A C++ demangler is a tool that converts mangled C++ symbols (created during compilation) back into their human-readable form, making it easier to understand function and variable names in error messages and debugging.
Here's a simple example of using the `c++filt` demangler tool:
echo "_Z3fooi" | c++filt
This command will output:
foo(int)
What is Demangling?
Demangling refers to the process of converting mangled names, which are the encoded representations of function, variable, or class names produced by C++ compilers, back into human-readable format.
Why You Need a C++ Demangler
In C++, names may be mangled to support function overloading, namespaces, and templates, which allows the same name to be reused in different contexts. However, this can make it challenging for developers to read error messages or understand the stack traces during debugging. A C++ demangler helps make sense of these mangled names, improving code readability and simplifying debugging efforts.
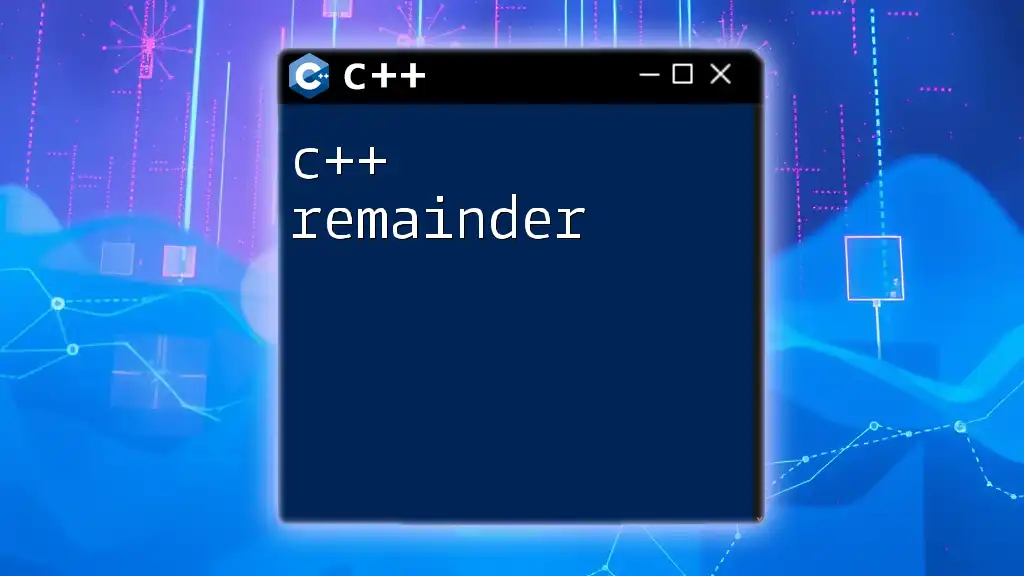
Understanding Mangled Names in C++
What Causes Name Mangling?
Name mangling occurs when the C++ compiler generates unique names for functions and variables to manage overloading and maintain scope visibility. This means that the same function name can appear in different contexts, and the compiler must ensure each version is distinct when generating the final binary.
Examples of Mangled Names
Consider the following C++ function:
void myFunction(int, double) { /* implementation */ }
The mangled name might look something like `_Z12myFunctionid`. Here, the encoding provides context on the function's name and parameters. After demangling, it becomes clear that it references the original function signature.
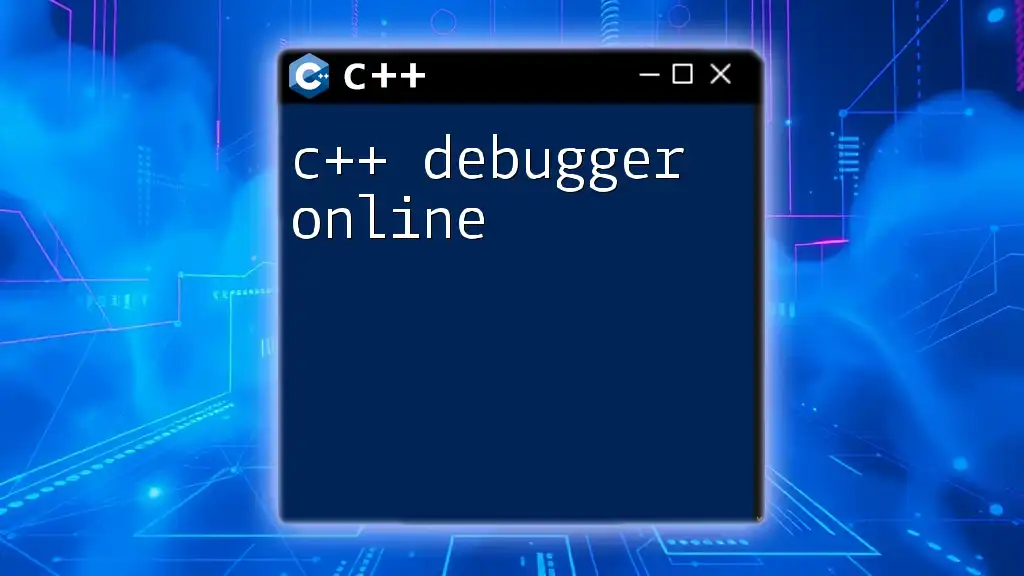
The Role of C++ Demanglers
What Does a C++ Demangler Do?
A C++ demangler translates these mangled names back to their original, human-readable form. This is crucial when you want to analyze compiler error messages, debug applications, or read symbol tables.
Common Use Cases for C++ Demanglers
C++ demanglers have several practical applications:
-
Analyzing error messages from the compiler: When you receive an error about an undefined reference, the mangled name often appears in the error message. Demangling it can reveal the actual function or class name.
-
Working with symbol tables: Debugging tools often use symbol tables containing mangled names. Demanglers make this information accessible.
-
Debugging with tools like GDB: When diving deep into a program using GDB, demangled names help clarify what functions or classes you are inspecting.
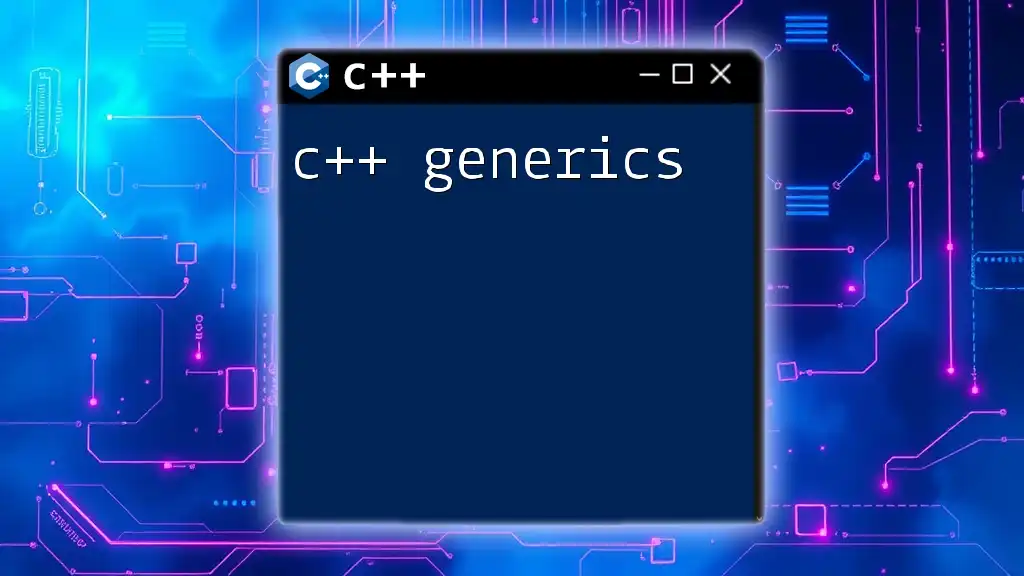
Tools and Libraries for Demangling
GNU C++ Demangler
One of the most accessible tools for demangling is the `c++filt` command included with GNU binutils.
To use it, you can run:
echo "_Z12myFunctionid" | c++filt
This command outputs the demangled name: `void myFunction(int, double)`.
Demangling in Microsoft Visual C++
For Windows users, the `undname` tool can be utilized. To demangle a name like `?myFunction@@YAXHNN@Z`, simply run:
undname ?myFunction@@YAXHNN@Z
Online Demangling Tools
There are several online platforms that offer demangling services. You can paste your mangled names, and these tools will instantly convert them into readable formats. Popular websites include:
- [Demangler.com](https://demangler.com)
- [C++filt.com](https://www.cppfilt.com)
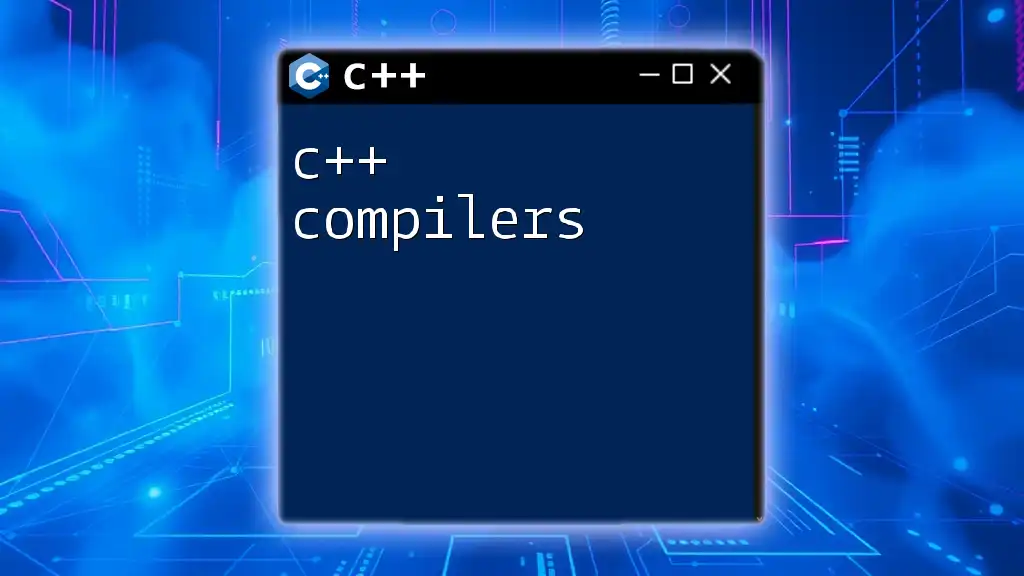
Writing Your Own C++ Demangler
Exploring the Demangling Algorithms
If you're interested in how demangling works under the hood, it's worth exploring the algorithms that parse mangled names. Demangling typically involves breaking down the mangled name into its constituents, like namespaces, classes, and function parameters.
Basic Implementation in C++
Here’s a simple example to start with the implementation:
#include <iostream>
// Example of a simple demangler function
std::string demangle(const std::string& mangled) {
// Implementation logic here
return ""; // Placeholder for demangled string
}
int main() {
std::string mangledName = "_Z12myFunctionid";
std::cout << "Demangled: " << demangle(mangledName) << std::endl;
return 0;
}
This is a basic structure, and you'd fill in the actual demangling logic to convert the mangled input into its original format.
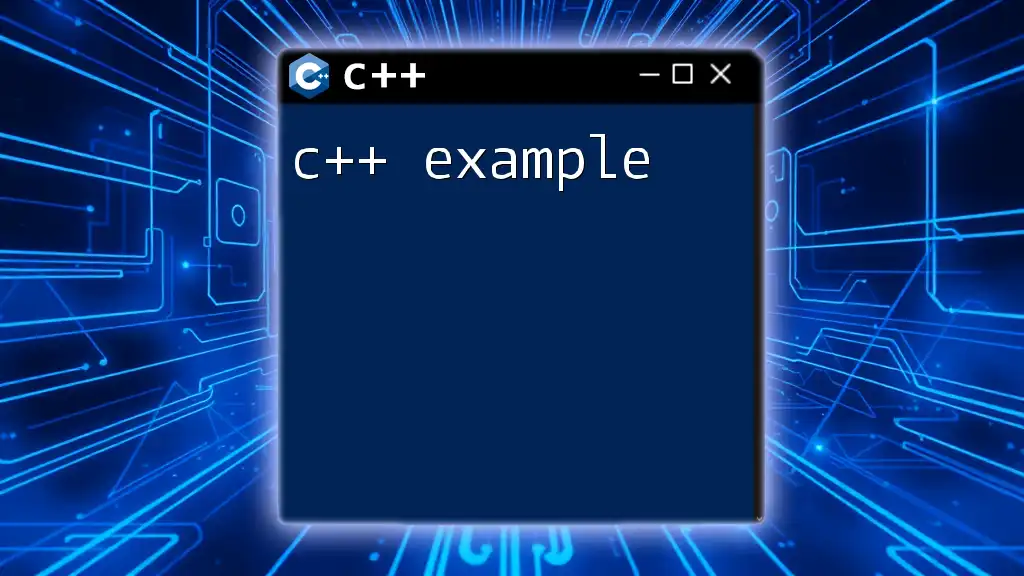
Challenges in C++ Demangling
Common Pitfalls and Issues
When working with C++ demanglers, be aware of the following:
-
Compiler-specific mangling: Different compilers may produce varying mangled names for the same function, which can complicate demangling efforts.
-
Handling complex templates and namespaces: These scenarios can result in exceptionally intricate mangled names that may be harder to demangle accurately.
Best Practices for Effective Demangling
To make the most of your demangling efforts, follow these best practices:
- Use the right tools for your specific compiler to ensure accuracy.
- When debugging, demangle any mangled names reported in error messages for clearer insights.
- Practice demangling with various examples to become familiar with different naming schemes.
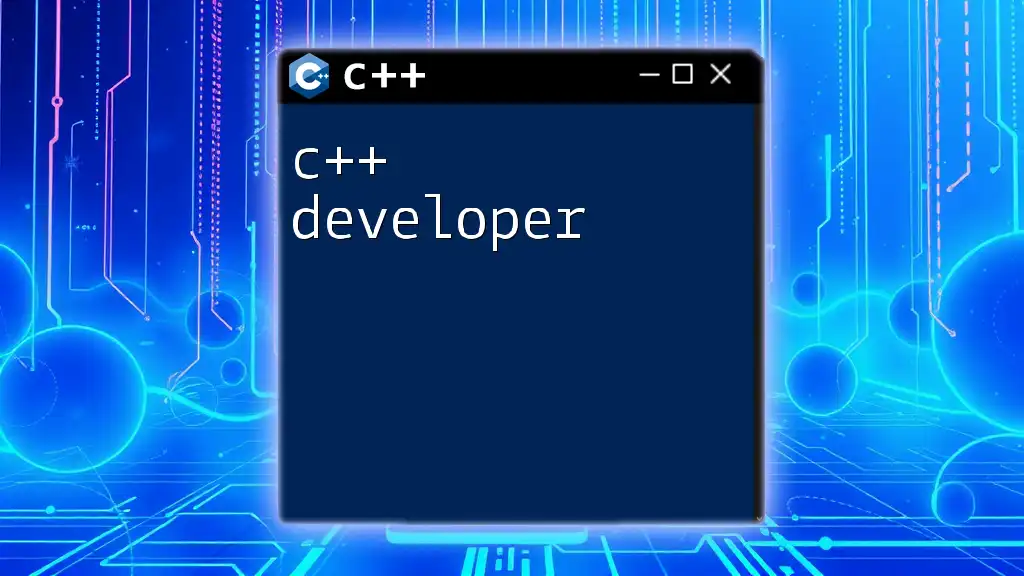
Conclusion
Using a C++ demangler is a vital practice for improving code readability and enhancing debugging capabilities. It allows developers to make sense of complicated error messages and function references. With a deeper understanding of name mangling and the tools available, you can effectively leverage demangling techniques in your C++ development journey.
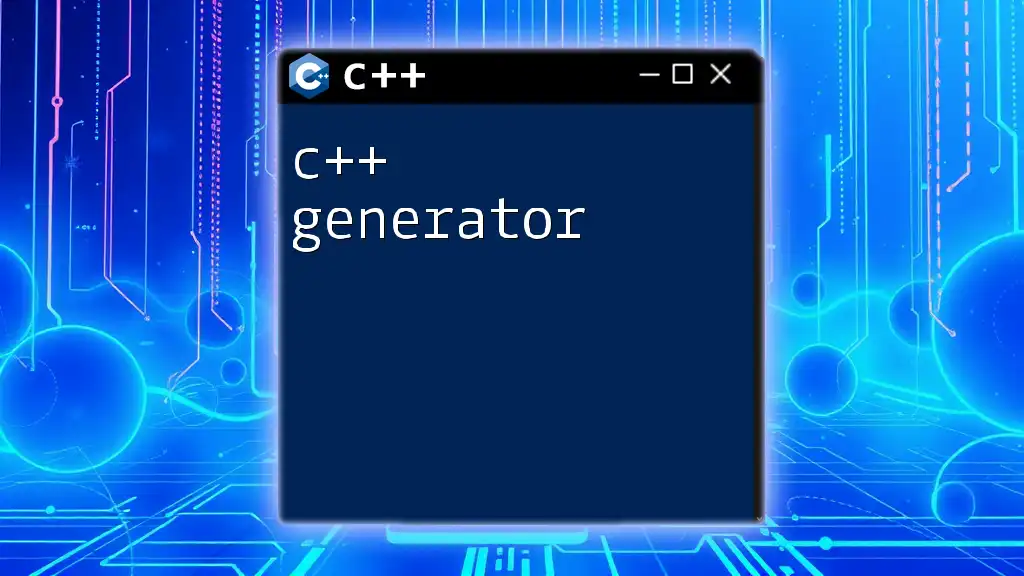
Additional Resources
Books and Documentation
- "The C++ Programming Language" by Bjarne Stroustrup: A comprehensive resource to learn C++ best practices.
- C++ Standard Library documentation: Useful for understanding standard features and functions.
Online Forums and Communities
Participating in forums, such as Stack Overflow or Reddit’s r/cpp, can be invaluable for learning from other developers’ experiences and seeking advice on specific challenges you encounter while working with demangling.