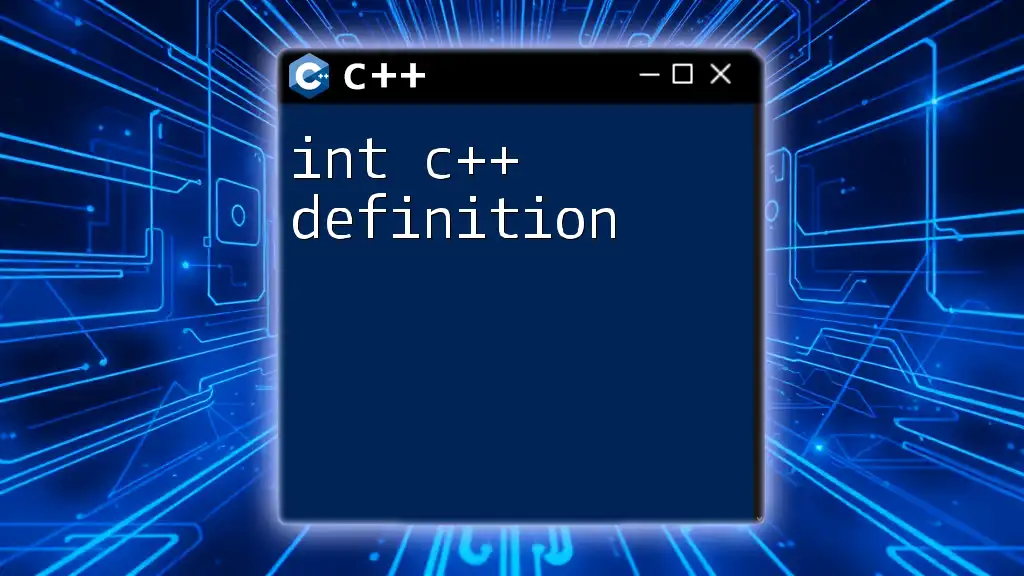
Additional Resources
Explore official documentation for each IDE to dive deeper into features and functionalities. Join community forums and browse tutorials to unlock the full potential of the tools at your disposal. Additionally, consider reading renowned C++ programming books or enrolling in online courses for a more structured learning approach.
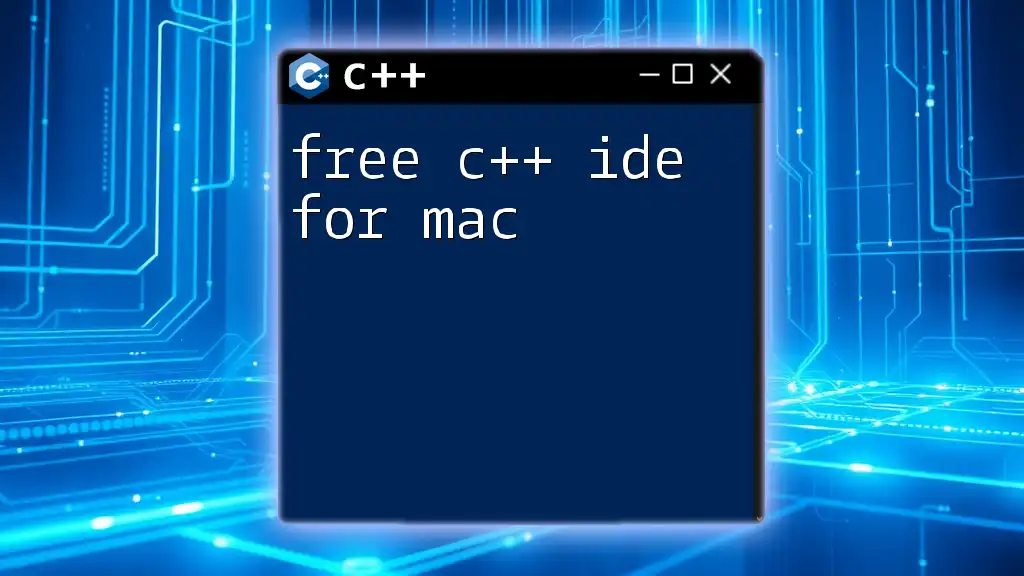
FAQ on C++ IDEs for Mac
As you immerse yourself in C++ development using these IDEs, you may encounter common questions about setup and usage. Mastering these often-overlooked tips can save you valuable time and enhance your programming experience.