A C++ generic class allows you to define a class template that can operate with any data type, enhancing code reusability and flexibility.
Here’s a simple example:
template <typename T>
class Box {
private:
T item;
public:
void setItem(T newItem) {
item = newItem;
}
T getItem() const {
return item;
}
};
What is a Generic Class?
A generic class in C++ allows programmers to define a class without specifying the exact data types that it will operate on. This means that generic classes can work with any data type, lending them incredible flexibility and reusability. By leveraging generic programming, developers can write code that is type-independent, thus avoiding redundancy and promoting cleaner, easier to maintain code.
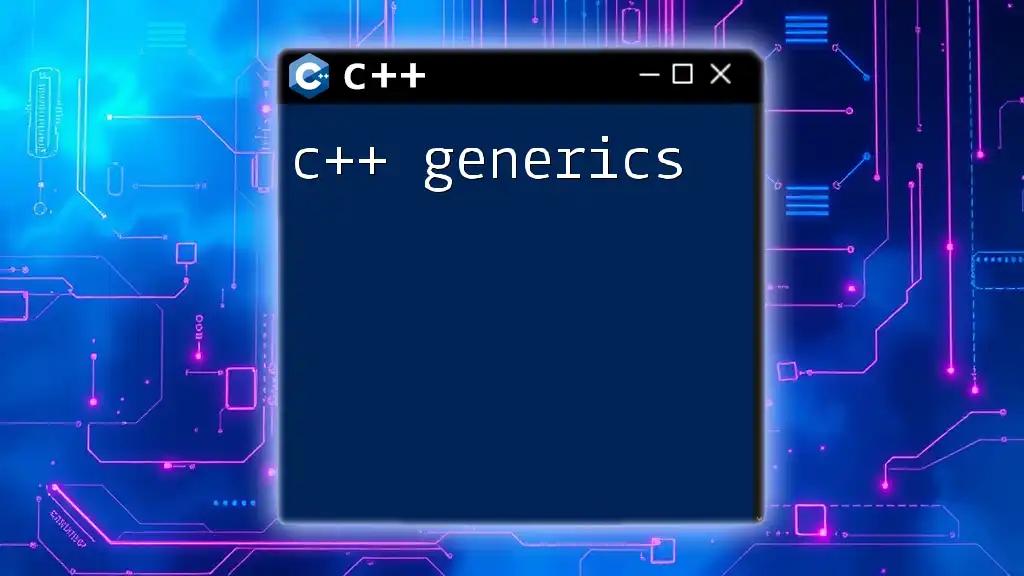
The Syntax of a Generic Class
Basic Structure
To define a generic class in C++, the `template` keyword is essential. This keyword introduces the template declaration, which is always followed by angle brackets containing one or more type parameters. Here's a breakdown of the syntax:
template <typename T>
class GenericClass {
public:
T data;
void display() {
std::cout << data << std::endl;
}
};
In this snippet:
- `template <typename T>` declares a template that takes a single type parameter, `T`.
- The class `GenericClass` can hold data of type `T` and has a member function `display()` that outputs the value of `data`.
Type Parameters
When using templates, there is a debate about the use of `typename` versus `class`. In the context of templates, both keywords can generally be used interchangeably. However, it’s worth noting:
- Use `typename` when you want to specifically indicate that you are declaring a type parameter.
- Use `class` if you prefer, though it may be less expressive in terms of intent.

Creating and Using Generic Classes
Instantiating Generic Classes
Creating instances of a generic class is straightforward. You specify the desired data type in angle brackets. Below, you will find an example showcasing the creation of instances with different data types:
GenericClass<int> intInstance;
intInstance.data = 10;
intInstance.display(); // Output: 10
GenericClass<std::string> stringInstance;
stringInstance.data = "Hello, C++!";
stringInstance.display(); // Output: Hello, C++!
In this code, `intInstance` is an instance of `GenericClass` with `int` as the type parameter, while `stringInstance` uses `std::string`. This demonstrates the flexibility of generic classes: the same class can manage different types of data seamlessly.
Advantages of Using Generic Classes
The use of generic classes offers several key advantages:
- Type Safety: By ensuring that operations on the data are type-checked at compile time, you reduce runtime errors.
- Reusability: One generic class can substitute for many specific classes, thereby enhancing code reusability and reducing duplication.
- Maintainability: It simplifies code modification because changes to a generic class automatically propagate to all instances.

Implementing More Complex Generic Classes
Generic Classes with Multiple Type Parameters
C++ allows for defining generic classes with more than one type parameter. This can be especially useful when representing relationships between different data types. Below is an example of a generic class that accepts two type parameters:
template <typename T1, typename T2>
class Pair {
public:
T1 first;
T2 second;
void show() {
std::cout << "First: " << first << ", Second: " << second << std::endl;
}
};
You can create instances of this class like so:
Pair<int, double> intDoublePair;
intDoublePair.first = 5;
intDoublePair.second = 3.14;
intDoublePair.show(); // Output: First: 5, Second: 3.14
This example illustrates how you can effectively hold different types of data together, enhancing the expressiveness of your designs.
Generic Classes with Member Functions
Generic classes can also have member functions that use the same or different template types. Below is an example of a member function that performs arithmetic operations:
template <typename T>
class Calculator {
public:
T add(T a, T b) {
return a + b;
}
};
You can instantiate the `Calculator` class and use its `add` function, as shown here:
Calculator<int> calc;
std::cout << calc.add(2, 3) << std::endl; // Output: 5
In this case, the `add` function accommodates any type which supports the `+` operator.

Special Use Cases of Generic Classes
Using Generic Classes with STL Containers
C++’s Standard Template Library (STL) provides a powerful set of generic classes and functions that can work great with user-defined generic classes. For instance, consider the following generic class that acts as a wrapper around `std::vector`:
template <typename T>
class VectorWrapper {
private:
std::vector<T> vec;
public:
void addElement(T element) {
vec.push_back(element);
}
void printElements() {
for (const auto& elem : vec) {
std::cout << elem << std::endl;
}
}
};
This combination allows you to store various elements in a dynamic array structure, maintaining type safety.
Handling Constraints in Generic Programming
Sometimes, you may want to impose specific constraints on the types that can be used as template parameters. C++ provides ways to enforce these constraints through static assertions. Here is an example:
template <typename T>
class NumericCheck {
static_assert(std::is_arithmetic<T>::value, "Template parameter must be a numeric type.");
public:
// Class implementation
};
The `static_assert` verifies that `T` is a numeric type at compile time, helping avoid potential errors in your code that could become challenging to diagnose.
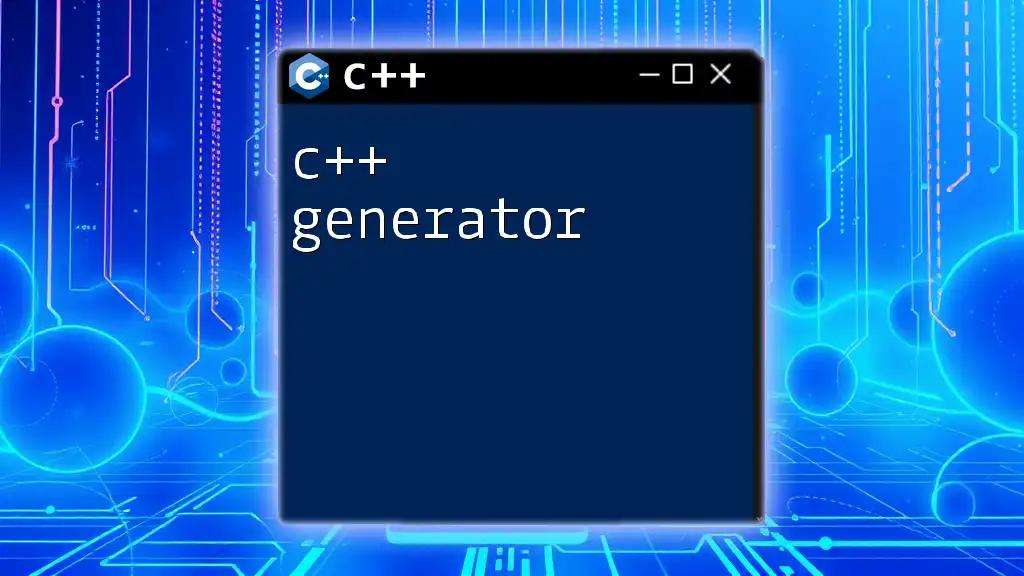
Conclusion
In summary, C++ generic classes promote a way to write cleaner, more maintainable, and reusable code. They encapsulate type independence, allowing developers to work flexibly across a variety of data types. As demonstrated, using generic classes with member functions and STL containers can expand their utility even further, making them a powerful tool in any C++ programmer's toolkit.
Encouraging exploration into these topics will undoubtedly enhance your programming skills and deepen your understanding of modern programming practices. Happy coding!
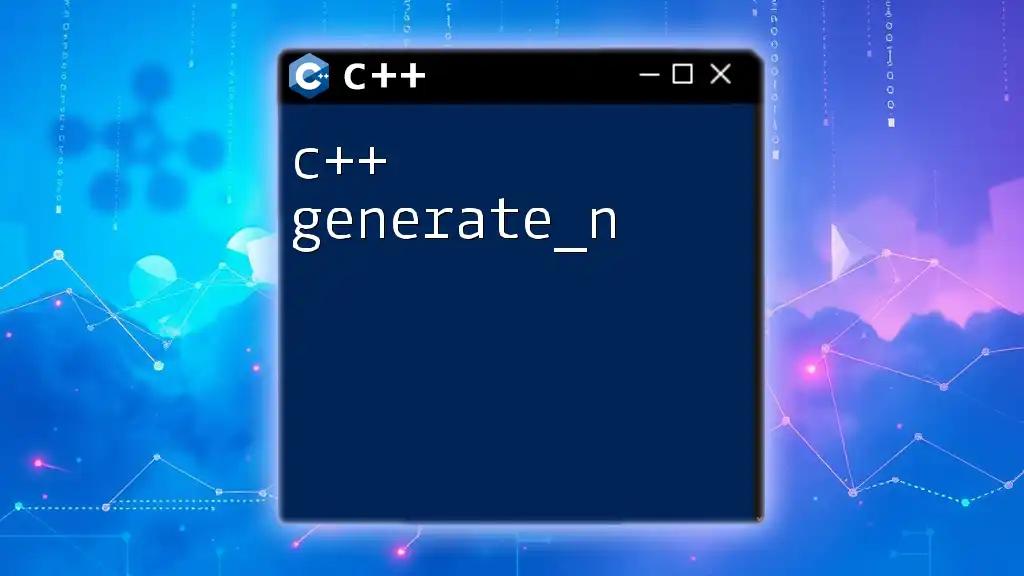
Additional Resources
As you continue your learning journey, consider exploring various resources such as books and online courses that delve further into C++ templates and generic programming concepts. Always strive to deepen your knowledge and apply these techniques in real-world projects!